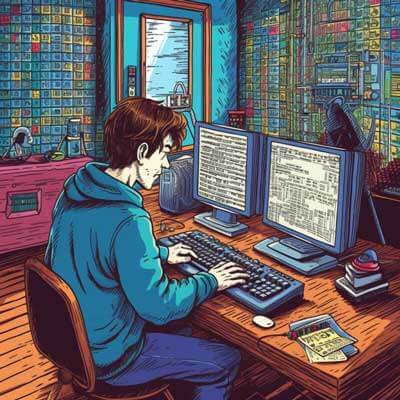
Table of Contents
Different Ways to Convert a String to Boolean in TypeScript
When working with TypeScript, there may be times when you need to convert a string to a boolean value. Fortunately, TypeScript provides several built-in methods and techniques to accomplish this task. In this tutorial, we will explore different ways to convert a string to a boolean in TypeScript.
Related Article: How to Work with Dynamic Objects in TypeScript
Using the Boolean Constructor
One of the simplest and most straightforward ways to convert a string to a boolean in TypeScript is by using the Boolean constructor. The Boolean constructor function takes a value and returns a boolean representation of that value. When converting a string to a boolean, passing the string to the Boolean constructor will return true if the string is not empty, and false otherwise.
Here's an example:
const str = "true";const boolValue = Boolean(str);console.log(boolValue); // Output: true
In the example above, we pass the string "true" to the Boolean constructor, which returns true because the string is not empty. If we were to pass an empty string or any other non-truthy value, the Boolean constructor would return false.
Using the === Operator
Another way to convert a string to a boolean in TypeScript is by using the strict equality (===) operator. The strict equality operator compares the value and type of two operands and returns true if they are equal, and false otherwise.
To convert a string to a boolean using the === operator, we can compare the string to a predefined string value that represents true. If the strings match, the result will be true; otherwise, it will be false.
Here's an example:
const str = "true";const boolValue = str === "true";console.log(boolValue); // Output: true
In the example above, we compare the string "true" to the predefined string value "true". Since the two strings match, the result of the comparison is true.
Using the toLowerCase() Method
The toLowerCase() method is a built-in method in TypeScript that converts a string to lowercase. This method can be used to convert a string to a boolean by first converting the string to lowercase and then comparing it to a predefined lowercase string value that represents true.
Here's an example:
const str = "TRUE";const boolValue = str.toLowerCase() === "true";console.log(boolValue); // Output: true
In the example above, we first convert the string "TRUE" to lowercase using the toLowerCase() method and then compare it to the predefined lowercase string value "true". Since the two strings match, the result of the comparison is true.
Related Article: How to Convert a String to a Number in TypeScript
Using the toUpperCase() Method
Similar to the toLowerCase() method, TypeScript also provides the toUpperCase() method, which converts a string to uppercase. This method can be used to convert a string to a boolean by first converting the string to uppercase and then comparing it to a predefined uppercase string value that represents true.
Here's an example:
const str = "true";const boolValue = str.toUpperCase() === "TRUE";console.log(boolValue); // Output: true
In the example above, we first convert the string "true" to uppercase using the toUpperCase() method and then compare it to the predefined uppercase string value "TRUE". Since the two strings match, the result of the comparison is true.
Using Regular Expressions for String to Boolean Conversion
Regular expressions can be a useful tool for pattern matching and string manipulation. In TypeScript, we can use regular expressions to convert a string to a boolean by matching the string against a pattern that represents a boolean value.
Here's an example:
const str = "true";const pattern = /^(true|1|yes|on)$/i;const boolValue = pattern.test(str);console.log(boolValue); // Output: true
In the example above, we define a regular expression pattern that matches the strings "true", "1", "yes", and "on". The i flag at the end of the pattern makes the matching case-insensitive. We then use the test() method of the regular expression object to check if the string matches the pattern. If the string matches the pattern, the test() method returns true, indicating that the string can be converted to a boolean.
Converting 'True' and 'False' Strings to Boolean
In some scenarios, you may need to convert specific strings like "true" and "false" to boolean values. TypeScript provides several approaches to achieve this conversion.
One common approach is to use the strict equality operator (===) to compare the string to the predefined string values "true" and "false". If the string matches either of these values, the result will be true or false, respectively.
Here's an example:
const str = "true";const boolValue = str === "true";console.log(boolValue); // Output: true
In the example above, we compare the string "true" to the predefined string value "true". Since the two strings match, the result of the comparison is true.
Similarly, we can convert the string "false" to a boolean using the same approach:
const str = "false";const boolValue = str === "false";console.log(boolValue); // Output: true
In this example, we compare the string "false" to the predefined string value "false", resulting in the conversion to a boolean value of true.
Converting 'Yes' and 'No' Strings to Boolean
In some cases, you may need to convert strings like "yes" and "no" to boolean values. TypeScript provides various methods to achieve this conversion.
One approach is to use the toLowerCase() method to convert the string to lowercase and then compare it to the predefined lowercase string values "yes" and "no". If the string matches either of these values, the result will be true or false, respectively.
Here's an example:
const str = "Yes";const boolValue = str.toLowerCase() === "yes";console.log(boolValue); // Output: true
In the example above, we first convert the string "Yes" to lowercase using the toLowerCase() method and then compare it to the predefined lowercase string value "yes". Since the two strings match, the result of the comparison is true.
Similarly, we can convert the string "No" to a boolean using the same approach:
const str = "No";const boolValue = str.toLowerCase() === "no";console.log(boolValue); // Output: true
In this example, we convert the string "No" to lowercase and compare it to the predefined lowercase string value "no", resulting in the conversion to a boolean value of true.
Related Article: Handling Types with TypeScript-eslint/ban-types
Converting 'On' and 'Off' Strings to Boolean
In certain situations, you may need to convert strings like "on" and "off" to boolean values. TypeScript provides various techniques for achieving this conversion.
One approach is to use the toLowerCase() method to convert the string to lowercase and then compare it to the predefined lowercase string values "on" and "off". If the string matches either of these values, the result will be true or false, respectively.
Here's an example:
const str = "On";const boolValue = str.toLowerCase() === "on";console.log(boolValue); // Output: true
In the example above, we first convert the string "On" to lowercase using the toLowerCase() method and then compare it to the predefined lowercase string value "on". Since the two strings match, the result of the comparison is true.
Similarly, we can convert the string "Off" to a boolean using the same approach:
const str = "Off";const boolValue = str.toLowerCase() === "off";console.log(boolValue); // Output: true
In this example, we convert the string "Off" to lowercase and compare it to the predefined lowercase string value "off", resulting in the conversion to a boolean value of true.
Converting '1' and '0' Strings to Boolean
In specific scenarios, you may need to convert strings like "1" and "0" to boolean values. TypeScript provides several approaches to achieve this conversion.
One common approach is to use the strict equality operator (===) to compare the string to the predefined string values "1" and "0". If the string matches either of these values, the result will be true or false, respectively.
Here's an example:
const str = "1";const boolValue = str === "1";console.log(boolValue); // Output: true
In the example above, we compare the string "1" to the predefined string value "1". Since the two strings match, the result of the comparison is true.
Similarly, we can convert the string "0" to a boolean using the same approach:
const str = "0";const boolValue = str === "0";console.log(boolValue); // Output: true
In this example, we compare the string "0" to the predefined string value "0", resulting in the conversion to a boolean value of true.
Handling Invalid or Unrecognized Values when Converting a String to Boolean
When converting a string to a boolean in TypeScript, it's important to consider how to handle invalid or unrecognized values. By default, TypeScript does not provide a built-in mechanism to handle such cases, so it's up to the developer to implement custom logic.
One approach is to use a conditional statement or a switch case to handle different cases. For example, you can have a default value or return an error message when the input string does not match any predefined values.
Here's an example using a conditional statement:
function convertStringToBoolean(str: string): boolean { if (str === "true") { return true; } else if (str === "false") { return false; } else { // Handle unrecognized values here return false; // or throw an error }}const str = "invalid";const boolValue = convertStringToBoolean(str);console.log(boolValue); // Output: false
In the example above, we define a function convertStringToBoolean
that takes a string as input and returns a boolean value. Inside the function, we use a conditional statement to check if the input string matches the predefined string values "true" and "false". If the input string matches either of these values, the corresponding boolean value is returned. Otherwise, we handle unrecognized values by returning a default value (false in this case) or throwing an error.
Customizing the Conversion Process from String to Boolean
In some cases, you may need to customize the conversion process from a string to a boolean in TypeScript. TypeScript provides various techniques to achieve this customization, depending on the specific requirements of your application.
One approach is to define a mapping object that associates certain strings with boolean values. You can then use this mapping object to convert the input string to the corresponding boolean value.
Here's an example:
const mapping = { "true": true, "yes": true, "on": true, "false": false, "no": false, "off": false,};function convertStringToBoolean(str: string): boolean { return mapping[str.toLowerCase()] || false;}const str = "yes";const boolValue = convertStringToBoolean(str);console.log(boolValue); // Output: true
In the example above, we define a mapping object that associates the strings "true", "yes", and "on" with the boolean value true, and the strings "false", "no", and "off" with the boolean value false. Inside the convertStringToBoolean
function, we convert the input string to lowercase using the toLowerCase() method and use it as a key to retrieve the corresponding boolean value from the mapping object. If the input string does not match any keys in the mapping object, the expression mapping[str.toLowerCase()]
will be falsy, and the default value (false in this case) will be returned.
Related Article: Comparing Go with TypeScript
Exploring Implicit Conversion from String to Boolean in TypeScript
In TypeScript, there is implicit conversion between certain types, including string and boolean. Implicit conversion means that TypeScript automatically converts a value from one type to another without the need for explicit conversion code.
When converting a string to a boolean in TypeScript, implicit conversion can occur when using the value of a string in a boolean context. This means that if you use a string value in a conditional statement, such as an if statement, TypeScript will automatically convert the string to a boolean value.
Here's an example:
const str = "true";if (str) { console.log("The string is truthy");} else { console.log("The string is falsy");}
In the example above, we use the string value "true" in an if statement. TypeScript implicitly converts the string to a boolean value, and the condition evaluates to true because the string is not empty. As a result, the message "The string is truthy" is logged to the console.
It's important to note that implicit conversion from string to boolean is based on the truthiness or falsiness of the string value. This means that an empty string, or a string that consists only of whitespace characters, will be converted to false. Any non-empty string, regardless of its contents, will be converted to true.
Exploring Explicit Conversion from String to Boolean in TypeScript
In addition to implicit conversion, TypeScript also allows for explicit conversion from one type to another using type casting or conversion functions.
When converting a string to a boolean explicitly in TypeScript, you can use the Boolean constructor function, as mentioned earlier. The Boolean constructor takes a value and returns a boolean representation of that value.
Here's an example:
const str = "true";const boolValue = Boolean(str);console.log(boolValue); // Output: true
In the example above, we use the Boolean constructor to explicitly convert the string "true" to a boolean value. The Boolean constructor takes the string as input and returns true because the string is not empty.
Another way to perform explicit conversion from a string to a boolean is by using the strict equality (===) operator, as mentioned earlier. By comparing the string to a predefined string value that represents true, you can explicitly convert the string to a boolean value.
Here's an example:
const str = "true";const boolValue = str === "true";console.log(boolValue); // Output: true
In the example above, we explicitly convert the string "true" to a boolean value by comparing it to the predefined string value "true". Since the two strings match, the result of the comparison is true.
Understanding the Difference between Implicit and Explicit Conversion
When converting a string to a boolean in TypeScript, it's important to understand the difference between implicit and explicit conversion.
Implicit conversion occurs automatically when TypeScript converts a value from one type to another without the need for explicit conversion code. This can happen when using the value of a string in a boolean context, such as an if statement. TypeScript implicitly converts the string to a boolean value based on its truthiness or falsiness.
On the other hand, explicit conversion requires explicit code to convert a value from one type to another. This can be done using type casting or conversion functions. When explicitly converting a string to a boolean in TypeScript, you can use the Boolean constructor or the strict equality operator to perform the conversion.
The main difference between implicit and explicit conversion is that implicit conversion happens automatically and does not require additional code, while explicit conversion requires explicit code to perform the conversion.
Recommended Approach to Convert a String to Boolean in TypeScript
When converting a string to a boolean in TypeScript, the recommended approach depends on the specific requirements and preferences of your application. However, there are a few considerations that can help guide your decision.
If you have a limited set of predefined string values that represent boolean values, such as "true" and "false", it's recommended to use the strict equality (===) operator to compare the string to these values. This approach is simple, straightforward, and easy to understand.
Here's an example:
const str = "true";const boolValue = str === "true";console.log(boolValue); // Output: true
On the other hand, if you need to handle a broader range of string values or customize the conversion process, it's recommended to use a mapping object or regular expressions. This allows for more flexibility and extensibility in handling different string values.
Here's an example using a mapping object:
const mapping = { "true": true, "yes": true, "on": true, "false": false, "no": false, "off": false,};function convertStringToBoolean(str: string): boolean { return mapping[str.toLowerCase()] || false;}const str = "yes";const boolValue = convertStringToBoolean(str);console.log(boolValue); // Output: true
Ultimately, the recommended approach is to choose the method that best suits your specific use case and provides the desired level of customization and simplicity.
Related Article: Tutorial on Circuit Breaker Pattern in TypeScript
Limitations and Edge Cases when Converting a String to Boolean in TypeScript
When converting a string to a boolean in TypeScript, there are certain limitations and edge cases to be aware of.
Firstly, the conversion process is based on the predefined string values that represent true and false. If the input string does not match any of these values, the conversion may not produce the desired result. It's important to handle unrecognized values appropriately, either by returning a default value or throwing an error.
Secondly, the conversion is case-sensitive by default. This means that "True" and "true" are considered different strings and may result in different boolean values. If you need case-insensitive conversion, you can use methods like toLowerCase() or regular expressions with the appropriate flags.
Another edge case to consider is when dealing with empty strings or strings that consist only of whitespace characters. By default, these strings are considered falsy and will be converted to false. If you need to handle these cases differently, you can implement custom logic to suit your specific requirements.
Lastly, the conversion process can be influenced by the specific environment or context in which your TypeScript code is running. Different platforms or frameworks may have their own conventions or rules for string to boolean conversion, so it's important to consider the context in which your code will be executed.
Built-in Function to Convert a String to Boolean in TypeScript
As of TypeScript 4.5, there is no built-in function specifically designed to convert a string to a boolean. However, TypeScript provides several techniques and methods, such as the Boolean constructor, strict equality operator, and string manipulation methods, that can be used to achieve this conversion.
Parsing a String as a Boolean in TypeScript
In TypeScript, there is no dedicated parsing function to convert a string to a boolean. However, you can use the Boolean constructor or other techniques mentioned earlier to parse a string as a boolean.
Here's an example using the Boolean constructor:
const str = "true";const boolValue = Boolean(str);console.log(boolValue); // Output: true
In this example, we use the Boolean constructor to parse the string "true" as a boolean. The Boolean constructor takes the string as input and returns the corresponding boolean representation.
Mapping String to Boolean Conversion in TypeScript
When converting a string to a boolean in TypeScript, you can use a mapping object to associate certain strings with boolean values. This allows for a more flexible and customizable conversion process.
Here's an example using a mapping object:
const mapping = { "true": true, "yes": true, "on": true, "false": false, "no": false, "off": false,};function convertStringToBoolean(str: string): boolean { return mapping[str.toLowerCase()] || false;}const str = "yes";const boolValue = convertStringToBoolean(str);console.log(boolValue); // Output: true
In the example above, we define a mapping object that associates the strings "true", "yes", and "on" with the boolean value true, and the strings "false", "no", and "off" with the boolean value false. We then define a function convertStringToBoolean
that takes a string as input, converts it to lowercase using the toLowerCase() method, and uses it as a key to retrieve the corresponding boolean value from the mapping object. If the input string does not match any keys in the mapping object, the expression mapping[str.toLowerCase()]
will be falsy, and the default value (false in this case) will be returned.
Related Article: Tutorial: Loading YAML Files in TypeScript