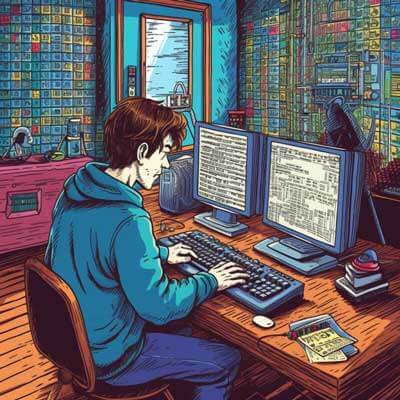
Table of Contents
In TypeScript, default values are used to assign a value to a variable or parameter if no value or undefined is provided. This helps in handling cases where a value may not be explicitly provided or when a value is intentionally left blank.
What is the Default Value in TypeScript?
A default value is a value that is automatically assigned to a variable or parameter if no other value is provided. In TypeScript, default values can be set for function parameters, function arguments, class properties, and more.
Related Article: Tutorial: Converting String to Bool in TypeScript
How to Set a Default Value for a Function Parameter in TypeScript?
To set a default value for a function parameter in TypeScript, you can simply assign a value to the parameter in the function declaration. Here's an example:
function greet(name: string = "World") { console.log(`Hello, ${name}!`); } greet(); // Output: Hello, World! greet("John"); // Output: Hello, John!
In the above example, the name
parameter in the greet
function is assigned a default value of "World". If no value is provided when calling the function, it will use the default value.
How to Use Default Arguments in TypeScript?
Default arguments allow you to provide default values for function arguments. This means that if an argument is not provided when calling the function, it will use the default value specified. Here's an example:
function multiply(a: number, b: number = 1) { return a * b; } console.log(multiply(5)); // Output: 5 console.log(multiply(5, 2)); // Output: 10
In the above example, the b
argument in the multiply
function is assigned a default value of 1. If no value is provided for b
when calling the function, it will use the default value of 1.
What is Default Initialization in TypeScript?
Default initialization is a feature in TypeScript that allows you to assign default values to class properties. When a class instance is created, these properties will be initialized with their default values if no other value is provided. Here's an example:
class Person { name: string = "John Doe"; age: number = 30; } const person = new Person(); console.log(person.name); // Output: John Doe console.log(person.age); // Output: 30
In the above example, the name
and age
properties of the Person
class are assigned default values of "John Doe" and 30, respectively. When a new instance of the Person
class is created, these properties will be initialized with their default values.
Related Article: How to Use the MouseEventHandlers in TypeScript
How to Use Default Imports in TypeScript?
Default imports allow you to import a default export from a module and assign it to a variable of your choice. This makes it easier to work with modules that have a default export. Here's an example:
// mathUtils.ts export default function add(a: number, b: number) { return a + b; } // main.ts import add from "./mathUtils"; console.log(add(2, 3)); // Output: 5
In the above example, the add
function is the default export of the mathUtils
module. When importing the add
function in the main.ts
file, we can use the import add from "./mathUtils"
syntax to assign the default export to a variable named add
.
How to Use Default Exports in TypeScript?
Default exports allow you to export a single value from a module as the default export. This means that when importing the module, you can choose any name for the imported value. Here's an example:
// mathUtils.ts export default function add(a: number, b: number) { return a + b; } // main.ts import myAddFunction from "./mathUtils"; console.log(myAddFunction(2, 3)); // Output: 5
In the above example, the add
function is the default export of the mathUtils
module. When importing the module in the main.ts
file, we can use the import myAddFunction from "./mathUtils"
syntax to assign the default export to a variable named myAddFunction
.
What are Default Modules in TypeScript?
Default modules are modules that have a default export. When a module has a default export, it can be imported using the import
statement without specifying a name for the imported value. Here's an example:
// mathUtils.ts export default { add(a: number, b: number) { return a + b; }, subtract(a: number, b: number) { return a - b; } } // main.ts import mathUtils from "./mathUtils"; console.log(mathUtils.add(2, 3)); // Output: 5 console.log(mathUtils.subtract(5, 3)); // Output: 2
In the above example, the mathUtils
module has a default export that is an object with add
and subtract
functions. When importing the module in the main.ts
file, we can use the import mathUtils from "./mathUtils"
syntax to access the exported functions.
What is a Default Interface in TypeScript?
In TypeScript, interfaces define the structure of an object. A default interface is an interface that is used as a default value for a variable or parameter. It provides a blueprint for the shape of the object and ensures that the object adheres to the defined structure. Here's an example:
interface Person { name: string; age: number; } function greet(person: Person = { name: "John Doe", age: 30 }) { console.log(`Hello, ${person.name}! You are ${person.age} years old.`); } greet(); // Output: Hello, John Doe! You are 30 years old. greet({ name: "Alice", age: 25 }); // Output: Hello, Alice! You are 25 years old.
In the above example, the Person
interface defines the structure of an object with name
and age
properties. The greet
function has a default parameter of type Person
, which means if no object is provided when calling the function, it will use the default value of { name: "John Doe", age: 30 }.
Related Article: Tutorial: Extending the Window Object in TypeScript
How to Define a Default Constructor in TypeScript?
In TypeScript, a constructor is a special method that is called when creating a new instance of a class. A default constructor is a constructor that is automatically generated if no constructor is defined in the class. Here's an example:
class Person { name: string; age: number; constructor(name: string = "John Doe", age: number = 30) { this.name = name; this.age = age; } } const person1 = new Person(); console.log(person1.name); // Output: John Doe console.log(person1.age); // Output: 30 const person2 = new Person("Alice", 25); console.log(person2.name); // Output: Alice console.log(person2.age); // Output: 25
In the above example, the Person
class has a default constructor that assigns default values to the name
and age
properties if no other values are provided.
How Does TypeScript Handle Default Values for Optional Parameters?
In TypeScript, optional parameters are parameters that can be omitted when calling a function. When a default value is provided for an optional parameter, TypeScript will use the default value if no value is provided during the function call. Here's an example:
function greet(name?: string, greeting: string = "Hello") { console.log(`${greeting}, ${name || "World"}!`); } greet(); // Output: Hello, World! greet("John"); // Output: Hello, John! greet("Alice", "Hi"); // Output: Hi, Alice!
In the above example, the name
parameter is optional, and the greeting
parameter has a default value of "Hello". If no value is provided for name
, it will default to "World". If no value is provided for greeting
, it will default to "Hello".