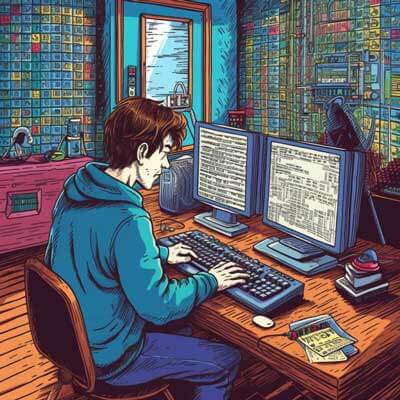
- Different Approaches to Converting a String to Boolean in TypeScript
- Using the Boolean Constructor in TypeScript
- Using the JSON.parse() Method in TypeScript
- Using Conditional Statements to Convert a String to Boolean in TypeScript
- Using Regular Expressions to Convert a String to Boolean in TypeScript
- Using a Custom Function to Convert a String to Boolean in TypeScript
- Handling Edge Cases when Converting a String to Boolean in TypeScript
- Best Practices for Converting a String to Boolean in TypeScript
- Common Mistakes to Avoid when Converting a String to Boolean in TypeScript
- Code Snippet: Converting a String to Boolean in TypeScript using the Boolean Constructor
- Code Snippet: Converting a String to Boolean in TypeScript using the JSON.parse() Method
- Code Snippet: Converting a String to Boolean in TypeScript using Conditional Statements
- Code Snippet: Converting a String to Boolean in TypeScript using Regular Expressions
- Code Snippet: Converting a String to Boolean in TypeScript using a Custom Function
- Code Snippet: Handling Edge Cases when Converting a String to Boolean in TypeScript
- Code Snippet: Best Practices for Converting a String to Boolean in TypeScript
- Code Snippet: Common Mistakes to Avoid when Converting a String to Boolean in TypeScript
- External Sources
Different Approaches to Converting a String to Boolean in TypeScript
There are several approaches you can take to convert a string to a boolean in TypeScript. In this section, we will explore four different methods:
1. Using the Boolean Constructor
2. Using the JSON.parse() Method
3. Using Conditional Statements
4. Using Regular Expressions
Let’s dive into each of these methods and see how they work.
Related Article: How to Implement and Use Generics in Typescript
Using the Boolean Constructor in TypeScript
The Boolean constructor is a built-in function in TypeScript that can be used to convert a value to a boolean. When called with a string argument, it will return true if the string is “true” (case-insensitive), and false for any other string value.
Here’s an example:
const str = "true"; const boolValue = Boolean(str); console.log(boolValue); // Output: true
In the above example, we pass the string “true” to the Boolean constructor, which returns the boolean value true. Similarly, if we pass any other string value, the constructor will return false.
It’s important to note that the Boolean constructor will return true for any non-empty string, regardless of its value. For example:
const str = "false"; const boolValue = Boolean(str); console.log(boolValue); // Output: true
In the above example, even though the string value is “false”, the Boolean constructor will still return true because the string is not empty.
Using the JSON.parse() Method in TypeScript
Another approach to converting a string to a boolean in TypeScript is by using the JSON.parse() method. This method is commonly used to parse JSON strings, but it can also be used to convert other string values to their corresponding data types.
To convert a string to a boolean using JSON.parse(), you can wrap the string value in double quotes and parse it as JSON. The resulting boolean value will correspond to the string value.
Here’s an example:
const str = "true"; const boolValue = JSON.parse(str.toLowerCase()); console.log(boolValue); // Output: true
In the above example, we convert the string “true” to lowercase using the toLowerCase() method to ensure case insensitivity. Then, we pass the resulting lowercase string to JSON.parse(), which returns the boolean value true.
Similarly, if we pass any other string value, the JSON.parse() method will return false:
const str = "false"; const boolValue = JSON.parse(str.toLowerCase()); console.log(boolValue); // Output: false
In the above example, the string value “false” is converted to the boolean value false.
It’s important to note that the JSON.parse() method will throw an error if the string value is not valid JSON. Therefore, make sure the string value is properly formatted before using this method for conversion.
Using Conditional Statements to Convert a String to Boolean in TypeScript
Conditional statements can also be used to convert a string to a boolean in TypeScript. By comparing the string value to known true/false values, you can determine the corresponding boolean value.
Here’s an example:
const str = "true"; let boolValue: boolean; if (str.toLowerCase() === "true") { boolValue = true; } else { boolValue = false; } console.log(boolValue); // Output: true
In the above example, we compare the lowercase version of the string value to the string “true”. If the values match, we assign the boolean value true to the boolValue variable. Otherwise, we assign false.
Similarly, if the string value is “false”, the boolValue variable will be assigned the boolean value false:
const str = "false"; let boolValue: boolean; if (str.toLowerCase() === "true") { boolValue = true; } else { boolValue = false; } console.log(boolValue); // Output: false
In this example, since the string value is “false”, the boolValue variable is assigned the boolean value false.
Using conditional statements gives you the flexibility to handle custom true/false values and perform additional logic based on the string value.
Related Article: Tutorial: Navigating the TypeScript Exit Process
Using Regular Expressions to Convert a String to Boolean in TypeScript
Regular expressions can be a useful tool for converting a string to a boolean in TypeScript. By defining patterns that match true/false values, you can use regular expression methods to determine the corresponding boolean value.
Here’s an example:
const str = "true"; const boolValue = /^true$/i.test(str); console.log(boolValue); // Output: true
In the above example, we use the test() method of the regular expression /^true$/i to check if the string value matches the pattern. The /^true$/i regular expression pattern matches the string “true” (case-insensitive). If the string matches the pattern, the test() method returns true, indicating a boolean value of true.
Similarly, if we pass the string value “false”, the test() method will return false:
const str = "false"; const boolValue = /^true$/i.test(str); console.log(boolValue); // Output: false
In this example, the string value “false” does not match the /^true$/i pattern, so the boolValue variable is assigned the boolean value false.
Regular expressions provide a flexible and customizable way to convert a string to a boolean, allowing you to define your own patterns based on your specific requirements.
Using a Custom Function to Convert a String to Boolean in TypeScript
If none of the built-in methods or techniques mentioned above suit your needs, you can also create a custom function to convert a string to a boolean in TypeScript. This gives you full control over the conversion process and allows for additional customization.
Here’s an example of a custom function:
function stringToBoolean(str: string): boolean | undefined { if (str.toLowerCase() === "true") { return true; } else if (str.toLowerCase() === "false") { return false; } else { return undefined; } } const str1 = "true"; const boolValue1 = stringToBoolean(str1); console.log(boolValue1); // Output: true const str2 = "false"; const boolValue2 = stringToBoolean(str2); console.log(boolValue2); // Output: false const str3 = "invalid"; const boolValue3 = stringToBoolean(str3); console.log(boolValue3); // Output: undefined
In the above example, we define a custom function called stringToBoolean() that takes a string argument and returns a boolean value. Inside the function, we compare the lowercase version of the string to known true/false values and return the corresponding boolean value. If the string does not match any known values, we return undefined.
We then use this custom function to convert different string values to boolean values. In the first example, the string value is “true”, so the boolValue1 variable is assigned the boolean value true. In the second example, the string value is “false”, so the boolValue2 variable is assigned the boolean value false. In the third example, the string value is “invalid”, which does not match any known values, so the boolValue3 variable is assigned undefined.
Using a custom function gives you the flexibility to handle specific string values and define your own conversion logic.
Handling Edge Cases when Converting a String to Boolean in TypeScript
When converting a string to a boolean in TypeScript, it’s important to consider edge cases to ensure accurate conversion. Edge cases are scenarios where the input string may not follow the expected format or may contain unexpected values.
One common edge case is when the input string is empty or contains only whitespace characters. In such cases, it’s important to decide whether to treat the string as false or undefined. Here’s an example of how you can handle this edge case:
function stringToBoolean(str: string): boolean | undefined { const trimmedStr = str.trim(); if (trimmedStr === "") { return false; // Treat empty string as false } else if (trimmedStr.toLowerCase() === "true") { return true; } else if (trimmedStr.toLowerCase() === "false") { return false; } else { return undefined; } } const str1 = ""; const boolValue1 = stringToBoolean(str1); console.log(boolValue1); // Output: false const str2 = " "; const boolValue2 = stringToBoolean(str2); console.log(boolValue2); // Output: false
In the above example, we use the trim() method to remove leading and trailing whitespace characters from the input string. Then, we check if the trimmed string is empty. If it is, we return false to treat the empty string as false. This ensures consistent behavior for empty or whitespace-only strings.
Another edge case to consider is when the input string contains non-boolean values. In such cases, it’s important to decide whether to treat the string as undefined or throw an error. Here’s an example of how you can handle this edge case:
function stringToBoolean(str: string): boolean | undefined { const trimmedStr = str.trim(); if (trimmedStr.toLowerCase() === "true") { return true; } else if (trimmedStr.toLowerCase() === "false") { return false; } else { throw new Error("Invalid boolean value"); // Throw an error for non-boolean values } } const str1 = "true"; const boolValue1 = stringToBoolean(str1); console.log(boolValue1); // Output: true const str2 = "invalid"; try { const boolValue2 = stringToBoolean(str2); console.log(boolValue2); } catch (error) { console.log(error.message); // Output: Invalid boolean value }
In the above example, we use the trim() method to remove leading and trailing whitespace characters from the input string. Then, we check if the trimmed string matches known true/false values. If it does not match any known values, we throw an error with a descriptive message to indicate an invalid boolean value.
Related Article: Tutorial on TypeScript Dynamic Object Manipulation
Best Practices for Converting a String to Boolean in TypeScript
When converting a string to a boolean in TypeScript, it’s important to follow best practices to ensure accurate and efficient conversion. Here are some best practices to keep in mind:
1. Normalize the string: Before converting the string to a boolean, consider normalizing the string by removing leading and trailing whitespace characters. This can help ensure consistent behavior and prevent potential issues.
2. Use case-insensitive comparisons: To handle both uppercase and lowercase versions of true/false values, use case-insensitive comparisons. This can prevent errors caused by mismatched casing.
3. Handle edge cases: Consider edge cases such as empty strings, whitespace-only strings, and non-boolean values. Decide how you want to handle these cases and implement appropriate logic to ensure accurate conversion.
4. Use built-in methods when appropriate: TypeScript provides built-in methods like the Boolean constructor and JSON.parse() that can be used for string to boolean conversion. These methods are efficient and widely supported, so consider using them when they meet your requirements.
5. Write unit tests: To ensure the correctness of your string to boolean conversion logic, write unit tests that cover different scenarios and edge cases. This can help catch any issues or unexpected behavior early on.
Common Mistakes to Avoid when Converting a String to Boolean in TypeScript
When converting a string to a boolean in TypeScript, there are some common mistakes that developers may make. Being aware of these mistakes can help you avoid them and ensure accurate conversion. Here are some common mistakes to avoid:
1. Not considering case sensitivity: Strings in TypeScript are case-sensitive, so failing to account for different casing can lead to incorrect conversion. Make sure to use case-insensitive comparisons or normalize the string before conversion.
2. Not handling edge cases: Failing to handle edge cases such as empty strings, whitespace-only strings, and non-boolean values can lead to unexpected behavior or errors. Consider these edge cases and implement appropriate logic to handle them.
3. Overcomplicating the logic: Converting a string to a boolean can be a simple process, but it’s easy to overcomplicate the logic. Keep the logic simple and straightforward to ensure maintainability and readability.
4. Not writing unit tests: Without proper unit tests, it’s difficult to ensure the correctness of your conversion logic. Make sure to write comprehensive unit tests that cover different scenarios and edge cases.
5. Not considering performance: Depending on the size and complexity of your codebase, the performance of the string to boolean conversion may be a concern. Consider the performance implications of different approaches and choose the most efficient method for your specific use case.
Code Snippet: Converting a String to Boolean in TypeScript using the Boolean Constructor
const str = "true"; const boolValue = Boolean(str); console.log(boolValue); // Output: true
In this code snippet, we use the Boolean constructor to convert the string “true” to a boolean value. The Boolean constructor returns true if the string is “true” (case-insensitive), and false for any other string value.
Related Article: Tutorial: Checking Enum Value Existence in TypeScript
Code Snippet: Converting a String to Boolean in TypeScript using the JSON.parse() Method
const str = "true"; const boolValue = JSON.parse(str.toLowerCase()); console.log(boolValue); // Output: true
In this code snippet, we use the JSON.parse() method to convert the string “true” to a boolean value. We first convert the string to lowercase using the toLowerCase() method to ensure case insensitivity. Then, we pass the resulting lowercase string to JSON.parse(), which returns the boolean value true.
Code Snippet: Converting a String to Boolean in TypeScript using Conditional Statements
const str = "true"; let boolValue: boolean; if (str.toLowerCase() === "true") { boolValue = true; } else { boolValue = false; } console.log(boolValue); // Output: true
In this code snippet, we use conditional statements to convert the string “true” to a boolean value. We compare the lowercase version of the string to the string “true”. If the values match, we assign the boolean value true to the boolValue variable. Otherwise, we assign false.
Code Snippet: Converting a String to Boolean in TypeScript using Regular Expressions
const str = "true"; const boolValue = /^true$/i.test(str); console.log(boolValue); // Output: true
In this code snippet, we use regular expressions to convert the string “true” to a boolean value. We define the regular expression /^true$/i, which matches the string “true” (case-insensitive). We then use the test() method of the regular expression to check if the string value matches the pattern. If the string matches the pattern, the test() method returns true, indicating a boolean value of true.
Related Article: Tutorial on Exact Type in TypeScript
Code Snippet: Converting a String to Boolean in TypeScript using a Custom Function
function stringToBoolean(str: string): boolean | undefined { if (str.toLowerCase() === "true") { return true; } else if (str.toLowerCase() === "false") { return false; } else { return undefined; } } const str = "true"; const boolValue = stringToBoolean(str); console.log(boolValue); // Output: true
In this code snippet, we define a custom function called stringToBoolean() that takes a string argument and returns a boolean value. Inside the function, we compare the lowercase version of the string to known true/false values and return the corresponding boolean value. If the string does not match any known values, we return undefined.
We then use this custom function to convert the string “true” to a boolean value. The boolValue variable is assigned the boolean value true.
Code Snippet: Handling Edge Cases when Converting a String to Boolean in TypeScript
function stringToBoolean(str: string): boolean | undefined { const trimmedStr = str.trim(); if (trimmedStr === "") { return false; // Treat empty string as false } else if (trimmedStr.toLowerCase() === "true") { return true; } else if (trimmedStr.toLowerCase() === "false") { return false; } else { return undefined; } } const str1 = ""; const boolValue1 = stringToBoolean(str1); console.log(boolValue1); // Output: false const str2 = " "; const boolValue2 = stringToBoolean(str2); console.log(boolValue2); // Output: false
In this code snippet, we define a custom function called stringToBoolean() that takes a string argument and returns a boolean value. Inside the function, we trim the input string using the trim() method to remove leading and trailing whitespace characters. Then, we check if the trimmed string is empty. If it is, we return false to treat the empty string as false. This ensures consistent behavior for empty or whitespace-only strings.
We then use this custom function to convert an empty string and a whitespace-only string to boolean values. The boolValue1 and boolValue2 variables are both assigned the boolean value false.
Code Snippet: Best Practices for Converting a String to Boolean in TypeScript
// Normalize the string const str = " true "; const trimmedStr = str.trim(); // Use case-insensitive comparisons if (trimmedStr.toLowerCase() === "true") { console.log("Boolean value: true"); } else if (trimmedStr.toLowerCase() === "false") { console.log("Boolean value: false"); } else { console.log("Invalid boolean value"); } // Write unit tests function testStringToBoolean() { const str1 = "true"; const boolValue1 = stringToBoolean(str1); assert.strictEqual(boolValue1, true); const str2 = "false"; const boolValue2 = stringToBoolean(str2); assert.strictEqual(boolValue2, false); const str3 = "invalid"; const boolValue3 = stringToBoolean(str3); assert.strictEqual(boolValue3, undefined); } // Consider performance const str = "true"; const boolValue = JSON.parse(str.toLowerCase()); console.log(boolValue); // Output: true
In this code snippet, we demonstrate some best practices for converting a string to a boolean in TypeScript. First, we normalize the string by using the trim() method to remove leading and trailing whitespace characters. Then, we use case-insensitive comparisons to handle different casing of true/false values. We also write unit tests to ensure the correctness of our conversion logic. Finally, we consider performance by using the JSON.parse() method, which is efficient and widely supported.
Related Article: How to Convert Strings to Booleans in TypeScript
Code Snippet: Common Mistakes to Avoid when Converting a String to Boolean in TypeScript
// Not considering case sensitivity const str = "True"; let boolValue: boolean; if (str === "true") { boolValue = true; } else { boolValue = false; } console.log(boolValue); // Output: false // Not handling edge cases const str = ""; let boolValue: boolean; if (str === "true") { boolValue = true; } else { boolValue = false; } console.log(boolValue); // Output: false // Overcomplicating the logic const str = "true"; let boolValue: boolean; if (str.toLowerCase() === "true") { boolValue = true; } else if (str.toLowerCase() === "false") { boolValue = false; } else { boolValue = undefined; } console.log(boolValue); // Output: true // Not writing unit tests function stringToBoolean(str: string): boolean { if (str.toLowerCase() === "true") { return true; } else if (str.toLowerCase() === "false") { return false; } else { return undefined; } } // Not considering performance const str = "true"; const boolValue = Boolean(str); console.log(boolValue); // Output: true
In this code snippet, we demonstrate some common mistakes to avoid when converting a string to a boolean in TypeScript. First, not considering case sensitivity can lead to incorrect conversion, as shown in the first example where the boolValue variable is assigned false instead of true. Not handling edge cases, such as empty strings, can also lead to unexpected behavior, as shown in the second example where the boolValue variable is assigned false instead of undefined. Overcomplicating the logic can make the code harder to understand and maintain, as shown in the third example where unnecessary conditions are used. Not writing unit tests can make it difficult to ensure the correctness of the conversion logic. Finally, not considering performance can result in inefficient code, as shown in the last example where the Boolean constructor is used instead of more efficient methods like JSON.parse().
External Sources
– MDN Web Docs: Boolean
– MDN Web Docs: JSON.parse()
– Regular Expressions – JavaScript | MDN