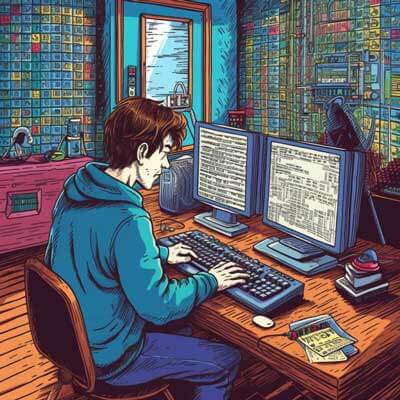
- Event Handling in TypeScript
- TypeScript Event Listeners
- Mouse Events in TypeScript
- Event Handler Functions in TypeScript
- Adding Event Listeners in TypeScript
- Handling Click Events in TypeScript
- Handling Mouseover Events in TypeScript
- Handling Mousedown Events in TypeScript
- Handling Mouseup Events in TypeScript
- Handling Mousemove Events in TypeScript
- Syntax for Adding Event Listeners in TypeScript
- External Sources
Event Handling in TypeScript
Event handling in TypeScript allows developers to create interactive web applications by responding to user actions such as clicks, mouse movements, and keyboard inputs. TypeScript provides a robust event handling mechanism that is based on the EventTarget interface and a set of event listener interfaces. By utilizing these features, developers can easily add event listeners to HTML elements and define callback functions to handle specific events.
In TypeScript, event handling is typically done using the addEventListener method, which allows developers to attach event listeners to HTML elements. The addEventListener method takes two parameters: the type of event to listen for and the callback function to be executed when the event occurs. For example, to handle a click event on a button element, you can use the following code:
const button = document.querySelector('button'); button.addEventListener('click', () => { console.log('Button clicked!'); });
In this example, the addEventListener method is used to attach a click event listener to the button element. When the button is clicked, the callback function is executed, and the message “Button clicked!” is logged to the console.
Related Article: Tutorial: Extending the Window Object in TypeScript
TypeScript Event Listeners
TypeScript provides several event listener interfaces that can be used to define the type of event to listen for and the properties and methods available on the event object. Some of the commonly used event listener interfaces in TypeScript include MouseEvent, KeyboardEvent, and Event.
The MouseEvent interface represents mouse-related events such as clicks, mouse movements, and scroll events. It provides properties such as clientX and clientY to get the coordinates of the mouse pointer, as well as methods like preventDefault to prevent the default behavior of the event. Here’s an example of using the MouseEvent interface to handle a click event:
const button = document.querySelector('button'); button.addEventListener('click', (event: MouseEvent) => { console.log(`Clicked at (${event.clientX}, ${event.clientY})`); });
In this example, the event parameter of the callback function is of type MouseEvent, allowing access to properties like clientX and clientY to get the coordinates of the mouse click.
Mouse Events in TypeScript
Mouse events are a type of events triggered by user interactions with the mouse, such as clicks, movements, and scroll events. TypeScript provides a set of event listener interfaces specifically designed for handling mouse events, including MouseEvent, MouseWheelEvent, and DragEvent.
The MouseEvent interface, as mentioned earlier, represents mouse-related events and provides properties and methods to interact with the event. The MouseWheelEvent interface represents mouse scroll events and provides properties like deltaX and deltaY to get the scroll amount. The DragEvent interface represents drag and drop events and provides properties like dataTransfer to access the dragged data.
Let’s take a look at an example that demonstrates the usage of mouse events in TypeScript:
const element = document.querySelector('.element'); element.addEventListener('click', (event: MouseEvent) => { console.log('Clicked on the element!'); }); element.addEventListener('mousemove', (event: MouseEvent) => { console.log(`Mouse moved at (${event.clientX}, ${event.clientY})`); }); element.addEventListener('wheel', (event: MouseWheelEvent) => { console.log(`Scroll amount: (${event.deltaX}, ${event.deltaY})`); }); element.addEventListener('dragstart', (event: DragEvent) => { console.log('Drag started!'); });
In this example, event listeners are attached to the element with the class “element” to handle click, mousemove, wheel, and dragstart events. The callback functions log different messages depending on the type of event.
Event Handler Functions in TypeScript
Event handler functions in TypeScript are callback functions that are executed when a specific event occurs. These functions are defined to handle events and can be attached to HTML elements using the addEventListener method.
Event handler functions in TypeScript can take an event parameter, which is an object that contains information about the event. The type of the event parameter depends on the type of event being handled. For example, if you’re handling a click event, the event parameter would be of type MouseEvent.
Here’s an example of defining an event handler function in TypeScript:
function handleClick(event: MouseEvent) { console.log('Button clicked!'); } const button = document.querySelector('button'); button.addEventListener('click', handleClick);
In this example, the handleClick function is defined to handle a click event. The function simply logs a message to the console when the button is clicked. The handleClick function is then passed as the callback function to the addEventListener method to attach the event listener to the button element.
Related Article: Working with HTML Button Elements in TypeScript
Adding Event Listeners in TypeScript
In TypeScript, event listeners can be added to HTML elements using the addEventListener method. This method takes two parameters: the type of event to listen for and the callback function to be executed when the event occurs.
Here’s an example of adding an event listener to a button element in TypeScript:
const button = document.querySelector('button'); button.addEventListener('click', () => { console.log('Button clicked!'); });
In this example, the addEventListener method is used to attach a click event listener to the button element. When the button is clicked, the callback function is executed, and the message “Button clicked!” is logged to the console.
Multiple event listeners can be added to the same HTML element, allowing different actions to be performed for different events. For example:
const button = document.querySelector('button'); button.addEventListener('click', () => { console.log('Button clicked!'); }); button.addEventListener('mouseover', () => { console.log('Mouse over the button!'); });
In this example, two event listeners are added to the button element: one for the click event and another for the mouseover event. When the button is clicked, the message “Button clicked!” is logged, and when the mouse is over the button, the message “Mouse over the button!” is logged.
Handling Click Events in TypeScript
Click events are triggered when the user clicks on an HTML element. In TypeScript, click events can be handled by adding a click event listener to the desired element using the addEventListener method.
Here’s an example of handling a click event in TypeScript:
const button = document.querySelector('button'); button.addEventListener('click', () => { console.log('Button clicked!'); });
In this example, a click event listener is added to the button element. When the button is clicked, the callback function is executed, and the message “Button clicked!” is logged to the console.
Multiple click event listeners can be added to the same HTML element, allowing different actions to be performed for different click events. For example:
const button = document.querySelector('button'); button.addEventListener('click', () => { console.log('Button clicked!'); }); button.addEventListener('click', () => { alert('Button clicked!'); });
In this example, two click event listeners are added to the button element. When the button is clicked, both callback functions are executed, logging the message “Button clicked!” to the console and displaying an alert dialog.
Handling Mouseover Events in TypeScript
Mouseover events are triggered when the user moves the mouse pointer over an HTML element. In TypeScript, mouseover events can be handled by adding a mouseover event listener to the desired element using the addEventListener method.
Here’s an example of handling a mouseover event in TypeScript:
const button = document.querySelector('button'); button.addEventListener('mouseover', () => { console.log('Mouse over the button!'); });
In this example, a mouseover event listener is added to the button element. When the mouse pointer is moved over the button, the callback function is executed, and the message “Mouse over the button!” is logged to the console.
Multiple mouseover event listeners can be added to the same HTML element, allowing different actions to be performed for different mouseover events. For example:
const button = document.querySelector('button'); button.addEventListener('mouseover', () => { console.log('Mouse over the button!'); }); button.addEventListener('mouseover', () => { alert('Mouse over the button!'); });
In this example, two mouseover event listeners are added to the button element. When the mouse pointer is moved over the button, both callback functions are executed, logging the message “Mouse over the button!” to the console and displaying an alert dialog.
Handling Mousedown Events in TypeScript
Mousedown events are triggered when the user presses a mouse button while the pointer is over an HTML element. In TypeScript, mousedown events can be handled by adding a mousedown event listener to the desired element using the addEventListener method.
Here’s an example of handling a mousedown event in TypeScript:
const button = document.querySelector('button'); button.addEventListener('mousedown', () => { console.log('Mouse button down on the button!'); });
In this example, a mousedown event listener is added to the button element. When a mouse button is pressed while the pointer is over the button, the callback function is executed, and the message “Mouse button down on the button!” is logged to the console.
Multiple mousedown event listeners can be added to the same HTML element, allowing different actions to be performed for different mousedown events. For example:
const button = document.querySelector('button'); button.addEventListener('mousedown', () => { console.log('Mouse button down on the button!'); }); button.addEventListener('mousedown', () => { alert('Mouse button down on the button!'); });
In this example, two mousedown event listeners are added to the button element. When a mouse button is pressed while the pointer is over the button, both callback functions are executed, logging the message “Mouse button down on the button!” to the console and displaying an alert dialog.
Handling Mouseup Events in TypeScript
Mouseup events are triggered when the user releases a mouse button that was previously pressed while the pointer was over an HTML element. In TypeScript, mouseup events can be handled by adding a mouseup event listener to the desired element using the addEventListener method.
Here’s an example of handling a mouseup event in TypeScript:
const button = document.querySelector('button'); button.addEventListener('mouseup', () => { console.log('Mouse button released on the button!'); });
In this example, a mouseup event listener is added to the button element. When a mouse button is released after being pressed while the pointer is over the button, the callback function is executed, and the message “Mouse button released on the button!” is logged to the console.
Multiple mouseup event listeners can be added to the same HTML element, allowing different actions to be performed for different mouseup events. For example:
const button = document.querySelector('button'); button.addEventListener('mouseup', () => { console.log('Mouse button released on the button!'); }); button.addEventListener('mouseup', () => { alert('Mouse button released on the button!'); });
In this example, two mouseup event listeners are added to the button element. When a mouse button is released after being pressed while the pointer is over the button, both callback functions are executed, logging the message “Mouse button released on the button!” to the console and displaying an alert dialog.
Handling Mousemove Events in TypeScript
Mousemove events are triggered when the user moves the mouse pointer while it is over an HTML element. In TypeScript, mousemove events can be handled by adding a mousemove event listener to the desired element using the addEventListener method.
Here’s an example of handling a mousemove event in TypeScript:
const button = document.querySelector('button'); button.addEventListener('mousemove', () => { console.log('Mouse moved over the button!'); });
In this example, a mousemove event listener is added to the button element. When the mouse pointer is moved over the button, the callback function is executed, and the message “Mouse moved over the button!” is logged to the console.
Multiple mousemove event listeners can be added to the same HTML element, allowing different actions to be performed for different mousemove events. For example:
const button = document.querySelector('button'); button.addEventListener('mousemove', () => { console.log('Mouse moved over the button!'); }); button.addEventListener('mousemove', () => { alert('Mouse moved over the button!'); });
In this example, two mousemove event listeners are added to the button element. When the mouse pointer is moved over the button, both callback functions are executed, logging the message “Mouse moved over the button!” to the console and displaying an alert dialog.
Syntax for Adding Event Listeners in TypeScript
The syntax for adding event listeners in TypeScript using the addEventListener method is as follows:
element.addEventListener(eventType, callbackFunction);
– element
is the HTML element to which the event listener is being added.
– eventType
is a string representing the type of event to listen for.
– callbackFunction
is the function to be executed when the event occurs.
Here’s an example that demonstrates the syntax for adding event listeners in TypeScript:
const button = document.querySelector('button'); button.addEventListener('click', () => { console.log('Button clicked!'); });
In this example, an event listener is added to the button element to listen for click events. When the button is clicked, the callback function is executed, and the message “Button clicked!” is logged to the console.
External Sources
– MDN Web Docs: EventTarget.addEventListener()
– MDN Web Docs: MouseEvent
– MDN Web Docs: KeyboardEvent
– MDN Web Docs: Event