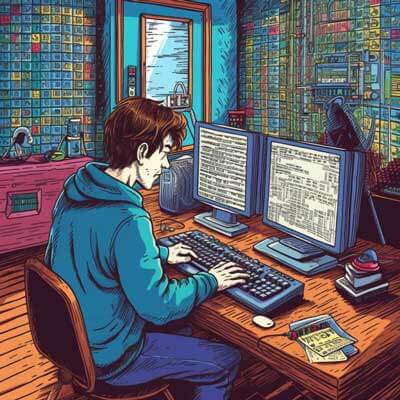
- How to Create a Dynamic Object in TypeScript?
- How to Define the Type of an Object in TypeScript?
- How Can I Access Dynamic Properties in TypeScript?
- How to Iterate Over the Properties of a Dynamic Object in TypeScript?
- Can I Add or Remove Properties from a TypeScript Object Dynamically?
- What is the Difference Between Object Type and Object Literal in TypeScript?
- How to Get the Keys of an Object in TypeScript?
- How to Dynamically Set the Value of a Property in TypeScript?
- What are the Advantages of Using Dynamic Objects in TypeScript?
- What is the Difference Between Dynamic Typing and Static Typing in TypeScript?
- External Sources
How to Create a Dynamic Object in TypeScript?
In TypeScript, you can create a dynamic object by using the any
type. The any
type allows you to assign any value to a variable without any type checking. This means that you can add and remove properties from the object without any restrictions. Here’s an example:
let dynamicObject: any = {}; dynamicObject.name = "John"; dynamicObject.age = 30;
In the above example, we create a dynamic object dynamicObject
and assign properties name
and age
with their respective values. Since dynamicObject
is of type any
, TypeScript allows us to add properties dynamically.
Related Article: How to Implement and Use Generics in Typescript
How to Define the Type of an Object in TypeScript?
While using dynamic objects with the any
type provides flexibility, it can also lead to potential type-related errors. To avoid these errors, it’s recommended to define the type of an object explicitly in TypeScript. You can define the type of an object using interface or type annotations. Here’s an example:
interface Person { name: string; age: number; } let typedObject: Person = { name: "John", age: 30, };
In the above example, we define an interface Person
with properties name
and age
. We then create an object typedObject
of type Person
and assign values to its properties. By defining the type of the object explicitly, TypeScript provides type checking and helps identify potential type errors during development.
How Can I Access Dynamic Properties in TypeScript?
To access dynamic properties in TypeScript, you can use the dot notation or square bracket notation. The dot notation is the most common way to access properties. Here’s an example:
let dynamicObject: any = { name: "John", age: 30, }; console.log(dynamicObject.name); // Output: John console.log(dynamicObject.age); // Output: 30
In the above example, we have a dynamic object dynamicObject
with properties name
and age
. We use the dot notation to access and log the values of the properties.
Alternatively, you can also use the square bracket notation to access dynamic properties. This is useful when the property name is stored in a variable. Here’s an example:
let dynamicObject: any = { name: "John", age: 30, }; let property = "name"; console.log(dynamicObject[property]); // Output: John
In the above example, we store the property name "name"
in a variable property
and then use the square bracket notation to access the dynamic property.
How to Iterate Over the Properties of a Dynamic Object in TypeScript?
To iterate over the properties of a dynamic object in TypeScript, you can use a for...in
loop. The for...in
loop iterates over all enumerable properties of an object. Here’s an example:
let dynamicObject: any = { name: "John", age: 30, }; for (let property in dynamicObject) { console.log(property + ": " + dynamicObject[property]); }
In the above example, we use a for...in
loop to iterate over the properties of the dynamic object dynamicObject
. Inside the loop, we access the value of each property using the square bracket notation.
This will output:
name: John age: 30
Related Article: Tutorial: Navigating the TypeScript Exit Process
Can I Add or Remove Properties from a TypeScript Object Dynamically?
In TypeScript, once you define the type of an object, you cannot add or remove properties from it dynamically. This is because TypeScript enforces static type checking and ensures that the object adheres to its defined type. If you try to add or remove properties from a statically typed object, TypeScript will throw a compile-time error.
However, if you have a dynamic object with the any
type, you can freely add or remove properties from it. Here’s an example:
let dynamicObject: any = { name: "John", age: 30, }; dynamicObject.address = "123 Main St"; delete dynamicObject.age;
In the above example, we have a dynamic object dynamicObject
with properties name
and age
. We add a new property address
and then remove the age
property using the delete
keyword. Since dynamicObject
is of type any
, TypeScript allows us to add and remove properties dynamically.
What is the Difference Between Object Type and Object Literal in TypeScript?
In TypeScript, the object type and object literal are two different concepts.
– Object Type: The object type in TypeScript refers to the type declaration of an object. It specifies the structure and properties of an object. Object types are defined using interfaces or type aliases. Here’s an example:
interface Person { name: string; age: number; } let person: Person = { name: "John", age: 30, };
In the above example, we define an object type Person
using the interface syntax. The Person
type specifies that an object of this type should have properties name
(string) and age
(number). We then create an object person
of type Person
that adheres to the defined structure.
– Object Literal: The object literal in TypeScript refers to the syntax for creating objects with specific property values directly. It is a shorthand notation to create objects without explicitly defining their types. Here’s an example:
let person = { name: "John", age: 30, };
In the above example, we create an object person
using the object literal syntax. The object literal directly specifies the property values (name: "John"
and age: 30
) without explicitly defining the type. TypeScript infers the type of the object based on the provided property values.
The key difference between object type and object literal is that the object type defines the structure and properties of an object, while the object literal is a shorthand notation to create objects with specific property values.
How to Get the Keys of an Object in TypeScript?
In TypeScript, you can get the keys of an object using the Object.keys()
method. The Object.keys()
method returns an array of strings that represent the enumerable properties of an object. Here’s an example:
let dynamicObject: any = { name: "John", age: 30, }; let keys = Object.keys(dynamicObject); console.log(keys); // Output: ["name", "age"]
In the above example, we use the Object.keys()
method to get the keys of the dynamic object dynamicObject
. The keys are returned as an array, which we then log to the console.
Related Article: Tutorial on TypeScript Dynamic Object Manipulation
How to Dynamically Set the Value of a Property in TypeScript?
To dynamically set the value of a property in TypeScript, you can use the dot notation or square bracket notation. The dot notation is the most common way to set the value of a property. Here’s an example:
let dynamicObject: any = {}; dynamicObject.name = "John"; dynamicObject.age = 30; console.log(dynamicObject); // Output: { name: "John", age: 30 }
In the above example, we create a dynamic object dynamicObject
and assign values to its properties name
and age
using the dot notation.
Alternatively, you can also use the square bracket notation to dynamically set the value of a property. This is useful when the property name is stored in a variable. Here’s an example:
let dynamicObject: any = {}; let property = "name"; dynamicObject[property] = "John"; console.log(dynamicObject); // Output: { name: "John" }
In the above example, we store the property name "name"
in a variable property
and then use the square bracket notation to dynamically set the value of the property.
What are the Advantages of Using Dynamic Objects in TypeScript?
Using dynamic objects in TypeScript provides flexibility and allows for dynamic property addition and removal. This can be advantageous in certain scenarios, such as:
1. Working with external APIs: When interacting with external APIs, the response objects may have dynamic properties that are not known during development. Using dynamic objects allows you to handle such scenarios without strict type checking.
2. Prototyping and experimentation: Dynamic objects enable rapid prototyping and experimentation by allowing you to add and remove properties dynamically. This flexibility can be useful when exploring different data structures or testing ideas.
3. Working with legacy code: When working with legacy codebases that lack type definitions, dynamic objects can be used to handle dynamic data structures without strict type checking.
However, it’s important to note that excessive use of dynamic objects with the any
type can lead to potential type-related errors and decreased maintainability. It’s recommended to use dynamic objects judiciously and define the type of objects whenever possible.
What is the Difference Between Dynamic Typing and Static Typing in TypeScript?
In TypeScript, dynamic typing and static typing are two different approaches to type checking.
– Dynamic Typing: Dynamic typing refers to the practice of allowing variables to hold values of any type during runtime. In dynamically typed languages like JavaScript, variables can be reassigned with values of different types without any restrictions. This flexibility can lead to potential runtime errors if the wrong type of value is assigned to a variable.
– Static Typing: Static typing, on the other hand, enforces type checking at compile time. In statically typed languages like TypeScript, variables have fixed types that are checked during compilation. This helps identify potential type errors before the code is executed. Static typing provides better code readability, maintainability, and early detection of bugs.
TypeScript combines the benefits of both dynamic typing and static typing. It introduces static type checking while maintaining compatibility with JavaScript’s dynamic nature. This allows developers to catch type-related errors during development and write more robust and maintainable code.
Related Article: Tutorial: Checking Enum Value Existence in TypeScript
External Sources
– TypeScript Handbook – Objects
– MDN Web Docs – Working with objects