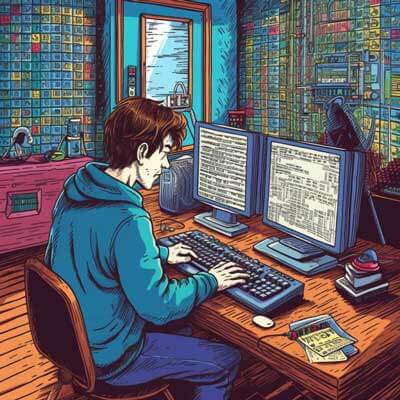
- Setting Up a TypeScript Project
- Installing React-Toastify
- Configuring React-Toastify
- Displaying Basic Toast Notifications
- Customizing Toast Notifications
- Handling Toast Actions
- Controlling Toast Lifespan
- Creating Multiple Toast Containers
- Adding Icons to Toast Notifications
- Closing Toast Notifications
- Positioning Toast Notifications
- Styling Toast Notifications
- Translations and Internationalization
- Handling Toast Events
- Using React-Toastify with Redux
- Testing React-Toastify Components
- FAQs and Troubleshooting
Setting Up a TypeScript Project
To use React-Toastify with TypeScript, you need to set up a TypeScript project first. Here are the steps to create a new TypeScript project:
Step 1: Install TypeScript globally if you haven’t already done so:
npm install -g typescript
Step 2: Create a new directory for your project and navigate into it:
mkdir react-toastify-typescript-example cd react-toastify-typescript-example
Step 3: Initialize a new TypeScript project:
tsc --init
This will create a tsconfig.json
file in your project directory.
Step 4: Install React and React-DOM packages:
npm install react react-dom
Step 5: Install additional TypeScript dependencies:
npm install --save-dev @types/react @types/react-dom
Now you have set up a basic TypeScript project and are ready to install React-Toastify.
Related Article: How to Run Typescript Ts-Node in Databases
Installing React-Toastify
To install React-Toastify, run the following command in your project directory:
npm install react-toastify
This will install React-Toastify and add it as a dependency in your package.json
file.
Configuring React-Toastify
Before we can start using React-Toastify, we need to configure it. Create a new file called ToastConfig.ts
and add the following code:
import { ToastContainer } from "react-toastify"; import "react-toastify/dist/ReactToastify.css"; ToastContainer.defaultProps = { position: "bottom-right", autoClose: 5000, hideProgressBar: false, newestOnTop: false, closeOnClick: true, rtl: false, pauseOnFocusLoss: true, draggable: true, pauseOnHover: true, }; export default ToastContainer;
This code sets the default configuration values for React-Toastify, such as the position of the toast notifications, the duration they are displayed, and the appearance of the progress bar.
Displaying Basic Toast Notifications
To display a basic toast notification, import the toast
function from React-Toastify and call it with a message as an argument. Here’s an example:
import { toast } from "react-toastify"; // Inside a React component's function body const handleClick = () => { toast("Hello, world!"); } return ( <button>Show Toast</button> );
This code creates a button that, when clicked, shows a toast notification with the message “Hello, world!”
You can also customize the appearance of the toast notifications. Let’s move on to the next section to learn more about customization.
Customizing Toast Notifications
React-Toastify provides various options to customize the appearance of toast notifications. Here are a few examples:
1. Changing the position of the toast notifications:
toast.configure({ position: "top-left", });
2. Changing the appearance of the progress bar:
toast.configure({ progressBar: true, progressClassName: "custom-progress-bar", });
3. Adding a custom CSS class to the toast notifications:
toast.configure({ className: "custom-toast", bodyClassName: "custom-toast-body", });
These are just a few examples of how you can customize your toast notifications. Refer to the React-Toastify documentation for a complete list of available options.
Handling Toast Actions
Toast notifications can also include action buttons that allow users to perform certain actions. To add an action button to a toast notification, use the toast
function’s onClick
option. Here’s an example:
import { toast } from "react-toastify"; // Inside a React component's function body const handleClick = () => { toast("Hello, world!", { onClick: () => { console.log("Toast clicked!"); }, closeOnClick: false, }); } return ( <button>Show Toast</button> );
In this example, when the toast notification is clicked, the onClick
callback function is called and a message is logged to the console.
Controlling Toast Lifespan
import { toast } from "react-toastify"; // Inside a React component's function body const handleClick = () => { toast("Hello, world!", { autoClose: false, // Disable auto-close }); } return ( <button>Show Toast</button> );
In this example, the toast notification will not automatically close and will remain visible until the user manually closes it.
Creating Multiple Toast Containers
React-Toastify supports multiple toast containers, which allow you to display toast notifications in different parts of your application. To create a new toast container, use the toast.configure
function. Here’s an example:
import { toast, ToastContainer } from "react-toastify"; // Inside a React component's function body const handleClick = () => { toast("Hello from container 1!"); toast("Hello from container 2!", { containerId: "container2" }); } return ( <div> <button>Show Toast</button> </div> );
In this example, two toast containers are created: one without a specified containerId
, which is the default container, and another with the containerId
“container2”. Each container can have its own configuration and position.
Adding Icons to Toast Notifications
You can add icons to toast notifications by using custom CSS classes or by using a library like Font Awesome. Here’s an example of adding an icon using Font Awesome:
Step 1: Install Font Awesome:
npm install @fortawesome/fontawesome-svg-core @fortawesome/free-solid-svg-icons @fortawesome/react-fontawesome
Step 2: Import the required packages and the desired icon:
import { toast } from "react-toastify"; import { FontAwesomeIcon } from "@fortawesome/react-fontawesome"; import { faInfoCircle } from "@fortawesome/free-solid-svg-icons";
Step 3: Use the progressClassName
and bodyClassName
options to add the icon:
toast.configure({ progressClassName: "custom-progress-bar", bodyClassName: "custom-toast-body", }); // Inside a React component's function body const handleClick = () => { toast( <div>Hello, world!</div> ); } return ( <button>Show Toast</button> );
In this example, the Font Awesome icon faInfoCircle
is added before the message “Hello, world!” in the toast notification.
Closing Toast Notifications
Toast notifications can be closed manually by the user or programmatically. To close a toast notification programmatically, you can use the toast.dismiss
function. Here’s an example:
import { toast } from "react-toastify"; // Inside a React component's function body const handleClick = () => { const toastId = toast("Hello, world!"); setTimeout(() => { toast.dismiss(toastId); }, 3000); } return ( <button>Show Toast</button> );
In this example, the toast.dismiss
function is called after a delay of 3000 milliseconds to close the toast notification.
Positioning Toast Notifications
React-Toastify allows you to position toast notifications in different parts of the screen. Some of the available positions include “top-left”, “top-center”, “top-right”, “bottom-left”, “bottom-center”, and “bottom-right”. Here’s an example:
toast.configure({ position: "top-center", });
This code sets the position of the toast notifications to the top center of the screen.
Styling Toast Notifications
You can customize the appearance of toast notifications by adding custom CSS classes. Here’s an example of adding a custom CSS class to toast notifications:
Step 1: Create a CSS file called ToastStyles.css
and add the following code:
.custom-toast { background-color: #333; color: #fff; } .custom-toast-body { font-size: 16px; }
Step 2: Import the CSS file in your project:
import "path/to/ToastStyles.css";
Step 3: Configure React-Toastify to use the custom CSS class:
toast.configure({ className: "custom-toast", bodyClassName: "custom-toast-body", });
In this example, the toast notifications will have a background color of #333 and white text color, and the font size will be 16 pixels.
Translations and Internationalization
React-Toastify supports translations and internationalization. You can use the toast.configure
function to set the translations for different languages. Here’s an example:
toast.configure({ autoClose: 5000, draggable: false, rtl: true, // Add more options for different languages }); // Inside a React component's function body const handleClick = () => { toast("Hello, world!"); } return ( <button>Show Toast</button> );
In this example, the toast notifications will automatically close after 5000 milliseconds, dragging will be disabled, and the layout will be right-to-left (RTL).
Handling Toast Events
React-Toastify provides event handlers for toast notifications. You can use these event handlers to perform actions when a toast is displayed, hidden, or clicked. Here’s an example:
import { toast } from "react-toastify"; // Inside a React component's function body const handleClick = () => { toast("Hello, world!", { onOpen: () => { console.log("Toast opened!"); }, onClose: () => { console.log("Toast closed!"); }, onClick: () => { console.log("Toast clicked!"); }, }); } return ( <button>Show Toast</button> );
In this example, when the toast notification is opened, closed, or clicked, a corresponding message is logged to the console.
Using React-Toastify with Redux
React-Toastify can be easily integrated with Redux for managing toast notifications in a centralized state. Here’s an example of how to use React-Toastify with Redux:
Step 1: Install Redux and React-Redux packages:
npm install redux react-redux
Step 2: Create a Redux store and configure React-Toastify to use the store:
import { createStore } from "redux"; import { Provider } from "react-redux"; import { ToastContainer, toast } from "react-toastify"; // Redux actions and reducers // ... const store = createStore(reducer); // Inside a React component's function body const handleClick = () => { store.dispatch({ type: "SHOW_TOAST", message: "Hello, world!" }); } return ( <button>Show Toast</button> );
In this example, the Redux store is created using the createStore
function, and the Provider
component from React-Redux is used to provide the store to the component tree. The toast
function is used inside a Redux action to show the toast notification.
Testing React-Toastify Components
When it comes to testing React-Toastify components, you can use popular testing libraries like Jest and React Testing Library. Here’s an example of how to test a component that uses React-Toastify:
Step 1: Install the required testing dependencies:
npm install --save-dev jest react-testing-library
Step 2: Create a test file for the component and write a test case:
import React from "react"; import { render, fireEvent } from "@testing-library/react"; import { toast } from "react-toastify"; import MyComponent from "./MyComponent"; jest.mock("react-toastify", () => ({ toast: jest.fn(), })); test("should show toast notification when button is clicked", () => { const { getByText } = render(); const button = getByText("Show Toast"); fireEvent.click(button); expect(toast).toHaveBeenCalledWith("Hello, world!"); });
In this example, the render
function from React Testing Library is used to render the component, and the fireEvent
function is used to simulate a button click. The toast
function is mocked using Jest’s mock
function, and the expectation checks if the toast
function was called with the correct message.
FAQs and Troubleshooting
Q: Is React-Toastify compatible with React Native?
A: No, React-Toastify is designed for web applications and is not compatible with React Native.
Q: How can I use custom icons in toast notifications?
A: You can use custom CSS classes or libraries like Font Awesome to add custom icons to toast notifications. Refer to the “Adding Icons to Toast Notifications” section for more details.
Q: How can I change the appearance of the progress bar?
A: You can customize the appearance of the progress bar by adding custom CSS classes. Refer to the “Customizing Toast Notifications” section for an example.
Q: Can I use React-Toastify with Redux?
A: Yes, React-Toastify can be easily integrated with Redux. Refer to the “Using React-Toastify with Redux” section for an example.
Q: How can I test components that use React-Toastify?
A: You can use testing libraries like Jest and React Testing Library to test components that use React-Toastify. Refer to the “Testing React-Toastify Components” section for an example.