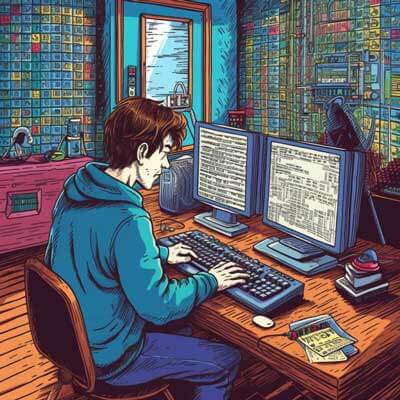
- Introduction to Rust Redis
- Installing and Configuring Redis
- Connecting to Redis Server
- Basic Data Operations
- Setting and Retrieving String Values
- Working with Lists
- Data Structures in Redis
- Working with Hashes
- Working with Sets
- Working with Sorted Sets
- Advanced Data Operations
- Working with Transactions and Pipelining
- Error Handling in Rust Redis
- Performance Considerations: Caching Strategies
- Cache-Aside Pattern
- Cache Invalidation
- Performance Considerations: Pub/Sub
- Publishing Messages
- Subscribing to Channels
- Best Practices for Rust Redis
- Real World Examples: Building a Chat Application
- Real World Examples: Building a Leaderboard
- Real World Examples: Implementing Rate Limiting
- Code Snippet: Working with Hashes
- Code Snippet: Working with Sets
- Code Snippet: Working with Sorted Sets
- Code Snippet: Pub/Sub Messaging
- Advanced Technique: Lua Scripting in Rust Redis
- Advanced Technique: Transactions and Pipelining
- Advanced Technique: Cluster Support in Rust Redis
Introduction to Rust Redis
Rust Redis is a powerful library that provides a native Rust interface for interacting with Redis, a popular in-memory data structure store. Redis is known for its speed and versatility, making it a popular choice for caching, messaging, and data storage in various applications.
With the Rust Redis library, developers can leverage the performance and safety guarantees of Rust while seamlessly integrating with Redis. This tutorial will guide you through the tools and techniques necessary to effectively use Rust Redis in your projects.
Related Article: Tutorial on Installing and Using redis-cli with Redis
Installing and Configuring Redis
Before diving into Rust Redis, you need to install and configure Redis on your system. Here are the steps to get started:
1. Visit the official Redis website (https://redis.io/) and download the latest stable release.
2. Extract the downloaded archive and navigate to the extracted folder using the terminal or command prompt.
3. Run the command make
to compile Redis.
4. Once the compilation is complete, run make test
to ensure that Redis is functioning correctly.
5. Finally, run make install
to install Redis on your system.
After installation, you can start the Redis server by running the redis-server
command. By default, Redis listens on port 6379. You can customize the configuration by modifying the redis.conf
file.
Connecting to Redis Server
To connect to a Redis server from your Rust application, you need to add the redis
crate to your project’s dependencies in the Cargo.toml
file:
[dependencies] redis = "0.20.0"
Once you’ve added the dependency, you can establish a connection to the Redis server using the redis::Client
struct:
use redis::Client; fn main() { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection().unwrap(); // Use the connection to interact with Redis }
In the example above, we create a new Redis client by specifying the server address and port. The get_connection()
method establishes a connection to the server and returns a redis::Connection
object that we can use to perform operations on Redis.
Basic Data Operations
Once you have a connection to the Redis server, you can start performing basic data operations. Here are a few examples:
Related Article: How to Configure a Redis Cluster
Setting and Retrieving String Values
use redis::{Client, Commands}; fn main() { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection().unwrap(); let _: () = connection.set("name", "John").unwrap(); let name: String = connection.get("name").unwrap(); println!("Name: {}", name); }
In the example above, we set a string value “John” with the key “name” using the set()
method. We can retrieve the value using the get()
method.
Working with Lists
use redis::{Client, Commands}; fn main() { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection().unwrap(); let _: () = connection.rpush("fruits", "apple").unwrap(); let _: () = connection.rpush("fruits", "banana").unwrap(); let fruits: Vec<String> = connection.lrange("fruits", 0, -1).unwrap(); println!("Fruits: {:?}", fruits); }
In this example, we use Redis lists to store a collection of fruits. We use the rpush()
method to append items to the list and the lrange()
method to retrieve all items.
These examples cover only a fraction of the basic data operations you can perform with Rust Redis. The library provides a wide range of methods for interacting with Redis’s various data structures, including sets, hashes, and sorted sets.
Data Structures in Redis
Redis offers several data structures that go beyond the basic key-value pair model. Let’s explore some of these data structures and how to work with them using Rust Redis.
Related Article: Redis vs MongoDB: A Detailed Comparison
Working with Hashes
Hashes in Redis are maps between string fields and string values. They are useful for representing objects with multiple properties. Here’s an example of working with hashes in Rust Redis:
use redis::{Client, Commands}; fn main() { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection().unwrap(); let _: () = connection.hset("user:123", "name", "John Doe").unwrap(); let _: () = connection.hset("user:123", "email", "john@example.com").unwrap(); let name: String = connection.hget("user:123", "name").unwrap(); let email: String = connection.hget("user:123", "email").unwrap(); println!("Name: {}", name); println!("Email: {}", email); }
In this example, we use the hset()
method to set the fields and values of a hash named “user:123”. We can then retrieve the field values using the hget()
method.
Working with Sets
Sets in Redis are unordered collections of unique strings. They are useful for storing tags, followers, or any other collection where uniqueness is required. Here’s an example of working with sets in Rust Redis:
use redis::{Client, Commands}; fn main() { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection().unwrap(); let _: () = connection.sadd("tags", "rust").unwrap(); let _: () = connection.sadd("tags", "redis").unwrap(); let _: () = connection.sadd("tags", "tutorial").unwrap(); let tags: Vec<String> = connection.smembers("tags").unwrap(); println!("Tags: {:?}", tags); }
In this example, we use the sadd()
method to add elements to a set named “tags”. We can retrieve all the members of the set using the smembers()
method.
Working with Sorted Sets
Sorted sets in Redis are similar to sets, but each member is associated with a score that allows the set to be ordered. They are useful for implementing leaderboards, rankings, or any other ordered collection. Here’s an example of working with sorted sets in Rust Redis:
use redis::{Client, Commands}; fn main() { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection().unwrap(); let _: () = connection.zadd("leaderboard", 100, "John").unwrap(); let _: () = connection.zadd("leaderboard", 200, "Jane").unwrap(); let _: () = connection.zadd("leaderboard", 150, "Alice").unwrap(); let leaderboard: Vec<String> = connection.zrange("leaderboard", 0, -1).unwrap(); println!("Leaderboard: {:?}", leaderboard); }
In this example, we use the zadd()
method to add members with scores to a sorted set named “leaderboard”. We can retrieve the members in ascending order using the zrange()
method.
These examples should provide you with a good starting point for working with Redis data structures using Rust Redis. Remember to consult the official documentation for more details on the available methods and options.
Related Article: Tutorial on Redis Queue Implementation
Advanced Data Operations
In addition to the basic data operations covered earlier, Rust Redis provides advanced features for manipulating data in Redis. Let’s explore some of these advanced data operations and how to use them in Rust.
Working with Transactions and Pipelining
Redis supports transactions, which allow you to group multiple commands into a single atomic operation. Rust Redis provides a convenient way to work with transactions using the transaction()
method. Here’s an example:
use redis::{Client, Commands, PipelineCommands}; fn main() { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection().unwrap(); let mut transaction = redis::transaction(&connection).unwrap(); transaction.set("name", "John").unwrap(); transaction.set("age", 30).unwrap(); let _: () = transaction.query(()).unwrap(); let name: String = connection.get("name").unwrap(); let age: i32 = connection.get("age").unwrap(); println!("Name: {}", name); println!("Age: {}", age); }
In this example, we create a transaction using the redis::transaction()
method and execute multiple commands within the transaction block. The query()
method is used to execute the transaction and commit the changes.
Pipelining is another advanced technique that allows you to send multiple commands to Redis without waiting for each response. Rust Redis provides a pipe()
method to work with pipelining. Here’s an example:
use redis::{Client, Commands, PipelineCommands}; fn main() { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection().unwrap(); let mut pipeline = redis::pipe(); pipeline.set("name", "John"); pipeline.set("age", 30); let _: () = pipeline.query(&connection).unwrap(); let name: String = connection.get("name").unwrap(); let age: i32 = connection.get("age").unwrap(); println!("Name: {}", name); println!("Age: {}", age); }
In this example, we use the redis::pipe()
method to create a pipeline and add multiple commands to it using the set()
method. The query()
method is used to execute the pipeline and retrieve the results.
These advanced data operations provide powerful ways to manipulate data in Redis. Transactions ensure atomicity and consistency, while pipelining improves performance by reducing round-trips to the server.
Error Handling in Rust Redis
Error handling is an essential aspect of writing robust and reliable code. When working with Rust Redis, it is important to handle errors properly to ensure the stability of your application. Rust Redis uses the redis::RedisResult
type to represent the result of Redis operations, which can be either Ok
or Err
. Here’s an example of error handling in Rust Redis:
use redis::{Client, Commands}; fn main() { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection(); match connection { Ok(connection) => { let _: () = connection.set("name", "John").unwrap(); let name: String = connection.get("name").unwrap(); println!("Name: {}", name); } Err(error) => { eprintln!("Failed to connect to Redis: {}", error); } } }
In this example, we use the match
expression to handle the Result
returned by client.get_connection()
. If the connection is successfully established, we can proceed with the Redis operations. Otherwise, we print an error message.
It is important to handle errors appropriately based on the requirements of your application. You can choose to log errors, retry operations, or gracefully handle failures based on the specific scenario.
Related Article: Tutorial: Installing Redis on Ubuntu
Performance Considerations: Caching Strategies
Caching is a common use case for Redis, and it can significantly improve the performance of your application. Rust Redis provides several strategies for implementing caching. Let’s explore some of these strategies and how to use them effectively.
Cache-Aside Pattern
The cache-aside pattern is a common caching strategy where your application first checks the cache for the requested data. If the data is found in the cache, it is returned to the application. Otherwise, the application retrieves the data from the primary data source, stores it in the cache, and returns it to the application. Here’s an example:
use redis::{Client, Commands}; fn get_data_from_cache_or_source(key: &str) -> Option<String> { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection().unwrap(); let cached_data: Option<String> = connection.get(key).unwrap(); match cached_data { Some(data) => Some(data), None => { let data = fetch_data_from_source(key); let _: () = connection.set(key, &data).unwrap(); Some(data) } } } fn fetch_data_from_source(key: &str) -> String { // Logic to fetch data from the primary data source // ... // Return the fetched data as a string unimplemented!() } fn main() { let key = "my_data"; let data = get_data_from_cache_or_source(key).unwrap(); println!("Data: {}", data); }
In this example, the get_data_from_cache_or_source()
function first checks if the data is available in the cache using the get()
method. If the data is found, it is returned. Otherwise, the function fetches the data from the primary data source using the fetch_data_from_source()
function, stores it in the cache using the set()
method, and returns the data.
Cache Invalidation
Cache invalidation is another important aspect of caching. When data in the primary data source is updated, you need to ensure that the corresponding data in the cache is invalidated to maintain consistency. Rust Redis provides various methods for cache invalidation, including:
– del(key: &str)
: Deletes the specified key from the cache.
– expire(key: &str, seconds: usize)
: Sets a time-to-live (TTL) for the specified key, after which it will be automatically deleted.
Here’s an example of cache invalidation using the del()
method:
use redis::{Client, Commands}; fn invalidate_cache(key: &str) { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection().unwrap(); let _: () = connection.del(key).unwrap(); } fn main() { let key = "my_data"; invalidate_cache(key); println!("Cache invalidated for key: {}", key); }
In this example, the invalidate_cache()
function deletes the specified key from the cache using the del()
method. This ensures that the next time the data is requested, it will be fetched from the primary data source and stored in the cache.
Related Article: Tutorial: Comparing Kafka vs Redis
Performance Considerations: Pub/Sub
Redis provides a publish/subscribe (pub/sub) messaging system that allows different parts of your application to communicate with each other in a decoupled manner. Rust Redis provides convenient methods for working with pub/sub messaging. Let’s explore how to use pub/sub effectively.
Publishing Messages
To publish a message using Rust Redis, you can use the publish()
method. Here’s an example:
use redis::{Client, Commands}; fn publish_message(channel: &str, message: &str) { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection().unwrap(); let _: usize = connection.publish(channel, message).unwrap(); } fn main() { let channel = "my_channel"; let message = "Hello, subscribers!"; publish_message(channel, message); println!("Message published to channel: {}", channel); }
In this example, the publish_message()
function publishes a message to the specified channel using the publish()
method. The method returns the number of subscribers that received the message.
Subscribing to Channels
To subscribe to a channel and receive messages, you can use the subscribe()
method. Here’s an example:
use redis::{Client, Commands}; fn subscribe_to_channel(channel: &str) { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let mut connection = client.get_connection().unwrap(); let mut pubsub = connection.as_pubsub(); pubsub.subscribe(channel).unwrap(); loop { let message = pubsub.get_message().unwrap(); let payload: String = message.get_payload().unwrap(); println!("Received message: {}", payload); } } fn main() { let channel = "my_channel"; subscribe_to_channel(channel); }
In this example, the subscribe_to_channel()
function subscribes to the specified channel using the subscribe()
method. The function enters an infinite loop and uses the get_message()
method to receive messages from the subscribed channel.
Related Article: Exploring Alternatives to Redis
Best Practices for Rust Redis
To effectively use Rust Redis in your projects, it is important to follow certain best practices. Here are some recommendations:
1. Use Connection Pooling: Establishing a connection to the Redis server can be an expensive operation. To improve performance, consider using connection pooling techniques to reuse connections across multiple requests.
2. Serialize and Deserialize Data: Redis stores data as strings, so you need to serialize and deserialize complex data structures to store and retrieve them from Redis. Consider using a serialization library like serde to handle this efficiently.
3. Handle Errors Gracefully: As mentioned earlier, proper error handling is crucial for writing robust code. Make sure to handle errors returned by Redis operations and implement appropriate error recovery or fallback mechanisms.
4. Monitor and Tune Performance: Monitor the performance of your Redis server and fine-tune its configuration based on your application’s requirements. Redis provides various configuration options to optimize performance, including memory management, persistence, and eviction policies.
5. Secure Redis: Redis does not provide built-in security mechanisms, so it is important to secure your Redis server by configuring authentication and firewall rules. Use strong passwords and limit access to trusted clients only.
These best practices will help you optimize the performance and reliability of your applications using Rust Redis.
Real World Examples: Building a Chat Application
Building a chat application is a practical use case for Rust Redis. Let’s explore how Rust Redis can be used to implement a simple chat application.
The chat application will have the following features:
– Users can join chat rooms.
– Users can send messages to chat rooms.
– Users can receive messages from chat rooms.
Here’s an example implementation using Rust Redis:
use redis::{Client, Commands, PubSubCommands, ToRedisArgs}; fn join_chat_room(user_id: &str, room_id: &str) { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection().unwrap(); let _: () = connection.sadd(room_id, user_id).unwrap(); } fn send_message(user_id: &str, room_id: &str, message: &str) { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection().unwrap(); let payload = format!("{}: {}", user_id, message); let _: usize = connection.publish(room_id, payload).unwrap(); } fn receive_messages(user_id: &str, room_id: &str) { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let mut connection = client.get_connection().unwrap(); let mut pubsub = connection.as_pubsub(); pubsub.subscribe(room_id).unwrap(); loop { let message = pubsub.get_message().unwrap(); let payload: String = message.get_payload().unwrap(); let sender_id = payload.split(':').next().unwrap(); if sender_id != user_id { let received_message = payload.splitn(2, ':').nth(1).unwrap(); println!("User {} received message: {}", user_id, received_message); } } } fn main() { let user1 = "user1"; let user2 = "user2"; let room = "chat_room"; join_chat_room(user1, room); join_chat_room(user2, room); send_message(user1, room, "Hello, user2!"); receive_messages(user2, room); }
In this example, the join_chat_room()
function adds a user to a chat room by adding their user ID to a Redis set. The send_message()
function publishes a message to the chat room, prefixing it with the sender’s user ID. The receive_messages()
function subscribes to the chat room and prints received messages, excluding messages sent by the current user.
This example demonstrates how Rust Redis can be used to build real-time communication features in your applications.
Real World Examples: Building a Leaderboard
Building a leaderboard is another practical use case for Rust Redis. Let’s explore how Rust Redis can be used to implement a simple leaderboard.
The leaderboard application will have the following features:
– Users can submit scores.
– Users can retrieve the top scores.
– Users can retrieve their own rank.
Here’s an example implementation using Rust Redis:
use redis::{Client, Commands}; fn submit_score(user_id: &str, score: u32) { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection().unwrap(); let _: () = connection.zadd("leaderboard", score, user_id).unwrap(); } fn get_top_scores(count: usize) -> Vec<(String, u32)> { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection().unwrap(); let scores: Vec<(String, u32)> = connection .zrevrange_withscores("leaderboard", 0, count as isize - 1) .unwrap(); scores } fn get_user_rank(user_id: &str) -> Option<usize> { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection().unwrap(); let rank: Option<usize> = connection.zrevrank("leaderboard", user_id).unwrap(); rank.map(|r| r + 1) } fn main() { let user1 = "user1"; let user2 = "user2"; let user3 = "user3"; submit_score(user1, 100); submit_score(user2, 150); submit_score(user3, 120); let top_scores = get_top_scores(2); for (user_id, score) in top_scores { println!("User: {}, Score: {}", user_id, score); } let user2_rank = get_user_rank(user2); println!("User2 Rank: {:?}", user2_rank); }
In this example, the submit_score()
function adds a user’s score to the leaderboard using the zadd()
method. The get_top_scores()
function retrieves the specified number of top scores from the leaderboard using the zrevrange_withscores()
method. The get_user_rank()
function retrieves the rank of a user from the leaderboard using the zrevrank()
method.
This example demonstrates how Rust Redis can be used to implement leaderboard functionality in your applications.
Related Article: Tutorial on Implementing Redis Sharding
Real World Examples: Implementing Rate Limiting
Implementing rate limiting is another practical use case for Rust Redis. Let’s explore how Rust Redis can be used to implement a basic rate limiting mechanism.
The rate limiting application will have the following features:
– Users can perform a limited number of actions within a specific time window.
– Once the limit is reached, further actions are blocked until the time window resets.
Here’s an example implementation using Rust Redis:
use redis::{Client, Commands}; fn allow_action(user_id: &str, limit: u32, time_window: u32) -> bool { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection().unwrap(); let key = format!("rate_limit:{}", user_id); let count: u32 = connection.incr(&key).unwrap(); if count == 1 { let _: () = connection.expire(&key, time_window).unwrap(); } count <= limit } fn main() { let user1 = "user1"; let user2 = "user2"; let limit = 5; let time_window = 60; for _ in 1..=limit { let allowed = allow_action(user1, limit, time_window); println!("User1 Action Allowed: {}", allowed); } let allowed = allow_action(user1, limit, time_window); println!("User1 Action Allowed: {}", allowed); let allowed = allow_action(user2, limit, time_window); println!("User2 Action Allowed: {}", allowed); }
In this example, the allow_action()
function checks whether a user is allowed to perform an action based on the specified rate limit and time window. The function uses the incr()
method to increment a counter associated with the user’s rate limit key. If the counter is 1, indicating the start of a new time window, the function sets an expiration time using the expire()
method. The function returns true
if the count is less than or equal to the limit, allowing the action.
This example demonstrates how Rust Redis can be used to implement rate limiting functionality in your applications.
Code Snippet: Working with Hashes
Working with hashes is a common requirement when using Redis. Rust Redis provides convenient methods to work with hashes. Here’s an example of working with hashes in Rust Redis:
use redis::{Client, Commands}; fn main() { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection().unwrap(); let _: () = connection.hset("user:123", "name", "John Doe").unwrap(); let _: () = connection.hset("user:123", "email", "john@example.com").unwrap(); let name: String = connection.hget("user:123", "name").unwrap(); let email: String = connection.hget("user:123", "email").unwrap(); println!("Name: {}", name); println!("Email: {}", email); }
In this example, the hset()
method is used to set the fields and values of a hash named “user:123”. We can then retrieve the field values using the hget()
method.
Working with hashes allows you to represent complex objects and easily access their properties in Redis.
Code Snippet: Working with Sets
Sets are another useful Redis data structure that can be efficiently used with Rust Redis. Here’s an example of working with sets in Rust Redis:
use redis::{Client, Commands}; fn main() { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection().unwrap(); let _: () = connection.sadd("tags", "rust").unwrap(); let _: () = connection.sadd("tags", "redis").unwrap(); let _: () = connection.sadd("tags", "tutorial").unwrap(); let tags: Vec<String> = connection.smembers("tags").unwrap(); println!("Tags: {:?}", tags); }
In this example, the sadd()
method is used to add elements to a set named “tags”. We can retrieve all the members of the set using the smembers()
method.
Working with sets allows you to store and manipulate collections of unique elements efficiently in Redis.
Related Article: Tutorial: Redis vs RabbitMQ Comparison
Code Snippet: Working with Sorted Sets
Sorted sets in Redis are a powerful data structure that provides ordering and uniqueness of elements. Here’s an example of working with sorted sets in Rust Redis:
use redis::{Client, Commands}; fn main() { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection().unwrap(); let _: () = connection.zadd("leaderboard", 100, "John").unwrap(); let _: () = connection.zadd("leaderboard", 200, "Jane").unwrap(); let _: () = connection.zadd("leaderboard", 150, "Alice").unwrap(); let leaderboard: Vec<String> = connection.zrange("leaderboard", 0, -1).unwrap(); println!("Leaderboard: {:?}", leaderboard); }
In this example, the zadd()
method is used to add members with scores to a sorted set named “leaderboard”. We can retrieve the members in ascending order using the zrange()
method.
Sorted sets provide a powerful way to store and retrieve ordered collections in Redis.
Code Snippet: Pub/Sub Messaging
Pub/sub messaging is a powerful feature of Redis that enables communication between different parts of your application. Here’s an example of using pub/sub messaging in Rust Redis:
use redis::{Client, Commands}; fn publish_message(channel: &str, message: &str) { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection().unwrap(); let _: usize = connection.publish(channel, message).unwrap(); } fn main() { let channel = "my_channel"; let message = "Hello, subscribers!"; publish_message(channel, message); println!("Message published to channel: {}", channel); }
In this example, the publish_message()
function publishes a message to the specified channel using the publish()
method.
This code snippet demonstrates how to publish messages using Rust Redis’s pub/sub messaging system.
Advanced Technique: Lua Scripting in Rust Redis
Redis supports Lua scripting, which allows you to execute custom scripts on the server. Rust Redis provides a convenient way to execute Lua scripts using the script()
method. Here’s an example:
use redis::{Client, Commands}; fn main() { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection().unwrap(); let script = r#" local key = KEYS[1] local value = ARGV[1] redis.call('set', key, value) return redis.call('get', key) "#; let result: String = connection .script::<_, String>(redis::ScriptingCommand::new(script).key("my_key").arg("my_value")) .unwrap(); println!("Result: {}", result); }
In this example, we define a Lua script that sets a key-value pair in Redis and retrieves the value. The script is executed using the script()
method, specifying the script code, keys, and arguments.
Related Article: Tutorial on Integrating Redis with Spring Boot
Advanced Technique: Transactions and Pipelining
Transactions and pipelining are advanced techniques that can significantly improve the performance and reliability of your Redis operations. Rust Redis provides convenient methods to work with transactions and pipelining.
To work with transactions, you can use the transaction()
method to create a transaction block and execute multiple commands within it. Here’s an example:
use redis::{Client, Commands, PipelineCommands}; fn main() { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection().unwrap(); let mut transaction = redis::transaction(&connection).unwrap(); transaction.set("name", "John").unwrap(); transaction.set("age", 30).unwrap(); let _: () = transaction.query(()).unwrap(); let name: String = connection.get("name").unwrap(); let age: i32 = connection.get("age").unwrap(); println!("Name: {}", name); println!("Age: {}", age); }
In this example, we create a transaction using the redis::transaction()
method and execute multiple commands within the transaction block. The query()
method is used to execute the transaction and commit the changes.
To work with pipelining, you can use the pipe()
method to create a pipeline and add multiple commands to it. Here’s an example:
use redis::{Client, Commands, PipelineCommands}; fn main() { let client = Client::open("redis://127.0.0.1:6379/").unwrap(); let connection = client.get_connection().unwrap(); let mut pipeline = redis::pipe(); pipeline.set("name", "John"); pipeline.set("age", 30); let _: () = pipeline.query(&connection).unwrap(); let name: String = connection.get("name").unwrap(); let age: i32 = connection.get("age").unwrap(); println!("Name: {}", name); println!("Age: {}", age); }
In this example, we use the redis::pipe()
method to create a pipeline and add multiple commands to it using the set()
method. The query()
method is used to execute the pipeline and retrieve the results.
Transactions ensure atomicity and consistency, while pipelining improves performance by reducing round-trips to the server. These advanced techniques can be used to optimize your Redis operations in Rust Redis.
Advanced Technique: Cluster Support in Rust Redis
Redis provides built-in support for clustering, allowing you to distribute data across multiple Redis nodes for scalability and high availability. Rust Redis includes support for Redis clusters, making it easy to work with clustered Redis deployments.
To connect to a Redis cluster from your Rust application, you can use the redis::cluster::ClusterConnection
struct. Here’s an example:
use redis::{cluster::ClusterConnection, Commands}; fn main() { let nodes = vec!["redis://127.0.0.1:7000", "redis://127.0.0.1:7001", "redis://127.0.0.1:7002"]; let connection = ClusterConnection::open(nodes).unwrap(); let _: () = connection.set("name", "John").unwrap(); let name: String = connection.get("name").unwrap(); println!("Name: {}", name); }
In this example, we create a ClusterConnection
by specifying the addresses of the Redis cluster nodes. We can then use the connection to perform operations on the cluster, such as setting and getting values.
Rust Redis handles the complexities of interacting with a Redis cluster, including key slot calculations, node discovery, and automatic redirection.
These advanced techniques and features demonstrate the power and flexibility of Rust Redis in handling complex scenarios and optimizing your Redis operations.