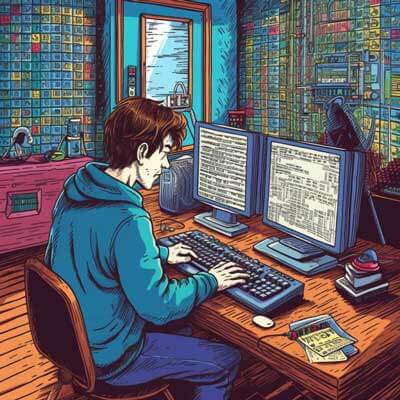
The java.lang.reflect.InvocationTargetException is a checked exception that occurs when a method is invoked through reflection and an exception is thrown by the invoked method. The InvocationTargetException is a wrapper exception that wraps the actual exception thrown by the invoked method.
To resolve the java.lang.reflect.InvocationTargetException, you need to identify and handle the root cause exception that is wrapped by the InvocationTargetException. Here are some steps you can follow to resolve this exception:
1. Inspect the stack trace
When you encounter the java.lang.reflect.InvocationTargetException, the first step is to inspect the stack trace to identify the root cause exception. The stack trace will provide you with information about the exception that was thrown by the invoked method. Look for the “Caused by” section in the stack trace to find the actual exception.
For example, consider the following stack trace:
java.lang.reflect.InvocationTargetException at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.example.MyClass.main(MyClass.java:10) Caused by: java.lang.NullPointerException at com.example.AnotherClass.someMethod(AnotherClass.java:15) ... 5 more
In this example, the root cause exception is a java.lang.NullPointerException that occurred in the com.example.AnotherClass.someMethod
method.
Related Article: Resolving MySQL Connection Issues in Java
2. Handle the root cause exception
Once you have identified the root cause exception, you need to handle it appropriately. The handling mechanism will depend on the type of exception and the requirements of your application. Here are some common strategies for handling exceptions:
– Catch and handle the exception: You can catch the root cause exception using a try-catch block and handle it accordingly. For example, if the root cause exception is a NullPointerException, you can handle it by performing null checks or providing a default value.
try { // Invoke the method } catch (NullPointerException e) { // Handle the exception System.out.println("NullPointerException occurred: " + e.getMessage()); }
– Rethrow the exception: If the root cause exception cannot be handled at the current level, you can rethrow it to an upper level where it can be handled appropriately. This allows for centralized exception handling and provides flexibility in how the exception is handled.
try { // Invoke the method } catch (Exception e) { // Log the exception or perform other actions throw e; }
– Wrap the exception: In some cases, you may need to wrap the root cause exception with a custom exception to provide additional context or to conform to a specific exception hierarchy. This can be useful when you want to abstract the underlying implementation details of the invoked method.
try { // Invoke the method } catch (Exception e) { // Wrap the exception with a custom exception throw new CustomException("An error occurred while invoking the method", e); }
3. Preventing InvocationTargetException
While resolving the java.lang.reflect.InvocationTargetException is important, it is also crucial to prevent its occurrence in the first place. Here are some best practices to follow to minimize the chances of encountering this exception:
– Validate inputs: Ensure that the inputs provided to the invoked method are valid and meet the required conditions. Perform necessary checks and validations before invoking the method to avoid potential exceptions.
– Handle exceptions within the invoked method: If you have control over the invoked method, handle any potential exceptions within the method itself. This will prevent the exceptions from being propagated as InvocationTargetException.
– Use appropriate error handling mechanisms: Make use of try-catch blocks and exception handling mechanisms to handle exceptions at the appropriate level. This will help in isolating and resolving exceptions more effectively.
– Test thoroughly: Perform comprehensive testing of your code, including the invoked methods, to identify and fix any potential issues or exceptions before deploying the code to production.
Related Article: How to Resolve java.lang.ClassNotFoundException in Java