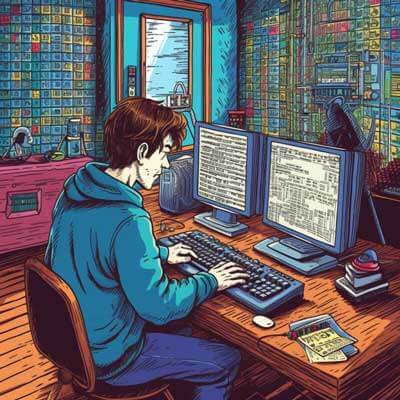
Table of Contents
What is a While Loop?
A while loop is a control flow statement that allows a block of code to be executed repeatedly as long as a specified condition is true. It is a fundamental looping construct in programming languages, including TypeScript. The while loop is used when the number of iterations is unknown or dependent on a condition that is evaluated at runtime.
Related Article: How to Check if a String is in Enum in TypeScript: A Tutorial
Syntax of a While Loop
The syntax of a while loop in TypeScript is as follows:
while (condition) { // code to be executed }
The condition is a boolean expression that determines whether the loop should continue or terminate. If the condition is true, the block of code inside the loop is executed. After each iteration, the condition is evaluated again, and if it is still true, the loop continues. If the condition is false, the loop is terminated, and the program execution continues with the next statement after the loop.
Breaking Out of a While Loop
To break out of a while loop prematurely, you can use the break
statement. When the break
statement is encountered inside a loop, the loop is immediately terminated, and the program execution continues with the next statement after the loop. This can be useful when you want to exit a loop based on a certain condition.
Here's an example that demonstrates the use of the break
statement in a while loop:
let i = 0; while (i < 5) { console.log(i); if (i === 3) { break; } i++; }
Output:
0 1 2 3
In this example, the loop is terminated when the value of i
is equal to 3. The break
statement is used to exit the loop at that point.
Skipping an Iteration in a While Loop
To skip the current iteration of a while loop and continue with the next iteration, you can use the continue
statement. When the continue
statement is encountered inside a loop, the remaining statements in the loop body are skipped, and the loop condition is evaluated again. The loop then either continues with the next iteration or terminates if the condition is false.
Here's an example that demonstrates the use of the continue
statement in a while loop:
let i = 0; while (i < 5) { i++; if (i === 3) { continue; } console.log(i); }
Output:
1 2 4 5
In this example, the value of i
is incremented at the beginning of each iteration. When the value of i
is equal to 3, the continue
statement is encountered, and the remaining statements in the loop body are skipped. The loop then continues with the next iteration.
Related Article: Using ESLint & eslint-config-standard-with-typescript
Multiple Conditions in a While Loop
In TypeScript, you can have multiple conditions in a while loop by using logical operators such as &&
(logical AND) and ||
(logical OR) to combine multiple conditions into a single boolean expression.
Here's an example that demonstrates the use of multiple conditions in a while loop:
let i = 0; let j = 0; while (i < 5 && j < 3) { console.log(i, j); i++; j++; }
Output:
0 0 1 1 2 2
In this example, the loop continues as long as both the conditions i < 5
and j < 3
are true. Once one of the conditions becomes false, the loop is terminated.
Code Snippet - While Loop Example
let i = 0; while (i < 5) { console.log(i); i++; }
Output:
0 1 2 3 4
This code snippet demonstrates a simple while loop that prints the values of i
from 0 to 4.
Nested While Loops
A nested while loop is a loop inside another loop. It allows you to perform more complex iterations by nesting one loop within another. Each time the outer loop iterates, the inner loop completes its full iteration before the outer loop moves to the next iteration.
Here's an example that demonstrates a nested while loop:
let i = 0; while (i < 3) { let j = 0; while (j < 3) { console.log(i, j); j++; } i++; }
Output:
0 0 0 1 0 2 1 0 1 1 1 2 2 0 2 1 2 2
In this example, the outer loop iterates from 0 to 2, and for each iteration of the outer loop, the inner loop iterates from 0 to 2. The values of i
and j
are printed for each iteration.
The Difference Between While Loop and Do-While Loop
The main difference between a while loop and a do-while loop is that a while loop evaluates the condition at the beginning of each iteration, while a do-while loop evaluates the condition at the end of each iteration. This means that a do-while loop always executes the loop body at least once, regardless of whether the condition is initially true or false.
Here's an example that demonstrates the difference between a while loop and a do-while loop:
let i = 5; while (i < 5) { console.log("While loop"); } do { console.log("Do-while loop"); } while (i < 5);
Output:
Do-while loop
In this example, the condition of the while loop is initially false, so the loop body is not executed at all. However, the condition of the do-while loop is evaluated at the end of the first iteration, and since the condition is false, the loop terminates. But the loop body is still executed once.
Related Article: Tutorial: Generating GUID in TypeScript
Creating an Infinite While Loop
An infinite while loop is a loop that runs indefinitely because the loop condition is always true. It continues to execute until it is terminated externally, such as by using the break
statement or by terminating the program.
Here's an example of creating an infinite while loop:
while (true) { console.log("This is an infinite loop"); }
Output:
This is an infinite loop This is an infinite loop This is an infinite loop ...
In this example, the condition of the while loop is always true, so the loop continues to execute indefinitely. To terminate the loop, you would need to manually stop the execution, such as by using the Ctrl+C
command.
Code Snippet - Using a While Loop in TypeScript
let i = 0; while (i < 5) { console.log(i); i++; }
Output:
0 1 2 3 4
This code snippet demonstrates a simple example of using a while loop in TypeScript. The loop starts with i
initialized to 0, and as long as i
is less than 5, the loop body is executed, incrementing i
by 1 in each iteration.
Can You Use a While Loop in TypeScript?
Yes, you can use a while loop in TypeScript. TypeScript is a superset of JavaScript, and it inherits all the looping constructs from JavaScript, including the while loop. The syntax and behavior of the while loop in TypeScript are the same as in JavaScript.
Syntax for a While Loop in TypeScript
The syntax for a while loop in TypeScript is the same as in JavaScript:
while (condition) { // code to be executed }
The condition is a boolean expression that determines whether the loop should continue or terminate. If the condition is true, the block of code inside the loop is executed. After each iteration, the condition is evaluated again, and if it is still true, the loop continues. If the condition is false, the loop is terminated, and the program execution continues with the next statement after the loop.
Related Article: Tutorial: Checking if a String is a Number in TypeScript
Breaking Out of a While Loop in TypeScript
To break out of a while loop prematurely in TypeScript, you can use the break
statement. When the break
statement is encountered inside a loop, the loop is immediately terminated, and the program execution continues with the next statement after the loop.
Here's an example that demonstrates the use of the break
statement in a while loop in TypeScript:
let i = 0; while (i < 5) { console.log(i); if (i === 3) { break; } i++; }
Output:
0 1 2 3
In this example, the loop is terminated when the value of i
is equal to 3. The break
statement is used to exit the loop at that point.
Skipping an Iteration in a While Loop in TypeScript
To skip the current iteration of a while loop and continue with the next iteration in TypeScript, you can use the continue
statement. When the continue
statement is encountered inside a loop, the remaining statements in the loop body are skipped, and the loop condition is evaluated again. The loop then either continues with the next iteration or terminates if the condition is false.
Here's an example that demonstrates the use of the continue
statement in a while loop in TypeScript:
let i = 0; while (i < 5) { i++; if (i === 3) { continue; } console.log(i); }
Output:
1 2 4 5
In this example, the value of i
is incremented at the beginning of each iteration. When the value of i
is equal to 3, the continue
statement is encountered, and the remaining statements in the loop body are skipped. The loop then continues with the next iteration.
Creating a Nested While Loop in TypeScript
A nested while loop in TypeScript is a loop inside another loop. It allows you to perform more complex iterations by nesting one loop within another. Each time the outer loop iterates, the inner loop completes its full iteration before the outer loop moves to the next iteration.
Here's an example that demonstrates a nested while loop in TypeScript:
let i = 0; while (i < 3) { let j = 0; while (j < 3) { console.log(i, j); j++; } i++; }
Output:
0 0 0 1 0 2 1 0 1 1 1 2 2 0 2 1 2 2
In this example, the outer loop iterates from 0 to 2, and for each iteration of the outer loop, the inner loop iterates from 0 to 2. The values of i
and j
are printed for each iteration.
Difference Between While Loop and Do-While Loop in TypeScript
The main difference between a while loop and a do-while loop in TypeScript is that a while loop evaluates the condition at the beginning of each iteration, while a do-while loop evaluates the condition at the end of each iteration. This means that a do-while loop always executes the loop body at least once, regardless of whether the condition is initially true or false.
Here's an example that demonstrates the difference between a while loop and a do-while loop in TypeScript:
let i = 5; while (i < 5) { console.log("While loop"); } do { console.log("Do-while loop"); } while (i < 5);
Output:
Do-while loop
In this example, the condition of the while loop is initially false, so the loop body is not executed at all. However, the condition of the do-while loop is evaluated at the end of the first iteration, and since the condition is false, the loop terminates. But the loop body is still executed once.
Related Article: Tutorial: Date Comparison in TypeScript
Creating an Infinite While Loop in TypeScript
An infinite while loop in TypeScript is a loop that runs indefinitely because the loop condition is always true. It continues to execute until it is terminated externally, such as by using the break
statement or by terminating the program.
Here's an example of creating an infinite while loop in TypeScript:
while (true) { console.log("This is an infinite loop"); }
Output:
This is an infinite loop This is an infinite loop This is an infinite loop ...
In this example, the condition of the while loop is always true, so the loop continues to execute indefinitely. To terminate the loop, you would need to manually stop the execution, such as by using the Ctrl+C
command.
Multiple Conditions in a While Loop in TypeScript
In TypeScript, you can have multiple conditions in a while loop by using logical operators such as &&
(logical AND) and ||
(logical OR) to combine multiple conditions into a single boolean expression.
Here's an example that demonstrates the use of multiple conditions in a while loop in TypeScript:
let i = 0; let j = 0; while (i < 5 && j < 3) { console.log(i, j); i++; j++; }
Output:
0 0 1 1 2 2
In this example, the loop continues as long as both the conditions i < 5
and j < 3
are true. Once one of the conditions becomes false, the loop is terminated.
Examples of Using a While Loop in TypeScript
Here are a few examples that demonstrate the usage of a while loop in TypeScript:
Example 1: Computing the factorial of a number
function factorial(n: number): number { let result = 1; let i = 1; while (i <= n) { result *= i; i++; } return result; } console.log(factorial(5)); // Output: 120
Example 2: Finding the sum of numbers in an array
const numbers = [1, 2, 3, 4, 5]; let sum = 0; let i = 0; while (i < numbers.length) { sum += numbers[i]; i++; } console.log(sum); // Output: 15
These examples demonstrate different use cases of a while loop in TypeScript, showcasing its versatility in performing repetitive tasks.
External Sources