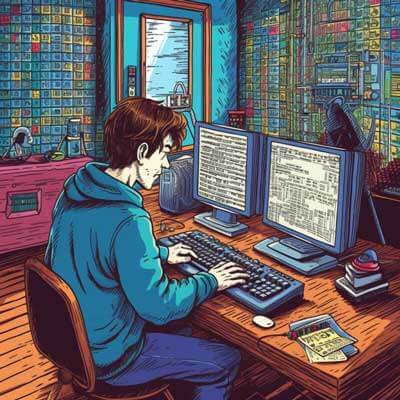
Table of Contents
Introduction
Kafka and Redis are two popular data storage and messaging systems used in modern software applications. While both serve different purposes and have distinct features, they are often compared when it comes to real-time data streaming, caching, and message queuing. In this tutorial, we will explore the strengths and weaknesses of Kafka and Redis, and discuss various use cases, best practices, performance considerations, and advanced techniques for working with these technologies.
Related Article: Tutorial on installing and using redis-cli in Redis
Overview of Kafka
Apache Kafka is a distributed streaming platform that allows applications to publish and subscribe to streams of records. It is designed to handle high volumes of data, provide fault-tolerance, and ensure real-time data processing. Kafka is known for its high throughput, low latency, and scalability. It follows a publish-subscribe model, where producers write data to topics, and consumers read data from these topics. Kafka guarantees the ordering of messages within a partition and allows parallel processing of messages across multiple partitions.
Code Snippet: Kafka Producer
import org.apache.kafka.clients.producer.*; import java.util.Properties; public class KafkaProducerExample { public static void main(String[] args) { String topicName = "my-topic"; String bootstrapServers = "localhost:9092"; Properties props = new Properties(); props.put("bootstrap.servers", bootstrapServers); props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer"); props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer"); Producer<String, String> producer = new KafkaProducer<>(props); for (int i = 0; i < 10; i++) { String key = "key-" + i; String value = "value-" + i; ProducerRecord<String, String> record = new ProducerRecord<>(topicName, key, value); producer.send(record); } producer.close(); } }
Code Snippet: Kafka Consumer
import org.apache.kafka.clients.consumer.*; import org.apache.kafka.common.TopicPartition; import java.util.Collections; import java.util.Properties; public class KafkaConsumerExample { public static void main(String[] args) { String topicName = "my-topic"; String bootstrapServers = "localhost:9092"; String groupId = "my-group"; Properties props = new Properties(); props.put("bootstrap.servers", bootstrapServers); props.put("key.deserializer", "org.apache.kafka.common.serialization.StringDeserializer"); props.put("value.deserializer", "org.apache.kafka.common.serialization.StringDeserializer"); props.put("group.id", groupId); Consumer<String, String> consumer = new KafkaConsumer<>(props); consumer.subscribe(Collections.singletonList(topicName)); while (true) { ConsumerRecords<String, String> records = consumer.poll(100); for (ConsumerRecord<String, String> record : records) { System.out.println("Received message: " + record.value()); } } } }
Related Article: Tutorial: Setting Up Redis Using Docker Compose
Overview of Redis
Redis is an open-source, in-memory data structure store that can be used as a database, cache, and message broker. It provides high-performance data storage and retrieval, and supports a wide range of data structures such as strings, lists, sets, hashes, and more. Redis is known for its simplicity, versatility, and low-latency operations. It offers various features like replication, persistence, clustering, and pub/sub messaging.
Code Snippet: Redis Cache Implementation
import redis # Connect to Redis redis_host = "localhost" redis_port = 6379 redis_db = 0 redis_client = redis.Redis(host=redis_host, port=redis_port, db=redis_db) # Set a key-value pair in Redis cache key = "my-key" value = "my-value" redis_client.set(key, value) # Get the value from Redis cache cached_value = redis_client.get(key) print("Cached value: " + cached_value.decode())
Code Snippet: Redis Pub/Sub Implementation
import redis # Connect to Redis redis_host = "localhost" redis_port = 6379 redis_db = 0 redis_client = redis.Redis(host=redis_host, port=redis_port, db=redis_db) # Publish a message to a channel channel = "my-channel" message = "Hello, Redis!" redis_client.publish(channel, message) # Subscribe to a channel and receive messages pubsub = redis_client.pubsub() pubsub.subscribe(channel) for message in pubsub.listen(): print("Received message: " + message['data'].decode())
Comparing Kafka and Redis
When comparing Kafka and Redis, it's important to consider their intended use cases and primary strengths. Kafka excels in handling high volumes of real-time data streaming, while Redis is well-suited for caching and pub/sub messaging scenarios. Here are some key differences between them:
- Kafka is designed as a distributed streaming platform, while Redis is an in-memory data store with additional features.
- Kafka provides fault-tolerant, scalable, and ordered message delivery across multiple consumers, making it ideal for event-driven architectures.
- Redis offers high-performance caching, with support for data persistence, replication, and various data structures.
Related Article: Tutorial on Redis Docker Compose
Use Case: Real-time Data Streaming
Real-time data streaming is a common use case where Kafka shines. It allows data to be ingested and processed in real-time, enabling applications to react immediately to changing data. Kafka's distributed architecture and fault-tolerant design make it suitable for high-throughput data streams. Here's an example of how Kafka can be used for real-time data streaming:
1. Set up a Kafka cluster with multiple brokers.
2. Create a topic to which data will be published.
3. Develop a Kafka producer application that publishes data to the topic.
4. Implement one or more Kafka consumer applications that process the data in real-time.
Use Case: Caching
Caching is a technique used to store frequently accessed data in memory for faster retrieval. Redis is widely used as a caching solution due to its speed and versatility. It supports various data structures and provides advanced caching features like expiration, eviction policies, and automatic cache population. Here's an example of how Redis can be used for caching:
1. Connect to a Redis instance.
2. Set a key-value pair in Redis cache.
3. Retrieve the value from the cache.
4. If the value is present, use it; otherwise, fetch it from the data source and store it in the cache for future use.
Use Case: Message Queues
Message queues are used to enable asynchronous communication between components of a system. Both Kafka and Redis can be used as message brokers, but they have different characteristics. Kafka provides ordered, fault-tolerant, and scalable message delivery, making it suitable for building event-driven architectures. Redis pub/sub messaging allows for real-time message broadcasting to multiple subscribers. Here's an example of using Kafka and Redis as message queues:
1. Set up a Kafka cluster or Redis instance.
2. Create a topic or channel for message communication.
3. Develop producer applications to publish messages to the topic or channel.
4. Implement consumer applications to subscribe and receive messages.
Best Practices for Using Kafka
When using Kafka, it is important to follow best practices to ensure optimal performance and reliability. Here are some best practices for working with Kafka:
- Use multiple partitions to enable parallelism and increase throughput.
- Monitor and configure appropriate retention policies for topics.
- Set up replication to provide fault-tolerance and high availability.
- Tune Kafka settings based on the specific requirements of your application.
- Use Kafka Connect for integrating with external systems.
Related Article: How to Use Redis with Django Applications
Best Practices for Using Redis
To make the most of Redis, it is recommended to follow certain best practices. Here are some best practices for using Redis:
- Use appropriate data structures for different use cases.
- Leverage Redis persistence options based on your requirements.
- Implement appropriate eviction and expiry policies for cache management.
- Utilize Redis clustering for scalability and high availability.
- Monitor Redis performance and resource utilization.
Real World Example: Event Streaming Platform
An event streaming platform is a common use case where Kafka is widely used. It allows real-time processing of events generated by various applications and systems. Here's an example of an event streaming platform architecture using Kafka:
- Producers generate events and publish them to Kafka topics.
- Consumers subscribe to the topics and process the events in real-time.
- Stream processing frameworks like Apache Flink or Spark Streaming can be used to perform complex event processing and analytics.
Real World Example: High Traffic Web Application
For a high traffic web application, Redis can be used as a caching layer to improve performance and reduce the load on the database. Here's an example architecture for a high traffic web application using Redis:
- Incoming requests are first checked in the Redis cache.
- If the data is present in the cache, it is served directly to the user.
- If the data is not present, the application retrieves it from the database, stores it in Redis for future requests, and serves it to the user.
Performance Considerations for Kafka
When using Kafka, it is important to understand and optimize its performance. Consider the following factors:
- Configure appropriate partitioning and replication factors for topics.
- Monitor and tune the Kafka cluster for optimal throughput and latency.
- Batch messages for efficient network utilization.
- Use compression for reducing network bandwidth usage.
- Consider hardware and network optimizations for better performance.
Related Article: How to use Redis with Laravel and PHP
Performance Considerations for Redis
To ensure optimal performance with Redis, consider the following factors:
- Choose the right Redis data structures for your use case.
- Carefully design the cache eviction and expiry policies.
- Monitor Redis memory usage and configure appropriate memory settings.
- Optimize network latency by placing Redis closer to the application.
- Utilize Redis clustering for horizontal scalability.
Advanced Technique: Kafka Topic Partitioning
Kafka topic partitioning allows for parallel processing of messages across multiple consumers. By partitioning a topic, Kafka ensures that messages with the same key are always published to the same partition. This allows for ordering guarantees within a partition. Here's an example of Kafka topic partitioning:
- Create a topic with multiple partitions.
- Publish messages to the topic with keys to ensure ordering within partitions.
- Consumers can be scaled horizontally to process messages from different partitions in parallel.
Advanced Technique: Redis Pub/Sub
Redis pub/sub messaging enables real-time message broadcasting to multiple subscribers. It follows a publish-subscribe pattern, where publishers send messages to channels, and subscribers receive messages from these channels. Here's an example of Redis pub/sub messaging:
- Connect to a Redis instance.
- Subscribe to channels of interest.
- Publish messages to the channels.
- Subscribers receive and process the messages in real-time.
Error Handling in Kafka
When working with Kafka, it is important to handle errors gracefully. Some common error handling practices include:
- Implementing retry policies for failed message processing.
- Monitoring and handling consumer lag to ensure timely processing.
- Handling network failures and reconnecting to the Kafka cluster.
- Using dead-letter queues to handle messages that couldn't be processed.
Related Article: Tutorial on Redis Sharding Implementation
Error Handling in Redis
When using Redis, it is important to handle errors effectively. Consider the following error handling practices:
- Check for Redis connection errors and handle them gracefully.
- Implement proper exception handling and error reporting in Redis client libraries.
- Monitor Redis server logs for any error messages or warnings.
- Ensure proper error handling during cache retrieval or storage operations.