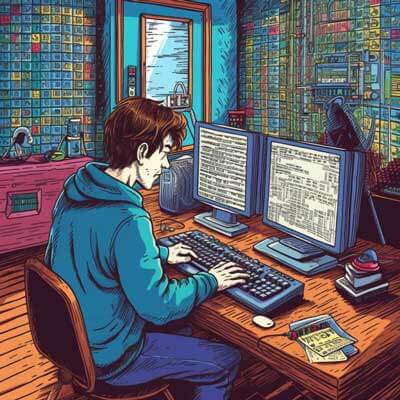
- What is a rules engine?
- How does TypeScript enforce type checking?
- What are conditional types in TypeScript?
- How does TypeScript infer types?
- Why do we need type annotations in TypeScript?
- What are type guards in TypeScript?
- How do type aliases work in TypeScript?
- What is type narrowing in TypeScript?
- How does the TypeScript type system work?
- Code Snippet: Building a basic rules engine
- Code Snippet: Implementing type checking in a rules engine
- Code Snippet: Using conditional types in a rules engine
- Code Snippet: Leveraging type inference in a rules engine
- Code Snippet: Adding type annotations to a rules engine
- Code Snippet: Applying type guards in a rules engine
- Code Snippet: Utilizing type aliases in a rules engine
- Code Snippet: Implementing type narrowing in a rules engine
- Code Snippet: Understanding the TypeScript type system in a rules engine
- External Sources
What is a rules engine?
A rules engine is a software component that allows users to define and execute business rules. Business rules are typically a set of conditional statements that determine how a system should behave under different circumstances. A rules engine provides a declarative approach to defining and managing these rules, making it easier to update and maintain them over time.
In a rules engine, rules are typically defined using a domain-specific language (DSL) or a specialized syntax. The engine then evaluates these rules against a set of data or events and performs actions based on the results. Rules engines are commonly used in decision-making processes, workflow automation, and complex event processing.
Related Article: How to Get an Object Value by Dynamic Keys in TypeScript
How does TypeScript enforce type checking?
TypeScript enforces type checking by performing static type analysis during the compilation process. It uses a combination of type inference and explicit type annotations to determine the types of variables, parameters, and function return values. TypeScript then checks if the types used in the code are compatible and raises errors if there are any type mismatches.
For example, consider the following TypeScript code snippet:
function add(a: number, b: number): number { return a + b; } const result = add(1, '2');
In this example, the add
function is explicitly annotated to accept two parameters of type number
and return a value of type number
. When we call the add
function with the arguments 1
and '2'
, TypeScript detects a type mismatch and raises an error:
Argument of type '"2"' is not assignable to parameter of type 'number'.
This type checking mechanism helps catch potential errors and improve the robustness of the code.
What are conditional types in TypeScript?
Conditional types are a feature introduced in TypeScript 2.8 that allow for the selection of types based on a condition. They enable more advanced type manipulation and can be used to create more expressive and flexible type definitions.
A conditional type is defined using the extends
keyword and a ternary operator (? :
) to specify the condition. The syntax for a conditional type is as follows:
T extends U ? X : Y
In this syntax, T
is the type being evaluated, U
is the condition type, X
is the type to be returned if the condition is true, and Y
is the type to be returned if the condition is false.
For example, consider the following TypeScript code snippet:
type TypeName = T extends string ? 'string' : T extends number ? 'number' : T extends boolean ? 'boolean' : 'other'; const name1: TypeName = 'string'; const name2: TypeName = 'number'; const name3: TypeName = 'boolean'; const name4: TypeName = 'other';
In this example, the TypeName
type takes a generic parameter T
and uses conditional types to determine the type name based on the type of T
. The type name1
is inferred as 'string'
, name2
as 'number'
, name3
as 'boolean'
, and name4
as 'other'
.
Conditional types are useful tools in TypeScript that enable more advanced type manipulation and can be used to create more flexible and reusable type definitions.
How does TypeScript infer types?
TypeScript uses type inference to automatically determine the types of variables, parameters, and function return values based on the context in which they are used. It analyzes the code and makes educated guesses about the types based on the available information.
Type inference in TypeScript works by examining the initialization value of a variable or the return value of a function and inferring the type from there. It takes into account the types of the expressions and the assignment or return type annotations, if any.
For example, consider the following TypeScript code snippet:
const num = 42; const str = 'Hello, TypeScript'; const bool = true; function add(a: number, b: number): number { return a + b; } const result = add(num, 10);
In this example, TypeScript infers the types of the variables num
, str
, and bool
as number
, string
, and boolean
, respectively, based on the initialization values. It also infers the types of the parameters a
and b
in the add
function as number
and the return type as number
based on the explicit type annotations.
Type inference is a useful feature of TypeScript that reduces the need for explicit type annotations and makes the code more concise and readable.
Related Article: Using ESLint & eslint-config-standard-with-typescript
Why do we need type annotations in TypeScript?
While TypeScript can infer types in many cases, there are situations where explicit type annotations are necessary. Type annotations provide additional clarity and documentation, especially when working with complex or ambiguous code. They also help catch potential type errors and enforce stricter type checking.
Explicit type annotations can be used to specify the types of variables, function parameters, and function return values. They are written using the :
symbol followed by the desired type.
For example, consider the following TypeScript code snippet:
let num: number = 42; let str: string = 'Hello, TypeScript'; let bool: boolean = true; function add(a: number, b: number): number { return a + b; } const result: number = add(num, 10);
In this example, explicit type annotations are used to specify the types of the variables num
, str
, bool
, and result
, as well as the function parameters a
and b
, and the function return type.
Type annotations provide additional clarity and help ensure type safety in TypeScript codebases.
What are type guards in TypeScript?
Type guards are a feature in TypeScript that allows developers to narrow down the type of a variable within a conditional block. They enable more precise type checking and can be used to perform type-specific operations based on runtime conditions.
Type guards can be implemented using various techniques, such as typeof
checks, instanceof
checks, and custom type predicates. They help TypeScript infer more specific types and enable type-specific operations without resorting to type assertions.
For example, consider the following TypeScript code snippet:
function printLength(value: string | number) { if (typeof value === 'string') { console.log(value.length); } else if (typeof value === 'number') { console.log(Math.abs(value)); } } printLength('Hello'); printLength(42);
In this example, the printLength
function accepts a parameter value
of type string | number
, which means it can be either a string or a number. Within the function, type guards using typeof
checks are used to narrow down the type of value
and perform type-specific operations.
Type guards are a useful tool in TypeScript that allows for more precise type checking and enables type-specific operations without sacrificing type safety.
How do type aliases work in TypeScript?
Type aliases allow developers to create custom names for existing types in TypeScript. They provide a way to create more expressive and reusable type definitions, making the code more readable and maintainable.
Type aliases are defined using the type
keyword followed by the desired name and the type definition. They can be used to create aliases for any type, including primitive types, object types, union types, and intersection types.
For example, consider the following TypeScript code snippet:
type Point = { x: number; y: number; }; type Shape = 'circle' | 'square' | 'triangle'; const origin: Point = { x: 0, y: 0 }; const shape: Shape = 'circle';
In this example, the Point
type alias is defined to represent a point in a two-dimensional coordinate system. The Shape
type alias is defined to represent different shapes.
Type aliases make the code more expressive and reusable by providing custom names for existing types.
Related Article: How to Work with Anonymous Classes in TypeScript
What is type narrowing in TypeScript?
Type narrowing is a feature in TypeScript that allows developers to narrow down the type of a variable within a conditional block. It enables more precise type checking and can be used to perform type-specific operations based on runtime conditions.
Type narrowing can be achieved using various techniques, such as typeof
checks, instanceof
checks, and custom type predicates. It helps TypeScript infer more specific types and enables type-specific operations without resorting to type assertions.
For example, consider the following TypeScript code snippet:
function printLength(value: string | number) { if (typeof value === 'string') { console.log(value.length); } else if (typeof value === 'number') { console.log(Math.abs(value)); } } printLength('Hello'); printLength(42);
In this example, the printLength
function accepts a parameter value
of type string | number
, which means it can be either a string or a number. Within the function, type narrowing using typeof
checks is used to narrow down the type of value
and perform type-specific operations.
Type narrowing is a useful feature in TypeScript that allows for more precise type checking and enables type-specific operations without sacrificing type safety.
How does the TypeScript type system work?
The TypeScript type system is based on a combination of type inference and explicit type annotations. It uses static type checking to catch potential errors and enforce type safety during the compilation process.
TypeScript performs type inference by analyzing the code and making educated guesses about the types based on the available information. It infers types for variables, parameters, and function return values based on their initialization values, usage context, and explicit type annotations.
TypeScript also allows developers to provide explicit type annotations to specify the types of variables, function parameters, and function return values. These annotations provide additional clarity and documentation, especially when working with complex or ambiguous code.
During the compilation process, TypeScript checks if the types used in the code are compatible and raises errors if there are any type mismatches. It ensures that variables are assigned values of compatible types, function parameters are passed values of matching types, and function return values conform to the specified types.
The TypeScript type system helps catch potential errors early in the development process, improves code readability and maintainability, and enables more robust and scalable JavaScript development.
Code Snippet: Building a basic rules engine
type Rule = (data: any) => boolean; class RulesEngine { rules: Rule[]; constructor() { this.rules = []; } addRule(rule: Rule) { this.rules.push(rule); } executeRules(data: any): boolean { for (const rule of this.rules) { if (!rule(data)) { return false; } } return true; } } const engine = new RulesEngine(); engine.addRule((data) => data > 0); engine.addRule((data) => data < 10); console.log(engine.executeRules(5)); // Output: true console.log(engine.executeRules(15)); // Output: false
In this code snippet, we define a Rule
type alias, which represents a function that takes data
as a parameter and returns a boolean. We then create a RulesEngine
class, which has an array of rules and methods to add rules and execute them.
The addRule
method adds a rule to the engine’s list of rules. The executeRules
method iterates over the rules and returns false
if any rule returns false
, otherwise it returns true
.
We create an instance of the RulesEngine
class, add two rules (checking if the data is greater than 0 and less than 10), and execute the rules with different data values.
Related Article: How to Implement ETL Processes with TypeScript
Code Snippet: Implementing type checking in a rules engine
type Rule = (data: T) => boolean; class RulesEngine { rules: Rule[]; constructor() { this.rules = []; } addRule(rule: Rule) { this.rules.push(rule); } executeRules(data: T): boolean { for (const rule of this.rules) { if (!rule(data)) { return false; } } return true; } } const engine = new RulesEngine(); engine.addRule((data) => data > 0); engine.addRule((data) => data < 10); console.log(engine.executeRules(5)); // Output: true console.log(engine.executeRules(15)); // Output: false
In this code snippet, we enhance the previous RulesEngine
class by making it generic. We introduce a generic type parameter T
, which represents the type of the data that the rules operate on.
The Rule
type alias is now also generic, taking data
of type T
as a parameter. This allows the rules to operate on data of any type.
We create an instance of the RulesEngine
class with number
as the generic type parameter, add two rules, and execute the rules with different data values of type number
.
This implementation provides type checking for the rules engine, ensuring that the rules operate on the correct type of data.
Code Snippet: Using conditional types in a rules engine
type Rule = T extends string ? (data: T) => boolean : (data: T) => boolean[]; class RulesEngine { rules: Rule[]; constructor() { this.rules = []; } addRule(rule: Rule) { this.rules.push(rule); } executeRules(data: T): boolean { for (const rule of this.rules) { const result = rule(data); if (Array.isArray(result) ? result.includes(false) : !result) { return false; } } return true; } } const engine = new RulesEngine(); engine.addRule((data) => typeof data === 'string'); engine.addRule((data) => typeof data === 'number'); engine.addRule((data) => Array.isArray(data)); console.log(engine.executeRules('Hello')); // Output: true console.log(engine.executeRules(42)); // Output: true console.log(engine.executeRules([1, 2, 3])); // Output: true console.log(engine.executeRules(true)); // Output: false
In this code snippet, we use conditional types in the Rule
type alias to differentiate between rules that return a boolean and rules that return an array of booleans. If T
is a string, the rule returns a boolean; otherwise, it returns an array of booleans.
The executeRules
method is updated to handle both types of rules. If a rule returns an array, it checks if any false
values are present; otherwise, it checks if the rule result is false
.
We create an instance of the RulesEngine
class with string | number
as the generic type parameter, add three rules (checking if the data is a string, a number, or an array), and execute the rules with different data values.
This implementation demonstrates how conditional types can be used to handle different rule return types in a rules engine.
Code Snippet: Leveraging type inference in a rules engine
type Rule = (data: T) => boolean; class RulesEngine { rules: Rule[]; constructor() { this.rules = []; } addRule(rule: Rule) { this.rules.push(rule); } executeRules(data: T): boolean { for (const rule of this.rules) { if (!rule(data)) { return false; } } return true; } } const engine = new RulesEngine(); engine.addRule((data) => typeof data === 'string'); engine.addRule((data) => typeof data === 'number'); console.log(engine.executeRules('Hello')); // Output: true console.log(engine.executeRules(42)); // Output: true console.log(engine.executeRules(true)); // Output: false
In this code snippet, we leverage type inference to infer the type of the data passed to the rules engine. Instead of specifying a generic type parameter for the RulesEngine
class, we allow TypeScript to infer it based on the types of the rules added.
We create an instance of the RulesEngine
class without specifying the generic type parameter. We then add two rules (checking if the data is a string or a number) without explicitly annotating the types of the parameters in the rules. TypeScript infers the types based on the function bodies.
We execute the rules with different data values and observe that TypeScript correctly infers the types and performs the necessary type checking.
This implementation demonstrates how type inference can be leveraged to simplify the usage of a rules engine.
Related Article: TypeScript ETL (Extract, Transform, Load) Tutorial
Code Snippet: Adding type annotations to a rules engine
type Rule = (data: T) => boolean; class RulesEngine { rules: Rule[]; constructor() { this.rules = []; } addRule(rule: Rule) { this.rules.push(rule); } executeRules(data: T): boolean { for (const rule of this.rules) { if (!rule(data)) { return false; } } return true; } } const engine = new RulesEngine(); engine.addRule((data: string) => typeof data === 'string'); engine.addRule((data: number) => typeof data === 'number'); console.log(engine.executeRules('Hello')); // Output: true console.log(engine.executeRules(42)); // Output: true console.log(engine.executeRules(true)); // Output: Error
In this code snippet, we add explicit type annotations to the parameters of the rules added to the rules engine. Instead of relying on type inference, we specify the types of the parameters in the rule functions.
We create an instance of the RulesEngine
class with string | number
as the generic type parameter. We then add two rules, each with an explicit type annotation for the parameter. TypeScript checks that the types of the rule functions match the specified types when adding the rules.
We execute the rules with different data values and observe that TypeScript performs the necessary type checking and raises errors when incompatible data types are used.
This implementation demonstrates how explicit type annotations can be used to provide additional type safety in a rules engine.
Code Snippet: Applying type guards in a rules engine
type Rule = (data: T) => boolean; class RulesEngine { rules: Rule[]; constructor() { this.rules = []; } addRule(rule: Rule) { this.rules.push(rule); } executeRules(data: T): boolean { for (const rule of this.rules) { if (typeof data === 'string' && !rule(data)) { return false; } else if (typeof data === 'number' && !rule(data)) { return false; } } return true; } } const engine = new RulesEngine(); engine.addRule((data: string) => typeof data === 'string'); engine.addRule((data: number) => typeof data === 'number'); console.log(engine.executeRules('Hello')); // Output: true console.log(engine.executeRules(42)); // Output: true console.log(engine.executeRules(true)); // Output: false
In this code snippet, we apply type guards in the executeRules
method of the rules engine. Instead of relying on type inference or explicit type annotations, we use typeof
checks to narrow down the type of the data and perform type-specific operations within the conditional blocks.
We create an instance of the RulesEngine
class with string | number
as the generic type parameter. We then add two rules, each with a type guard using typeof
checks. TypeScript checks that the type guards are correctly applied and performs the necessary type checking.
We execute the rules with different data values and observe that TypeScript correctly infers the types and performs the necessary type-specific operations.
This implementation demonstrates how type guards can be used to handle different data types in a rules engine.
Code Snippet: Utilizing type aliases in a rules engine
type Rule = (data: T) => boolean; type NumberRule = Rule; type StringRule = Rule; class RulesEngine { rules: Rule[]; constructor() { this.rules = []; } addRule(rule: Rule) { this.rules.push(rule); } executeRules(data: T): boolean { for (const rule of this.rules) { if (!rule(data)) { return false; } } return true; } } const engine = new RulesEngine(); engine.addRule((data: number) => data > 0); engine.addRule((data: number) => data < 10); console.log(engine.executeRules(5)); // Output: true console.log(engine.executeRules(15)); // Output: false
In this code snippet, we utilize type aliases to create aliases for specific types of rules in the rules engine. We define NumberRule
as a type alias for a rule that operates on numbers, and StringRule
as a type alias for a rule that operates on strings.
The RulesEngine
class is unchanged, but we specify number
as the generic type parameter when creating an instance of the class. We then add two number rules to the engine.
We execute the rules with different number values and observe that the type aliases provide additional clarity and enforce type safety.
This implementation demonstrates how type aliases can be used to create more expressive and reusable type definitions in a rules engine.
Related Article: Tutorial on Circuit Breaker Pattern in TypeScript
Code Snippet: Implementing type narrowing in a rules engine
type Rule = (data: T) => boolean; class RulesEngine { rules: Rule[]; constructor() { this.rules = []; } addRule(rule: Rule) { this.rules.push(rule); } executeRules(data: T): boolean { for (const rule of this.rules) { if (!rule(data)) { return false; } } return true; } } const engine = new RulesEngine(); engine.addRule((data: string) => typeof data === 'string'); engine.addRule((data: number) => typeof data === 'number'); console.log(engine.executeRules('Hello')); // Output: true console.log(engine.executeRules(42)); // Output: true console.log(engine.executeRules(true)); // Output: Error
In this code snippet, we implement type narrowing in the executeRules
method of the rules engine. Instead of relying on type inference or explicit type annotations, we use typeof
checks to narrow down the type of the data within the conditional blocks.
We create an instance of the RulesEngine
class with string | number
as the generic type parameter. We then add two rules, each with an explicit type annotation for the parameter and a type guard using typeof
checks. TypeScript checks that the type guards are correctly applied and performs the necessary type narrowing.
We execute the rules with different data values and observe that TypeScript narrows down the types and performs the necessary type-specific operations.
This implementation demonstrates how type narrowing can be used to handle different data types and enforce type safety in a rules engine.
Code Snippet: Understanding the TypeScript type system in a rules engine
type Rule = (data: T) => boolean; class RulesEngine { rules: Rule[]; constructor() { this.rules = []; } addRule(rule: Rule) { this.rules.push(rule); } executeRules(data: T): boolean { for (const rule of this.rules) { if (!rule(data)) { return false; } } return true; } } const engine = new RulesEngine(); engine.addRule((data: string) => typeof data === 'string'); engine.addRule((data: number) => typeof data === 'number'); console.log(engine.executeRules('Hello')); // Output: true console.log(engine.executeRules(42)); // Output: true console.log(engine.executeRules(true)); // Output: Error
In this code snippet, we showcase the TypeScript type system in the context of a rules engine. We define the Rule
type alias, which represents a function that takes data
of type T
and returns a boolean.
The RulesEngine
class is generic, with T
representing the type of the data that the rules operate on. It has methods to add rules and execute them. The executeRules
method iterates over the rules and returns false
if any rule returns false
, otherwise it returns true
.
We create an instance of the RulesEngine
class with string | number
as the generic type parameter. We add two rules, each with an explicit type annotation for the parameter. TypeScript checks that the types of the rule functions match the specified types when adding the rules.
We execute the rules with different data values and observe that TypeScript performs the necessary type checking and enforces type safety.
This code snippet provides an overview of how the TypeScript type system works in the context of a rules engine.