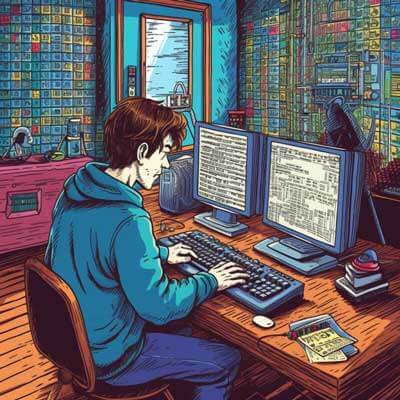
Table of Contents
Readonly Keyword in TypeScript
The readonly
keyword in TypeScript is used to define a read-only property or variable. Once a property or variable is marked as readonly
, it cannot be reassigned a new value. This provides a way to ensure that a property or variable remains constant and cannot be modified after its initial assignment.
Here's an example of using the readonly
keyword with a property:
class Person { readonly name: string; constructor(name: string) { this.name = name; } } const person = new Person("John"); console.log(person.name); // Output: John person.name = "Mike"; // Error: Cannot assign to 'name' because it is a read-only property
In the above example, the name
property of the Person
class is marked as readonly
. This means that once the name
property is assigned a value in the constructor, it cannot be changed.
The readonly
keyword can also be used with variables:
const PI: number = 3.14; console.log(PI); // Output: 3.14 PI = 3.14159; // Error: Cannot assign to 'PI' because it is a read-only property
In this example, the PI
variable is marked as readonly
, preventing it from being reassigned a new value.
Related Article: Tutorial: Date Comparison in TypeScript
Const Keyword in TypeScript
The const
keyword in TypeScript is used to define a constant variable. Once a variable is marked as const
, its value cannot be changed. This provides a way to ensure that a variable remains constant throughout the program.
Here's an example of using the const
keyword:
const PI: number = 3.14; console.log(PI); // Output: 3.14 PI = 3.14159; // Error: Cannot assign to 'PI' because it is a constant
In the above example, the PI
variable is marked as const
. This means that its value cannot be changed after it has been assigned.
Unlike readonly
, which can be applied to properties as well as variables, const
can only be used with variables.
Difference between Readonly and Const in TypeScript
While both readonly
and const
are used to define constants in TypeScript, there are some key differences between them.
The main difference is that readonly
can be used to define read-only properties as well as constants, whereas const
can only be used to define constant variables.
Another difference is that the readonly
keyword can be used with classes, interfaces, and type aliases, allowing you to define read-only properties for objects of those types. On the other hand, the const
keyword can only be used with variables.
Here's an example that demonstrates the difference between readonly
and const
:
interface Point { readonly x: number; readonly y: number; } const p1: Point = { x: 1, y: 2 }; console.log(p1.x, p1.y); // Output: 1, 2 p1.x = 3; // Error: Cannot assign to 'x' because it is a read-only property const p2 = { x: 3, y: 4 } as const; console.log(p2.x, p2.y); // Output: 3, 4 p2.x = 5; // Error: Cannot assign to 'x' because it is a constant
In this example, the Point
interface defines two read-only properties, x
and y
. The p1
variable is of type Point
and its properties are marked as readonly
, preventing them from being modified.
The p2
variable is created using the as const
syntax, which makes all of its properties readonly
by default. Any attempt to modify the x
property will result in an error.
When to Use Readonly in TypeScript
The readonly
keyword in TypeScript should be used when you want to create immutable objects or prevent modification of certain properties. By marking properties as readonly
, you ensure that their values cannot be changed once they have been assigned.
Here are some situations where you might want to use readonly
:
- When defining properties of an object that should not be modified after initialization.
- When defining properties of a class that should not be modified by external code.
- When defining properties of an interface or type alias that should not be modified when used to create new objects.
Consider the following example:
class Circle { readonly radius: number; constructor(radius: number) { this.radius = radius; } get area(): number { return Math.PI * this.radius ** 2; } } const circle = new Circle(5); console.log(circle.area); // Output: 78.53981633974483 circle.radius = 10; // Error: Cannot assign to 'radius' because it is a read-only property
In this example, the Circle
class has a radius
property that is marked as readonly
. This ensures that the radius
cannot be changed after it has been initialized in the constructor. This prevents accidental modification of the radius
property and helps maintain the integrity of the Circle
object.
Related Article: How to Set Default Values in TypeScript
When to Use Const in TypeScript
The const
keyword in TypeScript should be used when you want to define variables that should not be reassigned a new value. By marking variables as const
, you ensure that their values remain constant throughout the program.
Here are some situations where you might want to use const
:
- When defining constants that should not be changed.
- When defining variables that should not be reassigned a new value.
Consider the following example:
const PI: number = 3.14; console.log(PI); // Output: 3.14 PI = 3.14159; // Error: Cannot assign to 'PI' because it is a constant
In this example, the PI
variable is marked as const
, indicating that its value should not be changed. This ensures that the value of PI
remains constant throughout the program and prevents accidental modification.
Readonly vs Mutable in TypeScript
In TypeScript, the readonly
keyword is used to define read-only properties or variables, while the opposite of readonly
is mutable.
A mutable property or variable can be modified after its initial assignment. This means that its value can be changed, allowing for more flexibility in the program.
Here's an example that demonstrates the difference between readonly and mutable properties:
class Person { readonly name: string; age: number; constructor(name: string, age: number) { this.name = name; this.age = age; } } const person = new Person("John", 25); console.log(person.name, person.age); // Output: John, 25 person.name = "Mike"; // Error: Cannot assign to 'name' because it is a read-only property person.age = 30; // Valid: age is mutable and can be modified console.log(person.name, person.age); // Output: John, 30
In this example, the name
property of the Person
class is marked as readonly
, while the age
property is mutable. This means that the name
property cannot be modified after its initial assignment, while the age
property can be modified.
The use of readonly
and mutable properties depends on the specific requirements of your program. If you have properties that should not be modified after initialization, you can mark them as readonly
. If you need properties that can be modified, you can leave them as mutable.
Const vs Let in TypeScript
In TypeScript, the const
and let
keywords are used to declare variables. While both keywords are used to define variables, there are some differences between them.
The const
keyword is used to define variables that should not be reassigned a new value. Once a variable is marked as const
, its value cannot be changed.
On the other hand, the let
keyword is used to define variables that can be reassigned a new value. Variables declared with let
are mutable and can be modified throughout the program.
Here's an example that demonstrates the difference between const
and let
:
const PI: number = 3.14; console.log(PI); // Output: 3.14 PI = 3.14159; // Error: Cannot assign to 'PI' because it is a constant let count: number = 0; console.log(count); // Output: 0 count = 1; console.log(count); // Output: 1
In this example, the PI
variable is declared with the const
keyword, indicating that its value should not be changed. Any attempt to reassign a new value to PI
will result in an error.
On the other hand, the count
variable is declared with the let
keyword, indicating that its value can be changed. It is initially assigned a value of 0
, but it can be modified later in the program.
The choice between const
and let
depends on whether you need a variable to remain constant or if you need it to be mutable.
How Does the Readonly Keyword Affect Object Properties in TypeScript
In TypeScript, the readonly
keyword is used to define read-only properties for objects. When a property is marked as readonly
, it cannot be modified after its initial assignment.
Here's an example that demonstrates how the readonly
keyword affects object properties:
interface Point { readonly x: number; readonly y: number; } const origin: Point = { x: 0, y: 0 }; console.log(origin.x, origin.y); // Output: 0, 0 origin.x = 1; // Error: Cannot assign to 'x' because it is a read-only property origin.y = 1; // Error: Cannot assign to 'y' because it is a read-only property
In this example, the Point
interface defines two read-only properties, x
and y
. The origin
object is of type Point
and its properties are marked as readonly
, preventing them from being modified.
Any attempt to modify the x
or y
property will result in an error.
The readonly
keyword provides a way to enforce immutability for object properties, ensuring that they cannot be changed once they have been assigned.
Related Article: Tutorial: Converting String to Boolean in TypeScript
How Does the Const Keyword Affect Variable Assignment in TypeScript
In TypeScript, the const
keyword is used to define constant variables. Once a variable is marked as const
, its value cannot be changed. This means that the variable cannot be reassigned a new value.
Here's an example that demonstrates how the const
keyword affects variable assignment:
const PI: number = 3.14; console.log(PI); // Output: 3.14 PI = 3.14159; // Error: Cannot assign to 'PI' because it is a constant
In this example, the PI
variable is declared with the const
keyword, indicating that its value should not be changed. Any attempt to reassign a new value to PI
will result in an error.
The const
keyword provides a way to define constants in TypeScript, ensuring that their values remain constant throughout the program.
Are Readonly and Const Interchangeable in TypeScript
While readonly
and const
are used to define constants in TypeScript, they are not interchangeable.
The readonly
keyword is used to define read-only properties or variables. Once a property or variable is marked as readonly
, its value cannot be changed.
On the other hand, the const
keyword is used to define constant variables. Once a variable is marked as const
, its value cannot be reassigned a new value.
Here's an example that demonstrates the difference between readonly
and const
:
readonly MAX_VALUE: number = 100; const PI: number = 3.14; MAX_VALUE = 200; // Error: Cannot assign to 'MAX_VALUE' because it is a read-only property PI = 3.14159; // Error: Cannot assign to 'PI' because it is a constant
In this example, the MAX_VALUE
property is marked as readonly
, indicating that its value cannot be changed. Any attempt to modify the MAX_VALUE
property will result in an error.
The PI
variable is declared with the const
keyword, indicating that its value should not be changed. Any attempt to reassign a new value to PI
will also result in an error.
While both readonly
and const
are used to define constants, they have different use cases and cannot be used interchangeably.
External Sources
- TypeScript Documentation: Readonly modifier