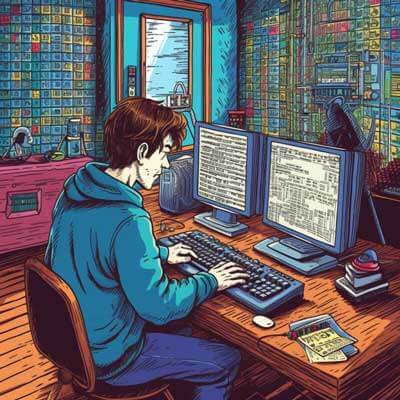
Table of Contents
Overview of YAML Files
YAML (YAML Ain't Markup Language) is a human-readable data serialization format. It is often used for configuration files and data exchange between languages that support different data structures. YAML uses indentation and special characters to represent data in a structured manner.
YAML files are easy to read and write, making them a popular choice for configuration files in many projects. They can contain key-value pairs, lists, nested data structures, and even complex objects. Here's an example of a YAML file:
name: John Doe age: 30 email: john.doe@example.com hobbies: - reading - hiking - coding address: city: New York state: NY
In this example, the YAML file represents a person's information, including their name, age, email, hobbies, and address. The data is structured using indentation to indicate nesting levels.
Related Article: Tutorial: Converting String to Bool in TypeScript
Benefits of Using YAML in TypeScript
Using YAML in TypeScript has several benefits:
1. Human-readable and writable: YAML's syntax is designed to be easy to read and write for humans. This makes it more approachable for developers, especially those who are not familiar with complex data formats like JSON.
2. Flexible and expressive: YAML supports a wide range of data types and structures, including lists, dictionaries, and nested objects. This flexibility allows developers to represent complex data structures in a concise and readable manner.
3. Easy integration with TypeScript: TypeScript has native support for parsing and manipulating JSON objects. YAML can be easily converted to JSON, allowing TypeScript developers to work with YAML data using familiar JSON APIs and libraries.
4. Widely supported: YAML is a widely supported format across different programming languages. There are many libraries available for parsing and generating YAML in TypeScript and other languages.
Introduction to TypeScript YAML Libraries
There are several TypeScript libraries available for parsing and generating YAML. These libraries provide convenient APIs for working with YAML data in TypeScript projects. Here are some popular ones:
1. js-yaml: js-yaml is a JavaScript library that provides a YAML parser and serializer. It supports both YAML 1.1 and YAML 1.2 specifications and has a simple and intuitive API. js-yaml can be used in TypeScript projects by installing the js-yaml
package and using it with TypeScript's type annotations.
2. yaml: yaml is another JavaScript library for working with YAML in TypeScript. It provides a more lightweight and minimalistic API compared to js-yaml. yaml focuses on providing a simple and efficient way to parse and generate YAML data.
3. yamljs: yamljs is a JavaScript library that provides a YAML parser and serializer. It has a simple and straightforward API and can be easily integrated into TypeScript projects. yamljs supports YAML 1.2 specifications and provides options for customizing the parsing and serialization process.
These libraries offer similar functionalities for working with YAML data in TypeScript. The choice of library depends on your specific requirements and preferences.
Exploring Different YAML Loaders in TypeScript
In TypeScript, there are different approaches to loading YAML files. Let's explore some of these approaches and see how they can be used in TypeScript projects.
1. Using fs and js-yaml: One way to load a YAML file in TypeScript is to use the fs module from Node.js along with the js-yaml library. Here's an example:
import * as fs from 'fs'; import * as yaml from 'js-yaml'; const fileContents = fs.readFileSync('data.yaml', 'utf8'); const data = yaml.load(fileContents); console.log(data);
In this example, we use the readFileSync
function from the fs module to read the contents of the YAML file. Then, we use the load
function from the js-yaml library to parse the YAML data into a JavaScript object.
2. Using fetch and js-yaml: If you are working in a browser environment or have access to the fetch
function, you can use it to load the YAML file and then parse it using js-yaml. Here's an example:
import * as yaml from 'js-yaml'; fetch('data.yaml') .then(response => response.text()) .then(fileContents => { const data = yaml.load(fileContents); console.log(data); });
In this example, we use the fetch
function to fetch the YAML file. We then use the text
method of the response object to get the file contents as a string. Finally, we parse the YAML data using the load
function from js-yaml.
These are just two examples of how you can load YAML files in TypeScript. Depending on your project requirements, you may need to use different approaches or libraries.
Related Article: Tutorial: Date Subtraction in TypeScript
Step-by-Step Guide to Loading YAML Files in TypeScript
Loading YAML files in TypeScript involves a few steps:
1. Install the required libraries: First, you need to install the required libraries for working with YAML in TypeScript. You can use npm or yarn to install them. For example:
npm install js-yaml
2. Load the YAML file: Once you have the required libraries installed, you can load the YAML file. This can be done using different approaches, such as using the fs module in Node.js or the fetch function in a browser environment.
3. Parse the YAML data: After loading the YAML file, you need to parse the YAML data into a JavaScript object. This can be done using the appropriate functions or methods provided by the YAML library you are using.
4. Use the parsed data: Once the YAML data is parsed, you can use it in your TypeScript code as a JavaScript object. You can access its properties, iterate over arrays, and perform any other operations you need.
Here's an example that demonstrates these steps using the js-yaml library and the fs module in Node.js:
import * as fs from 'fs'; import * as yaml from 'js-yaml'; const fileContents = fs.readFileSync('data.yaml', 'utf8'); const data = yaml.load(fileContents); console.log(data);
In this example, we use the readFileSync
function from the fs module to read the contents of the YAML file. Then, we use the load
function from the js-yaml library to parse the YAML data into a JavaScript object. Finally, we log the parsed data to the console.
Converting YAML to TypeScript Object
After loading and parsing a YAML file in TypeScript, you can convert the resulting JavaScript object into a TypeScript object by defining a corresponding interface. This allows you to leverage TypeScript's static type checking and code completion features when working with the parsed YAML data.
Let's say we have the following YAML file representing a person's information:
name: John Doe age: 30 email: john.doe@example.com
To convert this YAML data into a TypeScript object, we can define an interface that matches the structure of the YAML data:
interface Person { name: string; age: number; email: string; }
Then, we can cast the parsed YAML data to this interface:
const person: Person = data as Person;
Now, we can use the person
object with TypeScript's static type checking and code completion:
console.log(person.name); // John Doe console.log(person.age); // 30 console.log(person.email); // john.doe@example.com
Best Practices for Handling YAML Parsing in TypeScript
When handling YAML parsing in TypeScript, there are some best practices you can follow to ensure clean and maintainable code. Here are a few recommendations:
1. Use TypeScript interfaces: Define TypeScript interfaces that match the structure of your YAML data. This allows you to leverage TypeScript's static type checking and code completion features.
2. Separate parsing logic: Separate the YAML parsing logic from the rest of your code. This makes it easier to test and maintain the parsing functionality independently.
3. Error handling: Handle parsing errors gracefully. When parsing YAML data, there is a chance of encountering invalid or malformed data. Make sure to handle these cases and provide meaningful error messages to the users.
4. Validation: Consider adding validation logic to ensure the parsed YAML data meets your expected structure and constraints. This can help catch potential issues early and provide a better user experience.
5. Documentation: Document the expected structure and format of the YAML data. This can be helpful for other developers working with the code and can serve as a reference for future maintenance.
Here's an example that demonstrates some of these best practices:
import * as fs from 'fs'; import * as yaml from 'js-yaml'; interface Person { name: string; age: number; email: string; } function parseYamlFile(filePath: string): Person { const fileContents = fs.readFileSync(filePath, 'utf8'); const data = yaml.load(fileContents) as Person; if (!data.name || !data.age || !data.email) { throw new Error('Invalid YAML data'); } return data; } try { const person = parseYamlFile('data.yaml'); console.log(person.name, person.age, person.email); } catch (error) { console.error(error.message); }
This example separates the YAML parsing logic into a parseYamlFile
function. It defines a Person
interface and performs basic validation to ensure the parsed data has the required properties. If any validation fails, it throws an error with a meaningful message.
Common Issues and Troubleshooting Tips
When working with YAML parsing in TypeScript, you may encounter some common issues or errors. Here are a few troubleshooting tips to help you resolve them:
1. Invalid YAML data: If the YAML data is invalid or malformed, the parsing process may fail. Check the YAML file for any syntax errors, missing or incorrect indentation, or other issues that may cause parsing errors.
2. Missing libraries: Make sure you have the required YAML parsing library installed and imported correctly. Double-check the package name and the import statements to ensure everything is set up correctly.
3. Type mismatches: If you are converting the parsed YAML data to TypeScript objects using interfaces, make sure the interface matches the structure of the YAML data. Check for any mismatches in property names or types that may cause type errors.
4. File not found: If you are loading the YAML file using file system functions like readFileSync
, make sure the file exists in the specified path. Double-check the file path and ensure it is relative to the correct directory.
5. Encoding issues: If you encounter encoding issues when reading the YAML file, check the file encoding and make sure it matches the encoding specified when reading the file.
6. Version compatibility: Ensure that the version of the YAML parsing library you are using is compatible with the version of TypeScript and other dependencies in your project. Check the library's documentation for version compatibility information.
These troubleshooting tips should help you diagnose and resolve common issues when working with YAML parsing in TypeScript.
Related Article: Tutorial: Checking if a String is a Number in TypeScript
Code Snippets for YAML Parsing in TypeScript
Here are some code snippets that demonstrate YAML parsing in TypeScript using different libraries:
Using js-yaml:
import * as fs from 'fs'; import * as yaml from 'js-yaml'; const fileContents = fs.readFileSync('data.yaml', 'utf8'); const data = yaml.load(fileContents); console.log(data);
Using yamljs:
import * as fs from 'fs'; import * as yaml from 'yamljs'; const fileContents = fs.readFileSync('data.yaml', 'utf8'); const data = yaml.parse(fileContents); console.log(data);
These snippets show how to load a YAML file using the fs module and parse it using the respective libraries. The parsed YAML data is then logged to the console.
Using a YAML Parser in TypeScript Projects
To use a YAML parser in a TypeScript project, you need to install the required library and import it into your code. Here's an example of how to use the js-yaml library in a TypeScript project:
1. Install the js-yaml library:
npm install js-yaml
2. Import and use the library:
import * as fs from 'fs'; import * as yaml from 'js-yaml'; const fileContents = fs.readFileSync('data.yaml', 'utf8'); const data = yaml.load(fileContents); console.log(data);
In this example, we import the fs
module from Node.js to read the contents of the YAML file. Then, we import the yaml
module from the js-yaml library to parse the YAML data. Finally, we log the parsed data to the console.
Make sure to check the documentation of the specific YAML library you are using for more details on how to use it in a TypeScript project.
Real-World Examples of YAML Parsing in TypeScript
YAML parsing is commonly used in TypeScript projects for various purposes. Here are a few real-world examples where YAML parsing can be useful:
1. Configuration files: Many projects use YAML files for configuration purposes. For example, a web application may have a YAML file that specifies database connection details, API keys, and other configuration options. Parsing these YAML files allows the application to easily read and use the configuration data.
2. Data exchange: YAML can be used as a data exchange format between different systems or components. For example, a microservices architecture may use YAML to represent data transferred between services. Parsing the YAML data allows each service to extract the relevant information and work with it.
3. Test fixtures: YAML files can be used to define test fixtures or sample data for automated tests. Parsing these files allows the tests to easily load the required data and use it in test scenarios.
These are just a few examples of how YAML parsing can be used in real-world TypeScript projects. Depending on your specific use case, there may be other scenarios where YAML parsing is beneficial.
Available TypeScript Libraries for YAML Parsing
There are several TypeScript libraries available for parsing and generating YAML. Here are some popular ones:
1. js-yaml: js-yaml is a JavaScript library that provides a YAML parser and serializer. It supports both YAML 1.1 and YAML 1.2 specifications and has a simple and intuitive API.
2. yaml: yaml is another JavaScript library for working with YAML in TypeScript. It provides a lightweight and minimalistic API for parsing and generating YAML data.
3. yamljs: yamljs is a JavaScript library that provides a YAML parser and serializer. It has a simple and straightforward API and supports YAML 1.2 specifications.
These libraries offer similar functionalities for working with YAML data in TypeScript. The choice of library depends on your specific requirements and preferences. Make sure to check the documentation and community support for each library before making a decision.
Related Article: Building a Rules Engine with TypeScript
Comparing Different YAML to TypeScript Conversion Approaches
When converting YAML data to TypeScript objects, there are multiple approaches you can take. Let's compare two common approaches: using interfaces and using classes.
Using interfaces:
interface Person { name: string; age: number; email: string; } const person: Person = { name: 'John Doe', age: 30, email: 'john.doe@example.com' };
In this approach, we define an interface that matches the structure of the YAML data. We then create a TypeScript object that conforms to this interface. This approach is simple and straightforward, but it does not provide any behavior or methods associated with the object.
Using classes:
class Person { name: string; age: number; email: string; constructor(name: string, age: number, email: string) { this.name = name; this.age = age; this.email = email; } sayHello() { console.log(`Hello, my name is ${this.name}.`); } } const person = new Person('John Doe', 30, 'john.doe@example.com'); person.sayHello();
In this approach, we define a class that represents a person. The class has properties for name, age, and email, as well as a method for saying hello. We then create an instance of the class and call its methods. This approach provides more flexibility and allows for encapsulating behavior with the data.
The choice between using interfaces and classes depends on your specific requirements. If you only need to represent the structure of the YAML data, interfaces may be sufficient. If you need to add behavior or methods to the data, classes can be a better choice.
Integrating YAML Parsing in TypeScript Build Pipelines
Integrating YAML parsing in TypeScript build pipelines can be useful for automating the process of loading and parsing YAML files during the build process. Here's how you can integrate YAML parsing in a TypeScript build pipeline using a popular build tool like webpack:
1. Install the required libraries: First, install the required libraries for working with YAML parsing in TypeScript. For example, you can use npm to install the js-yaml library:
npm install js-yaml
2. Configure the build pipeline: Configure your build pipeline to include the YAML files and the YAML parsing logic. For example, in webpack, you can use the file-loader
to load the YAML files and the js-yaml
library to parse them:
// webpack.config.js const yaml = require('js-yaml'); const fs = require('fs'); module.exports = { entry: './src/index.ts', output: { filename: 'bundle.js', path: __dirname + '/dist', }, module: { rules: [ { test: /\.yaml$/, use: [ { loader: 'file-loader', options: { name: '[name].[ext]', outputPath: 'data', }, }, ], }, ], }, resolve: { extensions: ['.ts', '.js'], }, // ... };
In this example, we configure webpack to use the file-loader
to load the YAML files and copy them to the dist/data
directory. We can then use the js-yaml
library to parse these files in our TypeScript code.
3. Load and parse the YAML files: In your TypeScript code, you can import the YAML files and parse them using the js-yaml
library:
import * as yaml from 'js-yaml'; import data from './data/data.yaml'; const parsedData = yaml.load(data); console.log(parsedData);
In this example, we import the js-yaml
library and the YAML file using a relative path. We then use the load
function from the js-yaml
library to parse the YAML data.
Tips for Efficiently Using TypeScript YAML Libraries
Here are some tips to efficiently use TypeScript YAML libraries:
1. Choose the right library: Choose a library that best suits your project's requirements in terms of features, performance, and community support. Consider factors like ease of use, documentation quality, and maintenance activity when selecting a library.
2. Use async/await: If you are loading YAML files asynchronously, consider using async/await or promise-based APIs for better readability and error handling. This can help avoid callback hell and make your code more maintainable.
3. Cache parsed data: If you frequently load and parse the same YAML files, consider caching the parsed data to avoid unnecessary parsing. This can significantly improve performance, especially if the YAML files are large or the parsing process is expensive.
4. Handle errors gracefully: When working with YAML parsing, make sure to handle errors gracefully. Validate the YAML data and provide meaningful error messages to help users identify and fix issues.
5. Optimize performance: If performance is a concern, consider using libraries optimized for speed or exploring alternative approaches, such as using streaming parsers or custom parsing logic.
6. Keep dependencies up to date: Regularly update your TypeScript YAML library and other dependencies to benefit from bug fixes, performance improvements, and new features. Stay connected with the library's community to stay informed about updates and best practices.
These tips can help you efficiently use TypeScript YAML libraries in your projects and ensure a smooth development experience.
Advanced Techniques for Customizing YAML Parsing in TypeScript
While most YAML parsing libraries provide convenient APIs for parsing YAML data, there may be cases where you need to customize the parsing process. Here are some advanced techniques for customizing YAML parsing in TypeScript:
1. Custom schema: Some YAML parsing libraries allow you to define custom schemas to handle specific requirements. For example, you can define custom tags or customize the parsing of specific data types.
2. Custom loaders: If you have complex data structures or need to transform the parsed data, you can write custom loaders that preprocess or postprocess the YAML data. This can be useful for data validation, transformation, or normalization.
3. Custom resolvers: YAML resolvers allow you to customize how the YAML parser resolves references or includes external files. This can be useful when working with YAML files that reference other files or have complex interdependencies.
4. Event-based parsing: Some YAML parsing libraries provide event-based APIs that allow you to process the YAML data in a streaming fashion. This can be useful for handling large YAML files or processing data incrementally.
5. Extending the library: If the YAML parsing library you are using supports extension or customization mechanisms, you can extend or modify its behavior to fit your specific needs. This can involve subclassing or augmenting the library's classes or functions.
These advanced techniques require a deep understanding of the YAML parsing library you are using and may involve more complex code. Make sure to consult the library's documentation and community resources for guidance on customizing the parsing process.
Related Article: How to Merge Objects in TypeScript
Exploring TypeScript YAML Parser Performance
When working with YAML parsing in TypeScript, performance can be a concern, especially when dealing with large or complex YAML files. Here are some factors to consider when exploring TypeScript YAML parser performance:
1. Library performance: Different YAML parsing libraries may have different performance characteristics. Consider benchmarking multiple libraries to find one that performs well for your specific use case.
2. Parsing options: Some YAML parsing libraries provide options or flags that can affect performance. For example, you may be able to disable certain features or validation checks to improve parsing speed.
3. File size: The size of the YAML file can impact parsing performance. Larger files may take more time to load and parse. If possible, consider splitting large YAML files into smaller, more manageable files.
4. Caching: As mentioned earlier, caching parsed YAML data can significantly improve performance, especially if you frequently load and parse the same files. Consider implementing a caching mechanism to avoid unnecessary parsing.
5. Streaming parsing: If you are dealing with very large YAML files, consider using streaming parsing techniques. Streaming parsers process the YAML data incrementally, which can reduce memory usage and improve performance.
6. Profiling and optimization: If performance is a critical requirement, use profiling tools to identify performance bottlenecks and optimize your code accordingly. Measure the impact of different optimizations to ensure they provide the desired performance improvements.
Security Considerations When Parsing YAML in TypeScript
When parsing YAML in TypeScript, it's important to consider security aspects to prevent vulnerabilities. Here are some security considerations when parsing YAML in TypeScript:
1. Input validation: Validate the input YAML data to ensure it conforms to expected structures and formats. This can help prevent injection attacks or unexpected behavior due to malicious or malformed input.
2. Escape characters: Be cautious when parsing YAML data that includes user-generated content. Ensure that escape characters and special characters are properly handled to prevent code injection or other security vulnerabilities.
3. File access: When loading YAML files, be mindful of the file paths and access permissions. Avoid loading YAML files from untrusted sources or allowing arbitrary file access based on user input.
4. Keep dependencies up to date: Regularly update your YAML parsing library and other dependencies to benefit from security fixes. Stay connected with the library's community to stay informed about security updates and best practices.
5. Secure deployment: Ensure that the deployment environment for your TypeScript project follows security best practices. This includes keeping dependencies up to date, enabling security features provided by the hosting platform, and following secure coding practices.
6. Code reviews and audits: Perform regular code reviews and security audits of your TypeScript code that handles YAML parsing. This can help identify and fix security vulnerabilities or potential weaknesses in your implementation.
Frequently Asked Questions about YAML Parsing in TypeScript
Here are some frequently asked questions about YAML parsing in TypeScript:
1. Q: Can I use YAML in TypeScript without parsing it?
A: Yes, TypeScript has native support for working with JSON objects. If your YAML data can be converted to JSON, you can directly use it without parsing. However, if you need to work with YAML-specific features, parsing is necessary.
2. Q: How can I handle YAML parsing errors in TypeScript?
A: YAML parsing libraries in TypeScript usually throw exceptions or return error objects when encountering parsing errors. You can handle these errors using try-catch blocks or promise error handling mechanisms.
3. Q: Is it possible to parse YAML asynchronously in TypeScript?
A: Yes, many YAML parsing libraries in TypeScript support asynchronous parsing. You can use async/await or promise-based APIs to parse YAML files asynchronously.
4. Q: Can I parse YAML files in a browser environment using TypeScript?
A: Yes, you can parse YAML files in a browser environment using TypeScript. However, you may need to use a different approach, such as using the fetch API or XMLHttpRequest to load the YAML file.
5. Q: Are there any TypeScript-specific YAML parsing libraries?
A: While there are no TypeScript-specific YAML parsing libraries, many JavaScript libraries for YAML parsing can be used in TypeScript projects without any issues.
These are just a few frequently asked questions about YAML parsing in TypeScript. If you have more specific questions, consult the documentation of the YAML parsing library you are using or seek help from the library's community resources.
External Sources
For more information on YAML parsing in TypeScript, you can refer to the following external sources: