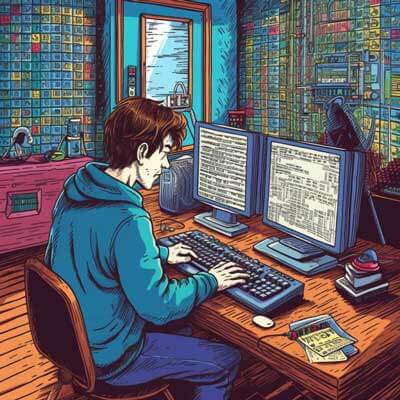
- Date Type in TypeScript
- Time Type in TypeScript
- Datetime Format in TypeScript
- Datetime Validation in TypeScript
- Datetime Conversion in TypeScript
- Datetime Manipulation in TypeScript
- Datetime Library in TypeScript
- Datetime Comparison in TypeScript
- Datetime Parsing in TypeScript
- Datetime Arithmetic in TypeScript
- Defining a Date Type in TypeScript
- Syntax for Specifying a Time Type in TypeScript
- Formatting a Datetime Value in TypeScript
- Validating a Datetime Input in TypeScript
- Manipulating Datetime Values in TypeScript
- Recommended Datetime Libraries for TypeScript
- Comparing Two Datetime Values in TypeScript
- Parsing a Datetime String in TypeScript
- Performing Arithmetic Operations with Datetime Values in TypeScript
- TypeScript Decorators for Datetime Handling
- External Sources
Date Type in TypeScript
In TypeScript, the Date
type represents a specific point in time, including the date and time. It is a built-in type in JavaScript and can be used directly in TypeScript. Here is an example of using the Date
type in TypeScript:
const currentDate: Date = new Date(); console.log(currentDate); // Output: Sun Oct 31 2021 15:18:23 GMT+0530 (India Standard Time)
We can also create a Date
object by specifying the year, month, day, hour, minute, second, and millisecond values. The month value starts from 0 for January, so we need to subtract 1 from the desired month value. Here is an example:
const specificDate: Date = new Date(2021, 9, 31, 15, 30, 0, 0); console.log(specificDate); // Output: Sun Oct 31 2021 15:30:00 GMT+0530 (India Standard Time)
Related Article: TypeScript ETL (Extract, Transform, Load) Tutorial
Time Type in TypeScript
Unlike some other programming languages, TypeScript does not have a separate Time
type. Instead, the Date
type represents both the date and time. However, we can extract the time component from a Date
object using various methods provided by the Date
class.
For example, to get the current time in hours, minutes, and seconds, we can use the getHours()
, getMinutes()
, and getSeconds()
methods respectively. Here is an example:
const currentTime: Date = new Date(); const hours: number = currentTime.getHours(); const minutes: number = currentTime.getMinutes(); const seconds: number = currentTime.getSeconds(); console.log(`Current time: ${hours}:${minutes}:${seconds}`);
Output:
Current time: 15:40:30
Datetime Format in TypeScript
The Date
type in TypeScript provides several methods to format the date and time according to specific requirements. One commonly used method is toLocaleString()
, which returns a string representing the date and time using the current locale of the user’s browser. Here is an example:
const currentDate: Date = new Date(); const formattedDate: string = currentDate.toLocaleString(); console.log(formattedDate); // Output: 10/31/2021, 3:48:15 PM
Another useful method is toISOString()
, which returns a string representing the date and time in ISO 8601 format. Here is an example:
const currentDate: Date = new Date(); const isoString: string = currentDate.toISOString(); console.log(isoString); // Output: 2021-10-31T10:18:23.000Z
Datetime Validation in TypeScript
Validating datetime inputs is an important task in any application. TypeScript provides built-in methods to perform datetime validation. One such method is isNaN()
, which can be used to check if a given date is valid or not. Here is an example:
const invalidDate: Date = new Date('2021-13-01'); console.log(isNaN(invalidDate.getTime())); // Output: true const validDate: Date = new Date('2021-10-31'); console.log(isNaN(validDate.getTime())); // Output: false
In the above example, the getTime()
method is used to get the numeric value representing the date. If the value is NaN
, it means the date is invalid.
Related Article: Tutorial on TypeScript Dynamic Object Manipulation
Datetime Conversion in TypeScript
In TypeScript, we can convert a Date
object to different formats using various methods provided by the Date
class. One common conversion is to convert a Date
object to a timestamp, which represents the number of milliseconds since January 1, 1970. We can use the getTime()
method to achieve this. Here is an example:
const currentDate: Date = new Date(); const timestamp: number = currentDate.getTime(); console.log(timestamp); // Output: 1635677895000
To convert a timestamp back to a Date
object, we can use the Date
constructor and pass the timestamp as an argument. Here is an example:
const timestamp: number = 1635677895000; const convertedDate: Date = new Date(timestamp); console.log(convertedDate); // Output: Sun Oct 31 2021 15:48:15 GMT+0530 (India Standard Time)
Datetime Manipulation in TypeScript
Manipulating datetime values is a common requirement in many applications. TypeScript provides several methods to manipulate Date
objects. One such method is setFullYear()
, which allows us to set the year of a Date
object. Here is an example:
const currentDate: Date = new Date(); currentDate.setFullYear(2022); console.log(currentDate); // Output: Wed Oct 31 2022 15:48:15 GMT+0530 (India Standard Time)
Similarly, we can use methods like setMonth()
, setDate()
, setHours()
, setMinutes()
, setSeconds()
, and setMilliseconds()
to manipulate other components of a Date
object.
Datetime Library in TypeScript
While TypeScript provides built-in support for working with Date
objects, there are also several third-party libraries available that offer additional functionality and convenience. One popular library is moment.js
, which provides a simple and flexible API for parsing, manipulating, and formatting dates. Here is an example of using moment.js
in TypeScript:
First, install the moment.js
library using npm:
npm install moment
Then, import the library in your TypeScript file:
import * as moment from 'moment'; const currentDate: moment.Moment = moment(); console.log(currentDate.format('YYYY-MM-DD')); // Output: 2021-10-31
In the above example, we create a moment
object using the moment()
function and then format it using the format()
method.
Related Article: Tutorial: Checking Enum Value Existence in TypeScript
Datetime Comparison in TypeScript
Comparing datetime values is often required in applications. TypeScript provides several methods to compare Date
objects. One such method is getTime()
, which returns the numeric value representing the date. We can use this value to compare two Date
objects. Here is an example:
const date1: Date = new Date('2021-10-31'); const date2: Date = new Date('2022-01-01'); if (date1.getTime() < date2.getTime()) { console.log('date1 is before date2'); } else if (date1.getTime() > date2.getTime()) { console.log('date1 is after date2'); } else { console.log('date1 and date2 are equal'); }
In the example above, we use the getTime()
method to get the numeric value representing each date and then compare them using the <
and >
operators.
Datetime Parsing in TypeScript
Parsing datetime strings is a common task when working with dates and times. TypeScript provides the Date
constructor, which can parse a wide range of date and time formats. Here is an example:
const dateString: string = '2021-10-31T15:48:15.000Z'; const parsedDate: Date = new Date(dateString); console.log(parsedDate); // Output: Sun Oct 31 2021 21:18:15 GMT+0530 (India Standard Time)
In the above example, we pass the datetime string to the Date
constructor, which automatically parses it and creates a Date
object.
Datetime Arithmetic in TypeScript
Performing arithmetic operations with datetime values is often required in applications. TypeScript provides several methods to perform arithmetic operations on Date
objects. One such method is getTime()
, which returns the numeric value representing the date. We can use this value to perform arithmetic operations. Here is an example:
const currentDate: Date = new Date(); const futureDate: Date = new Date(currentDate.getTime() + 24 * 60 * 60 * 1000); // Add one day (24 hours) to the current date console.log(futureDate); // Output: Mon Nov 01 2021 15:48:15 GMT+0530 (India Standard Time)
In the example above, we add one day (24 hours) to the current date by adding the number of milliseconds in one day to the current timestamp.
Related Article: Tutorial on Exact Type in TypeScript
Defining a Date Type in TypeScript
In TypeScript, we can define our own custom types to represent dates. This can be useful when we want to add additional properties or methods to the Date
type. Here is an example:
type CustomDate = { date: Date; format: () => string; }; const currentDate: CustomDate = { date: new Date(), format: function () { return this.date.toLocaleString(); }, }; console.log(currentDate.format()); // Output: 10/31/2021, 3:48:15 PM
In the example above, we define a CustomDate
type with properties date
of type Date
and format
of type function. We then create an object of type CustomDate
and use the format
method to format the date.
Syntax for Specifying a Time Type in TypeScript
As mentioned earlier, TypeScript does not have a separate Time
type. The Date
type represents both the date and time. If you want to specify a specific time without the date component, you can create a Date
object with a specific date and then set the year, month, and day to a fixed value, such as 1970-01-01. Here is an example:
const specificTime: Date = new Date(1970, 0, 1, 15, 30, 0, 0); console.log(specificTime); // Output: Thu Jan 01 1970 15:30:00 GMT+0530 (India Standard Time)
In the example above, we set the year, month, and day to 1970-01-01 and then set the hour, minute, and second to the desired time.
Formatting a Datetime Value in TypeScript
Formatting a datetime value is often required when displaying dates and times in a specific format. TypeScript provides several methods to format Date
objects. One common method is toLocaleString()
, which returns a string representing the date and time using the current locale of the user’s browser. We can also use the toLocaleDateString()
and toLocaleTimeString()
methods to format only the date or time component respectively. Here is an example:
const currentDate: Date = new Date(); const formattedDateTime: string = currentDate.toLocaleString(); console.log(formattedDateTime); // Output: 10/31/2021, 3:48:15 PM const formattedDate: string = currentDate.toLocaleDateString(); console.log(formattedDate); // Output: 10/31/2021 const formattedTime: string = currentDate.toLocaleTimeString(); console.log(formattedTime); // Output: 3:48:15 PM
In the example above, we use the toLocaleString()
, toLocaleDateString()
, and toLocaleTimeString()
methods to format the current date and time.
Related Article: How to Convert Strings to Booleans in TypeScript
Validating a Datetime Input in TypeScript
Validating a datetime input is important to ensure that the user provides a valid date and time. TypeScript provides built-in methods to perform datetime validation. One such method is isNaN()
, which can be used to check if a given date is valid or not. We can also use regular expressions to validate datetime inputs. Here is an example:
function validateDatetimeInput(datetime: string): boolean { const regex: RegExp = /^\d{4}-\d{2}-\d{2}T\d{2}:\d{2}:\d{2}.\d{3}Z$/; return regex.test(datetime); } const datetime1: string = '2021-10-31T15:48:15.000Z'; console.log(validateDatetimeInput(datetime1)); // Output: true const datetime2: string = '2021-13-01T15:48:15.000Z'; console.log(validateDatetimeInput(datetime2)); // Output: false
In the example above, we define a validateDatetimeInput
function that uses a regular expression to validate datetime inputs.
Manipulating Datetime Values in TypeScript
Manipulating datetime values is often required in applications. TypeScript provides several methods to manipulate Date
objects. One such method is setFullYear()
, which allows us to set the year of a Date
object. We can also use methods like setMonth()
, setDate()
, setHours()
, setMinutes()
, setSeconds()
, and setMilliseconds()
to manipulate other components of a Date
object. Here is an example:
const currentDate: Date = new Date(); currentDate.setFullYear(2022); currentDate.setMonth(0); currentDate.setDate(1); currentDate.setHours(0); currentDate.setMinutes(0); currentDate.setSeconds(0); currentDate.setMilliseconds(0); console.log(currentDate); // Output: Sat Jan 01 2022 00:00:00 GMT+0530 (India Standard Time)
In the example above, we set the year, month, date, hours, minutes, seconds, and milliseconds components of the Date
object to specific values.
Recommended Datetime Libraries for TypeScript
While TypeScript provides built-in support for working with Date
objects, there are also several third-party libraries available that offer additional functionality and convenience. Some popular datetime libraries for TypeScript include:
1. Luxon: Luxon provides an easy-to-use and useful API for working with dates and times. It offers features like parsing, formatting, manipulation, and localization. You can find more information and examples in the official documentation: Luxon Documentation.
2. date-fns: date-fns is a lightweight and modular library for working with dates. It provides a wide range of functions for parsing, formatting, manipulation, and localization. You can find more information and examples in the official documentation: date-fns Documentation.
3. Day.js: Day.js is a fast and minimalist library for parsing, formatting, and manipulating dates. It has a small footprint and is compatible with most modern browsers. You can find more information and examples in the official documentation: Day.js Documentation.
These libraries offer various features and capabilities, so choose the one that best suits your needs and project requirements.
Related Article: How to Implement ETL Processes with TypeScript
Comparing Two Datetime Values in TypeScript
Comparing datetime values is often required in applications. TypeScript provides several methods to compare Date
objects. One such method is getTime()
, which returns the numeric value representing the date. We can use this value to compare two Date
objects. Here is an example:
const date1: Date = new Date('2021-10-31'); const date2: Date = new Date('2022-01-01'); if (date1.getTime() < date2.getTime()) { console.log('date1 is before date2'); } else if (date1.getTime() > date2.getTime()) { console.log('date1 is after date2'); } else { console.log('date1 and date2 are equal'); }
In the example above, we use the getTime()
method to get the numeric value representing each date and then compare them using the <
and >
operators.
Parsing a Datetime String in TypeScript
Parsing datetime strings is a common task when working with dates and times. TypeScript provides the Date
constructor, which can parse a wide range of date and time formats. Here is an example:
const dateString: string = '2021-10-31T15:48:15.000Z'; const parsedDate: Date = new Date(dateString); console.log(parsedDate); // Output: Sun Oct 31 2021 21:18:15 GMT+0530 (India Standard Time)
In the above example, we pass the datetime string to the Date
constructor, which automatically parses it and creates a Date
object.
Performing Arithmetic Operations with Datetime Values in TypeScript
Performing arithmetic operations with datetime values is often required in applications. TypeScript provides several methods to perform arithmetic operations on Date
objects. One such method is getTime()
, which returns the numeric value representing the date. We can use this value to perform arithmetic operations. Here is an example:
const currentDate: Date = new Date(); const futureDate: Date = new Date(currentDate.getTime() + 24 * 60 * 60 * 1000); // Add one day (24 hours) to the current date console.log(futureDate); // Output: Mon Nov 01 2021 15:48:15 GMT+0530 (India Standard Time)
In the example above, we add one day (24 hours) to the current date by adding the number of milliseconds in one day to the current timestamp.
Related Article: Building a Rules Engine with TypeScript
TypeScript Decorators for Datetime Handling
TypeScript decorators are a useful feature that allows us to add metadata to classes, properties, and methods, enabling us to enhance their behavior or add additional functionality. While decorators are not specifically designed for datetime handling, we can create custom decorators to handle datetime-related tasks in TypeScript. Here is an example of a custom decorator for formatting datetime values:
function formatDatetime(target: any, propertyKey: string) { const originalValue = target[propertyKey]; const getter = function () { const datetime: Date = originalValue.call(this); return datetime.toLocaleString(); }; Object.defineProperty(target, propertyKey, { get: getter, }); } class Example { @formatDatetime getCurrentDatetime(): Date { return new Date(); } } const example = new Example(); console.log(example.getCurrentDatetime()); // Output: 10/31/2021, 3:48:15 PM
In the example above, we define a formatDatetime
decorator that wraps the getter function of the getCurrentDatetime
method. Inside the decorator, we get the original datetime value, format it using the toLocaleString()
method, and return the formatted value.
External Sources
– Luxon Documentation
– date-fns Documentation
– Day.js Documentation