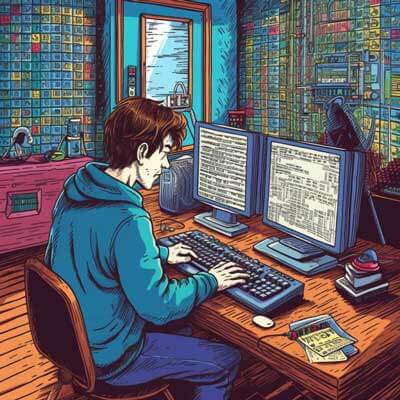
- Setting a Value in TypeScript
- Setting the Value of a Variable in TypeScript
- Setting the Value of an Object Property in TypeScript
- Updating a Value in TypeScript
- Setting the Value of a Property in TypeScript
- Changing a Value in TypeScript
- Setting a Value to null in TypeScript
- Setting a Value to undefined in TypeScript
- Setting a Value to a Boolean in TypeScript
- Setting a Value to a Number in TypeScript
- External Sources
Setting a Value in TypeScript
In TypeScript, you can set a value to a variable or property using the =
operator. This allows you to assign a specific value to a variable or change the value of an existing variable. Let’s take a look at some examples:
Example 1: Setting a value to a variable
let name: string; name = "John"; console.log(name); // Output: John
In this example, we declare a variable name
of type string
and assign the value “John” to it. We then log the value of the name
variable to the console, which will output “John”.
Example 2: Setting a value to a property
class Person { name: string; } let person = new Person(); person.name = "John"; console.log(person.name); // Output: John
In this example, we define a class Person
with a property name
of type string
. We then create an instance of the Person
class and set the value of the name
property to “John”. Finally, we log the value of the name
property to the console, which will output “John”.
Related Article: How to Implement and Use Generics in Typescript
Setting the Value of a Variable in TypeScript
To set the value of a variable in TypeScript, you can use the =
operator followed by the desired value. Let’s look at an example:
Example:
let age: number; age = 25; console.log(age); // Output: 25
In this example, we declare a variable age
of type number
and assign the value 25 to it. We then log the value of the age
variable to the console, which will output 25.
Setting the Value of an Object Property in TypeScript
To set the value of an object property in TypeScript, you can use the =
operator followed by the desired value. Let’s see an example:
Example:
let person = { name: "John", age: 25 }; person.name = "Jane"; console.log(person.name); // Output: Jane
In this example, we define an object person
with properties name
and age
. We initially set the value of the name
property to “John” and the value of the age
property to 25. Then, we update the value of the name
property to “Jane”. Finally, we log the updated value of the name
property to the console, which will output “Jane”.
Updating a Value in TypeScript
To update a value in TypeScript, you can simply assign a new value to the variable or property. Let’s take a look at an example:
Example 1: Updating a value of a variable
let age: number; age = 25; console.log(age); // Output: 25 age = 30; console.log(age); // Output: 30
In this example, we declare a variable age
of type number
and assign the initial value 25 to it. We then log the value of the age
variable to the console, which will output 25. After that, we update the value of the age
variable to 30 and log it again, which will output 30.
Example 2: Updating a value of an object property
let person = { name: "John", age: 25 }; console.log(person.age); // Output: 25 person.age = 30; console.log(person.age); // Output: 30
In this example, we define an object person
with properties name
and age
. We initially set the value of the age
property to 25. We then log the value of the age
property to the console, which will output 25. After that, we update the value of the age
property to 30 and log it again, which will output 30.
Related Article: Tutorial: Navigating the TypeScript Exit Process
Setting the Value of a Property in TypeScript
In TypeScript, you can set the value of a property using the =
operator. This allows you to assign a specific value to a property or change the value of an existing property. Let’s take a look at an example:
Example:
class Person { name: string; age: number; } let person = new Person(); person.name = "John"; person.age = 25; console.log(person.name); // Output: John console.log(person.age); // Output: 25
In this example, we define a class Person
with properties name
and age
. We then create an instance of the Person
class and set the values of the name
and age
properties. Finally, we log the values of the name
and age
properties to the console, which will output “John” and 25, respectively.
Changing a Value in TypeScript
To change a value in TypeScript, you can simply assign a new value to the variable or property. Let’s see an example:
Example 1: Changing the value of a variable
let age: number; age = 25; console.log(age); // Output: 25 age = 30; console.log(age); // Output: 30
In this example, we declare a variable age
of type number
and assign the initial value 25 to it. We then log the value of the age
variable to the console, which will output 25. After that, we change the value of the age
variable to 30 and log it again, which will output 30.
Example 2: Changing the value of an object property
let person = { name: "John", age: 25 }; console.log(person.age); // Output: 25 person.age = 30; console.log(person.age); // Output: 30
In this example, we define an object person
with properties name
and age
. We initially set the value of the age
property to 25. We then log the value of the age
property to the console, which will output 25. After that, we change the value of the age
property to 30 and log it again, which will output 30.
Setting a Value to null in TypeScript
In TypeScript, you can set a value to null
to indicate the absence of an object or the intentional assignment of a null value. Let’s see an example:
Example:
let person: string | null; person = "John"; console.log(person); // Output: John person = null; console.log(person); // Output: null
In this example, we declare a variable person
that can hold a value of type string
or null
. We initially assign the value “John” to the person
variable and log it to the console, which will output “John”. After that, we set the value of the person
variable to null
and log it again, which will output null
.
Related Article: Tutorial on TypeScript Dynamic Object Manipulation
Setting a Value to undefined in TypeScript
In TypeScript, you can set a value to undefined
to indicate the absence of a value or the intentional assignment of an undefined value. Let’s see an example:
Example:
let age: number | undefined; console.log(age); // Output: undefined age = 25; console.log(age); // Output: 25 age = undefined; console.log(age); // Output: undefined
In this example, we declare a variable age
that can hold a value of type number
or undefined
. Initially, the value of the age
variable is undefined
, and when logged to the console, it will output undefined
. We then set the value of the age
variable to 25 and log it again, which will output 25. Finally, we set the value of the age
variable to undefined
and log it again, which will output undefined
.
Setting a Value to a Boolean in TypeScript
In TypeScript, you can set a value to a boolean using the =
operator followed by either true
or false
. Let’s see an example:
Example:
let isRaining: boolean; isRaining = true; console.log(isRaining); // Output: true isRaining = false; console.log(isRaining); // Output: false
In this example, we declare a variable isRaining
of type boolean
and assign the value true
to it. We then log the value of the isRaining
variable to the console, which will output true
. After that, we assign the value false
to the isRaining
variable and log it again, which will output false
.
Setting a Value to a Number in TypeScript
To set a value to a number in TypeScript, you can use the =
operator followed by a numeric value. Let’s look at an example:
Example:
let age: number; age = 25; console.log(age); // Output: 25
In this example, we declare a variable age
of type number
and assign the value 25 to it. We then log the value of the age
variable to the console, which will output 25.
Related Article: Tutorial: Checking Enum Value Existence in TypeScript
External Sources
Here are some external sources for further reading:
– TypeScript Documentation: https://www.typescriptlang.org/docs/