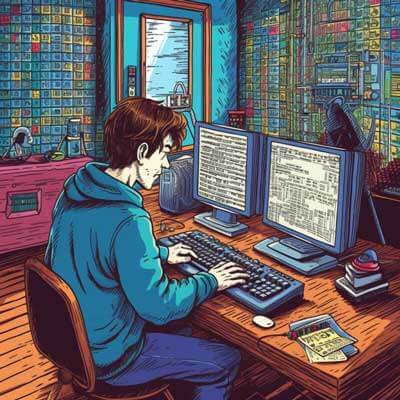
Table of Contents
Converting Java objects to JSON is a common task in modern software development. JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write, and easy for machines to parse and generate. Jackson is a popular Java library for working with JSON. In this guide, we will explore various techniques and best practices for converting Java objects to JSON using Jackson.
Why is this question asked?
The question of how to convert Java objects to JSON with Jackson is commonly asked because JSON has become the de facto standard for data interchange in many modern applications. With the rise of web APIs and microservices architecture, JSON is often used to send and receive data between different components of an application or between different applications. Therefore, knowing how to convert Java objects to JSON is essential for developers working on projects that involve data serialization and deserialization.
Related Article: Java Do-While Loop Tutorial
Potential reasons for converting Java objects to JSON:
- Sending data over a network: JSON is often used as the data format for sending data over the network in web APIs or microservices. Converting Java objects to JSON allows the data to be easily transmitted and reconstructed on the receiving end.
- Storing data in a NoSQL database: NoSQL databases often store data in JSON format. Converting Java objects to JSON allows the data to be stored and retrieved from a NoSQL database.
- Exchanging data with JavaScript applications: JSON is a native data format in JavaScript, so converting Java objects to JSON enables seamless communication between Java backend and JavaScript frontend applications.
Techniques for converting Java objects to JSON with Jackson:
Jackson provides several ways to convert Java objects to JSON. Here are two common techniques:
1. Using ObjectMapper class:
The ObjectMapper class in the Jackson library provides methods to convert Java objects to JSON and vice versa. To convert a Java object to JSON, you can use the writeValueAsString() method of the ObjectMapper class. This method serializes the Java object into a JSON string.
Here's an example that demonstrates how to convert a Java object to JSON using the ObjectMapper class:
import com.fasterxml.jackson.databind.ObjectMapper; public class Main { public static void main(String[] args) throws Exception { // Create an instance of the object to be converted Person person = new Person("John", 30); // Create an instance of ObjectMapper ObjectMapper objectMapper = new ObjectMapper(); // Convert the object to JSON string String jsonString = objectMapper.writeValueAsString(person); // Print the JSON string System.out.println(jsonString); } } class Person { private String name; private int age; public Person(String name, int age) { this.name = name; this.age = age; } // Getters and setters omitted for brevity }
Output:
{"name":"John","age":30}
2. Using annotations:
Jackson also provides annotations that can be used to customize the serialization process. You can annotate the Java object's fields or getters/setters with annotations such as @JsonProperty, @JsonGetter, @JsonSetter, etc., to control the JSON property names and other serialization options.
Here's an example that demonstrates how to use annotations to convert a Java object to JSON:
import com.fasterxml.jackson.annotation.JsonProperty; public class Main { public static void main(String[] args) throws Exception { // Create an instance of the object to be converted Person person = new Person("John", 30); // Create an instance of ObjectMapper ObjectMapper objectMapper = new ObjectMapper(); // Convert the object to JSON string String jsonString = objectMapper.writeValueAsString(person); // Print the JSON string System.out.println(jsonString); } } class Person { @JsonProperty("full_name") private String name; @JsonProperty("years_old") private int age; public Person(String name, int age) { this.name = name; this.age = age; } // Getters and setters omitted for brevity }
Output:
{"full_name":"John","years_old":30}
Best practices and suggestions:
- Use meaningful field names: When converting Java objects to JSON, it is a good practice to use field names that are meaningful and descriptive. This helps in maintaining the readability and understandability of the resulting JSON.
- Handle null values: Jackson, by default, excludes null values from the generated JSON. If you want to include null values in the JSON, you can configure the ObjectMapper to include them using the setSerializationInclusion() method.
- Ignore unknown properties: By default, Jackson throws an exception if it encounters an unknown property (a property in the JSON that does not exist in the Java object). If you want to ignore unknown properties, you can use the @JsonIgnoreProperties(ignoreUnknown = true) annotation at the class level.
- Customize date and time formats: Jackson provides various annotations and configuration options to customize the serialization and deserialization of date and time values. For example, you can use the @JsonFormat annotation to specify a custom date/time format.
Related Article: Java Printf Method Tutorial
Alternative ideas:
- Gson library: Gson is another popular Java library for converting Java objects to JSON and vice versa. It provides a similar set of features as Jackson and can be used as an alternative if you prefer its API or have specific requirements.
- JSON-B: JSON-B is a standard API for converting Java objects to JSON and vice versa, introduced in Java EE 8. It provides a set of annotations and configuration options similar to Jackson and Gson. If you are working on a Java EE project, using JSON-B can be a good alternative.