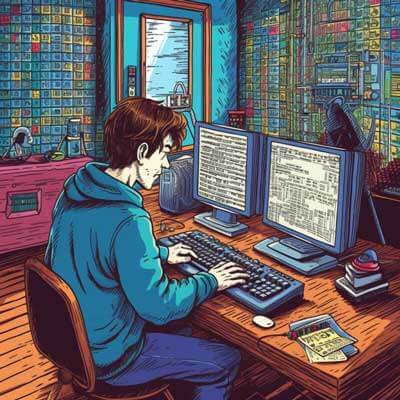
Table of Contents
Introduction to Redis
Redis is an open-source, in-memory data structure store that can be used as a database, cache, and message broker. It is known for its high performance and versatility, making it a popular choice for various applications. In this chapter, we will explore the basics of Redis and its key features.
Related Article: Tutorial: Redis vs RabbitMQ Comparison
Key Features of Redis
- Redis supports various data types, including strings, hashes, lists, sets, and sorted sets.
- It provides atomic operations, allowing multiple operations to be executed as a single unit.
- Redis offers persistence options, allowing data to be stored on disk for durability.
- It supports replication and clustering, enabling high availability and scalability.
- Redis has built-in support for pub/sub messaging, allowing messages to be published and subscribed to by clients.
Example: Storing and Retrieving Data from Redis
// Storing data in Redis $redis->set('name', 'John'); $redis->set('age', 25); // Retrieving data from Redis $name = $redis->get('name'); $age = $redis->get('age'); echo "Name: $name, Age: $age";
Example: Using Redis for Counting Page Views
// Incrementing page views in Redis $redis->incr('page_views'); // Retrieving page views from Redis $pageViews = $redis->get('page_views'); echo "Total Page Views: $pageViews";
Related Article: Tutorial on Rust Redis: Tools and Techniques
Setting up Redis in a Laravel app
In this chapter, we will learn how to set up Redis in a Laravel application. Laravel provides excellent support for Redis out of the box, making it easy to integrate Redis into your application.
Setting up Redis Configuration
To start using Redis in Laravel, you need to configure your Redis connection in the config/database.php
file. Update the redis
section with the appropriate connection details:
'redis' => [ 'client' => 'predis', 'default' => [ 'host' => env('REDIS_HOST', '127.0.0.1'), 'password' => env('REDIS_PASSWORD', null), 'port' => env('REDIS_PORT', 6379), 'database' => env('REDIS_DB', 0), ], ],
Using Redis in Laravel Controllers
Once the Redis configuration is set up, you can start using Redis in your Laravel controllers. Laravel provides a convenient Redis facade for interacting with Redis.
use Illuminate\Support\Facades\Redis; class UserController extends Controller { public function show($id) { $user = Redis::get('user:' . $id); // Rest of the code... } }
Connecting Laravel with Redis
In this chapter, we will explore how to connect Laravel with Redis using the Redis facade. We will cover the basic operations such as storing and retrieving data from Redis.
Related Article: Redis Tutorial: How to Use Redis
Storing Data in Redis
To store data in Redis, you can use the set
method of the Redis facade. The set
method allows you to specify the key and value for the data you want to store.
use Illuminate\Support\Facades\Redis; Redis::set('name', 'John');
Retrieving Data from Redis
To retrieve data from Redis, you can use the get
method of the Redis facade. The get
method allows you to retrieve the value associated with a specific key.
use Illuminate\Support\Facades\Redis; $name = Redis::get('name');
Caching with Redis
Redis is widely used for caching in Laravel applications. In this chapter, we will explore how to leverage Redis as a cache store in Laravel and improve the performance of your application.
Configuring Redis as the Cache Store
To configure Redis as the cache store in Laravel, update the config/cache.php
file. Set the driver
option to redis
and specify your Redis connection details.
'default' => env('CACHE_DRIVER', 'redis'), 'redis' => [ 'driver' => 'redis', 'connection' => 'default', ],
Related Article: Tutorial on Installing and Using redis-cli with Redis
Using Redis for Caching
Once Redis is configured as the cache store, you can start using it for caching in Laravel. Laravel provides a simple and intuitive API for caching.
use Illuminate\Support\Facades\Cache; // Caching a value Cache::put('name', 'John', 60); // Retrieving a cached value $name = Cache::get('name');
Session Management with Redis
Redis can also be used as the session driver in Laravel, offering a scalable and efficient solution for managing sessions. In this chapter, we will explore how to configure Laravel to use Redis as the session driver.
Configuring Redis as the Session Driver
To configure Laravel to use Redis as the session driver, update the config/session.php
file. Set the driver
option to redis
and specify your Redis connection details.
'driver' => env('SESSION_DRIVER', 'redis'), 'connection' => env('SESSION_CONNECTION', 'default'),
Using Redis for Session Management
Once Redis is configured as the session driver, Laravel will automatically handle session management using Redis. You can access session data using the session
helper or the Request
instance.
// Storing data in the session session(['name' => 'John']); // Retrieving data from the session $name = session('name');
Related Article: Tutorial: Kafka vs Redis
Using Redis for Queuing
Redis provides a useful and efficient queuing system that can be easily integrated into Laravel applications. In this chapter, we will explore how to use Redis for queuing jobs in Laravel.
Configuring Redis as the Queue Driver
To configure Redis as the queue driver in Laravel, update the config/queue.php
file. Set the default
option to redis
and specify your Redis connection details.
'default' => env('QUEUE_CONNECTION', 'redis'), 'connections' => [ 'redis' => [ 'driver' => 'redis', 'connection' => 'default', 'queue' => env('REDIS_QUEUE', 'default'), 'retry_after' => 90, 'block_for' => null, ], ],
Using Redis for Queuing Jobs
Once Redis is configured as the queue driver, you can start using it for queuing jobs in Laravel. Laravel provides a simple and expressive API for working with queues.
use Illuminate\Support\Facades\Queue; use App\Jobs\ProcessEmail; // Dispatching a job to the Redis queue Queue::push(new ProcessEmail($email)); // Running the queue worker php artisan queue:work
Real-world use case: Building a chat application with Laravel and Redis
In this chapter, we will explore a real-world use case of using Laravel and Redis to build a chat application. We will cover the architecture, implementation, and key features of the chat application.
Related Article: Tutorial on installing and using redis-cli in Redis
Architecture Overview
The chat application will use Laravel for the backend and Redis for real-time messaging. The frontend will be implemented using JavaScript frameworks such as Vue.js or React.js.
Implementing Real-time Messaging with Redis
To implement real-time messaging with Redis, we can leverage the pub/sub feature of Redis. The backend will publish messages to specific channels, and the frontend will subscribe to these channels to receive real-time updates.
Key Features of the Chat Application
- User authentication and authorization
- Real-time messaging using Redis pub/sub
- Message persistence using Redis
- Typing indicators and read receipts
- Message history and search functionality
Best practices for Redis integration
In this chapter, we will discuss some best practices for integrating Redis into your Laravel application. Following these best practices will help you optimize performance, ensure data integrity, and prevent common pitfalls.
Related Article: Tutorial on Redis Sharding Implementation
Use Appropriate Data Structures
Choose the right data structure in Redis based on your use case. For example, use hashes for storing objects, sets for storing unique values, and sorted sets for ranking data.
Handle Errors Gracefully
Ensure that your code gracefully handles errors when interacting with Redis. Use try-catch blocks to catch and handle exceptions, and implement appropriate error handling strategies.
Limit the Size of Stored Data
Be mindful of the size of data you store in Redis. Storing large amounts of data can impact performance and memory usage. Consider using data compression or pagination techniques if needed.
Handling Errors when using Redis
In this chapter, we will discuss common errors that can occur when using Redis and how to handle them effectively in your Laravel application.
Related Article: How to Configure a Redis Cluster
Connection Errors
Connection errors can occur when the Redis server is not accessible or when there is a network issue. To handle connection errors, you can use try-catch blocks and implement appropriate error handling logic.
Key Not Found Errors
When retrieving data from Redis, there might be cases where the requested key does not exist. To handle key not found errors, you can check the return value of the get
method and handle the case accordingly.
Optimizing Performance with Redis
In this chapter, we will explore techniques for optimizing performance when using Redis in your Laravel application. By following these techniques, you can improve the overall performance and responsiveness of your application.
Use Pipelining
Pipelining is a technique that allows you to send multiple commands to Redis in a single network call, reducing network overhead. Use the pipeline
method of the Redis facade to execute multiple commands efficiently.
use Illuminate\Support\Facades\Redis; $redis = Redis::pipeline(); $redis->set('name', 'John'); $redis->get('name'); $results = $redis->execute();
Related Article: Tutorial on Configuring a Redis Cluster
Implement Lua Scripting
Lua scripting allows you to execute complex operations on the Redis server, reducing the number of round trips between the client and server. Use the eval
method of the Redis facade to execute Lua scripts.
use Illuminate\Support\Facades\Redis; $script = " local name = redis.call('get', KEYS[1]) return 'Hello, ' .. name "; $result = Redis::eval($script, 1, 'name');
Performance consideration: Scaling Redis
In this chapter, we will discuss considerations for scaling Redis in your Laravel application. Scaling Redis involves distributing the workload across multiple Redis instances to handle increased traffic and improve performance.
Replication
Redis supports replication, allowing you to create one or more slave instances that replicate data from a master instance. Replication helps distribute the read workload and provides fault tolerance.
Clustering
Redis clustering allows you to distribute data across multiple Redis instances, providing horizontal scalability. Clustering automatically handles data partitioning and ensures high availability.
Related Article: Redis Intro & Redis Alternatives
Performance consideration: Choosing the right data structures in Redis
In this chapter, we will discuss the importance of choosing the right data structures in Redis to optimize performance. Different data structures have different performance characteristics, and choosing the appropriate one can significantly impact the performance of your application.
Strings
Strings are the simplest data type in Redis and are often used to store simple data such as user preferences or cache values. They have constant-time complexity for read and write operations.
Hashes
Hashes are useful for storing and retrieving complex objects in Redis. They provide constant-time complexity for individual field access, making them suitable for scenarios where you need to access specific fields frequently.
Lists
Lists are ideal for scenarios that require maintaining an ordered collection of elements. They provide constant-time complexity for inserting and deleting elements at both ends of the list.
Related Article: Tutorial on Redis Docker Compose
Advanced technique: Pub/Sub messaging with Redis
In this chapter, we will explore the advanced technique of pub/sub messaging with Redis. Pub/sub allows multiple clients to subscribe to channels and receive messages published to those channels in real-time.
Publishing Messages
To publish a message to a specific channel, you can use the publish
method of the Redis facade. Specify the channel and the message as arguments to the publish
method.
use Illuminate\Support\Facades\Redis; Redis::publish('chat', 'Hello, world!');
Subscribing to Channels
To subscribe to a channel and receive messages in real-time, you can use the subscribe
method of the Redis facade. Provide a callback function to handle the received messages.
use Illuminate\Support\Facades\Redis; Redis::subscribe(['chat'], function ($message) { echo "Received message: $message"; });
Advanced technique: Lua scripting in Redis
In this chapter, we will explore the advanced technique of Lua scripting in Redis. Lua scripting allows you to execute complex operations on the Redis server, providing flexibility and performance benefits.
Related Article: Tutorial on AWS Elasticache Redis Implementation
Writing Lua Scripts
Lua scripts in Redis are executed atomically, ensuring consistency and preventing race conditions. You can write Lua scripts directly in your PHP code using the eval
method of the Redis facade.
use Illuminate\Support\Facades\Redis; $script = " local name = redis.call('get', KEYS[1]) return 'Hello, ' .. name "; $result = Redis::eval($script, 1, 'name');
Advanced technique: Redis pipelines
In this chapter, we will explore the advanced technique of Redis pipelines. Pipelines allow you to send multiple commands to Redis in a single network call, significantly improving performance when executing multiple commands sequentially.
Using Pipelines
To use pipelines in Laravel, you can use the pipeline
method of the Redis facade. The pipeline
method returns an instance of the Pipeline
class, which allows you to queue multiple commands and execute them efficiently.
use Illuminate\Support\Facades\Redis; $redis = Redis::pipeline(); $redis->set('name', 'John'); $redis->get('name'); $results = $redis->execute();
Code Snippet: Storing and retrieving data from Redis
use Illuminate\Support\Facades\Redis; // Storing data in Redis Redis::set('name', 'John'); // Retrieving data from Redis $name = Redis::get('name'); echo "Name: $name";
Related Article: Tutorial: Setting Up Redis Using Docker Compose
Code Snippet: Implementing caching with Redis
use Illuminate\Support\Facades\Cache; // Caching a value Cache::put('name', 'John', 60); // Retrieving a cached value $name = Cache::get('name'); echo "Name: $name";
Code Snippet: Using Redis for queuing jobs
use Illuminate\Support\Facades\Queue; use App\Jobs\ProcessEmail; // Dispatching a job to the Redis queue Queue::push(new ProcessEmail($email)); // Running the queue worker php artisan queue:work
Code Snippet: Subscribing to Redis channels
use Illuminate\Support\Facades\Redis; Redis::subscribe(['chat'], function ($message) { echo "Received message: $message"; });
Code Snippet: Executing Lua scripts in Redis
use Illuminate\Support\Facades\Redis; $script = " local name = redis.call('get', KEYS[1]) return 'Hello, ' .. name "; $result = Redis::eval($script, 1, 'name'); echo "Result: $result";