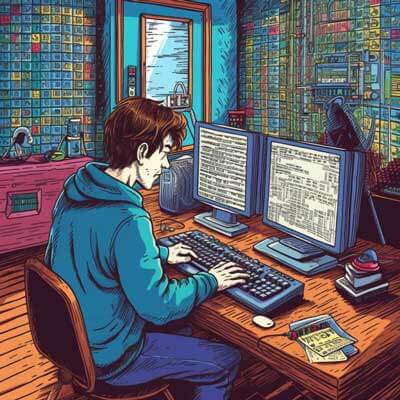
- Introduction to Redis
- Installation and Setup
- Basic Data Types and Operations
- Strings
- Lists
- Set Commands and Functions
- Hash Commands and Functions
- Sorted Set Commands and Functions
- Key Management and Expiration
- Pub/Sub Messaging
- Transactions in Redis
- Lua Scripting
- Redis in High Availability Environments
- Scaling Redis
- Redis Cluster
- Redis Best Practices
- Use Case: Caching with Redis
- Use Case: Real-time Analytics with Redis
- Use Case: Leaderboards with Redis
- Real World Example: Building a Chat Application
- Real World Example: Implementing a Task Queue
- Real World Example: Rate Limiting
- Performance Consideration: Memory Optimization
- Performance Consideration: Scaling Read Operations
- Performance Consideration: Handling Write Operations
- Performance Consideration: Redis Persistence
- Advanced Technique: Redis Streams
- Advanced Technique: Redis Modules
- Advanced Technique: Redis Pipelining
- Code Snippet: Atomic Operations
- Code Snippet: Lua Scripting Examples
- Code Snippet: Working with Redis Sets
- Error Handling and Debugging
Introduction to Redis
Redis is an open-source, in-memory data structure store that can be used as a database, cache, and message broker. It is known for its high performance and versatility, making it a popular choice for various use cases. Redis supports a wide range of data types and provides efficient operations for manipulating and retrieving data. In this chapter, we will explore the fundamental concepts of Redis and how it can be used to solve real-world problems.
Related Article: Tutorial: Setting Up Redis Using Docker Compose
Installation and Setup
To get started with Redis, you need to install it on your system. The installation process may vary depending on your operating system. Here, we will cover the general steps for setting up Redis on a Linux machine:
1. Download the latest stable release of Redis from the official website or use a package manager like apt or yum.
2. Extract the downloaded file and navigate to the extracted directory.
3. Compile Redis using the make command.
4. After the compilation is complete, run the redis-server command to start the Redis server.
5. You can test if Redis is running by executing the redis-cli command in a separate terminal window. This will open the Redis command-line interface.
6. You are now ready to interact with Redis and perform various operations.
Basic Data Types and Operations
Redis supports several data types, including strings, lists, sets, hashes, sorted sets, and more. Each data type has its own set of operations for manipulating and retrieving data. Let’s explore some of the basic data types and their operations:
Strings
Strings in Redis are binary-safe and can hold any type of data, such as text, JSON, or binary data. Here are some examples of string operations:
# Set a string value SET mykey "Hello Redis" # Get the value of a key GET mykey # Increment a numeric value INCR mycounter # Append a value to an existing string APPEND mykey " World"
Related Article: Tutorial: Redis vs RabbitMQ Comparison
Lists
Lists in Redis are ordered collections of strings. They can be used to implement queues, stacks, and more. Here are some examples of list operations:
# Push elements to the head of a list LPUSH mylist "element1" LPUSH mylist "element2" # Push elements to the tail of a list RPUSH mylist "element3" RPUSH mylist "element4" # Get the length of a list LLEN mylist # Get elements from a list LRANGE mylist 0 -1
Set Commands and Functions
Sets in Redis are unordered collections of unique strings. They provide efficient operations for membership testing and set operations such as union, intersection, and difference. Here are some examples of set operations:
# Add elements to a set SADD myset "element1" SADD myset "element2" # Check if an element exists in a set SISMEMBER myset "element1" # Get all elements in a set SMEMBERS myset # Perform set operations SUNION set1 set2 SINTER set1 set2 SDIFF set1 set2
Hash Commands and Functions
Hashes in Redis are field-value pairs, where the field is a string and the value can be any Redis data type. Hashes are useful for representing objects or dictionaries. Here are some examples of hash operations:
# Set field-value pairs in a hash HSET myhash field1 "value1" HSET myhash field2 "value2" # Get the value of a field in a hash HGET myhash field1 # Get all field-value pairs in a hash HGETALL myhash # Increment a numeric field in a hash HINCRBY myhash field1 10
Related Article: Tutorial: Kafka vs Redis
Sorted Set Commands and Functions
Sorted sets in Redis are similar to sets but each element is associated with a score, which is used to order the elements. Sorted sets are useful for implementing leaderboards, rankings, and range queries. Here are some examples of sorted set operations:
# Add elements to a sorted set with scores ZADD leaderboard 100 "player1" ZADD leaderboard 200 "player2" # Get the rank of an element in a sorted set ZRANK leaderboard "player1" # Get the top elements from a sorted set ZREVRANGE leaderboard 0 9 WITHSCORES # Increment the score of an element in a sorted set ZINCRBY leaderboard 50 "player1"
Key Management and Expiration
In Redis, keys are used to identify and store data. It is important to manage keys efficiently and set expiration times for keys when necessary. Redis provides several commands and functions for key management and expiration. Here are some examples:
# Set an expiration time for a key EXPIRE mykey 60 # Get the remaining time to live for a key TTL mykey # Delete a key DEL mykey # Get information about a key TYPE mykey
Pub/Sub Messaging
Redis supports publish/subscribe messaging, allowing clients to subscribe to channels and receive messages published to those channels. This feature is useful for building real-time applications, chat systems, and message queues. Here are some examples of pub/sub operations:
# Subscribe to a channel SUBSCRIBE channel1 # Publish a message to a channel PUBLISH channel1 "Hello, subscribers!" # Unsubscribe from a channel UNSUBSCRIBE channel1
Related Article: Tutorial: Integrating Redis with Spring Boot
Transactions in Redis
Redis provides a simple mechanism for executing a group of commands as a single atomic operation. This ensures that either all commands are executed or none of them is executed. Transactions are useful for maintaining data consistency and integrity. Here is an example of a transaction:
# Start a transaction MULTI # Add commands to the transaction SET key1 "value1" SET key2 "value2" # Execute the transaction EXEC
Lua Scripting
Redis allows you to write Lua scripts and execute them on the server side. Lua scripting provides flexibility and allows you to perform complex operations that are not available through built-in commands. Here is an example of a Lua script:
-- Define the Lua script local result = redis.call('GET', 'mykey') redis.call('SET', 'anotherkey', result) -- Execute the Lua script redis.call('EVAL', 'script', 0)
Redis in High Availability Environments
In high availability environments, it is crucial to ensure that Redis remains available and responsive even in the face of failures. Redis provides features such as replication, sentinel, and cluster to achieve high availability. Here are some considerations for deploying Redis in high availability environments:
– Replication: Redis supports master-slave replication, where a master Redis instance is replicated to one or more slave instances. This provides data redundancy and allows for failover when the master node fails.
– Sentinel: Redis Sentinel is a separate process that monitors the health of Redis instances and performs automatic failover when necessary. It ensures that a new master is elected and the system remains available.
– Cluster: Redis Cluster allows you to distribute data across multiple Redis instances, providing scalability and fault tolerance. It automatically handles node failures and redistributes data.
Related Article: Tutorial: Installing Redis on Ubuntu
Scaling Redis
As the amount of data and traffic increases, you may need to scale Redis to handle the load efficiently. Redis provides several techniques for scaling, depending on your requirements. Here are some approaches for scaling Redis:
– Vertical scaling: Increase the capacity of a single Redis instance by upgrading the hardware or allocating more resources to it. This approach is suitable when the workload can be handled by a single Redis instance.
– Horizontal scaling: Distribute the data across multiple Redis instances using techniques such as sharding or Redis Cluster. This allows you to handle a larger amount of data and traffic by leveraging the resources of multiple instances.
– Caching: Use Redis as a caching layer in front of a database or other backend systems. This can help alleviate the load on the backend and improve overall performance.
Redis Cluster
Redis Cluster is a distributed implementation of Redis that allows you to scale Redis horizontally and achieve high availability. It divides the data into multiple slots and distributes them across multiple Redis instances. Redis Cluster provides automatic sharding, node failure detection, and failover. Here are some key features of Redis Cluster:
– Automatic data sharding: Redis Cluster automatically distributes the data across multiple instances using a hash slot mechanism. Each instance is responsible for a subset of hash slots.
– Node failure detection: Redis Cluster monitors the health of the nodes and detects failures. When a node fails, the cluster redistributes the affected hash slots to other healthy nodes.
– Automatic failover: Redis Cluster provides automatic failover for master nodes. When a master node fails, a new master is elected from the slave nodes. This ensures that the system remains available and responsive.
– Redis Cluster also supports read scalability, allowing you to perform read operations on slave nodes and distribute the workload.
Redis Best Practices
When using Redis, it is important to follow certain best practices to ensure optimal performance, reliability, and security. Here are some recommended best practices for using Redis:
– Use Redis data structures appropriately: Choose the right data structure for your use case to ensure efficient operations and minimize memory usage. Understand the characteristics and limitations of each data type.
– Monitor Redis performance: Regularly monitor Redis performance using tools like Redis Monitoring or Redis Sentinel. Keep an eye on key metrics such as memory usage, throughput, and latency.
– Use Redis persistence: Configure Redis to persist data to disk using either RDB snapshots or AOF logs. This provides data durability and allows for recovery in case of a server restart or crash.
– Secure Redis: Protect your Redis instance by enabling authentication, using SSL/TLS for client-server communication, and configuring proper firewall rules. Regularly update Redis to the latest stable version to benefit from security patches.
Related Article: Tutorial: Installing Redis on Ubuntu
Use Case: Caching with Redis
One of the most common use cases for Redis is caching. Redis provides fast in-memory storage, making it an ideal choice for caching frequently accessed data. Caching with Redis can significantly improve the performance of your application by reducing the load on the backend systems. Here is an example of how to use Redis for caching:
# Check if the data exists in the cache data = redis.get('key') if data is None: # If the data is not in the cache, retrieve it from the backend data = backend.get_data() # Store the data in the cache with an expiration time redis.set('key', data) redis.expire('key', 300) # Set the expiration time to 5 minutes else: # If the data is in the cache, use it directly process_data(data)
Use Case: Real-time Analytics with Redis
Redis can also be used for real-time analytics, where you need to process and analyze large volumes of data in real-time. Redis provides efficient data structures and operations that enable real-time analytics pipelines. Here is an example of how to use Redis for real-time analytics:
# Receive data from a stream or a message queue data = receive_data() # Store the data in a sorted set with a timestamp as the score redis.zadd('analytics', {data: time.time()}) # Perform real-time analytics on the data results = redis.zrangebyscore('analytics', min=timestamp1, max=timestamp2) # Process and display the results process_results(results)
Use Case: Leaderboards with Redis
Redis is well-suited for implementing leaderboards, where you need to maintain rankings and scores for a large number of users. Redis sorted sets provide efficient operations for updating and querying leaderboards. Here is an example of how to implement leaderboards with Redis:
# Add a user and their score to the leaderboard redis.zadd('leaderboard', {'user1': 100}) redis.zadd('leaderboard', {'user2': 200}) # Get the rank and score of a user rank = redis.zrank('leaderboard', 'user1') score = redis.zscore('leaderboard', 'user1') # Get the top users in the leaderboard top_users = redis.zrevrange('leaderboard', start=0, stop=9, withscores=True)
Related Article: Tutorial: Comparing Kafka vs Redis
Real World Example: Building a Chat Application
Let’s consider a real-world example of building a chat application using Redis. Redis can be used to store chat messages, maintain user presence, and facilitate real-time communication. Here are some key components and operations involved in building a chat application with Redis:
1. Storing chat messages: Use Redis lists or sorted sets to store chat messages. Each message can be stored as a JSON object with fields such as sender, recipient, timestamp, and content.
2. User presence: Use Redis sets or sorted sets to track the online status of users. Add users to a set when they log in and remove them when they log out. This allows you to display the online status of users and send messages to online users only.
3. Real-time communication: Use Redis pub/sub messaging to facilitate real-time communication between users. When a user sends a message, publish it to the appropriate channel. Subscribed users will receive the message in real-time.
4. Message history: Use Redis lists or sorted sets to maintain a history of chat messages. You can limit the size of the list or set to store only the most recent messages.
5. Notifications: Use Redis pub/sub or other mechanisms to send notifications to users when they receive new messages or when they are mentioned in a chat.
Real World Example: Implementing a Task Queue
Redis can be used as a task queue to manage and distribute background tasks in a distributed system. Redis provides efficient data structures and operations for implementing task queues. Here are some key components and operations involved in implementing a task queue with Redis:
1. Queueing tasks: Use Redis lists to enqueue tasks. Each task can be represented as a JSON object with fields such as task ID, task type, and task data.
2. Processing tasks: Use multiple worker processes or threads to process tasks from the queue. Workers can use Redis’s atomic operations such as BLPOP or BRPOP to fetch tasks from the queue.
3. Task status and progress: Use Redis hashes to store the status and progress of tasks. Each task can have a corresponding hash with fields such as status, progress, and result.
4. Retrying failed tasks: In case of task failures, you can use Redis’s sorted sets to store failed tasks and their retry timestamps. Workers can periodically check the sorted set and retry failed tasks.
5. Monitoring and reporting: Use Redis’s data structures and operations to collect and aggregate task metrics, such as task durations, success rates, and error rates. This can help monitor and optimize the performance of the task queue.
Real World Example: Rate Limiting
Rate limiting is a common technique used to control the rate at which clients can access a service or API. Redis can be used to implement rate limiting mechanisms efficiently. Here are some key components and operations involved in implementing rate limiting with Redis:
1. Counting requests: Use Redis’s atomic operations such as INCR or HINCRBY to increment a counter for each request from a client. The counter can be stored as a key-value pair, where the key represents the client or the API endpoint.
2. Expiration and reset: Use Redis’s TTL or EXPIRE to set an expiration time for the counter. This allows you to automatically reset the counter after a certain period, effectively limiting the rate of requests.
3. Throttling requests: Use Redis’s counters and expiration mechanism to enforce rate limits. By checking the counter value before processing a request, you can decide whether to allow, throttle, or reject the request based on the desired rate limit.
4. Distributed rate limiting: Redis can be used in a distributed environment to implement rate limiting across multiple servers or instances. Each server can have its own counters, and a central Redis instance can be used for synchronization and coordination.
Related Article: Tutorial on Rust Redis: Tools and Techniques
Performance Consideration: Memory Optimization
Redis stores data primarily in memory, which makes memory optimization crucial for performance and cost efficiency. Here are some techniques for optimizing memory usage in Redis:
– Compression: Redis provides built-in compression for string values larger than a certain threshold. Use the COMPRESS command to enable compression and reduce memory usage for large strings.
– Data structure optimization: Choose the most appropriate data structure for your use case to minimize memory usage. For example, use Redis hashes instead of separate keys for storing object fields.
– Expire keys: Set appropriate expiration times for keys to ensure that unused data is automatically evicted from memory. This helps free up memory and prevent memory exhaustion.
– Memory eviction policies: Configure Redis to use appropriate memory eviction policies such as LRU (Least Recently Used) or LFU (Least Frequently Used) based on your use case. These policies ensure that the most frequently accessed data remains in memory.
– Memory fragmentation: Monitor memory fragmentation in Redis and take appropriate actions to prevent it. Fragmentation can affect memory utilization and performance. Use the MEMORY FRAGMENTATION command to check memory fragmentation levels.
Performance Consideration: Scaling Read Operations
As the load on your Redis instance increases, you may need to scale the read operations to handle the increased traffic. Here are some techniques for scaling read operations in Redis:
– Replication: Use Redis replication to create read replicas of your master instance. Replicas can handle read operations, allowing you to distribute the read load across multiple instances.
– Caching: Use Redis as a cache in front of a database or other backend systems. By caching frequently accessed data, you can reduce the load on the backend and improve read performance.
– Sharding: If your data set is too large to fit in a single Redis instance, you can shard the data across multiple instances. Each instance will be responsible for a subset of the data, allowing you to distribute the read load.
– Load balancing: Use load balancers to distribute read requests across multiple Redis instances. This ensures that the read load is evenly distributed and no single instance is overwhelmed.
Performance Consideration: Handling Write Operations
Handling write operations efficiently is crucial for the performance and scalability of your Redis deployment. Here are some techniques for handling write operations in Redis:
– Pipelining: Use Redis pipelining to send multiple commands to the server in a single round trip. This reduces the network latency and improves the throughput of write operations.
– Lua scripting: Use Redis Lua scripting to bundle multiple write commands into a single atomic operation. This ensures that either all commands are executed or none of them is executed, maintaining data consistency.
– Asynchronous writes: If immediate consistency is not a requirement, you can use asynchronous writes to improve the performance of write operations. Instead of waiting for the write to be completed, you can acknowledge the write and process it in the background.
– Sharding: If your write load is too high for a single Redis instance, you can shard the data across multiple instances. Each instance will be responsible for a subset of the data, allowing you to distribute the write load.
Related Article: Tutorial on Redis Sharding Implementation
Performance Consideration: Redis Persistence
Redis provides two mechanisms for persistence: RDB snapshots and AOF logs. Persistence is important for data durability and recovery in case of server restarts or crashes. Here are some considerations for Redis persistence:
– RDB snapshots: RDB snapshots are point-in-time snapshots of the Redis dataset. They are stored as binary files on disk. RDB snapshots are efficient for full backups and can be used for disaster recovery.
– AOF logs: AOF (Append-Only File) logs store a log of write operations in a human-readable format. AOF logs can be used to replay the write operations and restore the dataset. They provide better durability than RDB snapshots, but at the cost of increased disk space usage and slower recovery time.
– Persistence frequency: Configure the frequency of RDB snapshots and AOF log rewrites based on your durability and recovery requirements. More frequent snapshots and rewrites provide better durability but can impact performance.
– Asynchronous persistence: Redis supports asynchronous persistence, where write operations are acknowledged before they are persisted to disk. This improves the performance of write operations by reducing disk I/O latency. However, it introduces a risk of data loss in case of a server crash before the data is persisted.
Advanced Technique: Redis Streams
Redis Streams is a data structure designed for handling streams of data, such as event streams or log streams. Streams provide features such as persistence, ordering, and retention of messages. Here are some key features and operations related to Redis Streams:
– Stream data structure: A stream is essentially a log-like data structure with an ordered sequence of entries. Each entry in the stream represents an event or a message and has an associated unique ID.
– Publishing to a stream: To publish a message to a stream, you append it to the stream with an ID. Redis automatically assigns a unique ID to each message.
– Consuming from a stream: Consumers can read messages from a stream using the XREAD command. Messages can be read in blocking or non-blocking mode, and multiple consumers can read from the same stream concurrently.
– Consumer groups: Redis Streams support consumer groups, which allow multiple consumers to consume messages from a stream in a coordinated manner. Each consumer group maintains its own offset in the stream, ensuring that every message is processed by at least one consumer in the group.
– Retention and pruning: Redis Streams provide features for automatic retention and pruning of old messages. You can set a maximum length or a maximum time for the stream, and Redis will automatically remove old messages when the limit is reached.
Advanced Technique: Redis Modules
Redis Modules are extensions to Redis that provide additional functionality beyond the core Redis features. Modules can be developed by the Redis community or by third-party developers. Here are some examples of Redis Modules:
– RediSearch: RediSearch is a full-text search module for Redis. It provides useful search capabilities such as fuzzy search, stemming, and numeric filtering.
– RedisGears: RedisGears is a distributed data processing framework for Redis. It allows you to perform complex data processing and analysis using Python or JavaScript scripts.
– ReJSON: ReJSON is a module that adds support for JSON data to Redis. It allows you to store, query, and manipulate JSON documents directly in Redis.
– RedisGraph: RedisGraph is a graph database module for Redis. It allows you to store and query graph data using the Cypher query language.
– RedisBloom: RedisBloom is a module that provides probabilistic data structures such as Bloom filters, Count-Min Sketch, and Top-K. These data structures are useful for tasks such as set membership testing and approximate counting.
Related Article: Tutorial on Redis Sentinel: A Deep Look
Advanced Technique: Redis Pipelining
Redis Pipelining is a technique that allows you to send multiple commands to the Redis server in a single network round trip. This can significantly improve the throughput and latency of Redis operations, especially when dealing with multiple small commands. Here is an example of how to use Redis Pipelining:
# Start a pipeline pipe = redis.pipeline() # Add multiple commands to the pipeline pipe.set('key1', 'value1') pipe.get('key2') pipe.incr('key3') # Execute the pipeline and retrieve the results result1, result2, result3 = pipe.execute()
Code Snippet: Atomic Operations
Redis provides atomic operations, which means that a command is executed as a single isolated operation without interference from other clients or commands. Here is an example of an atomic operation in Redis:
# Increment a counter atomically redis.incr('counter')
Code Snippet: Lua Scripting Examples
Redis Lua scripting allows you to execute complex operations on the server side. Here are some examples of Lua scripting in Redis:
-- Example 1: Set multiple keys with a single command redis.call('MSET', 'key1', 'value1', 'key2', 'value2') -- Example 2: Retrieve multiple keys with a single command return redis.call('MGET', 'key1', 'key2')
Related Article: Tutorial on Redis Queue Implementation
Code Snippet: Working with Redis Sets
Redis sets provide efficient operations for storing and manipulating collections of unique elements. Here are some examples of working with Redis sets:
# Add elements to a set redis.sadd('myset', 'element1') redis.sadd('myset', 'element2') # Check if an element exists in a set redis.sismember('myset', 'element1') # Get all elements in a set redis.smembers('myset')
Error Handling and Debugging
When working with Redis, it is important to handle errors and exceptions properly and have mechanisms in place for debugging and troubleshooting. Here are some tips for error handling and debugging in Redis:
– Use Redis’s error replies: Redis returns specific error replies for certain conditions, such as wrong command syntax or invalid arguments. Make sure to check and handle these error replies appropriately.
– Enable Redis logging: Configure Redis to log important events and errors. The log files can be useful for debugging and troubleshooting issues.
– Monitor Redis metrics: Use tools like Redis Monitoring or Redis Sentinel to monitor key metrics such as memory usage, CPU utilization, and network traffic. This can help identify performance bottlenecks and potential issues.
– Use Redis’s built-in commands for inspection: Redis provides several commands for inspecting the state of the server, such as INFO, KEYS, and CLIENT LIST. These commands can be useful for debugging and troubleshooting.
– Handle network and connection errors: Redis is a network-based service, so it is important to handle network errors and connection failures gracefully. Implement appropriate error handling and retry mechanisms in your client code.