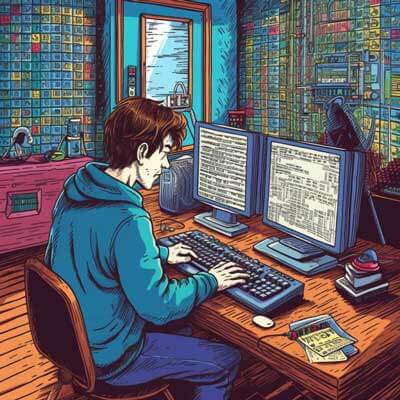
How to Check if a String is in Enum
In TypeScript, an enum is a way to define a set of named constant values. It allows you to create a group of related values that can be assigned to a variable or used as a type. Sometimes, you may need to check if a given string value exists in an enum. TypeScript provides several methods to achieve this.
One way to check if a string is in an enum is by using the keyof
operator and the in
operator. The keyof
operator returns the union of all property names of a type, and the in
operator checks if a property exists in an object.
Here’s an example that demonstrates how to check if a string is in an enum:
enum Fruit { Apple = "apple", Banana = "banana", Orange = "orange" } function isFruit(value: string): value is Fruit { return value in Fruit; } console.log(isFruit("apple")); // Output: true console.log(isFruit("grape")); // Output: false
In this example, we have an enum called Fruit
with three values: Apple
, Banana
, and Orange
. The isFruit
function takes a string parameter and checks if the value exists in the Fruit
enum using the in
operator. The function returns a boolean indicating whether the value is a valid fruit.
Another way to check if a string is in an enum is by using the Object.values
method. This method returns an array of all enum values. You can then use the includes
method to check if the given string value is present in the array.
Here’s an example that demonstrates this approach:
enum Color { Red = "red", Blue = "blue", Green = "green" } function isColor(value: string): value is Color { return Object.values(Color).includes(value); } console.log(isColor("red")); // Output: true console.log(isColor("yellow")); // Output: false
In this example, we have an enum called Color
with three values: Red
, Blue
, and Green
. The isColor
function takes a string parameter and checks if the value exists in the Color
enum by using Object.values
to get an array of all enum values and then using the includes
method to check if the value is present in the array.
These are two common methods to check if a string is in an enum in TypeScript. Choose the method that best suits your needs and the specific requirements of your project.
External sources:
– TypeScript documentation on enums: https://www.typescriptlang.org/docs/handbook/enums.html
– MDN web docs on the in
operator: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/in