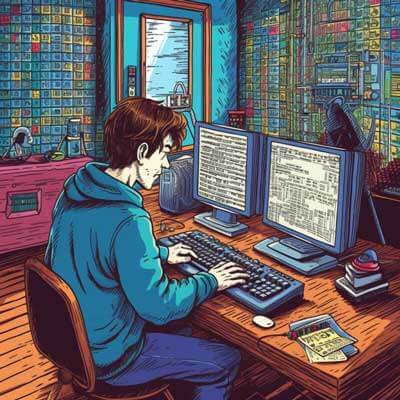
Table of Contents
The Importance of Saving Tree Data Structures in a Java File
In software development, tree data structures play a vital role in organizing and managing hierarchical data. Trees are widely used in various domains, such as file systems, network routing, and data analysis. When working with tree data structures, it is often necessary to persist them for future use or to share them across different systems. In Java, one common approach to saving tree data structures is by writing them to a file.
Saving tree data structures in a Java file offers several benefits. Firstly, it allows us to store the tree in a persistent storage medium, such as a hard disk, which ensures that the data is not lost when the program terminates. Secondly, it enables us to share the tree with other programs or systems, facilitating interoperability. Lastly, saving tree data structures in a file provides a convenient way to store and retrieve complex hierarchical data, allowing for efficient data management and analysis.
In the following sections, we will explore different techniques and mechanisms for saving tree data structures in a Java file and discuss the significance of serialization in achieving data persistence.
Related Article: How to Use Hibernate in Java
Understanding File Handling
Before diving into the process of saving tree data structures in a Java file, it is essential to have a basic understanding of file handling in Java. File handling involves performing operations on files, such as creating, reading, writing, and deleting. In Java, the java.io
package provides classes and methods for file handling.
To work with files in Java, we need to consider two main aspects: file streams and file objects. A file stream is a channel through which data is read from or written to a file. It represents a sequence of bytes that can be read from or written to a file. File objects, on the other hand, provide a way to interact with the file system, such as creating, deleting, or renaming files.
Java provides several classes for file handling, such as File
, FileInputStream
, FileOutputStream
, and FileReader
. These classes allow us to perform various file operations, such as opening a file, reading or writing data to a file, and closing the file.
Let's take a look at an example of how to create a file and write data to it using the FileOutputStream
class:
import java.io.FileOutputStream; import java.io.IOException; public class FileHandlingExample { public static void main(String[] args) { try { FileOutputStream fileOutputStream = new FileOutputStream("data.txt"); String data = "Hello, World!"; byte[] bytes = data.getBytes(); fileOutputStream.write(bytes); fileOutputStream.close(); System.out.println("Data written to file successfully."); } catch (IOException e) { e.printStackTrace(); } } }
In the above example, we create a FileOutputStream
object and pass the name of the file we want to create as a parameter. We then convert the string data into bytes using the getBytes()
method and write the bytes to the file using the write()
method. Finally, we close the file using the close()
method.
Understanding file handling in Java is crucial for saving tree data structures in a Java file. It provides the necessary foundation for performing file operations and manipulating the file system.
Serialization and Its Significance
Serialization is the process of converting an object into a stream of bytes, which can then be stored in a file or transmitted over a network. In Java, serialization is primarily used for saving objects to a file or sending objects across a network. It allows objects to be saved and restored without losing their state or structure.
When it comes to saving tree data structures in a Java file, serialization plays a crucial role. By serializing a tree data structure, we can convert it into a stream of bytes and write it to a file. This serialized representation can be easily stored, shared, or transmitted, and can be later deserialized to reconstruct the original tree data structure.
Java provides the java.io.Serializable
interface, which is a marker interface that indicates that a class can be serialized. By implementing this interface, we can enable the serialization and deserialization of objects of that class. The Serializable
interface does not have any methods, and it acts as a tagging interface.
To serialize a tree data structure in Java, we can make use of the serialization mechanism provided by the Java standard library. This mechanism allows objects to be serialized using the ObjectOutputStream
class and deserialized using the ObjectInputStream
class.
In the next sections, we will explore these classes in more detail and understand how they can be used to save and retrieve tree data structures in a Java file.
Exploring ObjectOutputStream
The ObjectOutputStream
class in Java is used to serialize objects and write them to an output stream. It provides methods for writing primitive data types, objects, and arrays to an output stream. When it comes to saving tree data structures in a Java file, the ObjectOutputStream
class is a crucial component.
To use the ObjectOutputStream
class, we need to create an instance of it and pass an output stream as a parameter. In the context of saving tree data structures in a file, we can pass a FileOutputStream
object as the output stream.
Let's take a look at an example of how to use the ObjectOutputStream
class to serialize and save a tree data structure to a Java file:
import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectOutputStream; public class TreeSerializationExample { public static void main(String[] args) { try { FileOutputStream fileOutputStream = new FileOutputStream("tree.ser"); ObjectOutputStream objectOutputStream = new ObjectOutputStream(fileOutputStream); // Create and populate the tree data structure Tree tree = new Tree(); tree.insert(10); tree.insert(5); tree.insert(15); // Serialize and save the tree to a file objectOutputStream.writeObject(tree); objectOutputStream.close(); fileOutputStream.close(); System.out.println("Tree data structure saved successfully."); } catch (IOException e) { e.printStackTrace(); } } }
In the above example, we create a FileOutputStream
object and pass the name of the file we want to create as a parameter. We then create an ObjectOutputStream
object and pass the FileOutputStream
object as a parameter. Next, we create a tree data structure and populate it with some values. Finally, we use the writeObject()
method of the ObjectOutputStream
class to serialize and save the tree to the file.
The ObjectOutputStream
class provides various other methods for writing different types of data, such as writeInt()
, writeDouble()
, and writeUTF()
. These methods can be used to write primitive types, strings, and other data types to the output stream.
Related Article: How to Convert a String to an Array in Java
Utilizing ObjectInputStream for Reading
The ObjectInputStream
class in Java is used to deserialize objects and read them from an input stream. It provides methods for reading primitive data types, objects, and arrays from an input stream. When it comes to retrieving tree data structures from a Java file, the ObjectInputStream
class is an important component.
To use the ObjectInputStream
class, we need to create an instance of it and pass an input stream as a parameter. In the context of retrieving tree data structures from a file, we can pass a FileInputStream
object as the input stream.
Let's take a look at an example of how to use the ObjectInputStream
class to deserialize and retrieve a tree data structure from a Java file:
import java.io.FileInputStream; import java.io.IOException; import java.io.ObjectInputStream; public class TreeDeserializationExample { public static void main(String[] args) { try { FileInputStream fileInputStream = new FileInputStream("tree.ser"); ObjectInputStream objectInputStream = new ObjectInputStream(fileInputStream); // Deserialize and retrieve the tree data structure Tree tree = (Tree) objectInputStream.readObject(); objectInputStream.close(); fileInputStream.close(); // Perform operations on the tree data structure tree.printInorder(); // Example operation System.out.println("Tree data structure retrieved successfully."); } catch (IOException | ClassNotFoundException e) { e.printStackTrace(); } } }
In the above example, we create a FileInputStream
object and pass the name of the file we want to read from as a parameter. We then create an ObjectInputStream
object and pass the FileInputStream
object as a parameter. Next, we use the readObject()
method of the ObjectInputStream
class to deserialize and retrieve the tree data structure from the file. Finally, we can perform any desired operations on the tree data structure.
The ObjectInputStream
class provides various other methods for reading different types of data, such as readInt()
, readDouble()
, and readUTF()
. These methods can be used to read primitive types, strings, and other data types from the input stream.
Examining the writeObject Method
In Java, the ObjectOutputStream
class provides the writeObject()
method for serializing an object and writing it to an output stream. This method is used to save tree data structures in a Java file.
The writeObject()
method takes an object as a parameter and writes it to the output stream. The object being written must implement the java.io.Serializable
interface, which acts as a marker interface indicating that the object can be serialized.
Let's take a look at an example of how to use the writeObject()
method to serialize and save a tree data structure to a Java file:
import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectOutputStream; public class TreeSerializationExample { public static void main(String[] args) { try { FileOutputStream fileOutputStream = new FileOutputStream("tree.ser"); ObjectOutputStream objectOutputStream = new ObjectOutputStream(fileOutputStream); // Create and populate the tree data structure Tree tree = new Tree(); tree.insert(10); tree.insert(5); tree.insert(15); // Serialize and save the tree to a file objectOutputStream.writeObject(tree); objectOutputStream.close(); fileOutputStream.close(); System.out.println("Tree data structure saved successfully."); } catch (IOException e) { e.printStackTrace(); } } }
In the above example, we create an instance of the ObjectOutputStream
class and pass a FileOutputStream
object as a parameter. We then create a tree data structure and populate it with some values. Finally, we use the writeObject()
method to serialize and save the tree to the file.
It is worth noting that the writeObject()
method can also be used to serialize and save multiple objects to a file. In this case, the objects must be written and read in the same order to ensure proper deserialization.
Understanding the readObject Method
In Java, the ObjectInputStream
class provides the readObject()
method for deserializing an object and reading it from an input stream. This method is used to retrieve tree data structures from a Java file.
The readObject()
method reads an object from the input stream and returns it. The object being read must have been previously serialized and written to the input stream using the writeObject()
method.
Let's take a look at an example of how to use the readObject()
method to deserialize and retrieve a tree data structure from a Java file:
import java.io.FileInputStream; import java.io.IOException; import java.io.ObjectInputStream; public class TreeDeserializationExample { public static void main(String[] args) { try { FileInputStream fileInputStream = new FileInputStream("tree.ser"); ObjectInputStream objectInputStream = new ObjectInputStream(fileInputStream); // Deserialize and retrieve the tree data structure Tree tree = (Tree) objectInputStream.readObject(); objectInputStream.close(); fileInputStream.close(); // Perform operations on the tree data structure tree.printInorder(); // Example operation System.out.println("Tree data structure retrieved successfully."); } catch (IOException | ClassNotFoundException e) { e.printStackTrace(); } } }
In the above example, we create an instance of the ObjectInputStream
class and pass a FileInputStream
object as a parameter. We then use the readObject()
method to deserialize and retrieve the tree data structure from the file. Finally, we can perform any desired operations on the tree data structure.
It is important to note that the readObject()
method returns an Object
type. In order to use the retrieved object as a specific type, such as a tree data structure, we need to cast it to the appropriate type, as shown in the example above.
Working with Binary Files
When saving tree data structures in a Java file, it is common to use binary files rather than plain text files. Binary files store data in binary format, which is more efficient and compact compared to plain text files. Binary files are particularly useful when working with complex data structures, such as tree data structures.
In Java, binary files can be created and manipulated using the FileInputStream
and FileOutputStream
classes, as well as their respective subclasses, such as DataInputStream
and DataOutputStream
. These classes provide methods for reading and writing various data types, such as integers, doubles, and strings, in binary format.
Let's take a look at an example of how to save a tree data structure in a binary file using the DataOutputStream
class:
import java.io.DataOutputStream; import java.io.FileOutputStream; import java.io.IOException; public class BinaryFileExample { public static void main(String[] args) { try { FileOutputStream fileOutputStream = new FileOutputStream("tree.bin"); DataOutputStream dataOutputStream = new DataOutputStream(fileOutputStream); // Create and populate the tree data structure Tree tree = new Tree(); tree.insert(10); tree.insert(5); tree.insert(15); // Write tree data to binary file writeTreeData(tree, dataOutputStream); dataOutputStream.close(); fileOutputStream.close(); System.out.println("Tree data structure saved to binary file successfully."); } catch (IOException e) { e.printStackTrace(); } } private static void writeTreeData(Tree tree, DataOutputStream dataOutputStream) throws IOException { if (tree == null) { dataOutputStream.writeInt(-1); return; } dataOutputStream.writeInt(tree.getData()); writeTreeData(tree.getLeftChild(), dataOutputStream); writeTreeData(tree.getRightChild(), dataOutputStream); } }
In the above example, we create a FileOutputStream
object and pass the name of the binary file we want to create as a parameter. We then create a DataOutputStream
object and pass the FileOutputStream
object as a parameter. Next, we create a tree data structure and populate it with some values. Finally, we use the writeTreeData()
method to write the tree data to the binary file.
The writeTreeData()
method recursively traverses the tree and writes the data of each node to the binary file using the writeInt()
method of the DataOutputStream
class. If a node is null, indicating an empty child node, a sentinel value of -1 is written to the binary file.
Reading tree data from a binary file can be done using the DataInputStream
class. This class provides methods for reading various data types from a binary file, such as readInt()
, readDouble()
, and readUTF()
. These methods can be used to read the data written by the DataOutputStream
class.
Related Article: Tutorial: Best Practices for Java Singleton Design Pattern
Ensuring Data Persistence with Serialization
Data persistence refers to the ability to store and retrieve data beyond the lifetime of a program or session. When it comes to saving tree data structures in a Java file, data persistence is crucial to ensure that the data remains intact and retrievable even after the program terminates.
Serialization provides a mechanism for achieving data persistence by converting objects into a stream of bytes that can be stored in a file. By serializing a tree data structure, we can save it to a file and retrieve it later, ensuring the persistence of the data.
In Java, the serialization and deserialization process is handled by the ObjectOutputStream
and ObjectInputStream
classes, respectively. These classes allow objects to be serialized and deserialized, enabling the persistence of tree data structures.
The serialization process involves converting an object into a stream of bytes using the ObjectOutputStream
class. This stream of bytes can then be written to a file using file handling techniques. The deserialization process, on the other hand, involves reading the stream of bytes from a file and converting it back into an object using the ObjectInputStream
class.
The Serialization Process
The serialization process in Java involves converting an object into a stream of bytes that can be stored in a file or transmitted over a network. This process is handled by the ObjectOutputStream
class and enables the persistence of tree data structures.
To serialize a tree data structure in Java, we need to follow these steps:
1. Create an instance of the ObjectOutputStream
class and pass an output stream as a parameter. This output stream can be a FileOutputStream
object, which allows us to write the serialized data to a file.
2. Create and populate the tree data structure that we want to serialize.
3. Use the writeObject()
method of the ObjectOutputStream
class to serialize the tree data structure. This method takes the tree object as a parameter and writes it to the output stream.
4. Close the ObjectOutputStream
and the output stream to release any system resources.
Here's an example that demonstrates the serialization process for a tree data structure in Java:
import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectOutputStream; public class TreeSerializationExample { public static void main(String[] args) { try { FileOutputStream fileOutputStream = new FileOutputStream("tree.ser"); ObjectOutputStream objectOutputStream = new ObjectOutputStream(fileOutputStream); // Create and populate the tree data structure Tree tree = new Tree(); tree.insert(10); tree.insert(5); tree.insert(15); // Serialize and save the tree to a file objectOutputStream.writeObject(tree); objectOutputStream.close(); fileOutputStream.close(); System.out.println("Tree data structure saved successfully."); } catch (IOException e) { e.printStackTrace(); } } }
In the above example, we create an instance of the FileOutputStream
class and pass the name of the file we want to create as a parameter. We then create an instance of the ObjectOutputStream
class and pass the FileOutputStream
object as a parameter. Next, we create a tree data structure and populate it with some values. Finally, we use the writeObject()
method of the ObjectOutputStream
class to serialize and save the tree to the file.
The Deserialization Process
The deserialization process in Java involves reading a stream of bytes from a file or a network and converting it back into an object. This process is handled by the ObjectInputStream
class and allows us to retrieve tree data structures from a file.
To deserialize a tree data structure in Java, we need to follow these steps:
1. Create an instance of the ObjectInputStream
class and pass an input stream as a parameter. This input stream can be a FileInputStream
object, which allows us to read the serialized data from a file.
2. Use the readObject()
method of the ObjectInputStream
class to deserialize the tree data structure. This method reads the serialized object from the input stream and returns it.
3. Close the ObjectInputStream
and the input stream to release any system resources.
Here's an example that demonstrates the deserialization process for a tree data structure in Java:
import java.io.FileInputStream; import java.io.IOException; import java.io.ObjectInputStream; public class TreeDeserializationExample { public static void main(String[] args) { try { FileInputStream fileInputStream = new FileInputStream("tree.ser"); ObjectInputStream objectInputStream = new ObjectInputStream(fileInputStream); // Deserialize and retrieve the tree data structure Tree tree = (Tree) objectInputStream.readObject(); objectInputStream.close(); fileInputStream.close(); // Perform operations on the tree data structure tree.printInorder(); // Example operation System.out.println("Tree data structure retrieved successfully."); } catch (IOException | ClassNotFoundException e) { e.printStackTrace(); } } }
In the above example, we create an instance of the FileInputStream
class and pass the name of the file we want to read from as a parameter. We then create an instance of the ObjectInputStream
class and pass the FileInputStream
object as a parameter. Next, we use the readObject()
method of the ObjectInputStream
class to deserialize and retrieve the tree data structure from the file. Finally, we can perform any desired operations on the tree data structure.
Storing Tree Data Structures in a Java File
Storing tree data structures in a Java file involves the process of serializing the tree and writing it to a file. The serialized representation of the tree, which consists of a stream of bytes, can be easily stored in a file using file handling techniques.
To store a tree data structure in a Java file, we need to perform the following steps:
1. Create an instance of the ObjectOutputStream
class and pass an output stream as a parameter. This output stream can be a FileOutputStream
object, which allows us to write the serialized data to a file.
2. Create and populate the tree data structure that we want to store.
3. Use the writeObject()
method of the ObjectOutputStream
class to serialize the tree data structure. This method takes the tree object as a parameter and writes it to the output stream.
4. Close the ObjectOutputStream
and the output stream to release any system resources.
Here's an example that demonstrates how to store a tree data structure in a Java file:
import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectOutputStream; public class TreeSerializationExample { public static void main(String[] args) { try { FileOutputStream fileOutputStream = new FileOutputStream("tree.ser"); ObjectOutputStream objectOutputStream = new ObjectOutputStream(fileOutputStream); // Create and populate the tree data structure Tree tree = new Tree(); tree.insert(10); tree.insert(5); tree.insert(15); // Serialize and save the tree to a file objectOutputStream.writeObject(tree); objectOutputStream.close(); fileOutputStream.close(); System.out.println("Tree data structure saved successfully."); } catch (IOException e) { e.printStackTrace(); } } }
In the above example, we create an instance of the FileOutputStream
class and pass the name of the file we want to create as a parameter. We then create an instance of the ObjectOutputStream
class and pass the FileOutputStream
object as a parameter. Next, we create a tree data structure and populate it with some values. Finally, we use the writeObject()
method of the ObjectOutputStream
class to serialize and save the tree to the file.
Related Article: Java Exception Handling Tutorial
Retrieving Tree Data Structures from a File
Retrieving tree data structures from a file involves the process of deserializing the tree and reading it from a file. The deserialization process converts the serialized representation of the tree, which consists of a stream of bytes, back into an object that can be used in the program.
To retrieve a tree data structure from a file in Java, we need to perform the following steps:
1. Create an instance of the ObjectInputStream
class and pass an input stream as a parameter. This input stream can be a FileInputStream
object, which allows us to read the serialized data from a file.
2. Use the readObject()
method of the ObjectInputStream
class to deserialize the tree data structure. This method reads the serialized object from the input stream and returns it.
3. Close the ObjectInputStream
and the input stream to release any system resources.
Here's an example that demonstrates how to retrieve a tree data structure from a Java file:
import java.io.FileInputStream; import java.io.IOException; import java.io.ObjectInputStream; public class TreeDeserializationExample { public static void main(String[] args) { try { FileInputStream fileInputStream = new FileInputStream("tree.ser"); ObjectInputStream objectInputStream = new ObjectInputStream(fileInputStream); // Deserialize and retrieve the tree data structure Tree tree = (Tree) objectInputStream.readObject(); objectInputStream.close(); fileInputStream.close(); // Perform operations on the tree data structure tree.printInorder(); // Example operation System.out.println("Tree data structure retrieved successfully."); } catch (IOException | ClassNotFoundException e) { e.printStackTrace(); } } }
In the above example, we create an instance of the FileInputStream
class and pass the name of the file we want to read from as a parameter. We then create an instance of the ObjectInputStream
class and pass the FileInputStream
object as a parameter. Next, we use the readObject()
method of the ObjectInputStream
class to deserialize and retrieve the tree data structure from the file. Finally, we can perform any desired operations on the tree data structure.
Steps for Saving a Tree Data Structure in Java
To save a tree data structure in Java, we can follow a series of steps that involve serialization and file handling techniques. These steps ensure that the tree data structure is serialized, saved to a file, and can be retrieved later.
Here are the steps for saving a tree data structure in Java:
1. Create an instance of the ObjectOutputStream
class and pass an output stream as a parameter. This output stream can be a FileOutputStream
object, which allows us to write the serialized data to a file.
2. Create and populate the tree data structure that we want to save.
3. Use the writeObject()
method of the ObjectOutputStream
class to serialize the tree data structure. This method takes the tree object as a parameter and writes it to the output stream.
4. Close the ObjectOutputStream
and the output stream to release any system resources.
Here's an example that demonstrates the steps for saving a tree data structure in Java:
import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectOutputStream; public class TreeSerializationExample { public static void main(String[] args) { try { FileOutputStream fileOutputStream = new FileOutputStream("tree.ser"); ObjectOutputStream objectOutputStream = new ObjectOutputStream(fileOutputStream); // Create and populate the tree data structure Tree tree = new Tree(); tree.insert(10); tree.insert(5); tree.insert(15); // Serialize and save the tree to a file objectOutputStream.writeObject(tree); objectOutputStream.close(); fileOutputStream.close(); System.out.println("Tree data structure saved successfully."); } catch (IOException e) { e.printStackTrace(); } } }
Syntax for Writing Tree Data Structures to a Binary File
When writing tree data structures to a binary file in Java, we can use the DataOutputStream
class to write various data types in binary format. This class provides methods for writing integers, doubles, strings, and other data types to a binary file.
The syntax for writing tree data structures to a binary file using the DataOutputStream
class is as follows:
import java.io.DataOutputStream; import java.io.FileOutputStream; import java.io.IOException; public class BinaryFileExample { public static void main(String[] args) { try { FileOutputStream fileOutputStream = new FileOutputStream("tree.bin"); DataOutputStream dataOutputStream = new DataOutputStream(fileOutputStream); // Create and populate the tree data structure Tree tree = new Tree(); tree.insert(10); tree.insert(5); tree.insert(15); // Write tree data to binary file writeTreeData(tree, dataOutputStream); dataOutputStream.close(); fileOutputStream.close(); System.out.println("Tree data structure saved to binary file successfully."); } catch (IOException e) { e.printStackTrace(); } } private static void writeTreeData(Tree tree, DataOutputStream dataOutputStream) throws IOException { if (tree == null) { dataOutputStream.writeInt(-1); return; } dataOutputStream.writeInt(tree.getData()); writeTreeData(tree.getLeftChild(), dataOutputStream); writeTreeData(tree.getRightChild(), dataOutputStream); } }
In the above example, we create an instance of the FileOutputStream
class and pass the name of the binary file we want to create as a parameter. We then create an instance of the DataOutputStream
class and pass the FileOutputStream
object as a parameter. Next, we create a tree data structure and populate it with some values. Finally, we use the writeTreeData()
method to write the tree data to the binary file.
The writeTreeData()
method recursively traverses the tree and writes the data of each node to the binary file using the writeInt()
method of the DataOutputStream
class. If a node is null, indicating an empty child node, a sentinel value of -1 is written to the binary file.
Deserializing a Tree Data Structure in Java
Deserializing a tree data structure in Java involves the process of reading a serialized object from a file and converting it back into a tree object that can be used in the program. This process is handled by the ObjectInputStream
class and allows us to retrieve tree data structures from a file.
To deserialize a tree data structure in Java, we need to perform the following steps:
1. Create an instance of the ObjectInputStream
class and pass an input stream as a parameter. This input stream can be a FileInputStream
object, which allows us to read the serialized data from a file.
2. Use the readObject()
method of the ObjectInputStream
class to deserialize the tree data structure. This method reads the serialized object from the input stream and returns it.
3. Close the ObjectInputStream
and the input stream to release any system resources.
Here's an example that demonstrates how to deserialize a tree data structure in Java:
import java.io.FileInputStream; import java.io.IOException; import java.io.ObjectInputStream; public class TreeDeserializationExample { public static void main(String[] args) { try { FileInputStream fileInputStream = new FileInputStream("tree.ser"); ObjectInputStream objectInputStream = new ObjectInputStream(fileInputStream); // Deserialize and retrieve the tree data structure Tree tree = (Tree) objectInputStream.readObject(); objectInputStream.close(); fileInputStream.close(); // Perform operations on the tree data structure tree.printInorder(); // Example operation System.out.println("Tree data structure retrieved successfully."); } catch (IOException | ClassNotFoundException e) { e.printStackTrace(); } } }
In the above example, we create an instance of the FileInputStream
class and pass the name of the file we want to read from as a parameter. We then create an instance of the ObjectInputStream
class and pass the FileInputStream
object as a parameter. Next, we use the readObject()
method of the ObjectInputStream
class to deserialize and retrieve the tree data structure from the file. Finally, we can perform any desired operations on the tree data structure.
Related Article: How to Use a Scanner Class in Java
Ensuring Data Persistence While Saving Tree Data Structures
When saving tree data structures in a Java file, ensuring data persistence is crucial to prevent data loss and maintain the integrity of the tree. Data persistence refers to the ability to store and retrieve data beyond the lifetime of a program or session.
To ensure data persistence while saving tree data structures in Java, we can follow these practices:
1. Use serialization: Serialize the tree data structure using the ObjectOutputStream
class to convert it into a stream of bytes. This serialized representation can be easily stored in a file or transmitted over a network.
2. Choose a suitable file format: When saving tree data structures, it is important to choose a file format that supports binary data storage and retrieval. Binary file formats are more efficient and compact compared to plain text files, making them ideal for storing complex data structures like trees.
3. Close the streams: After saving the tree data structure to a file, make sure to close the output streams, such as ObjectOutputStream
or DataOutputStream
, as well as the file streams, such as FileOutputStream
. Closing the streams releases system resources and ensures proper data persistence.
4. Handle exceptions: When performing file operations, such as saving or retrieving tree data structures, it is important to handle exceptions properly. Use try-catch blocks to catch any potential exceptions, such as IOException
, and handle them gracefully to prevent data loss or corruption.
Techniques for Saving Tree Data Structures in a Java File
When it comes to saving tree data structures in a Java file, there are several techniques and mechanisms available. These techniques provide different ways to store and retrieve tree data structures, depending on the specific requirements and constraints of the application.
Here are some techniques for saving tree data structures in a Java file:
1. Serialization: Serialization is a commonly used technique for saving tree data structures in a Java file. It involves converting the tree object into a stream of bytes using the ObjectOutputStream
class and writing it to a file. The serialized data can be easily stored, shared, or transmitted, and can be later deserialized to reconstruct the tree object.
2. Binary file storage: Binary file storage is another technique for saving tree data structures in a Java file. It involves writing the tree data in binary format using classes such as DataOutputStream
and DataInputStream
. This technique is particularly useful for complex data structures, as it provides a more efficient and compact representation compared to plain text files.
3. Custom file formats: In some cases, it may be necessary to use custom file formats to save tree data structures in a Java file. Custom file formats allow for more control over the data storage and retrieval process, enabling specific optimizations or requirements specific to the application. However, implementing custom file formats can be more complex and may require additional effort.
4. External libraries: There are also external libraries available that provide specialized support for saving tree data structures in a Java file. These libraries may offer advanced features, such as compression, encryption, or indexing, that can enhance the storage and retrieval process. Examples of such libraries include Apache Avro, Google Protocol Buffers, or Java Object Serialization libraries.
The choice of technique depends on various factors, including the complexity of the tree data structure, the performance requirements, and the compatibility with other systems or languages. It is important to carefully evaluate these factors and choose the most suitable technique for saving tree data structures in a Java file.
Exploring Data Persistence in Java
Data persistence is a fundamental concept in software development that refers to the ability to store and retrieve data beyond the lifetime of a program or session. In Java, data persistence can be achieved using various techniques and mechanisms, such as file handling, databases, or serialization.
When it comes to saving tree data structures in Java, data persistence plays a crucial role in ensuring that the data remains intact and retrievable even after the program terminates. By persisting tree data structures, we can store complex hierarchical data in a persistent storage medium, such as a file or a database, and retrieve it for future use or analysis.
In Java, data persistence can be achieved through different approaches:
1. File handling: File handling is a basic technique for achieving data persistence in Java. It involves performing operations on files, such as creating, reading, writing, and deleting. By saving tree data structures in a file, we can store the data in a persistent storage medium, ensuring that it is not lost when the program terminates.
2. Databases: Databases provide a more advanced and robust approach to data persistence in Java. By storing tree data structures in a database, we can benefit from features such as structured querying, indexing, transaction management, and data integrity. Databases offer a higher level of data management and can handle large volumes of data efficiently.
3. Serialization: Serialization is a mechanism provided by Java for achieving data persistence by converting objects into a stream of bytes that can be stored in a file or transmitted over a network. By serializing tree data structures, we can easily store and retrieve them, ensuring their persistence beyond the lifetime of the program.
Each approach has its own advantages and considerations. File handling is simple and straightforward, but may not be suitable for large or complex data sets. Databases provide advanced features and scalability, but require additional setup and maintenance. Serialization offers a compact and portable format, but may not be suitable for querying or complex data manipulation.
The choice of data persistence technique depends on factors such as the size and complexity of the data, performance requirements, and interoperability with other systems or languages. It is important to carefully evaluate these factors and choose the most appropriate technique for achieving data persistence while saving tree data structures in Java.
The Importance of Saving Tree Data Structures in Java
Tree data structures play a crucial role in organizing and managing hierarchical data in software development. They are widely used in various domains, such as file systems, network routing, and data analysis. When working with tree data structures, it is often necessary to persist them for future use or to share them across different systems.
Saving tree data structures in Java offers several benefits:
1. Data persistence: Saving tree data structures in Java ensures that the data remains intact and retrievable even after the program terminates. By persisting tree data structures, we can store them in a persistent storage medium, such as a file or a database, and retrieve them for future use or analysis.
2. Sharing and interoperability: Saving tree data structures in Java allows for easy sharing and interoperability with other programs or systems. Serialized tree data structures can be easily transmitted over a network or shared with other Java applications, facilitating collaboration and data exchange.
3. Efficient data management: Saving tree data structures in Java provides a convenient way to store and retrieve complex hierarchical data. By persisting tree data structures, we can efficiently manage and manipulate the data, perform queries, and analyze the structure and content of the tree.
4. Data analysis and visualization: Saved tree data structures in Java can be easily loaded into data analysis or visualization tools for further analysis or visualization. This enables us to gain insights from the tree data, identify patterns or trends, and make informed decisions based on the analyzed data.
Related Article: How to Manage Collections Without a SortedList in Java
Additional Resources
- GeeksforGeeks - How to Serialize and Deserialize a Binary Tree in Java?