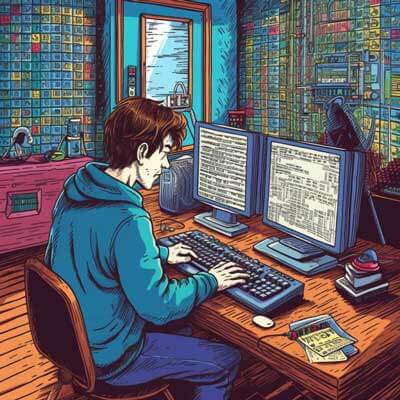
- Introduction to String Substring
- Basics of Substring
- Syntax of Substring
- Usage of Substring in Coding
- Case Study: Applying Substring in Real World Scenarios
- Best Practices When Using Substring
- Performance Considerations: Efficiency of Substring
- Advanced Techniques: Managing Substring
- Error Handling with Substring
- Code Snippet 1: Extracting Substring from a String
- Code Snippet 2: Using Substring with Loop Structures
- Code Snippet 3: Substring within Conditional Statements
- Code Snippet 4: Substring with Arrays
- Code Snippet 5: Substring in Object-Oriented Programming
Introduction to String Substring
The substring operation in Java is used to extract a portion of a string. It allows you to retrieve a specific section of characters from a larger string. Understanding how to use substring effectively is essential for manipulating and working with strings in Java.
Related Article: How To Parse JSON In Java
Basics of Substring
To extract a substring from a string, you need to specify the starting and ending indices. The starting index is inclusive, meaning that the character at that position will be included in the substring. The ending index is exclusive, so the character at that position will not be included in the substring.
Here’s an example that demonstrates the basic usage of substring:
String str = "Hello, World!"; String substring = str.substring(7, 12); System.out.println(substring);
Output:
World
In this example, we start extracting the substring from index 7, which corresponds to the character ‘W’, and end at index 12, which corresponds to the character ‘!’. The resulting substring is “World”.
Syntax of Substring
The syntax of the substring method is as follows:
String substring = str.substring(startIndex, endIndex);
The startIndex
parameter specifies the index of the first character to include in the substring. The endIndex
parameter specifies the index of the character immediately following the last character to include in the substring.
Usage of Substring in Coding
Substring can be used in various scenarios to manipulate and process strings. Let’s explore a few common use cases and see how substring can be applied.
Example 1: Extracting the Domain Name from a URL
String url = "https://www.example.com"; int start = url.indexOf("://") + 3; int end = url.indexOf("/", start); String domain = url.substring(start, end); System.out.println(domain);
Output:
www.example.com
In this example, we extract the domain name from a URL by finding the starting index after “://”, and the ending index before the first occurrence of “/”. The resulting substring is the domain name “www.example.com”.
Example 2: Formatting Phone Numbers
String phoneNumber = "1234567890"; String formattedNumber = phoneNumber.substring(0, 3) + "-" + phoneNumber.substring(3, 6) + "-" + phoneNumber.substring(6); System.out.println(formattedNumber);
Output:
123-456-7890
In this example, we format a phone number by extracting substrings for the area code, the first three digits, and the last four digits. We then concatenate these substrings with hyphens to create the desired format.
Related Article: How To Convert Array To List In Java
Case Study: Applying Substring in Real World Scenarios
In real-world scenarios, substring can be utilized in various ways to solve practical problems. Let’s consider a case study where substring is applied to manipulate and analyze textual data.
Case Study: Analyzing Email Addresses
Suppose you have a list of email addresses and you need to extract the username and domain name for further analysis. Here’s how substring can be used to achieve this:
String email = "johndoe@example.com"; int atIndex = email.indexOf("@"); String username = email.substring(0, atIndex); String domain = email.substring(atIndex + 1); System.out.println("Username: " + username); System.out.println("Domain: " + domain);
Output:
Username: johndoe Domain: example.com
In this case study, we use the indexOf
method to find the index of the “@” symbol, which separates the username and domain name in an email address. We then extract the substrings before and after “@” to obtain the desired information.
Best Practices When Using Substring
When working with substring in Java, it is important to keep a few best practices in mind to ensure efficient and correct usage. Here are some recommendations:
1. Be mindful of the indices: Remember that the starting index is inclusive and the ending index is exclusive. Make sure to select the appropriate indices to retrieve the desired substring accurately.
2. Handle index out of bounds: If the specified indices fall outside the bounds of the string, a StringIndexOutOfBoundsException
will be thrown. Always validate the indices and handle potential exceptions to avoid runtime errors.
3. Consider String length: When extracting substrings, be aware of the length of the original string. Ensure that the specified indices do not exceed the length to prevent unexpected behavior or errors.
4. Immutable strings: Keep in mind that strings in Java are immutable, meaning they cannot be modified once created. Each substring operation creates a new string object, so excessive substring usage on large strings can result in unnecessary memory overhead.
Performance Considerations: Efficiency of Substring
While substring is a convenient tool for manipulating strings, it’s important to consider its performance implications, especially when dealing with large strings or performing substring operations in a loop.
Each call to the substring method creates a new string object, copying the underlying character array. This can result in unnecessary memory allocations and impact performance, especially if used extensively.
To mitigate performance issues, consider alternatives such as using character arrays or StringBuilder when performing complex string manipulations. These approaches can be more efficient, especially when dealing with large strings or repetitive substring operations.
Related Article: How To Iterate Over Entries In A Java Map
Advanced Techniques: Managing Substring
In addition to the basic usage of substring, there are advanced techniques that can be employed when working with strings in Java. Let’s explore a few advanced techniques to manage substrings effectively.
Technique 1: Immutable Substring
To create an immutable substring without copying the underlying character array, you can use the String
constructor that takes a character array, offset, and length as parameters. This allows you to create a substring that references the original string’s character array, saving memory and improving performance.
String str = "Hello, World!"; String immutableSubstring = new String(str.toCharArray(), 7, 5); System.out.println(immutableSubstring);
Output:
World
In this example, we create an immutable substring using the String
constructor, specifying the character array, offset (starting index), and length. The resulting substring “World” shares the same character array as the original string.
Technique 2: StringBuilder with Substring
When performing multiple substring operations, using a StringBuilder
can be more efficient than concatenating strings directly. The StringBuilder
class provides a convenient way to build strings efficiently.
String str = "Lorem ipsum dolor sit amet"; StringBuilder builder = new StringBuilder(); builder.append(str.substring(0, 5)) .append(" ") .append(str.substring(6, 11)) .append(" ") .append(str.substring(12, 17)); String result = builder.toString(); System.out.println(result);
Output:
Lorem ipsum dolor
In this example, we use a StringBuilder
to concatenate three substrings separated by spaces. This approach avoids unnecessary string copies and improves performance compared to direct string concatenation.
Error Handling with Substring
When working with substring, it’s crucial to handle potential errors or unexpected input to ensure the reliability of your code. Here are some error handling considerations when using substring:
1. Validate indices: Before calling the substring method, validate the indices to ensure they are within the bounds of the string. If the indices are invalid, handle the error appropriately, such as throwing an exception or providing default values.
2. Handle empty strings: If the original string is empty, calling substring would result in an IndexOutOfBoundsException
. Check for empty strings and handle them gracefully to prevent runtime errors.
3. Null checks: Verify that the string object is not null before performing any substring operations. Calling substring on a null reference will result in a NullPointerException
.
By incorporating proper error handling techniques, you can make your code more robust and prevent unexpected failures when working with substring in Java.
Code Snippet 1: Extracting Substring from a String
String str = "Hello, World!"; String substring = str.substring(7, 12); System.out.println(substring);
Output:
World
This code snippet demonstrates the basic usage of substring to extract a substring from a string. The resulting substring is “World”.
Related Article: How To Split A String In Java
Code Snippet 2: Using Substring with Loop Structures
String sentence = "The quick brown fox jumps over the lazy dog"; String[] words = sentence.split(" "); for (String word : words) { String firstLetter = word.substring(0, 1); System.out.println(firstLetter); }
Output:
T q b f j o t l d
In this code snippet, we split a sentence into an array of words using the split
method. Then, in a loop structure, we extract the first letter of each word using substring and print it.
Code Snippet 3: Substring within Conditional Statements
String str = "Java is a versatile programming language"; if (str.substring(0, 4).equals("Java")) { System.out.println("The string starts with 'Java'"); } else { System.out.println("The string does not start with 'Java'"); }
Output:
The string starts with 'Java'
In this code snippet, we use substring within a conditional statement to check if a string starts with the word “Java”. If the condition is true, a corresponding message is printed.
Code Snippet 4: Substring with Arrays
String[] names = {"John Doe", "Jane Smith", "Michael Johnson"}; for (String name : names) { String lastName = name.substring(name.indexOf(" ") + 1); System.out.println(lastName); }
Output:
Doe Smith Johnson
In this code snippet, we iterate over an array of full names and extract the last name from each name using substring. The last name is obtained by finding the index of the space character and extracting the substring starting from the next character.
Related Article: How To Convert Java Objects To JSON With Jackson
Code Snippet 5: Substring in Object-Oriented Programming
public class Person { private String name; public Person(String name) { this.name = name; } public String getInitials() { String[] names = name.split(" "); StringBuilder initials = new StringBuilder(); for (String n : names) { initials.append(n.substring(0, 1)); } return initials.toString(); } } // Usage: Person person = new Person("John Doe"); System.out.println(person.getInitials());
Output:
JD
In this code snippet, we demonstrate how substring can be used within an object-oriented programming context. The Person
class has a getInitials
method that extracts the first letter of each name component and concatenates them into initials.