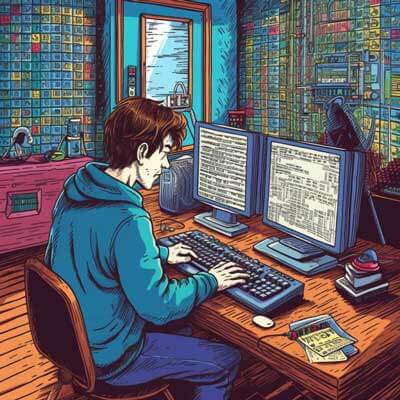
- Introduction to Redis Queue
- Basic Examples of Using Redis Queue
- Advanced Examples of Using Redis Queue
- Error Handling in Redis Queue
- Performance Considerations: Scaling Redis Queue
- Performance Considerations: Optimizing Redis Queue
- Use Case 1: Task Processing
- Use Case 2: Background Jobs
- Use Case 3: Event Sourcing
- Best Practices for Redis Queue Implementation
- Real World Example 1: Building a Job Queue
- Real World Example 2: Implementing a Task Scheduler
- Real World Example 3: Creating a Message Broker
- Code Snippet: Adding a Job to Redis Queue
- Code Snippet: Retrieving and Processing Jobs from Redis Queue
- Code Snippet: Monitoring Redis Queue
- Advanced Technique 1: Job Prioritization
- Advanced Technique 2: Job Retry Strategies
- Advanced Technique 3: Distributed Queue Architecture
- Advanced Technique 4: Job Dependencies
- Advanced Technique 5: Rate Limiting
- Advanced Technique 6: Dead Letter Queue
Introduction to Redis Queue
Redis Queue is a powerful tool for managing and processing tasks asynchronously. It is built on top of Redis, a popular in-memory data store, and provides a simple yet efficient way to handle background jobs, task scheduling, and event sourcing. With its lightweight design and fast performance, Redis Queue has become a preferred choice for developers working with distributed systems and microservices architectures.
Related Article: Leveraging Redis for Caching Frequently Used Queries
Basic Examples of Using Redis Queue
To get started with Redis Queue, you need to install the Redis server and the Python Redis library. Once the setup is complete, you can begin using Redis Queue with the following basic examples:
Example 1: Adding a Job to Redis Queue
from redis import Redis from rq import Queue # Connect to Redis server redis_conn = Redis() # Create a queue queue = Queue(connection=redis_conn) # Add a job to the queue job = queue.enqueue('my_module.my_function', arg1, arg2, arg3)
Example 2: Retrieving and Processing Jobs from Redis Queue
from redis import Redis from rq import Queue, Worker # Connect to Redis server redis_conn = Redis() # Create a queue queue = Queue(connection=redis_conn) # Create a worker worker = Worker([queue], connection=redis_conn) # Start the worker worker.work()
Advanced Examples of Using Redis Queue
Redis Queue provides advanced features that can be utilized for more complex use cases. Here are a couple of examples:
Example 1: Job Prioritization
from redis import Redis from rq import Queue # Connect to Redis server redis_conn = Redis() # Create a high-priority queue high_priority_queue = Queue('high', connection=redis_conn) # Create a low-priority queue low_priority_queue = Queue('low', connection=redis_conn) # Add a high-priority job high_priority_job = high_priority_queue.enqueue('my_module.my_function', arg1, arg2, arg3) # Add a low-priority job low_priority_job = low_priority_queue.enqueue('my_module.my_function', arg1, arg2, arg3)
Example 2: Job Retry Strategies
from redis import Redis from rq import Queue # Connect to Redis server redis_conn = Redis() # Create a queue with retry options queue = Queue(connection=redis_conn, retry=3, retry_interval=[10, 20, 30]) # Add a job that will be retried up to 3 times with increasing intervals job = queue.enqueue('my_module.my_function', arg1, arg2, arg3)
Error Handling in Redis Queue
When working with Redis Queue, it is important to handle errors effectively to ensure the reliability of your application. Redis Queue provides mechanisms for capturing and handling errors during job execution. Here’s an example of error handling in Redis Queue:
from redis import Redis from rq import Queue # Connect to Redis server redis_conn = Redis() # Create a queue queue = Queue(connection=redis_conn) # Define a function that may raise an exception def my_function(arg1, arg2): # Perform some operation result = perform_operation(arg1, arg2) # Check if the operation failed if result is None: # Raise an exception to indicate the failure raise Exception('Operation failed') # Add a job to the queue job = queue.enqueue(my_function, arg1, arg2) # Retrieve and handle job execution errors try: result = job.result except Exception as e: # Handle the error handle_error(e)
Related Article: Analyzing Redis Query Rate per Second with Sidekiq
Performance Considerations: Scaling Redis Queue
As your application grows and the workload increases, it is important to consider the scalability of your Redis Queue implementation. Scaling Redis Queue involves distributing the workload across multiple Redis instances and workers. Here are a few considerations for scaling Redis Queue:
– Use Redis Cluster for horizontal scaling: Redis Cluster allows you to distribute your data across multiple Redis instances, providing high availability and fault tolerance.
– Increase the number of worker processes: By increasing the number of worker processes, you can parallelize the execution of jobs and handle a higher workload.
– Utilize Redis Sentinel for high availability: Redis Sentinel provides automatic failover and monitoring for Redis instances, ensuring that your Redis Queue stays operational even in the event of failures.
Performance Considerations: Optimizing Redis Queue
To optimize the performance of your Redis Queue implementation, you can consider the following techniques:
– Minimize network round trips: Reduce the number of Redis commands and pipeline multiple commands into a single request to minimize network latency.
– Use Redis pipelines: Pipelining allows you to send multiple commands to Redis without waiting for the response, reducing the overhead of network round trips.
– Enable Redis compression: Redis supports compression of values larger than a certain threshold. Enabling compression can reduce memory usage and network bandwidth.
– Monitor Redis performance: Utilize tools like Redis Monitor or Redis Sentinel to monitor the performance of your Redis server and identify bottlenecks.
Use Case 1: Task Processing
Redis Queue is commonly used for task processing in web applications. Tasks can be offloaded to background workers, allowing the main application to respond quickly to user requests. Here’s an example of using Redis Queue for task processing:
from redis import Redis from rq import Queue # Connect to Redis server redis_conn = Redis() # Create a queue queue = Queue(connection=redis_conn) # Define a task function def send_email(to, subject, body): # Send email logic send_email(to, subject, body) # Add a task to the queue task = queue.enqueue(send_email, 'user@example.com', 'Hello', 'Welcome to our website!')
Related Article: How to use Redis with Laravel and PHP
Use Case 2: Background Jobs
Redis Queue is also suitable for running background jobs that require long execution times or periodic scheduling. Here’s an example of using Redis Queue for background jobs:
from redis import Redis from rq import Queue, Worker # Connect to Redis server redis_conn = Redis() # Create a queue queue = Queue(connection=redis_conn) # Create a worker worker = Worker([queue], connection=redis_conn) # Start the worker worker.work()
Use Case 3: Event Sourcing
Redis Queue can be used for event sourcing, which involves capturing and storing events that represent changes to the state of an application. Here’s an example of using Redis Queue for event sourcing:
from redis import Redis from rq import Queue # Connect to Redis server redis_conn = Redis() # Create a queue queue = Queue(connection=redis_conn) # Publish an event to the queue event = {'type': 'user_registered', 'data': {'user_id': 123, 'name': 'John Doe'}} queue.enqueue('event_handler.handle_event', event)
Best Practices for Redis Queue Implementation
To ensure the smooth operation and efficient usage of Redis Queue, consider the following best practices:
– Monitor Redis Queue: Regularly monitor the performance and health of your Redis Queue implementation using tools like Redis Monitor or Redis Sentinel.
– Handle errors gracefully: Implement error handling mechanisms to handle exceptions and failures during job execution.
– Optimize job execution: Optimize your job functions to minimize execution time and avoid unnecessary operations.
– Use proper queue names: Use descriptive queue names that reflect the purpose of the jobs they contain.
– Implement job timeouts: Set appropriate timeouts for jobs to prevent them from running indefinitely.
Related Article: Tutorial on Redis Docker Compose
Real World Example 1: Building a Job Queue
In this real-world example, we will build a job queue system using Redis Queue. The job queue will handle tasks asynchronously, allowing other parts of the application to continue processing user requests. Here’s an example implementation:
from redis import Redis from rq import Queue # Connect to Redis server redis_conn = Redis() # Create a queue queue = Queue(connection=redis_conn) # Define a task function def process_task(task_id): # Process the task process_task(task_id) # Add tasks to the queue for task_id in range(1, 100): queue.enqueue(process_task, task_id)
Real World Example 2: Implementing a Task Scheduler
In this real-world example, we will implement a task scheduler using Redis Queue. The task scheduler will allow us to schedule tasks to be executed at specific times or intervals. Here’s an example implementation:
from redis import Redis from rq_scheduler import Scheduler # Connect to Redis server redis_conn = Redis() # Create a scheduler scheduler = Scheduler(connection=redis_conn) # Define a scheduled task function def send_reminder(to, message): # Send reminder logic send_reminder(to, message) # Schedule a task to be executed once scheduler.enqueue_at(datetime(2022, 1, 1, 12, 0), send_reminder, 'user@example.com', 'Hello!') # Schedule a task to be executed every day at 9 AM scheduler.enqueue_cron('0 9 * * *', send_reminder, 'user@example.com', 'Good morning!')
Real World Example 3: Creating a Message Broker
In this real-world example, we will create a message broker using Redis Queue. The message broker will facilitate communication between different parts of a distributed system. Here’s an example implementation:
from redis import Redis from rq import Queue # Connect to Redis server redis_conn = Redis() # Create a queue for incoming messages incoming_queue = Queue('incoming', connection=redis_conn) # Create a queue for outgoing messages outgoing_queue = Queue('outgoing', connection=redis_conn) # Define a function to handle incoming messages def handle_message(message): # Process the message process_message(message) # Listen for incoming messages while True: # Retrieve a message from the incoming queue message = incoming_queue.dequeue() if message: # Handle the message handle_message(message)
Related Article: Tutorial on Redis Sentinel: A Deep Look
Code Snippet: Adding a Job to Redis Queue
To add a job to Redis Queue, use the enqueue
method of the queue object. Here’s an example:
from redis import Redis from rq import Queue # Connect to Redis server redis_conn = Redis() # Create a queue queue = Queue(connection=redis_conn) # Add a job to the queue job = queue.enqueue('my_module.my_function', arg1, arg2, arg3)
Code Snippet: Retrieving and Processing Jobs from Redis Queue
To retrieve and process jobs from Redis Queue, use a worker. Here’s an example:
from redis import Redis from rq import Queue, Worker # Connect to Redis server redis_conn = Redis() # Create a queue queue = Queue(connection=redis_conn) # Create a worker worker = Worker([queue], connection=redis_conn) # Start the worker worker.work()
Code Snippet: Monitoring Redis Queue
To monitor the performance and health of your Redis Queue implementation, you can use tools like Redis Monitor or Redis Sentinel. Here’s an example of using Redis Monitor:
redis-cli monitor
Related Article: Tutorial: Integrating Redis with Spring Boot
Advanced Technique 1: Job Prioritization
Redis Queue allows you to prioritize jobs by assigning them to different queues or using custom job priorities. Here’s an example:
from redis import Redis from rq import Queue # Connect to Redis server redis_conn = Redis() # Create a high-priority queue high_priority_queue = Queue('high', connection=redis_conn) # Create a low-priority queue low_priority_queue = Queue('low', connection=redis_conn) # Add a high-priority job high_priority_job = high_priority_queue.enqueue('my_module.my_function', arg1, arg2, arg3) # Add a low-priority job low_priority_job = low_priority_queue.enqueue('my_module.my_function', arg1, arg2, arg3)
Advanced Technique 2: Job Retry Strategies
Redis Queue provides built-in support for job retry strategies, allowing you to automatically retry failed jobs. Here’s an example:
from redis import Redis from rq import Queue # Connect to Redis server redis_conn = Redis() # Create a queue with retry options queue = Queue(connection=redis_conn, retry=3, retry_interval=[10, 20, 30]) # Add a job that will be retried up to 3 times with increasing intervals job = queue.enqueue('my_module.my_function', arg1, arg2, arg3)
Advanced Technique 3: Distributed Queue Architecture
Redis Queue can be used in a distributed architecture by using Redis Cluster or Redis Sentinel. This allows you to scale your Redis Queue implementation across multiple Redis instances and handle high workloads. Here’s an example:
from rediscluster import RedisCluster from rq import Queue # Connect to Redis Cluster redis_nodes = [{'host': 'redis1.example.com', 'port': 7000}, {'host': 'redis2.example.com', 'port': 7000}] redis_conn = RedisCluster(startup_nodes=redis_nodes) # Create a queue queue = Queue(connection=redis_conn) # Add a job to the queue job = queue.enqueue('my_module.my_function', arg1, arg2, arg3)
Related Article: Tutorial on Redis Sharding Implementation
Advanced Technique 4: Job Dependencies
Redis Queue allows you to define dependencies between jobs, ensuring that certain jobs are executed only after their dependencies have completed. Here’s an example:
from redis import Redis from rq import Queue # Connect to Redis server redis_conn = Redis() # Create a queue queue = Queue(connection=redis_conn) # Add a job with dependencies job1 = queue.enqueue('my_module.my_function', arg1, arg2, arg3) job2 = queue.enqueue('my_module.my_function', arg1, arg2, arg3) job3 = queue.enqueue('my_module.my_function', arg1, arg2, arg3) # Set job2 and job3 as dependencies for job1 job1.dependency = [job2, job3] job1.save()
Advanced Technique 5: Rate Limiting
Redis Queue supports rate limiting to control the rate at which jobs are executed. Here’s an example:
from redis import Redis from rq import Queue # Connect to Redis server redis_conn = Redis() # Create a queue with rate limiting queue = Queue(connection=redis_conn, burst_limit=10, burst_timeout=5) # Add jobs to the queue for i in range(100): job = queue.enqueue('my_module.my_function', arg1, arg2, arg3)
Advanced Technique 6: Dead Letter Queue
Redis Queue provides a dead letter queue mechanism to handle failed jobs. Failed jobs can be moved to a dedicated queue for further analysis or manual processing. Here’s an example:
from redis import Redis from rq import Queue # Connect to Redis server redis_conn = Redis() # Create a queue queue = Queue(connection=redis_conn) # Create a dead letter queue dlq = Queue('dead_letter', connection=redis_conn) # Move failed jobs to the dead letter queue queue.move_to(dlq, failed=True)