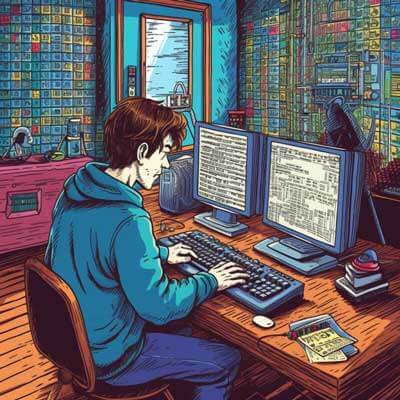
Table of Contents
Benefits of Extending Global Window Variable
Extending the global window variable in TypeScript can bring several benefits to your codebase. By adding custom properties and methods to the window object, you can enhance the functionality and capabilities of your web application. Here are some of the key benefits:
1. Access to Global Scope: The window object represents the global scope in a web browser environment. By extending the window object, you can easily access and manipulate global variables and functions from anywhere in your TypeScript code.
2. Improved Code Organization: Adding custom properties and methods to the window object allows you to organize your code in a modular and structured manner. Instead of scattering global variables and functions throughout your codebase, you can encapsulate them within the window object, making it easier to manage and maintain.
3. Global Configuration: You can use the extended window object to store global configuration settings for your application. This makes it convenient to access and modify these settings from different parts of your codebase.
4. Third-Party Library Integration: Extending the window object can be useful when integrating third-party libraries or frameworks. Some libraries may require access to global variables or functions, and by adding them to the window object, you can ensure seamless integration without polluting the global namespace.
5. Compatibility with Existing Code: If you have existing JavaScript code that relies on global variables or functions, extending the window object allows you to maintain compatibility with that code. You can add the necessary properties and methods to the window object and ensure that your TypeScript code can interact with the existing JavaScript code seamlessly.
Related Article: How to Convert a String to a Number in TypeScript
Example:
Suppose you have a web application that requires access to a global configuration object. You can extend the window object in TypeScript to add a "config" property that stores the configuration settings. Here's how you can do it:
// Extend the window object interface Window { config: { apiUrl: string; apiKey: string; }; } // Access the configuration console.log(window.config.apiUrl); // Output: https://api.example.com console.log(window.config.apiKey); // Output: ABC123
In this example, we define an interface "Window" and add a nested "config" property to it. This allows us to access the configuration settings through the "window.config" object.
Accessing the Window Object in TypeScript
Accessing the window object in TypeScript is straightforward. Since the window object is part of the global scope, you can directly reference it in your code without any imports or additional setup.
Here's an example of accessing the window object in TypeScript:
// Access the window object console.log(window);
The above code will log the window object to the console, displaying all its properties and methods.
It's important to note that the window object is only available in a web browser environment. If you're working in a different environment, such as Node.js, the window object will not be available.
Adding Properties to the Window Object in TypeScript
Adding properties to the window object in TypeScript is a straightforward process. You can extend the "Window" interface and define the new property within it.
Here's an example of adding a custom property "myProperty" to the window object:
// Extend the window object interface Window { myProperty: string; } // Assign a value to the custom property window.myProperty = "Hello, world!"; // Access the custom property console.log(window.myProperty); // Output: Hello, world!
In this example, we define the "myProperty" property within the "Window" interface. We can then assign a value to this property using the "window" object and access it later.
Adding properties to the window object can be useful for storing global variables or application-specific data that needs to be accessed from multiple parts of your codebase.
Related Article: Tutorial: Date Comparison in TypeScript
Extending Global Scope in TypeScript
Extending the global scope in TypeScript refers to adding custom properties and methods to the window object, which represents the global scope in a web browser environment.
To extend the global scope, you need to define the new properties and methods within the "Window" interface. This allows you to access and use them throughout your TypeScript code.
Here's an example of extending the global scope by adding a custom method "myMethod" to the window object:
// Extend the window object interface Window { myMethod: () => void; } // Define the custom method window.myMethod = () => { console.log("Custom method called!"); }; // Call the custom method window.myMethod(); // Output: Custom method called!
In this example, we define the "myMethod" method within the "Window" interface. We can then assign an arrow function to this method using the "window" object. Finally, we can call the custom method as any other regular method.
Extending the global scope allows you to add custom functionality to the window object, making it accessible from any part of your codebase.
Adding Methods to the Window Object in TypeScript
Adding methods to the window object in TypeScript is similar to adding properties. You can extend the "Window" interface and define the new method within it.
Here's an example of adding a custom method "myMethod" to the window object:
// Extend the window object interface Window { myMethod: () => void; } // Define the custom method window.myMethod = () => { console.log("Custom method called!"); }; // Call the custom method window.myMethod(); // Output: Custom method called!
In this example, we define the "myMethod" method within the "Window" interface. We can then assign an arrow function to this method using the "window" object. Finally, we can call the custom method as any other regular method.
Adding methods to the window object can be useful for encapsulating functionality that needs to be accessed globally. It allows you to organize your code and make it more maintainable.
Custom Properties in the Window Object in TypeScript
Adding custom properties to the window object in TypeScript allows you to store application-specific data that can be accessed globally. These properties can be used to share data between different parts of your codebase or integrate with third-party libraries.
Here's an example of adding a custom property "myProperty" to the window object:
// Extend the window object interface Window { myProperty: string; } // Assign a value to the custom property window.myProperty = "Hello, world!"; // Access the custom property console.log(window.myProperty); // Output: Hello, world!
In this example, we define the "myProperty" property within the "Window" interface. We can then assign a value to this property using the "window" object and access it later.
Custom properties in the window object can be particularly useful when you need to pass data between different pages or components of your web application.
Purpose of Extending the Window Object in TypeScript
The purpose of extending the window object in TypeScript is to enhance the functionality and capabilities of your web application. By adding custom properties and methods to the window object, you can access and manipulate global variables, store application-specific data, and integrate with third-party libraries.
Some common purposes of extending the window object include:
1. Global Configuration: You can use the extended window object to store global configuration settings for your application. This makes it convenient to access and modify these settings from different parts of your codebase.
2. Third-Party Library Integration: Extending the window object can be useful when integrating third-party libraries or frameworks. Some libraries may require access to global variables or functions, and by adding them to the window object, you can ensure seamless integration without polluting the global namespace.
3. Improved Code Organization: Adding custom properties and methods to the window object allows you to organize your code in a modular and structured manner. Instead of scattering global variables and functions throughout your codebase, you can encapsulate them within the window object, making it easier to manage and maintain.
4. Compatibility with Existing Code: If you have existing JavaScript code that relies on global variables or functions, extending the window object allows you to maintain compatibility with that code. You can add the necessary properties and methods to the window object and ensure that your TypeScript code can interact with the existing JavaScript code seamlessly.
The purpose of extending the window object is to provide a centralized location for accessing and managing global variables, functions, and application-specific data.
Related Article: How to Work with Dynamic Objects in TypeScript
Limitations of Extending the Window Object in TypeScript
While extending the window object in TypeScript can be a useful technique, it also comes with certain limitations and considerations. Here are some of the limitations to keep in mind:
1. Global Namespace: Extending the window object adds properties and methods to the global namespace. This can lead to potential naming conflicts if multiple scripts or libraries extend the window object with the same property or method names. It's important to carefully choose unique names to prevent conflicts.
2. Browser Compatibility: The window object is specific to web browser environments. If your TypeScript code needs to run in a different environment, such as Node.js, the window object will not be available. You should ensure that your code gracefully handles cases where the window object is not present.
3. Code Clutter: Adding too many custom properties and methods to the window object can clutter the global namespace and make the codebase harder to maintain. It's important to carefully consider the necessity of each extension and avoid overextending the window object.
4. Testing and Mocking: When extending the window object, it can be challenging to write unit tests or mock the extended properties and methods. You may need to use techniques such as dependency injection or mocking frameworks to properly test code that interacts with the extended window object.
5. Security Concerns: Extending the window object introduces potential security risks, especially if the extended properties or methods are accessible from untrusted sources. It's important to validate and sanitize any inputs to prevent security vulnerabilities like cross-site scripting (XSS) attacks.
Preventing Conflicts when Extending the Window Object in TypeScript
To prevent naming conflicts when extending the window object in TypeScript, it's important to choose unique names for your custom properties and methods. This helps avoid clashes with existing or future extensions to the window object by other scripts or libraries.
Here are some strategies to prevent conflicts when extending the window object:
1. Use Descriptive Prefixes: Prefix your custom properties and methods with a descriptive name that is unlikely to clash with existing or future extensions. For example, if you're adding a custom property for storing user data, you could use "myAppUserData" to reduce the chances of conflicts.
2. Namespace Objects: Instead of directly extending the window object, consider using a namespace object to encapsulate your custom properties and methods. This helps create a separate namespace and reduces the chances of clashes. For example:
// Create a namespace object namespace MyNamespace { export const myProperty: string = "Hello, world!"; export function myMethod(): void { console.log("Custom method called!"); } } // Access the custom property and method console.log(MyNamespace.myProperty); // Output: Hello, world! MyNamespace.myMethod(); // Output: Custom method called!
In this example, we define a namespace object "MyNamespace" and add the custom property "myProperty" and method "myMethod" to it. This keeps the custom extensions separate from the window object.
3. Use Unique Names: Choose unique names for your custom properties and methods to minimize the chances of conflicts. Consider using prefixes, suffixes, or unique identifiers to make the names distinctive.