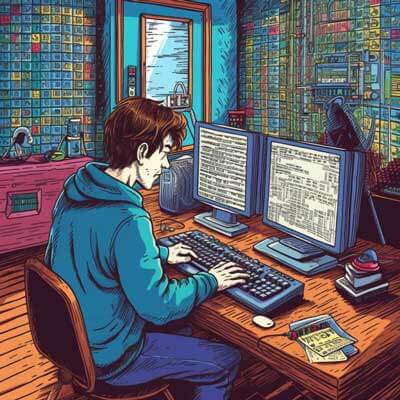
- Defining an Enum in TypeScript
- Defining a String Enum in TypeScript
- Checking if a String is in an Enum
- Checking if an Enum has a Specific Value
- Converting an Enum Value to its String Representation
- Validating a String against an Enum
- Comparing a String with an Enum Value
- Understanding the Difference between a String Enum and a Regular Enum
- Iterating over the Values of an Enum
- Assigning Custom String Values to an Enum
- External Sources
Defining an Enum in TypeScript
In TypeScript, an enum is a way to define a set of named constants. It allows you to assign a name to each value, making your code more readable and easier to understand. Enum values can be either numeric or string-based.
To define an enum in TypeScript, you can use the enum
keyword followed by the name of the enum and a set of values in curly braces. Each value is assigned a name and an optional numeric or string value.
Here’s an example of how to define a numeric enum in TypeScript:
enum Color { Red, Green, Blue, }
In this example, the Color
enum is defined with three values: Red
, Green
, and Blue
. By default, the values of a numeric enum start from 0 and increment by 1 for each subsequent value. So, Red
is assigned the value 0, Green
is assigned 1, and Blue
is assigned 2.
You can also explicitly assign numeric values to enum values. Here’s an example:
enum Weekday { Monday = 1, Tuesday = 2, Wednesday = 3, Thursday = 4, Friday = 5, Saturday = 6, Sunday = 7, }
In this example, the Weekday
enum is defined with seven values, starting from 1 and incrementing by 1 for each subsequent value. This allows you to have more control over the values assigned to the enum.
Related Article: How to Implement and Use Generics in Typescript
Defining a String Enum in TypeScript
In addition to numeric enums, TypeScript also supports string enums. A string enum is similar to a numeric enum, but the values are assigned string literals instead of numeric values.
To define a string enum in TypeScript, you can use the enum
keyword followed by the name of the enum and a set of values in curly braces. Each value is assigned a name and a string literal.
Here’s an example of how to define a string enum in TypeScript:
enum Direction { Up = "UP", Down = "DOWN", Left = "LEFT", Right = "RIGHT", }
In this example, the Direction
enum is defined with four values: Up
, Down
, Left
, and Right
. Each value is assigned a string literal, making it easier to read and understand the meaning of each enum value.
You can also use computed values to assign string literals to enum values. Here’s an example:
enum LogLevel { Error = "ERROR", Warning = "WARNING", Info = "INFO", Debug = "DEBUG", }
In this example, the LogLevel
enum is defined with four values. Each value is assigned a string literal that is computed based on its name. This allows you to have more flexibility in assigning string values to enum values.
Checking if a String is in an Enum
In TypeScript, you can check if a string is in an enum by using the in
operator. The in
operator checks if a specified property is in an object or if a specified index is in an array. When used with an enum, it checks if a specified string is one of the enum values.
Here’s an example of how to check if a string is in an enum:
enum Fruit { Apple = "APPLE", Banana = "BANANA", Orange = "ORANGE", } function isFruit(value: string): boolean { return value in Fruit; } console.log(isFruit("APPLE")); // Output: true console.log(isFruit("MANGO")); // Output: false
In this example, the isFruit
function takes a value
parameter of type string and checks if it is in the Fruit
enum using the in
operator. The function returns true
if the value
is one of the enum values, and false
otherwise.
You can use this approach to check if a string is in any enum in TypeScript.
Checking if an Enum has a Specific Value
To check if an enum has a specific value, you can use the hasOwnProperty
method and pass the value as a parameter. The hasOwnProperty
method checks if an object has a specified property.
Here’s an example of how to check if an enum has a specific value:
enum Language { JavaScript = "JavaScript", Python = "Python", TypeScript = "TypeScript", Java = "Java", } function hasLanguage(value: string): boolean { return Language.hasOwnProperty(value); } console.log(hasLanguage("Python")); // Output: true console.log(hasLanguage("Ruby")); // Output: false
In this example, the hasLanguage
function takes a value
parameter of type string and checks if it is a property of the Language
enum using the hasOwnProperty
method. The function returns true
if the value
is one of the enum values, and false
otherwise.
You can use this approach to check if an enum has a specific value in TypeScript.
Related Article: Tutorial: Navigating the TypeScript Exit Process
Converting an Enum Value to its String Representation
In TypeScript, you can convert an enum value to its string representation by using the toString
method. The toString
method returns a string that represents the specified enum value.
Here’s an example of how to convert an enum value to its string representation:
enum Direction { Up = "UP", Down = "DOWN", Left = "LEFT", Right = "RIGHT", } function getDirectionString(value: Direction): string { return value.toString(); } console.log(getDirectionString(Direction.Left)); // Output: "LEFT" console.log(getDirectionString(Direction.Right)); // Output: "RIGHT"
In this example, the getDirectionString
function takes a value
parameter of type Direction
enum and uses the toString
method to convert it to its string representation. The function returns the string representation of the enum value.
You can use this approach to convert any enum value to its string representation in TypeScript.
Validating a String against an Enum
To validate a string against an enum in TypeScript, you can use the enum
keyword followed by the name of the enum and the as const
type assertion. The as <a href="https://www.squash.io/tutorial-readonly-vs-const-in-typescript/">const
type assertion tells TypeScript to infer the literal types of the enum values.
Here’s an example of how to validate a string against an enum:
enum Fruit { Apple = "APPLE", Banana = "BANANA", Orange = "ORANGE", } function isFruit(value: string): value is Fruit { return [Fruit.Apple, Fruit.Banana, Fruit.Orange].includes(value as Fruit); } console.log(isFruit("APPLE")); // Output: true console.log(isFruit("MANGO")); // Output: false
In this example, the isFruit
function takes a value
parameter of type string and uses the includes
method to check if it is included in the array of enum values. The function returns true
if the value
is one of the enum values, and false
otherwise.
You can use this approach to validate a string against any enum in TypeScript.
Comparing a String with an Enum Value
To compare a string with an enum value in TypeScript, you can use the equality operator (===
) to check if the string is equal to the enum value.
Here’s an example of how to compare a string with an enum value:
enum Language { JavaScript = "JavaScript", Python = "Python", TypeScript = "TypeScript", Java = "Java", } function isLanguage(value: string): boolean { return value === Language.TypeScript; } console.log(isLanguage("JavaScript")); // Output: false console.log(isLanguage("TypeScript")); // Output: true
In this example, the isLanguage
function takes a value
parameter of type string and checks if it is equal to the Language.<a href="https://www.squash.io/tutorial-checking-enum-value-existence-in-typescript/">TypeScript
enum value using the equality operator (===
). The function returns true
if the value
is equal to the enum value, and false
otherwise.
You can use this approach to compare a string with any enum value in TypeScript.
Related Article: Tutorial on TypeScript Dynamic Object Manipulation
Understanding the Difference between a String Enum and a Regular Enum
In TypeScript, the main difference between a string enum and a regular enum is the type of values they can have. A regular enum can have numeric values or no values assigned at all, while a string enum can only have string literal values assigned to its members.
Regular enums:
enum Color { Red, Green, Blue, } console.log(Color.Red); // Output: 0 console.log(Color.Green); // Output: 1 console.log(Color.Blue); // Output: 2
String enums:
enum Direction { Up = "UP", Down = "DOWN", Left = "LEFT", Right = "RIGHT", } console.log(Direction.Up); // Output: "UP" console.log(Direction.Down); // Output: "DOWN" console.log(Direction.Left); // Output: "LEFT" console.log(Direction.Right); // Output: "RIGHT"
Regular enums are useful when you need to represent a set of numeric values or when you don’t need to assign specific values to the enum members. String enums, on the other hand, are useful when you want to assign meaningful string literals to the enum members.
Iterating over the Values of an Enum
To iterate over the values of an enum in TypeScript, you can use the Object.values
method. The Object.values
method returns an array of the enumerable property values of an object.
Here’s an example of how to iterate over the values of an enum:
enum Direction { Up = "UP", Down = "DOWN", Left = "LEFT", Right = "RIGHT", } function iterateEnumValues(enumObject: any): string[] { return Object.values(enumObject); } console.log(iterateEnumValues(Direction)); // Output: ["UP", "DOWN", "LEFT", "RIGHT"]
In this example, the iterateEnumValues
function takes an enumObject
parameter of type any
and uses the Object.values
method to iterate over the values of the enum. The function returns an array of the enum values.
You can use this approach to iterate over the values of any enum in TypeScript.
Assigning Custom String Values to an Enum
In TypeScript, you can assign custom string values to an enum by explicitly assigning string literals to the enum members. This allows you to have more control over the values assigned to the enum.
Here’s an example of how to assign custom string values to an enum:
enum LogLevel { Error = "ERROR", Warning = "WARNING", Info = "INFO", Debug = "DEBUG", } console.log(LogLevel.Error); // Output: "ERROR" console.log(LogLevel.Warning); // Output: "WARNING" console.log(LogLevel.Info); // Output: "INFO" console.log(LogLevel.Debug); // Output: "DEBUG"
In this example, the LogLevel
enum is defined with four values, and each value is assigned a custom string literal. This allows you to have more meaningful values for the enum members.
You can use this approach to assign custom string values to any enum in TypeScript.
Related Article: Tutorial: Checking Enum Value Existence in TypeScript
External Sources
– TypeScript Official Documentation – Enums
– TypeScript Deep Dive – Enums