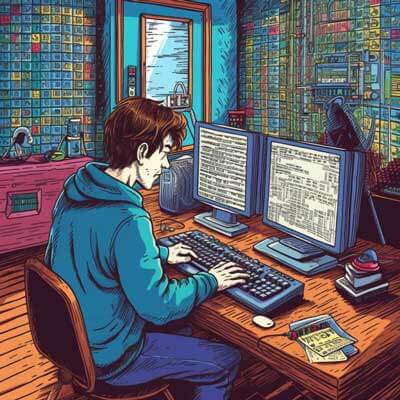
- Iterating Over Object Keys in TypeScript
- Iterating Over Object Values in TypeScript
- Iterating Over a Dictionary in TypeScript
- Using for…in Loop to Iterate Over Objects in TypeScript
- Using for…in Loop to Iterate Over Dictionaries in TypeScript
- Using for…of Loop to Iterate Over Objects in TypeScript
- Using for…of Loop to Iterate Over Dictionaries in TypeScript
- Using forEach to Iterate Over Objects in TypeScript
- Using forEach to Iterate Over Dictionaries in TypeScript
- The Difference Between for…in and for…of Loops in TypeScript
- External Sources
Iterating Over Object Keys in TypeScript
In TypeScript, you can easily iterate over the keys of an object using a for…in loop. The for…in loop allows you to loop through all the enumerable properties of an object, including its inherited properties. Here’s an example:
const obj = {a: 1, b: 2, c: 3}; for (let key in obj) { console.log(key); // Output: a, b, c }
In the above example, we define an object called obj
with three key-value pairs. We then use a for…in loop to iterate over the keys of the object and log each key to the console.
Another way to iterate over object keys in TypeScript is by using the Object.keys()
method. This method returns an array of the object’s own enumerable property names. Here’s an example:
const obj = {a: 1, b: 2, c: 3}; Object.keys(obj).forEach(key => { console.log(key); // Output: a, b, c });
In this example, we use the Object.keys()
method to get an array of the object’s keys and then use the forEach()
method to iterate over the array and log each key to the console.
Related Article: How to Implement and Use Generics in Typescript
Iterating Over Object Values in TypeScript
To iterate over the values of an object in TypeScript, you can use the Object.values()
method. This method returns an array of the object’s own enumerable property values. Here’s an example:
const obj = {a: 1, b: 2, c: 3}; Object.values(obj).forEach(value => { console.log(value); // Output: 1, 2, 3 });
In this example, we use the Object.values()
method to get an array of the object’s values and then use the forEach()
method to iterate over the array and log each value to the console.
Iterating Over a Dictionary in TypeScript
In TypeScript, a dictionary is essentially an object where the keys are strings and the values can be of any type. To iterate over a dictionary in TypeScript, you can use the same methods mentioned earlier for iterating over object keys and values. Here’s an example:
const dictionary = {name: "John", age: 30, city: "New York"}; for (let key in dictionary) { console.log(key + ": " + dictionary[key]); // Output: name: John, age: 30, city: New York } Object.keys(dictionary).forEach(key => { console.log(key + ": " + dictionary[key]); // Output: name: John, age: 30, city: New York }); Object.values(dictionary).forEach(value => { console.log(value); // Output: John, 30, New York });
In this example, we define a dictionary called dictionary
with three key-value pairs. We use a for…in loop, Object.keys()
, and Object.values()
to iterate over the dictionary and log the keys and values to the console.
Using for…in Loop to Iterate Over Objects in TypeScript
The for…in loop in TypeScript allows you to iterate over the enumerable properties of an object. It iterates over the keys of the object, including inherited properties. Here’s an example:
const obj = {a: 1, b: 2, c: 3}; for (let key in obj) { console.log(key); // Output: a, b, c }
In this example, we define an object called obj
with three key-value pairs. We use a for…in loop to iterate over the keys of the object and log each key to the console.
Related Article: How to Check If a String is in an Enum in TypeScript
Using for…in Loop to Iterate Over Dictionaries in TypeScript
You can also use the for…in loop to iterate over dictionaries in TypeScript, as dictionaries are essentially objects with string keys and any type of values. Here’s an example:
const dictionary = {name: "John", age: 30, city: "New York"}; for (let key in dictionary) { console.log(key + ": " + dictionary[key]); // Output: name: John, age: 30, city: New York }
In this example, we define a dictionary called dictionary
with three key-value pairs. We use a for…in loop to iterate over the keys of the dictionary and log each key-value pair to the console.
Using for…of Loop to Iterate Over Objects in TypeScript
The for…of loop in TypeScript allows you to iterate over the iterable objects, such as arrays, strings, and certain built-in objects. However, it cannot be used directly to iterate over objects or dictionaries. Here’s an example of using for…of loop to iterate over an array:
const arr = [1, 2, 3]; for (let value of arr) { console.log(value); // Output: 1, 2, 3 }
In this example, we define an array called arr
with three elements. We use a for…of loop to iterate over the elements of the array and log each element to the console.
Using for…of Loop to Iterate Over Dictionaries in TypeScript
To iterate over a dictionary in TypeScript using a for…of loop, you first need to convert the dictionary into an array of key-value pairs using the Object.entries()
method. Here’s an example:
const dictionary = {name: "John", age: 30, city: "New York"}; for (let [key, value] of Object.entries(dictionary)) { console.log(key + ": " + value); // Output: name: John, age: 30, city: New York }
In this example, we define a dictionary called dictionary
with three key-value pairs. We use the Object.entries()
method to convert the dictionary into an array of key-value pairs. Then, we use a for…of loop to iterate over the array and log each key-value pair to the console.
Related Article: Tutorial on TypeScript Dynamic Object Manipulation
Using forEach to Iterate Over Objects in TypeScript
The forEach()
method is available on arrays in TypeScript, but not on objects or dictionaries directly. However, you can still use it by first converting the object or dictionary into an array using the Object.entries()
method. Here’s an example:
const obj = {a: 1, b: 2, c: 3}; Object.entries(obj).forEach(([key, value]) => { console.log(key + ": " + value); // Output: a: 1, b: 2, c: 3 });
In this example, we define an object called obj
with three key-value pairs. We use the Object.entries()
method to convert the object into an array of key-value pairs. Then, we use the forEach()
method to iterate over the array and log each key-value pair to the console.
Using forEach to Iterate Over Dictionaries in TypeScript
To iterate over a dictionary in TypeScript using the forEach()
method, you first need to convert the dictionary into an array of key-value pairs using the Object.entries()
method. Here’s an example:
const dictionary = {name: "John", age: 30, city: "New York"}; Object.entries(dictionary).forEach(([key, value]) => { console.log(key + ": " + value); // Output: name: John, age: 30, city: New York });
In this example, we define a dictionary called dictionary
with three key-value pairs. We use the Object.entries()
method to convert the dictionary into an array of key-value pairs. Then, we use the forEach()
method to iterate over the array and log each key-value pair to the console.
The Difference Between for…in and for…of Loops in TypeScript
The for…in loop is used to iterate over the keys of an object, including inherited properties, whereas the for…of loop is used to iterate over the elements of an iterable object, such as an array, string, or certain built-in objects. The for…in loop is typically used for iterating over objects or dictionaries, while the for…of loop is used for iterating over arrays or other iterable objects.
Related Article: Tutorial: Checking Enum Value Existence in TypeScript
External Sources
– TypeScript Handbook: Iterators and Generators
– MDN Web Docs: for…in
– MDN Web Docs: for…of