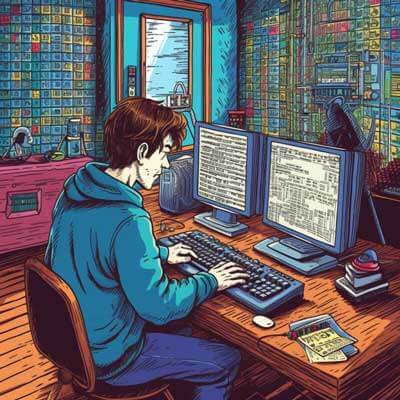
Table of Contents
Converting Strings to Booleans Using the Boolean() Function
One of the simplest ways to convert a string to a boolean value in TypeScript is by using the Boolean() function. The Boolean() function takes a value as an argument and returns the corresponding boolean representation of that value.
Here's an example of how to use the Boolean() function to convert a string to a boolean:
const str = "true"; const boolValue = Boolean(str); console.log(boolValue); // Output: true
In the above example, the Boolean() function is called with the string "true" as the argument. The function returns the boolean value true, which is then assigned to the variable boolValue.
It's important to note that the Boolean() function follows a specific set of rules to determine the boolean representation of a value. According to these rules, the string "true" (case-insensitive) will be converted to true, while any other non-empty string will be converted to true. An empty string, as well as the string "false" (case-insensitive), will be converted to false.
Here's an example that demonstrates the boolean conversion rules of the Boolean() function:
console.log(Boolean("true")); // Output: true console.log(Boolean("false")); // Output: true console.log(Boolean("")); // Output: false console.log(Boolean("any other string")); // Output: true
In the above example, the Boolean() function is called with different strings as arguments. The console.log() statements show the corresponding boolean representation of each string.
Using the Boolean() function is a straightforward and efficient way to convert strings to booleans in TypeScript. However, it's important to keep in mind the boolean conversion rules of the function to ensure accurate results.
Related Article: How to Verify if a Value is in Enum in TypeScript
Using the === Operator for String to Boolean Conversion
In addition to using the Boolean() function, you can also use the === operator for string to boolean conversion in TypeScript. The === operator is the strict equality operator in TypeScript, and it returns true if the operands are equal and of the same type.
Here's an example of how to use the === operator for string to boolean conversion:
const str = "true"; const boolValue = str === "true"; console.log(boolValue); // Output: true
In the above example, the === operator compares the string variable str with the string "true". If the strings are equal, the operator returns true, indicating that the string represents a boolean value.
It's important to note that the === operator performs a strict comparison, meaning that it compares both the value and the type of the operands. If you want to convert a string to a boolean using the === operator, you need to ensure that the string has the same value and type as the desired boolean representation.
Here's an example that demonstrates the use of the === operator for string to boolean conversion:
console.log("true" === "true"); // Output: true console.log("false" === "true"); // Output: false console.log("" === ""); // Output: true console.log("any other string" === "true"); // Output: false
In the above example, the === operator is used to compare different strings. The console.log() statements show the result of each comparison.
Using the === operator for string to boolean conversion can be useful in situations where you need to perform a strict comparison and ensure both the value and type of the operands are the same.
Converting Strings to Booleans with if-else Statements
Another approach to converting strings to booleans in TypeScript is by using if-else statements. By utilizing conditional statements, you can explicitly check the value of a string and assign the corresponding boolean value.
Here's an example of how to use if-else statements for string to boolean conversion:
const str = "true"; let boolValue: boolean; if (str === "true") { boolValue = true; } else if (str === "false") { boolValue = false; } else { // Handle invalid string boolValue = false; } console.log(boolValue); // Output: true
In the above example, the value of the string variable str is checked using if-else statements. If the string is equal to "true", the boolValue variable is assigned the value true. If the string is equal to "false", the boolValue variable is assigned the value false. If neither condition is met, indicating an invalid string, the boolValue variable is assigned the value false.
Using if-else statements for string to boolean conversion provides flexibility in handling different string values. You can define specific conditions for different string representations and handle them accordingly.
Using Regular Expressions for String to Boolean Conversion
Regular expressions can also be used for converting strings to booleans in TypeScript. By defining specific patterns and using regular expression methods, you can extract boolean values from strings.
Here's an example of how to use regular expressions for string to boolean conversion:
const str = "1"; const pattern = /^(true|1|yes)$/i; const boolValue = pattern.test(str); console.log(boolValue); // Output: true
In the above example, a regular expression pattern is defined using the /^(true|1|yes)$/i syntax. This pattern matches the strings "true", "1", and "yes" in a case-insensitive manner. The test() method is then used to check if the string variable str matches the pattern. If a match is found, the method returns true, indicating that the string represents a boolean value.
Regular expressions provide a useful and flexible way to convert strings to booleans in TypeScript. By defining specific patterns, you can handle different string representations and extract the corresponding boolean values.
Related Article: Tutorial: Date Comparison in TypeScript
Converting Strings to Booleans with Ternary Operators
Ternary operators offer a concise way to convert strings to booleans in TypeScript. By using the ? : syntax, you can define a condition and assign different values based on the result of the condition.
Here's an example of how to use ternary operators for string to boolean conversion:
const str = "true"; const boolValue = str === "true" ? true : false; console.log(boolValue); // Output: true
In the above example, the === operator is used to compare the string variable str with the string "true". If the strings are equal, the condition evaluates to true, and the value true is assigned to the boolValue variable. Otherwise, if the condition evaluates to false, the value false is assigned to the boolValue variable.
Using ternary operators for string to boolean conversion can simplify your code and make it more concise. However, it's important to ensure that the condition evaluates to a boolean value to avoid unexpected results.
Handling Edge Cases in String to Boolean Conversion
When converting strings to booleans in TypeScript, it's important to consider edge cases and handle them appropriately. Edge cases refer to situations where the input string may not conform to the expected boolean representations.
Here are some common edge cases to consider when converting strings to booleans:
1. Empty String: If the input string is empty, it's important to handle this case and assign an appropriate boolean value. In most cases, an empty string should be converted to false.
const str = ""; const boolValue = Boolean(str); // false
2. Invalid String: If the input string does not match any of the expected boolean representations, it's important to handle this case and assign an appropriate boolean value. In most cases, an invalid string should be converted to false.
const str = "invalid"; const boolValue = Boolean(str); // false
3. Case Sensitivity: When comparing strings for boolean conversion, it's important to consider case sensitivity. In most cases, the comparison should be case-insensitive to cover different variations of boolean representations.
const str = "True"; const boolValue = str.toLowerCase() === "true"; // true
Best Practices for Converting Strings to Booleans in TypeScript
When converting strings to booleans in TypeScript, it's important to follow best practices to ensure efficient and reliable code. Here are some best practices to consider:
1. Use the Boolean() function for simple and straightforward conversions. The Boolean() function provides a concise way to convert strings to booleans based on a specific set of rules.
2. Use the === operator for strict comparisons. If you need to perform a strict comparison and ensure both the value and type of the operands are the same, the === operator can be a reliable choice.
3. Utilize if-else statements for handling different string representations. If you have specific conditions for different string values, if-else statements can provide flexibility in handling those cases.
4. Consider using regular expressions for more complex string representations. Regular expressions offer a useful way to define specific patterns and extract boolean values from strings.
5. Take into account edge cases and handle them appropriately. Empty strings and invalid strings should be handled explicitly to avoid unexpected results.
6. Follow consistent naming conventions and use descriptive variable names. This helps improve code readability and maintainability.
7. Write unit tests to validate your string to boolean conversion code. Unit tests can help identify any issues or unexpected behavior in your code.
Common Mistakes to Avoid in String to Boolean Conversion
When converting strings to booleans in TypeScript, there are some common mistakes that developers may make. By being aware of these mistakes, you can avoid potential issues and ensure accurate conversions. Here are some common mistakes to avoid:
1. Forgetting to handle edge cases: It's important to handle edge cases such as empty strings and invalid strings to avoid unexpected results. Failing to handle these cases can lead to inaccurate conversions.
2. Not considering case sensitivity: When comparing strings for boolean conversion, it's important to consider case sensitivity. Comparisons should be case-insensitive to cover different variations of boolean representations.
3. Using the wrong comparison operator: Using the wrong comparison operator can lead to incorrect results. Make sure to use the === operator for strict comparisons and the == operator for loose comparisons.
4. Overcomplicating the code: String to boolean conversion can be simple and straightforward. Avoid overcomplicating the code by using unnecessary conditions or complex logic.
5. Not testing the code thoroughly: It's important to thoroughly test your string to boolean conversion code to ensure it works as expected. Write unit tests to validate the behavior of your code and catch any potential issues.
Related Article: Tutorial: Extending the Window Object in TypeScript
Exploring External Libraries for String to Boolean Conversion
While TypeScript provides built-in functionality for converting strings to booleans, you may also consider exploring external libraries that offer additional features and flexibility. These libraries can provide more advanced string parsing capabilities or support for specific use cases.
One popular library for string to boolean conversion in JavaScript and TypeScript is "lodash". Lodash is a utility library that provides a wide range of functions for common programming tasks, including string manipulation and conversion.
Here's an example of how to use the "lodash" library for string to boolean conversion:
import { toLower } from "lodash"; const str = "TRUE"; const boolValue = toLower(str) === "true"; console.log(boolValue); // Output: true
In the above example, the toLower() function from the "lodash" library is used to convert the input string to lowercase. The === operator is then used to compare the lowercase string with the string "true", resulting in the boolean value true.
Creating Custom Functions for String to Boolean Conversion
In addition to using built-in functions and external libraries, you can also create custom functions for string to boolean conversion in TypeScript. Custom functions allow you to define your own logic and handle specific requirements.
Here's an example of how to create a custom function for string to boolean conversion:
function stringToBoolean(str: string): boolean { if (str === "true") { return true; } else if (str === "false") { return false; } else { return false; } } const boolValue = stringToBoolean("true"); console.log(boolValue); // Output: true
In the above example, a custom function called stringToBoolean() is defined. The function takes a string as an argument and returns a boolean value based on the string representation. The function uses if-else statements to handle different string values and return the appropriate boolean value.
Custom functions provide flexibility and allow you to define your own logic for string to boolean conversion. They can be useful in situations where you have specific requirements or need to handle complex string representations.
Mapping Specific Strings to Boolean Values in TypeScript
In some cases, you may need to map specific strings to boolean values in TypeScript. This can be achieved by using an object or a map to define the mapping between strings and boolean values. By utilizing this mapping, you can easily convert strings to booleans based on the defined rules.
Here's an example of how to map specific strings to boolean values in TypeScript:
const stringToBooleanMap: { [key: string]: boolean } = { "true": true, "false": false, "1": true, "0": false, "yes": true, "no": false, }; const str = "yes"; const boolValue = stringToBooleanMap[str]; console.log(boolValue); // Output: true
In the above example, an object called stringToBooleanMap is defined, which maps specific strings to boolean values. The keys of the object represent the strings, and the values represent the corresponding boolean values. By accessing the object with a specific string, you can retrieve the corresponding boolean value.
Mapping specific strings to boolean values can be useful when you have a predefined set of string representations and want to convert them to booleans based on your own rules.
Checking if a String Represents a Boolean Value in TypeScript
In some cases, you may need to check if a string represents a boolean value in TypeScript, without actually converting it to a boolean. This can be achieved by using the === operator to perform a strict comparison between the string and the expected boolean representations.
Here's an example of how to check if a string represents a boolean value in TypeScript:
function isBooleanString(str: string): boolean { return str === "true" || str === "false"; } console.log(isBooleanString("true")); // Output: true console.log(isBooleanString("false")); // Output: true console.log(isBooleanString("any other string")); // Output: false
In the above example, a custom function called isBooleanString() is defined. The function takes a string as an argument and returns true if the string represents a boolean value (i.e., "true" or "false"). The function uses the === operator to perform a strict comparison between the string and the expected boolean representations.
Related Article: How to Configure the Awesome TypeScript Loader
Frequently Asked Questions about String to Boolean Conversion in TypeScript
Q: Can I convert any string to a boolean in TypeScript?
A: No, you can only convert specific strings to booleans in TypeScript. The Boolean() function and other methods we discussed in this tutorial follow specific rules to determine the boolean representation of a string.
Q: What happens if I try to convert an empty string to a boolean in TypeScript?
A: An empty string will be converted to false by the Boolean() function and other methods we discussed in this tutorial. This is consistent with the boolean conversion rules defined in TypeScript.
Q: How can I handle case sensitivity when converting strings to booleans in TypeScript?
A: To handle case sensitivity, you can convert the input string to lowercase or uppercase before performing the comparison. This ensures that the comparison is case-insensitive.
Q: Can I use regular expressions to convert complex string representations to booleans in TypeScript?
A: Yes, regular expressions can be used to convert complex string representations to booleans in TypeScript. By defining specific patterns and using regular expression methods, you can extract boolean values from strings based on your own rules.
Q: Are there any external libraries that can help with string to boolean conversion in TypeScript?
A: Yes, there are external libraries like "lodash" that provide additional functionality for string to boolean conversion in TypeScript. These libraries can offer more advanced string parsing capabilities or support for specific use cases.