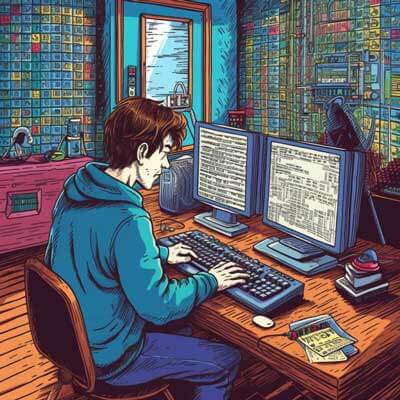
- Different ways to check if a value is in an enum
- Using switch statements to check if a value is in an enum
- Using arrays to check if a value is in an enum
- Checking if a value exists in an enum using object representation
- Comparing a value with the values in an enum
- Validating if a value is a valid member of an enum
- Checking if a value matches any of the values in an enum
- Exploring built-in functions or utilities in TypeScript for enum value checking
- The best way to verify if a value exists in a TypeScript enum
- Examining different methods to check if a value is in an enum
- Using code snippets to check if a value is in an enum
- Understanding the process of checking if a value is in an enum
- External Sources
Different ways to check if a value is in an enum
Enums in TypeScript are a useful feature that allow developers to define a set of named constants. These named constants can then be used to represent a finite set of possible values. In some cases, you may need to check if a value exists within an enum. TypeScript provides several ways to accomplish this. Let’s explore some of the different methods to check if a value is in an enum.
Related Article: How to Implement and Use Generics in Typescript
Using switch statements to check if a value is in an enum
One way to check if a value is in an enum is by using switch statements. Switch statements allow you to compare a value against multiple cases and execute different code blocks based on the matching case. Here’s an example of how you can use a switch statement to check if a value is in an enum:
enum Colors { Red = "RED", Blue = "BLUE", Green = "GREEN" } function checkColor(color: string): boolean { switch (color) { case Colors.Red: case Colors.Blue: case Colors.Green: return true; default: return false; } } console.log(checkColor("RED")); // Output: true console.log(checkColor("YELLOW")); // Output: false
In the above code, we define an enum called Colors
with three possible values: “RED”, “BLUE”, and “GREEN”. We then define a function checkColor
that takes a parameter color
and uses a switch statement to compare it against the enum values. If the color
parameter matches any of the enum values, the function returns true
, otherwise it returns false
.
Using arrays to check if a value is in an enum
Another way to check if a value is in an enum is by using arrays. You can create an array of the enum values and then use the includes
method to check if the value exists in the array. Here’s an example:
enum Colors { Red = "RED", Blue = "BLUE", Green = "GREEN" } function checkColor(color: string): boolean { const colorsArray: string[] = Object.values(Colors); return colorsArray.includes(color); } console.log(checkColor("RED")); // Output: true console.log(checkColor("YELLOW")); // Output: false
In the above code, we use the Object.values
method to get an array of all the values in the Colors
enum. We then use the includes
method to check if the color
parameter exists in the colorsArray
. If it exists, the function returns true
, otherwise it returns false
.
Checking if a value exists in an enum using object representation
In TypeScript, enums are represented as objects at runtime. This means that you can check if a value exists in an enum by using the in
operator to check if the value is a property of the enum object. Here’s an example:
enum Colors { Red = "RED", Blue = "BLUE", Green = "GREEN" } function checkColor(color: string): boolean { return color in Colors; } console.log(checkColor("RED")); // Output: true console.log(checkColor("YELLOW")); // Output: false
In the above code, we use the in
operator to check if the color
parameter is a property of the Colors
enum object. If it is a property, the function returns true
, otherwise it returns false
.
Related Article: How to Check If a String is in an Enum in TypeScript
Comparing a value with the values in an enum
You can also check if a value exists in an enum by directly comparing it with the enum values using the equality operator (==
or ===
). Here’s an example:
enum Colors { Red = "RED", Blue = "BLUE", Green = "GREEN" } function checkColor(color: string): boolean { return color === Colors.Red || color === Colors.Blue || color === Colors.Green; } console.log(checkColor("RED")); // Output: true console.log(checkColor("YELLOW")); // Output: false
In the above code, we compare the color
parameter with each enum value using the equality operator. If the color
parameter matches any of the enum values, the function returns true
, otherwise it returns false
.
Validating if a value is a valid member of an enum
If you want to check if a value is a valid member of an enum, TypeScript provides a type guard called keyof
. The keyof
type guard allows you to check if a value is a valid property of an object type. Here’s an example:
enum Colors { Red = "RED", Blue = "BLUE", Green = "GREEN" } function checkColor(color: string): color is keyof typeof Colors { return color in Colors; } console.log(checkColor("RED")); // Output: true console.log(checkColor("YELLOW")); // Output: false
In the above code, we use the keyof
type guard to check if the color
parameter is a valid property of the Colors
enum object. If it is a valid property, the function returns true
, otherwise it returns false
.
Checking if a value matches any of the values in an enum
If you want to check if a value matches any of the values in an enum, you can use the includes
method along with the Object.values
method to get an array of enum values. Here’s an example:
enum Colors { Red = "RED", Blue = "BLUE", Green = "GREEN" } function checkColor(color: string): boolean { return Object.values(Colors).includes(color); } console.log(checkColor("RED")); // Output: true console.log(checkColor("YELLOW")); // Output: false
In the above code, we use the Object.values
method to get an array of all the values in the Colors
enum. We then use the includes
method to check if the color
parameter exists in the array. If it exists, the function returns true
, otherwise it returns false
.
Related Article: Tutorial on TypeScript Dynamic Object Manipulation
Exploring built-in functions or utilities in TypeScript for enum value checking
TypeScript provides several built-in functions and utilities that can be used to check if a value is in an enum. One such utility is the enum
keyword itself, which allows you to define an enum and use it to check if a value exists within the enum. Here’s an example:
enum Colors { Red = "RED", Blue = "BLUE", Green = "GREEN" } function checkColor(color: string): boolean { return color in Colors; } console.log(checkColor("RED")); // Output: true console.log(checkColor("YELLOW")); // Output: false
In the above code, we define an enum called Colors
with three possible values: “RED”, “BLUE”, and “GREEN”. We then define a function checkColor
that takes a parameter color
and uses the in
operator to check if the color
parameter is a property of the Colors
enum object. If it is a property, the function returns true
, otherwise it returns false
.
The best way to verify if a value exists in a TypeScript enum
There is no one-size-fits-all answer to the best way to verify if a value exists in a TypeScript enum. The best approach depends on the specific use case and requirements of your project. However, some common methods include using switch statements, arrays, object representation, direct comparison, and built-in utilities provided by TypeScript.
If you need to check if a value is in an enum, you can consider the following factors to determine the best approach:
– The complexity of the enum: If the enum has a large number of values, using switch statements or direct comparison may become cumbersome. In this case, using arrays or object representation can provide a more concise and scalable solution.
– Performance requirements: Different methods have different performance characteristics. If performance is a critical factor, you may need to benchmark and compare the performance of different methods to choose the best one.
– Code readability and maintainability: Consider the readability and maintainability of the code. Choose an approach that is easy to understand and maintain by other developers working on the project.
Ultimately, the best way to verify if a value exists in a TypeScript enum is the one that meets your specific requirements and provides a balance between performance, readability, and maintainability.
Examining different methods to check if a value is in an enum
In this section, we have explored different methods to check if a value is in a TypeScript enum. We have looked at using switch statements, arrays, object representation, direct comparison, and built-in utilities provided by TypeScript. Each method has its own advantages and disadvantages, and the best approach depends on the specific use case and requirements of your project. By understanding the different methods available, you can choose the most appropriate method to check if a value exists in a TypeScript enum.
Related Article: Tutorial: Checking Enum Value Existence in TypeScript
Using code snippets to check if a value is in an enum
Here are some code snippets that demonstrate different methods to check if a value is in a TypeScript enum:
Using switch statements:
enum Colors { Red = "RED", Blue = "BLUE", Green = "GREEN" } function checkColor(color: string): boolean { switch (color) { case Colors.Red: case Colors.Blue: case Colors.Green: return true; default: return false; } } console.log(checkColor("RED")); // Output: true console.log(checkColor("YELLOW")); // Output: false
Using arrays:
enum Colors { Red = "RED", Blue = "BLUE", Green = "GREEN" } function checkColor(color: string): boolean { const colorsArray: string[] = Object.values(Colors); return colorsArray.includes(color); } console.log(checkColor("RED")); // Output: true console.log(checkColor("YELLOW")); // Output: false
Using object representation:
enum Colors { Red = "RED", Blue = "BLUE", Green = "GREEN" } function checkColor(color: string): boolean { return color in Colors; } console.log(checkColor("RED")); // Output: true console.log(checkColor("YELLOW")); // Output: false
Using direct comparison:
enum Colors { Red = "RED", Blue = "BLUE", Green = "GREEN" } function checkColor(color: string): boolean { return color === Colors.Red || color === Colors.Blue || color === Colors.Green; } console.log(checkColor("RED")); // Output: true console.log(checkColor("YELLOW")); // Output: false
Using built-in utilities in TypeScript:
enum Colors { Red = "RED", Blue = "BLUE", Green = "GREEN" } function checkColor(color: string): boolean { return color in Colors; } console.log(checkColor("RED")); // Output: true console.log(checkColor("YELLOW")); // Output: false
These code snippets demonstrate the different methods that can be used to check if a value is in a TypeScript enum. They provide a starting point for implementing enum value checking in your own projects.
Understanding the process of checking if a value is in an enum
The process of checking if a value is in a TypeScript enum involves comparing the value against the enum values using different methods such as switch statements, arrays, object representation, direct comparison, or built-in utilities provided by TypeScript.
When using switch statements, you define cases for each enum value and compare the value against these cases. If the value matches any of the cases, it is considered to be in the enum.
When using arrays, you create an array of the enum values and use the includes
method to check if the value exists in the array.
When using object representation, you use the in
operator to check if the value is a property of the enum object.
When using direct comparison, you compare the value with each enum value using the equality operator (==
or ===
). If the value matches any of the enum values, it is considered to be in the enum.
When using built-in utilities provided by TypeScript, such as the enum
keyword or the keyof
type guard, you can check if the value is a valid member of the enum.
External Sources
– TypeScript Handbook: Enums
– TypeScript Deep Dive: Enums
– TypeScript – Enums