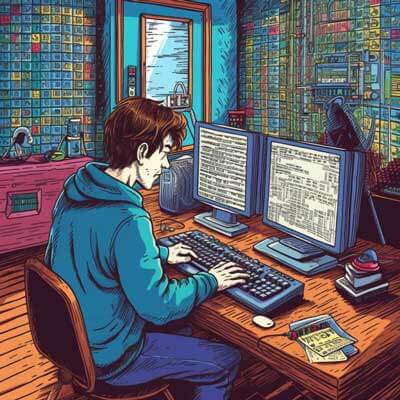
- Introduction to the Interview Process
- What to Expect in a Java Programming Interview
- Example Interview Question: Reverse a String
- Example Interview Question: Find the Maximum Element in an Array
- Interview Questions on Basic Concepts
- Understanding Java Variables and Data Types
- Understanding Control Flow Statements
- Interview Questions on Object-Oriented Principles
- Understanding Object-Oriented Concepts in Java
- Example Interview Question: Implementing Inheritance
- Example Interview Question: Implementing Polymorphism
- Use Case: Implementing Singleton Pattern
- Implementing a Singleton Class in Java
- Example Use Case: Logging Service as a Singleton
- Best Practice: Proper Use of Date and Time Classes
- Understanding the Date and Time API in Java
- Example Best Practice: Comparing Dates in Java
- Real World Example: Using Streams for Data Manipulation
- Introduction to Java Streams
- Performance Consideration: Choosing the Right Data Structure
- Choosing the Right Data Structure in Java
- Example Performance Consideration: ArrayList vs LinkedList
- Advanced Technique: Multi-threading and Concurrency
- Introduction to Multi-threading and Concurrency in Java
- Code Snippet Idea: Working with Arrays
- Working with Arrays in Java
- Code Snippet Idea: Exception Handling
- Exception Handling in Java
- Code Snippet Idea: Using Lambdas and Functional Interfaces
- Using Lambdas and Functional Interfaces in Java
- Code Snippet Idea: File I/O Operations
- File I/O Operations in Java
- Code Snippet Idea: JDBC Operations
- Performing JDBC Operations in Java
Introduction to the Interview Process
Related Article: How To Parse JSON In Java
What to Expect in a Java Programming Interview
In a Java programming interview, you can expect a combination of technical questions and coding exercises to assess your knowledge and skills in Java programming. The interview process may vary depending on the company and the position you are applying for. Here are some common types of questions you may encounter:
1. Conceptual Questions: These questions test your understanding of basic Java concepts such as inheritance, polymorphism, encapsulation, and abstraction. For example:
// Example of a conceptual question What is the difference between an abstract class and an interface in Java?
2. Coding Questions: These questions require you to write code to solve a specific problem or implement a given algorithm. You may be asked to write code on a whiteboard, in an online coding platform, or directly in an Integrated Development Environment (IDE). For example:
// Example of a coding question Write a Java program to find the factorial of a given number.
3. Design Questions: These questions assess your ability to design and implement complex software systems. You may be asked to design a class hierarchy, a database schema, or a system architecture. For example:
// Example of a design question Design a parking lot system using object-oriented principles in Java.
4. Behavioral Questions: These questions aim to understand your problem-solving skills, teamwork abilities, and how you handle challenges in a professional setting. For example:
// Example of a behavioral question Tell me about a time when you had to deal with a difficult team member and how you handled the situation.
Example Interview Question: Reverse a String
Here’s an example of a common interview question that tests your understanding of basic string manipulation in Java:
// Example of a coding question: Reverse a String public class ReverseString { public static String reverse(String str) { StringBuilder sb = new StringBuilder(); for (int i = str.length() - 1; i >= 0; i--) { sb.append(str.charAt(i)); } return sb.toString(); } public static void main(String[] args) { String original = "Hello, World!"; String reversed = reverse(original); System.out.println(reversed); } }
In this example, the reverse
method takes a string as input and uses a StringBuilder
to append the characters in reverse order. The main
method demonstrates how to use the reverse
method to reverse the string “Hello, World!” and print the result.
Example Interview Question: Find the Maximum Element in an Array
Another common interview question in Java is finding the maximum element in an array. Here’s an example solution:
// Example of a coding question: Find the Maximum Element in an Array public class FindMaximumElement { public static int findMax(int[] array) { int max = Integer.MIN_VALUE; for (int num : array) { if (num > max) { max = num; } } return max; } public static void main(String[] args) { int[] numbers = {5, 10, 3, 8, 15}; int maxNumber = findMax(numbers); System.out.println("The maximum number is: " + maxNumber); } }
In this example, the findMax
method takes an array of integers as input and iterates through the array to find the maximum element. The main
method demonstrates how to use the findMax
method to find the maximum number in the array [5, 10, 3, 8, 15] and print the result.
Related Article: How To Convert Array To List In Java
Interview Questions on Basic Concepts
Understanding Java Variables and Data Types
In Java, variables are used to store data of different types. Understanding variable declaration, initialization, and data types is essential for Java programming. Let’s explore some interview questions that test your knowledge of these concepts.
1. What is the difference between a primitive data type and a reference data type in Java?
2. Explain the difference between int
and Integer
in Java.
3. What is the default value of an int
variable in Java?
4. How do you declare and initialize a constant variable in Java?
5. What are the different types of numeric data types in Java? Provide examples.
Let’s take a look at an example that demonstrates variable declaration and initialization:
// Example of variable declaration and initialization public class VariableExample { public static void main(String[] args) { int age = 25; double height = 1.75; boolean isStudent = true; char grade = 'A'; String name = "John Doe"; System.out.println("Name: " + name); System.out.println("Age: " + age); System.out.println("Height: " + height); System.out.println("Is Student: " + isStudent); System.out.println("Grade: " + grade); } }
In this example, we declare and initialize variables of different types, such as int
, double
, boolean
, char
, and String
. The main
method then prints the values of these variables.
Understanding Control Flow Statements
Control flow statements are used to control the execution flow of a Java program. Mastering control flow statements is crucial for writing efficient and correct programs. Here are some interview questions related to control flow statements:
1. What is the difference between if
, else if
, and else
statements in Java?
2. Explain the purpose and usage of the switch
statement in Java.
3. What is the difference between a for
loop and a while
loop in Java?
4. How do you exit a loop prematurely in Java?
5. Describe the purpose and usage of the break
and continue
statements in Java.
Let’s take a look at an example that demonstrates the usage of control flow statements:
// Example of control flow statements public class ControlFlowExample { public static void main(String[] args) { int age = 18; if (age < 18) { System.out.println("You are not eligible to vote."); } else if (age >= 18 && age <= 65) { System.out.println("You are eligible to vote."); } else { System.out.println("You are eligible for senior citizen benefits."); } int number = 5; switch (number) { case 1: System.out.println("Number is 1."); break; case 2: System.out.println("Number is 2."); break; default: System.out.println("Number is neither 1 nor 2."); break; } for (int i = 0; i < 5; i++) { if (i == 3) { continue; } System.out.println(i); } } }
In this example, we demonstrate the usage of if
, else if
, and else
statements to determine voting eligibility based on age. We also use a switch
statement to print different messages based on the value of a number. Finally, we use a for
loop to print numbers from 0 to 4, excluding the number 3 using the continue
statement.
Related Article: How To Iterate Over Entries In A Java Map
Interview Questions on Object-Oriented Principles
Understanding Object-Oriented Concepts in Java
Object-oriented programming (OOP) is a fundamental concept in Java. Understanding the principles of OOP is crucial for writing modular and maintainable code. Let’s explore some interview questions related to OOP principles in Java.
1. What are the four main principles of OOP? Explain each principle.
2. What is the difference between a class and an object in Java?
3. How do you achieve code reusability in Java through inheritance and composition?
4. Explain the purpose and usage of access modifiers in Java.
5. Describe the concept of encapsulation and its benefits in Java.
Let’s take a look at an example that demonstrates the usage of OOP principles:
// Example of OOP principles public class OOPExample { public static void main(String[] args) { // Creating objects of different classes Circle circle = new Circle(5); Rectangle rectangle = new Rectangle(4, 6); // Accessing methods of objects double circleArea = circle.calculateArea(); double rectangleArea = rectangle.calculateArea(); // Printing the calculated areas System.out.println("Circle Area: " + circleArea); System.out.println("Rectangle Area: " + rectangleArea); } } // Circle class class Circle { private double radius; public Circle(double radius) { this.radius = radius; } public double calculateArea() { return Math.PI * radius * radius; } } // Rectangle class class Rectangle { private double length; private double width; public Rectangle(double length, double width) { this.length = length; this.width = width; } public double calculateArea() { return length * width; } }
In this example, we demonstrate the usage of OOP principles by creating two classes, Circle
and Rectangle
, representing geometric shapes. Each class has its own properties and methods. We create objects of these classes and access their methods to calculate the areas of a circle and a rectangle. The calculated areas are then printed to the console.
Example Interview Question: Implementing Inheritance
Inheritance is a key feature of object-oriented programming that allows classes to inherit properties and methods from other classes. Here’s an example question that tests your understanding of inheritance in Java:
// Example of an interview question: Implementing Inheritance public class Animal { protected String name; public Animal(String name) { this.name = name; } public void eat() { System.out.println(name + " is eating."); } public void sleep() { System.out.println(name + " is sleeping."); } } public class Dog extends Animal { public Dog(String name) { super(name); } public void bark() { System.out.println(name + " is barking."); } public static void main(String[] args) { Dog dog = new Dog("Buddy"); dog.eat(); dog.sleep(); dog.bark(); } }
In this example, we have an Animal
class with a name
property and eat
and sleep
methods. The Dog
class extends the Animal
class, inheriting the name
property and methods. It also adds a bark
method specific to dogs. In the main
method, we create a Dog
object and call the inherited methods (eat
and sleep
) as well as the dog-specific method (bark
).
Related Article: How To Split A String In Java
Example Interview Question: Implementing Polymorphism
Polymorphism is another important concept in object-oriented programming that allows objects of different classes to be treated as objects of a common superclass. Here’s an example question that tests your understanding of polymorphism in Java:
// Example of an interview question: Implementing Polymorphism public class Shape { public void draw() { System.out.println("Drawing a shape."); } } public class Circle extends Shape { @Override public void draw() { System.out.println("Drawing a circle."); } } public class Rectangle extends Shape { @Override public void draw() { System.out.println("Drawing a rectangle."); } } public class PolymorphismExample { public static void main(String[] args) { Shape shape1 = new Circle(); Shape shape2 = new Rectangle(); shape1.draw(); shape2.draw(); } }
In this example, we have a Shape
class with a draw
method. The Circle
and Rectangle
classes extend the Shape
class and override the draw
method to provide their specific implementations. In the main
method, we create objects of Circle
and Rectangle
and assign them to Shape
variables. By calling the draw
method on these variables, we achieve polymorphic behavior, where the appropriate draw
method implementation is called based on the actual object type.
Use Case: Implementing Singleton Pattern
Implementing a Singleton Class in Java
The singleton pattern is a creational design pattern that ensures a class has only one instance and provides a global point of access to it. Let’s explore how to implement a singleton class in Java.
1. Create a private constructor to prevent direct instantiation of the class.
2. Declare a private static variable to hold the single instance of the class.
3. Provide a public static method that returns the single instance of the class.
4. Use lazy initialization to create the instance only when it is requested for the first time.
5. Ensure thread safety when creating the instance in a multi-threaded environment.
Here’s an example implementation of a singleton class in Java:
// Example of implementing a singleton class public class Singleton { private static Singleton instance; private Singleton() { // Private constructor to prevent direct instantiation } public static Singleton getInstance() { if (instance == null) { synchronized (Singleton.class) { if (instance == null) { instance = new Singleton(); } } } return instance; } // Other methods and properties }
In this example, the Singleton
class has a private constructor to prevent direct instantiation. The getInstance
method returns the single instance of the class, creating it if necessary using lazy initialization and thread-safe double-checked locking.
Related Article: How To Convert Java Objects To JSON With Jackson
Example Use Case: Logging Service as a Singleton
A common use case for the singleton pattern is a logging service that needs to be accessed globally throughout an application. Here’s an example of how to implement a logging service as a singleton in Java:
// Example of a logging service implemented as a singleton public class Logger { private static Logger instance; private Logger() { // Private constructor to prevent direct instantiation } public static Logger getInstance() { if (instance == null) { synchronized (Logger.class) { if (instance == null) { instance = new Logger(); } } } return instance; } public void log(String message) { System.out.println("[LOG] " + message); } // Other methods and properties }
In this example, the Logger
class is implemented as a singleton. The getInstance
method ensures that only one instance of the Logger
class is created and returned. The log
method can be used to log messages throughout the application.
To use the logging service, you can call the getInstance
method to obtain the singleton instance and then call the log
method to log messages:
Logger logger = Logger.getInstance(); logger.log("Application started."); logger.log("Processing data..."); logger.log("Application finished.");
This approach ensures that the logging service is accessible globally and ensures that only one instance of the Logger
class is used throughout the application.
Best Practice: Proper Use of Date and Time Classes
Understanding the Date and Time API in Java
The Java Date and Time API, introduced in Java 8, provides a comprehensive set of classes for working with dates, times, time zones, durations, and periods. Properly using the Date and Time API is important to ensure accurate and efficient date and time manipulation in Java. Let’s explore some best practices for using the Date and Time API.
1. Use the java.time
package classes for date and time manipulation, such as LocalDate
, LocalTime
, LocalDateTime
, ZonedDateTime
, etc.
2. Avoid using the deprecated java.util.Date
and java.util.Calendar
classes.
3. Be aware of time zone considerations and use appropriate methods like withZoneSameInstant
and withZoneSameLocal
.
4. Use the java.time.format.DateTimeFormatter
class for parsing and formatting date and time strings.
5. Take advantage of the various utility methods provided by the Date and Time API, such as plus
, minus
, isBefore
, isAfter
, isEqual
, etc.
Let’s take a look at an example that demonstrates the proper use of the Date and Time API:
// Example of proper use of the Date and Time API import java.time.LocalDate; import java.time.LocalDateTime; import java.time.LocalTime; import java.time.format.DateTimeFormatter; public class DateAndTimeExample { public static void main(String[] args) { LocalDate currentDate = LocalDate.now(); LocalTime currentTime = LocalTime.now(); LocalDateTime currentDateTime = LocalDateTime.now(); System.out.println("Current Date: " + currentDate); System.out.println("Current Time: " + currentTime); System.out.println("Current Date and Time: " + currentDateTime); DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss"); String formattedDateTime = currentDateTime.format(formatter); System.out.println("Formatted Date and Time: " + formattedDateTime); } }
In this example, we use the java.time.LocalDate
, java.time.LocalTime
, and java.time.LocalDateTime
classes to get the current date, time, and date-time respectively. We then demonstrate the usage of the DateTimeFormatter
class to format the date and time as a string.
Related Article: Storing Contact Information in Java Data Structures
Example Best Practice: Comparing Dates in Java
When comparing dates in Java, it is important to consider the time zone and use appropriate methods to ensure accurate results. Here’s an example of a best practice for comparing dates in Java:
// Example of comparing dates in Java import java.time.LocalDate; public class DateComparisonExample { public static void main(String[] args) { LocalDate date1 = LocalDate.of(2022, 1, 1); LocalDate date2 = LocalDate.of(2022, 1, 2); if (date1.isBefore(date2)) { System.out.println(date1 + " is before " + date2); } else if (date1.isAfter(date2)) { System.out.println(date1 + " is after " + date2); } else { System.out.println(date1 + " is equal to " + date2); } } }
In this example, we create two LocalDate
objects representing different dates. We then use the isBefore
, isAfter
, and isEqual
methods to compare the dates and print the appropriate message.
By using the proper comparison methods provided by the Date and Time API, we ensure accurate and reliable date comparisons in Java.
Real World Example: Using Streams for Data Manipulation
Introduction to Java Streams
Java Streams provide a powerful and concise way to manipulate collections of data in a functional programming style. Streams allow you to perform operations such as filtering, mapping, reducing, and sorting on collections with ease. Let’s explore some commonly used stream operations and their syntax.
1. Filtering: Use the filter
method to select elements based on a given condition.
stream.filter(element -> element > 5)
2. Mapping: Use the map
method to transform elements based on a given function.
stream.map(element -> element * 2)
3. Reducing: Use the reduce
method to perform an operation on all elements of the stream and return a single result.
stream.reduce(0, (accumulator, element) -> accumulator + element)
4. Sorting: Use the sorted
method to sort the elements of the stream based on a given comparator.
stream.sorted()
Let’s take a look at an example that demonstrates the usage of streams for data manipulation:
// Example of using streams for data manipulation import java.util.Arrays; import java.util.List; public class StreamExample { public static void main(String[] args) { List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10); // Filter even numbers List<Integer> evenNumbers = numbers.stream() .filter(number -> number % 2 == 0) .toList(); System.out.println("Even Numbers: " + evenNumbers); // Map to square of each number List<Integer> squaredNumbers = numbers.stream() .map(number -> number * number) .toList(); System.out.println("Squared Numbers: " + squaredNumbers); // Reduce to find the sum of all numbers int sum = numbers.stream() .reduce(0, (accumulator, number) -> accumulator + number); System.out.println("Sum: " + sum); // Sort the numbers in descending order List<Integer> sortedNumbers = numbers.stream() .sorted((a, b) -> b.compareTo(a)) .toList(); System.out.println("Sorted Numbers: " + sortedNumbers); } }
In this example, we have a list of numbers and we use streams to perform various operations on the list. We filter the even numbers, map each number to its square, reduce the numbers to find their sum, and sort the numbers in descending order using streams.
Streams provide a concise and expressive way to manipulate data in Java, making your code more readable and maintainable.
Related Article: How to Convert JSON String to Java Object
Performance Consideration: Choosing the Right Data Structure
Choosing the Right Data Structure in Java
Choosing the right data structure is crucial for achieving optimal performance in your Java programs. Different data structures have different characteristics and trade-offs in terms of memory usage, access time, and insert/delete operations. Let’s explore some commonly used data structures in Java and their performance considerations.
1. ArrayList: Use when you need fast access to elements by index and frequent modifications at the end of the list. Insertion and deletion at arbitrary positions are slower.
2. LinkedList: Use when you need fast insertion and deletion at arbitrary positions, but access by index is slower.
3. HashSet: Use when you need fast insertion, deletion, and lookup of unique elements, but the order of elements is not important.
4. TreeSet: Use when you need elements to be sorted in a natural order or a custom order defined by a comparator. Insertion, deletion, and lookup are slower compared to HashSet.
5. HashMap: Use when you need fast insertion, deletion, and lookup of key-value pairs. Order of elements is not important.
6. TreeMap: Use when you need key-value pairs to be sorted in a natural order or a custom order defined by a comparator. Insertion, deletion, and lookup are slower compared to HashMap.
Consider the requirements of your program, such as the type and volume of data, the frequency of operations, and the desired performance characteristics, to choose the most appropriate data structure.
Example Performance Consideration: ArrayList vs LinkedList
The choice between an ArrayList and a LinkedList depends on the specific requirements of your program. Here’s an example that compares the performance of ArrayList and LinkedList for different operations:
// Example of performance comparison between ArrayList and LinkedList import java.util.ArrayList; import java.util.LinkedList; import java.util.List; public class ListPerformanceExample { public static void main(String[] args) { int size = 1000000; // ArrayList performance List<Integer> arrayList = new ArrayList<>(); long arrayListStart = System.currentTimeMillis(); for (int i = 0; i < size; i++) { arrayList.add(i); } long arrayListEnd = System.currentTimeMillis(); long arrayListTime = arrayListEnd - arrayListStart; System.out.println("ArrayList insertion time: " + arrayListTime + "ms"); // LinkedList performance List<Integer> linkedList = new LinkedList<>(); long linkedListStart = System.currentTimeMillis(); for (int i = 0; i < size; i++) { linkedList.add(i); } long linkedListEnd = System.currentTimeMillis(); long linkedListTime = linkedListEnd - linkedListStart; System.out.println("LinkedList insertion time: " + linkedListTime + "ms"); } }
In this example, we compare the performance of ArrayList and LinkedList for inserting a large number of elements. We measure the time taken for each operation and print the results.
The performance of ArrayList is generally better than LinkedList for random access by index, but LinkedList performs better for frequent insertions and deletions at arbitrary positions. Consider the specific requirements of your program when choosing between ArrayList and LinkedList.
Related Article: How to Retrieve Current Date and Time in Java
Advanced Technique: Multi-threading and Concurrency
Introduction to Multi-threading and Concurrency in Java
Multi-threading and concurrency are advanced techniques in Java that allow you to execute multiple tasks simultaneously and efficiently utilize system resources. Java provides built-in support for multi-threading and concurrency through the java.lang.Thread
class and the java.util.concurrent
package. Let’s explore some concepts and techniques related to multi-threading and concurrency.
1. Threads: A thread is a lightweight unit of execution that can run concurrently with other threads in a program. You can create and manage threads using the Thread
class or by implementing the Runnable
interface.
2. Synchronization: Synchronization is the technique used to control access to shared resources in a multi-threaded environment. The synchronized
keyword and the java.util.concurrent.locks
package provide mechanisms for synchronization.
3. Thread Pools: A thread pool is a collection of pre-initialized threads that are ready to perform tasks. Using a thread pool can improve performance by reusing threads instead of creating new ones.
4. Thread Safety: Thread safety refers to the property of a program or system that guarantees correct behavior when executed concurrently by multiple threads.
5. Concurrent Collections: The java.util.concurrent
package provides thread-safe versions of commonly used collections, such as ConcurrentHashMap
and ConcurrentLinkedQueue
, that can be safely accessed by multiple threads without explicit synchronization.
Let’s take a look at an example that demonstrates the usage of multi-threading and concurrency in Java:
// Example of multi-threading and concurrency in Java import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; public class ConcurrencyExample { public static void main(String[] args) { // Create a thread pool with 5 threads ExecutorService executor = Executors.newFixedThreadPool(5); // Submit tasks to the thread pool for (int i = 0; i < 10; i++) { Runnable task = new Task(i); executor.submit(task); } // Shutdown the thread pool executor.shutdown(); } } class Task implements Runnable { private int taskId; public Task(int taskId) { this.taskId = taskId; } @Override public void run() { System.out.println("Task " + taskId + " is executing."); // Perform the task... } }
In this example, we create a thread pool with 5 threads using the Executors.newFixedThreadPool
method. We then submit 10 tasks to the thread pool, each represented by an instance of the Task
class that implements the Runnable
interface. The tasks are executed concurrently by the threads in the thread pool.
Using multi-threading and concurrency can improve the performance and responsiveness of your Java programs, especially when dealing with computationally intensive or I/O-bound tasks.
Code Snippet Idea: Working with Arrays
Related Article: How to Reverse a String in Java
Working with Arrays in Java
Arrays are a fundamental data structure in Java that allow you to store and manipulate collections of elements of the same type. Understanding how to work with arrays is essential for many programming tasks. Let’s explore some common operations and techniques related to arrays in Java.
1. Declaring and Initializing Arrays: Use the square brackets []
to declare an array variable and the new
keyword to allocate memory for the array. Arrays can be initialized with literal values or dynamically populated at runtime.
2. Accessing Array Elements: Use the index value within square brackets to access individual elements of an array. The index starts from 0 for the first element.
3. Iterating over Arrays: Use loops, such as for
or foreach
, to iterate over the elements of an array and perform operations on them.
4. Sorting Arrays: Use the java.util.Arrays
class to sort arrays in ascending or descending order using methods like sort
.
5. Searching Arrays: Use methods like binarySearch
in the java.util.Arrays
class to search for a specific element in a sorted array.
6. Copying Arrays: Use methods like clone
or copyOf
in the java.util.Arrays
class to create copies of arrays.
Let’s take a look at an example that demonstrates these operations and techniques:
// Example of working with arrays in Java import java.util.Arrays; public class ArrayExample { public static void main(String[] args) { // Declaring and initializing an array int[] numbers = {5, 2, 8, 1, 9}; // Accessing array elements int firstNumber = numbers[0]; System.out.println("First Number: " + firstNumber); // Iterating over array elements for (int number : numbers) { System.out.println(number); } // Sorting the array in ascending order Arrays.sort(numbers); System.out.println("Sorted Numbers: " + Arrays.toString(numbers)); // Searching for an element in the sorted array int index = Arrays.binarySearch(numbers, 8); System.out.println("Index of 8: " + index); // Creating a copy of the array int[] copy = Arrays.copyOf(numbers, numbers.length); System.out.println("Copy of Numbers: " + Arrays.toString(copy)); } }
In this example, we declare and initialize an array of numbers. We then demonstrate accessing individual elements, iterating over the elements, sorting the array, searching for an element, and creating a copy of the array using various methods provided by the java.util.Arrays
class.
Arrays are a versatile and widely used data structure in Java, and mastering array manipulation is essential for many programming tasks.
Code Snippet Idea: Exception Handling
Exception Handling in Java
Exception handling is a critical aspect of programming in Java that allows you to gracefully handle errors and unexpected situations. Understanding how to handle exceptions is essential for writing robust and reliable code. Let’s explore some common exception handling techniques in Java.
1. Try-Catch Blocks: Use try-catch blocks to catch and handle exceptions that may be thrown by a block of code. The catch block specifies the type of exception to catch and the code to handle the exception.
2. Multiple Catch Blocks: Use multiple catch blocks to handle different types of exceptions separately. Catch blocks are evaluated in order, and the first matching catch block is executed.
3. Finally Block: Use a finally block to specify code that should be executed regardless of whether an exception occurred or not. The finally block is executed even if an exception is thrown, and it is used to release resources or perform cleanup tasks.
4. Throwing Exceptions: Use the throw
keyword to manually throw an exception. This is useful when you need to indicate an error condition or propagate an exception to a higher level.
5. Custom Exceptions: You can define your own custom exception classes by extending the Exception
class or one of its subclasses. Custom exceptions allow you to handle specific error conditions in a more meaningful way.
Let’s take a look at an example that demonstrates these exception handling techniques:
// Example of exception handling in Java import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; public class ExceptionExample { public static void main(String[] args) { BufferedReader reader = null; try { reader = new BufferedReader(new FileReader("file.txt")); String line; while ((line = reader.readLine()) != null) { System.out.println(line); } } catch (IOException e) { System.out.println("An error occurred: " + e.getMessage()); } finally { try { if (reader != null) { reader.close(); } } catch (IOException e) { System.out.println("An error occurred while closing the file: " + e.getMessage()); } } } }
In this example, we use a try-catch-finally block to handle exceptions that may occur when reading from a file. The try block contains the code that may throw an exception, and the catch block catches and handles the exception. The finally block ensures that the file is closed even if an exception occurs.
Exception handling allows you to gracefully handle errors and ensure that your program behaves correctly even in the presence of exceptions.
Related Article: How to Generate Random Integers in a Range in Java
Code Snippet Idea: Using Lambdas and Functional Interfaces
Using Lambdas and Functional Interfaces in Java
Lambdas and functional interfaces are powerful features introduced in Java 8 that allow you to write more concise and expressive code by treating functions as first-class citizens. Understanding how to use lambdas and functional interfaces is essential for writing modern Java code. Let’s explore some common use cases and techniques related to lambdas and functional interfaces.
1. Functional Interfaces: A functional interface is an interface that defines exactly one abstract method. Functional interfaces are used as the basis for lambda expressions and method references. Common functional interfaces in Java include Runnable
, Consumer
, Predicate
, Function
, etc.
2. Lambda Expressions: A lambda expression is a concise way to represent an anonymous function. It allows you to pass behavior as a parameter to methods or define simple functions inline. Lambda expressions are defined using the syntax (parameters) -> expression
or (parameters) -> { statements; }
, where the arrow ->
separates the parameters and the body of the lambda.
3. Method References: A method reference is a shorthand notation for referring to an existing method or a constructor by name. It allows you to treat methods as lambda expressions. Method references are defined using the syntax Class::method
or instance::method
, where Class
is the class containing the method and method
is the name of the method.
4. Stream API: The Stream API, introduced in Java 8, provides a functional programming-style way to process collections of data. Streams allow you to perform operations such as filtering, mapping, reducing, and sorting on collections using lambdas and functional interfaces.
Let’s take a look at an example that demonstrates these techniques:
// Example of using lambdas and functional interfaces in Java import java.util.Arrays; import java.util.List; import java.util.function.Predicate; public class LambdaExample { public static void main(String[] args) { List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5); // Using a lambda expression numbers.forEach((number) -> System.out.println(number)); // Using a functional interface Predicate<Integer> isEven = (number) -> number % 2 == 0; List<Integer> evenNumbers = filter(numbers, isEven); System.out.println("Even Numbers: " + evenNumbers); } public static List<Integer> filter(List<Integer> numbers, Predicate<Integer> predicate) { List<Integer> result = new ArrayList<>(); for (Integer number : numbers) { if (predicate.test(number)) { result.add(number); } } return result; } }
In this example, we use lambdas and functional interfaces to print the elements of a list and filter even numbers from the list. We define a lambda expression to specify the behavior of the forEach
method and a functional interface to define the condition for filtering even numbers.
Lambdas and functional interfaces allow you to write more concise and expressive code, making your programs easier to read and maintain.
Code Snippet Idea: File I/O Operations
Related Article: Java Equals Hashcode Tutorial
File I/O Operations in Java
File I/O operations are common tasks in Java that involve reading from and writing to files. Understanding how to perform file I/O operations is essential for working with external data and persisting application state. Let’s explore some common file I/O operations in Java.
1. Reading from Files: Use classes like FileReader
, BufferedReader
, and Scanner
to read data from files. The FileReader
class allows you to read characters from a file, while the BufferedReader
and Scanner
classes provide higher-level methods for reading lines and formatted data from files.
2. Writing to Files: Use classes like FileWriter
, BufferedWriter
, and PrintWriter
to write data to files. The FileWriter
class allows you to write characters to a file, while the BufferedWriter
and PrintWriter
classes provide higher-level methods for writing lines and formatted data to files.
3. File Manipulation: Use classes like File
, Path
, and Files
to perform file manipulation operations, such as creating, deleting, moving, and copying files. The Path
class represents a file or directory path, while the Files
class provides various static methods for common file operations.
4. Exception Handling: When performing file I/O operations, it is important to handle potential exceptions, such as IOException
, that may occur. Use try-catch blocks to catch and handle exceptions, and ensure that resources are properly closed using the close
method.
Let’s take a look at an example that demonstrates these file I/O operations:
// Example of file I/O operations in Java import java.io.BufferedReader; import java.io.BufferedWriter; import java.io.FileReader; import java.io.FileWriter; import java.io.IOException; public class FileIOExample { public static void main(String[] args) { String filePath = "file.txt"; // Writing to a file try (BufferedWriter writer = new BufferedWriter(new FileWriter(filePath))) { writer.write("Hello, World!"); } catch (IOException e) { System.out.println("An error occurred while writing to the file: " + e.getMessage()); } // Reading from a file try (BufferedReader reader = new BufferedReader(new FileReader(filePath))) { String line; while ((line = reader.readLine()) != null) { System.out.println(line); } } catch (IOException e) { System.out.println("An error occurred while reading from the file: " + e.getMessage()); } } }
In this example, we demonstrate writing the string “Hello, World!” to a file using a BufferedWriter
and reading the contents of the file using a BufferedReader
. We use try-with-resources statements to automatically close the file resources after they are used and catch any potential IOException
that may occur.
File I/O operations are essential for working with external data and persisting application state, and mastering these operations is crucial for many programming tasks.
Code Snippet Idea: JDBC Operations
Performing JDBC Operations in Java
JDBC (Java Database Connectivity) is a standard Java API for connecting and interacting with relational databases. Understanding how to perform JDBC operations is essential for working with databases in Java. Let’s explore some common JDBC operations in Java.
1. Establishing a Connection: Use the java.sql.Connection
interface to establish a connection to a database. The DriverManager
class provides methods for creating connections using the appropriate JDBC driver.
2. Executing SQL Statements: Use the java.sql.Statement
or java.sql.PreparedStatement
interface to execute SQL statements. The Statement
interface allows you to execute static SQL statements, while the PreparedStatement
interface allows you to execute parameterized SQL statements with placeholders.
3. Retrieving Result Sets: Use the java.sql.ResultSet
interface to retrieve data from the database as a result set. The result set provides methods for navigating through the rows and accessing the column values.
4. Handling Transactions: Use the java.sql.Connection
interface to manage transactions. The commit
and rollback
methods allow you to commit or rollback changes made within a transaction.
5. Exception Handling: When performing JDBC operations, it is important to handle potential exceptions, such as SQLException
, that may occur. Use try-catch blocks to catch and handle exceptions, and ensure that resources are properly closed using the close
method.
Let’s take a look at an example that demonstrates these JDBC operations:
// Example of performing JDBC operations in Java import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; public class JDBCExample { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/mydatabase"; String username = "root"; String password = "password"; try (Connection connection = DriverManager.getConnection(url, username, password)) { // Creating a table try (PreparedStatement createTableStatement = connection.prepareStatement( "CREATE TABLE IF NOT EXISTS customers (id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(255))")) { createTableStatement.executeUpdate(); } // Inserting data try (PreparedStatement insertStatement = connection.prepareStatement( "INSERT INTO customers (name) VALUES (?)")) { insertStatement.setString(1, "John Doe"); insertStatement.executeUpdate(); } // Querying data try (PreparedStatement selectStatement = connection.prepareStatement( "SELECT * FROM customers")) { ResultSet resultSet = selectStatement.executeQuery(); while (resultSet.next()) { int id = resultSet.getInt("id"); String name = resultSet.getString("name"); System.out.println("ID: " + id + ", Name: " + name); } } } catch (SQLException e) { System.out.println("An error occurred while performing JDBC operations: " + e.getMessage()); } } }
In this example, we demonstrate creating a table, inserting data, and querying data from a MySQL database using JDBC. We use try-with-resources statements to automatically close the JDBC resources after they are used and catch any potential SQLException
that may occur.
Performing JDBC operations allows you to interact with databases and manipulate data in a Java application, enabling powerful data-driven applications.