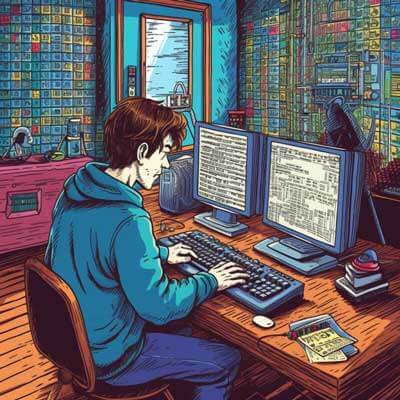
Table of Contents
Enum Types in TypeScript
Enum types in TypeScript allow you to define a set of named values. These named values can then be used as a type, providing a way to define a specific range of possible values for a variable or property. Enum types are particularly useful when you have a fixed set of options or when you want to restrict the possible values that a variable can take.
In TypeScript, enum types are declared using the enum
keyword. Each value in the enum is assigned a numeric value by default, starting from 0 and incrementing by 1 for each subsequent value. However, you can also explicitly assign values to the enum members if desired.
Here's an example of how to define an enum type in TypeScript:
enum Color { Red, Green, Blue, } let myColor: Color = Color.Green; console.log(myColor); // Output: 1
In this example, we define an enum type called Color
with three members: Red
, Green
, and Blue
. The values of the enum members are automatically assigned numeric values, with Red
being 0, Green
being 1, and Blue
being 2. We then assign the value Color.Green
to the variable myColor
and log its value, which is 1.
Enum types in TypeScript provide a way to represent a set of related values and make your code more expressive and self-documenting. They also help in catching potential bugs at compile-time by enforcing type safety.
Related Article: TypeScript While Loop Tutorial
Prisma Types
Prisma is an open-source database toolkit that provides an Object-Relational Mapping (ORM) layer and a query builder for TypeScript and JavaScript applications. It allows you to define your database schema using a declarative syntax and then generates a set of strongly-typed query functions based on that schema.
In Prisma, you define your database schema using a special Prisma schema file, which is written in a DSL (Domain-Specific Language) called Prisma Schema. The Prisma schema file describes the structure of your database tables, their relationships, and other metadata.
Prisma generates TypeScript types based on your Prisma schema, which you can then use in your application code for type checking and autocompletion. These generated types provide a strongly-typed API for interacting with your database and help in catching potential errors and bugs at compile-time.
Enum Types in TypeScript and Prisma
Enum types in TypeScript can be used in conjunction with Prisma to define and enforce restricted sets of values for certain fields in your database schema. By using enum types, you can ensure that only valid values are stored in the database and retrieved from it.
To define an enum type in Prisma, you can use the Enum
keyword in your Prisma schema file. You specify the name of the enum type along with its possible values. Prisma then generates the corresponding TypeScript type for the enum, which you can use in your application code.
Here's an example of how to define an enum type in Prisma:
enum Role { USER ADMIN MODERATOR } model User { id Int @id @default(autoincrement()) name String role Role }
In this example, we define an enum type called Role
with three possible values: USER
, ADMIN
, and MODERATOR
. We then use this enum type as the type for the role
field in the User
model. Prisma will generate the corresponding TypeScript type for the Role
enum, which you can use in your application code.
Using enum types in Prisma helps in ensuring that only valid values are stored in the database and retrieved from it. It provides an additional layer of type safety and helps in catching potential errors and bugs at compile-time.
Enum Fields in Prisma
Enum fields in Prisma allow you to define fields in your database schema that can only take a restricted set of values. These values are defined using enum types, which ensure that only valid values are stored in the database and retrieved from it.
To define an enum field in Prisma, you can use an enum type as the type for the field in your Prisma schema file. Prisma will then generate the corresponding database column with the specified enum type. The values stored in the column will be limited to the values defined in the enum type.
Here's an example of how to define an enum field in Prisma:
enum Gender { MALE FEMALE OTHER } model User { id Int @id @default(autoincrement()) name String gender Gender }
In this example, we define an enum type called Gender
with three possible values: MALE
, FEMALE
, and OTHER
. We then use this enum type as the type for the gender
field in the User
model. Prisma will generate the corresponding database column with the Gender
enum type, ensuring that only valid values are stored in the column.
Enum fields in Prisma provide a way to enforce restrictions on the values that can be stored in the database. They help in maintaining data integrity and catching potential errors and bugs at compile-time.
Related Article: Building a Rules Engine with TypeScript
Enum Values in TypeScript
Enum values in TypeScript represent the possible values that can be assigned to variables or properties of an enum type. They are defined as members of the enum type and can be used to restrict the range of values that a variable can take.
Enum values in TypeScript are represented by their corresponding numeric values by default. However, you can also explicitly assign values to enum members if desired. Enum values can be used in assignments, comparisons, and switch statements, providing a concise and expressive way to work with a fixed set of options.
Here's an example of how to work with enum values in TypeScript:
enum Direction { North, South, East, West, } function getOppositeDirection(direction: Direction): Direction { switch (direction) { case Direction.North: return Direction.South; case Direction.South: return Direction.North; case Direction.East: return Direction.West; case Direction.West: return Direction.East; default: throw new Error("Invalid direction"); } } let currentDirection: Direction = Direction.North; let oppositeDirection: Direction = getOppositeDirection(currentDirection); console.log(oppositeDirection); // Output: 1 (Direction.South)
In this example, we define an enum type called Direction
with four members: North
, South
, East
, and West
. We then define a function getOppositeDirection
that takes a parameter of type Direction
and returns the opposite direction. Inside the function, we use a switch statement to determine the opposite direction based on the input direction. We then assign the value Direction.North
to the variable currentDirection
and call the getOppositeDirection
function to get the opposite direction. Finally, we log the value of the oppositeDirection
variable, which is 1
(corresponding to Direction.South
).
Enum values in TypeScript provide a way to work with a fixed set of options in a type-safe manner. They help in making your code more readable, maintainable, and less error-prone.
Example of Enum in TypeScript
Here's an example that demonstrates the usage of an enum in TypeScript:
enum Weekday { Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, Sunday, } function isWeekend(day: Weekday): boolean { return day === Weekday.Saturday || day === Weekday.Sunday; } let today: Weekday = Weekday.Monday; console.log(isWeekend(today)); // Output: false
In this example, we define an enum type called Weekday
with seven members representing the days of the week. We then define a function isWeekend
that takes a parameter of type Weekday
and checks if the given day is a weekend day (Saturday or Sunday). Inside the function, we compare the input day with the Weekday.Saturday
and Weekday.Sunday
enum values.
We then assign the value Weekday.Monday
to the variable today
and call the isWeekend
function to check if today is a weekend day. Finally, we log the result, which is false
since Weekday.Monday
is not a weekend day.
This example demonstrates how enum types can be used to define a restricted set of options and perform type-safe operations based on those options.
Prisma Enum Documentation
Prisma provides comprehensive documentation on how to use enum types in your Prisma schema. The documentation covers various topics, including how to define enum types, how to use them as field types, and how to work with enum values in your application code.
You can find the Prisma enum documentation here.
The documentation provides detailed explanations, code examples, and best practices for working with enum types in Prisma. It is a valuable resource for understanding and leveraging the power of enum types in your Prisma-based projects.
Type Checking with Enum Types in TypeScript
TypeScript provides useful type checking capabilities that can help catch potential errors and bugs at compile-time. Enum types in TypeScript play a significant role in type checking by providing a way to restrict the range of possible values for variables or properties.
When you define a variable or property with an enum type, TypeScript ensures that only valid enum values can be assigned to it. This helps prevent assigning incorrect or unintended values to variables, reducing the likelihood of bugs and runtime errors.
Here's an example that demonstrates type checking with enum types in TypeScript:
enum Season { Spring, Summer, Autumn, Winter, } let currentSeason: Season = Season.Summer; currentSeason = "InvalidValue"; // Error: Type '"InvalidValue"' is not assignable to type 'Season'
In this example, we define an enum type called Season
with four members representing the seasons. We then declare a variable currentSeason
of type Season
and assign the value Season.Summer
to it. TypeScript ensures that only valid enum values can be assigned to currentSeason
.
If we try to assign an invalid value, such as "InvalidValue"
, TypeScript raises a type error, indicating that the assigned value is not assignable to the Season
enum type. This helps catch potential errors at compile-time and ensures that only valid enum values are used.
Type checking with enum types in TypeScript provides an additional layer of safety and helps in catching potential errors and bugs early in the development process.
Related Article: Tutorial: Working with Datetime Type in TypeScript
Purpose of Enum Types in TypeScript
The purpose of enum types in TypeScript is to provide a way to define a restricted set of named values. Enum types allow you to assign meaningful names to values, making your code more expressive and self-documenting.
Enum types in TypeScript are particularly useful in scenarios where you have a fixed set of options or when you want to provide a limited range of choices for a variable or property. They help in making your code more readable, maintainable, and less error-prone.
Defining Enum Types in TypeScript
To define an enum type in TypeScript, you use the enum
keyword followed by the name of the enum type and a set of member names. Each member name represents a possible value for the enum type.
Here's the syntax for defining an enum type in TypeScript:
enum EnumName { Member1, Member2, Member3, // ... }
In this syntax, EnumName
is the name of the enum type, and Member1
, Member2
, Member3
, and so on, are the member names representing the possible values.
Here's an example that demonstrates how to define an enum type in TypeScript:
enum Color { Red, Green, Blue, }
In this example, we define an enum type called Color
with three members: Red
, Green
, and Blue
. The values of the enum members are automatically assigned numeric values, with Red
being 0, Green
being 1, and Blue
being 2.
Defining enum types in TypeScript provides a way to represent a set of related values and enforce type safety in your code.
Using Enum Types in Prisma
Enum types in TypeScript can be used in conjunction with Prisma to define and enforce restricted sets of values for fields in your database schema. By using enum types, you can ensure that only valid values are stored in the database and retrieved from it.
To use an enum type in Prisma, you can define an enum type in your Prisma schema file and then use it as the type for a field in your model.
Here's an example that demonstrates how to use an enum type in Prisma:
enum Role { USER ADMIN MODERATOR } model User { id Int @id @default(autoincrement()) name String role Role }
In this example, we define an enum type called Role
with three possible values: USER
, ADMIN
, and MODERATOR
. We then use this enum type as the type for the role
field in the User
model.
Prisma will generate the corresponding database column with the specified enum type, ensuring that only valid values are stored in the column.
Using enum types in Prisma helps in maintaining data integrity and catching potential errors and bugs at compile-time. It provides an additional layer of type safety and ensures that only valid values are used in your database operations.
Benefits of Using Enum Types in Prisma
Using enum types in Prisma offers several benefits for your database operations:
1. Type Safety: Enum types provide a way to enforce type safety and restrict the range of possible values for fields in your database schema. This helps catch potential errors and bugs at compile-time, reducing the likelihood of runtime errors.
2. Data Integrity: By using enum types, you can ensure that only valid values are stored in the database and retrieved from it. This helps in maintaining data integrity and prevents inconsistencies or invalid data from being stored.
3. Expressive Code: Enum types allow you to assign meaningful names to values, making your code more expressive and self-documenting. This improves the readability and maintainability of your codebase.
4. Compile-Time Checks: Enum types enable compile-time checks for field values, helping in catching potential errors and bugs early in the development process. This saves time and effort by reducing the need for runtime debugging and error handling.
Using enum types in Prisma provides a useful way to define and enforce restricted sets of values for your database fields. It helps in ensuring data integrity, improving code quality, and reducing the likelihood of errors and bugs.
Related Article: How to Use the MouseEventHandlers in TypeScript
Converting Enum Types to String Types in TypeScript
In some cases, you may need to convert enum types to string types in TypeScript. This can be useful when you want to represent enum values as strings in your application code or when you need to serialize enum values for storage or transmission.
To convert enum types to string types in TypeScript, you can use type assertions or convert enum values explicitly using the .toString()
method.
Here's an example that demonstrates how to convert enum types to string types in TypeScript:
enum Color { Red, Green, Blue, } let myColor: Color = Color.Green; // Using type assertion let myColorAsString: string = Color[myColor] as string; console.log(myColorAsString); // Output: "Green" // Using .toString() method let myColorAsString2: string = myColor.toString(); console.log(myColorAsString2); // Output: "1"
In this example, we define an enum type called Color
with three members: Red
, Green
, and Blue
. We then assign the value Color.Green
to the variable myColor
and convert it to a string using type assertion (Color[myColor] as string
) and the .toString()
method (myColor.toString()
).
The resulting string values are "Green"
and "1"
respectively. Type assertion allows us to access the string representation of the enum value directly, while the .toString()
method converts the numeric value of the enum to a string.
Converting enum types to string types in TypeScript provides flexibility in representing enum values in different formats and contexts.
Setting Default Values for Enum Fields in Prisma
In Prisma, you can set default values for enum fields in your database schema. Default values are used when no explicit value is provided for a field during database operations.
To set a default value for an enum field in Prisma, you can use the @default
attribute in your Prisma schema file. You specify the default value as an argument to the @default
attribute.
Here's an example that demonstrates how to set a default value for an enum field in Prisma:
enum Role { USER ADMIN MODERATOR } model User { id Int @id @default(autoincrement()) name String role Role @default(USER) }
In this example, we define an enum type called Role
with three possible values: USER
, ADMIN
, and MODERATOR
. We then use this enum type as the type for the role
field in the User
model.
We set the default value of the role
field to Role.USER
using the @default
attribute. This means that if no explicit value is provided for the role
field during a create operation, it will default to Role.USER
.
Setting default values for enum fields in Prisma allows you to provide fallback values and ensure consistent data in your database.
Limitations of Using Enum Types in TypeScript
While enum types in TypeScript offer several benefits, they also have some limitations that you should be aware of:
1. Numeric Values: By default, enum members are assigned numeric values starting from 0 and incrementing by 1 for each subsequent member. This can lead to confusion when comparing enum values, as the numeric values may not always match the intended meaning of the enum members.
2. No Reverse Mapping: TypeScript enum types do not support reverse mapping by default. This means that you can't easily get the enum member name from its numeric value. However, you can work around this limitation by using type assertions or custom helper functions.
3. Limited Extensibility: Once an enum type is defined, you cannot add or remove members dynamically at runtime. Enum types are static and cannot be modified after they are defined. If you need dynamic sets of values, you may need to use alternative approaches such as string literal types or union types.
4. Lack of String Enum Support: TypeScript enum types are primarily based on numeric values. While you can assign string values to enum members, they are still treated as numeric values. This can be limiting if you need string-based enum types or want to perform string-based operations.
It's important to consider these limitations when deciding to use enum types in your TypeScript code. While enum types can provide type safety and enforced restrictions, they may not always be the best choice for every scenario.
Documentation for Using Enum Types in Prisma
Prisma provides detailed documentation on how to use enum types in your Prisma schema. The documentation covers various topics, including how to define enum types, how to use them as field types, and how to work with enum values in your application code.
You can find the Prisma documentation on enum types here.
The documentation provides comprehensive explanations, code examples, and best practices for working with enum types in Prisma. It is a valuable resource for understanding and leveraging the power of enum types in your Prisma-based projects.
Related Article: Tutorial on Typescript ts-ignore
Common Use Cases for Enum Types in TypeScript
Enum types in TypeScript are versatile and can be used in various scenarios. Some common use cases for enum types in TypeScript include:
1. Status Codes: Enum types can be used to represent status codes in APIs or network protocols. For example, you can define an enum type with members like OK
, NOT_FOUND
, FORBIDDEN
, and so on, to represent different HTTP status codes.
2. Configuration Options: Enum types can be used to represent different configuration options in your application. For example, you can define an enum type with members like DEBUG
, INFO
, and ERROR
to represent different logging levels.
3. User Roles: Enum types can be used to represent different user roles in your application. For example, you can define an enum type with members like USER
, ADMIN
, and MODERATOR
to represent different user roles and enforce access control.
4. Days of the Week: Enum types can be used to represent the days of the week in your application. For example, you can define an enum type with members like MONDAY
, TUESDAY
, and so on, to represent the days of the week and perform operations based on the current day.
These are just a few examples of how enum types can be used in TypeScript. Enum types provide a flexible and type-safe way to represent a restricted set of options in your code.