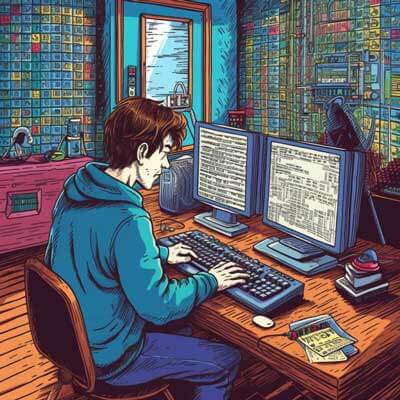
- Introduction
- Architecture of Redis Sharding
- Setting Up a Redis Cluster
- Performance Considerations for Redis Sharding
- Best Practices for Redis Sharding
- Error Handling in Redis Sharding
- Real World Examples of Redis Sharding
- Code Snippet: Implementing Redis Sharding
- Code Snippet: Load Balancing in Redis Sharding
- Code Snippet: Data Replication in Redis Sharding
- Advanced Technique: Consistent Hashing in Redis Sharding
- Advanced Technique: Data Partitioning in Redis Sharding
- Advanced Technique: Failover Mechanisms in Redis Sharding
- Advanced Technique: Scaling Redis Sharding
- Use Case: Redis Sharding for High Traffic Applications
- Use Case: Redis Sharding for Real-time Data Processing
- Use Case: Redis Sharding for Caching in Distributed Systems
Introduction
Redis is a popular open-source, in-memory data structure store that can be used as a database, cache, and message broker. Redis Sharding is a technique used to distribute data across multiple Redis instances, allowing for horizontal scalability and increased performance. In this tutorial, we will explore the implementation of Redis Sharding and its various aspects.
Related Article: Tutorial on Rust Redis: Tools and Techniques
Architecture of Redis Sharding
Redis Sharding involves partitioning data across multiple Redis instances, also known as shards. Each shard is responsible for a subset of the data, and collectively they form a distributed Redis cluster. The architecture typically consists of a client application, a sharding mechanism, and multiple Redis instances.
To implement Redis Sharding, a common approach is to use a consistent hashing algorithm. This algorithm maps keys to specific shards consistently, even when the number of shards changes. By distributing the data across multiple shards, Redis Sharding allows for parallel processing and improved performance.
Here is an example code snippet showing how consistent hashing can be implemented in Redis Sharding:
import hashlib def get_shard(key, num_shards): hash_value = hashlib.md5(key.encode()).hexdigest() shard_index = int(hash_value, 16) % num_shards return shard_index
In this code snippet, we use the MD5 hash function to generate a hash value for the key. We then convert the hash value to an integer and calculate the shard index by taking the modulus of the number of shards. This ensures that the key is consistently mapped to the same shard.
Setting Up a Redis Cluster
To set up a Redis cluster for sharding, we need to configure multiple Redis instances and establish communication between them. The Redis Cluster feature simplifies this process by providing automatic sharding and high availability.
To create a Redis cluster, we start by launching multiple Redis instances on different machines or servers. Each Redis instance is assigned a unique port number and given a specific role in the cluster, such as master or slave. The nodes communicate with each other using a gossip protocol to maintain cluster state and handle failover.
Here is an example code snippet demonstrating the creation of a Redis cluster using the Redis Cluster feature:
redis-cli --cluster create <ip1>:<port1> <ip2>:<port2> ... <ipn>:<portn> --cluster-replicas <num_replicas>
In this command, we specify the IP address and port number of each Redis instance in the cluster. We also specify the number of replicas to create for each master node using the --cluster-replicas
option. The Redis Cluster feature takes care of automatically assigning slots to the instances and setting up the necessary communication channels.
Performance Considerations for Redis Sharding
When implementing Redis Sharding, it is important to consider performance optimization techniques to ensure efficient data distribution and retrieval. Here are some key considerations:
1. Pipeline Commands: Redis supports pipelining, which allows multiple commands to be sent to the server in a single batch. This reduces network round-trips and improves overall performance. Here is an example code snippet demonstrating pipelining in Python:
with redis.pipeline() as pipe: pipe.set('key1', 'value1') pipe.get('key2') pipe.execute()
2. Connection Pooling: Maintaining a pool of reusable connections to the Redis instances can significantly improve performance by avoiding the overhead of establishing a new connection for every request. Popular Redis client libraries often provide built-in connection pooling mechanisms.
3. Data Compression: If the data stored in Redis is compressible, applying compression algorithms like gzip or Snappy can reduce memory usage and improve performance. However, it is important to consider the tradeoff between CPU utilization for compression and the benefits gained from reduced memory usage.
Related Article: Redis vs MongoDB: A Detailed Comparison
Best Practices for Redis Sharding
When implementing Redis Sharding, following best practices can help ensure a robust and efficient setup:
1. Monitor Cluster Health: Regularly monitor the health of the Redis cluster using tools like Redis Sentinel or Redis Cluster’s built-in monitoring features. This helps identify potential issues and enables proactive maintenance.
2. Data Distribution Strategy: Choose an appropriate data distribution strategy based on the workload characteristics. For example, if certain keys are accessed more frequently, consider using a separate shard for those keys to distribute the load evenly.
3. Shard Sizing: Determine the optimal size for each shard based on the expected data volume and the available system resources. Oversized shards can lead to increased memory usage and slower performance, while undersized shards may limit scalability.
Error Handling in Redis Sharding
When working with Redis Sharding, it is important to handle errors effectively to ensure data integrity and maintain system stability. Some common error scenarios and their handling techniques include:
1. Shard Unavailability: If a shard becomes unavailable due to network issues or hardware failures, it is important to handle the error gracefully. This may involve redirecting requests to other available shards or triggering a failover mechanism to promote a replica as the new master.
2. Network Errors: Redis clients should handle network errors, such as connection timeouts or socket errors, by retrying the operation or redirecting requests to alternative shards.
Here is an example code snippet demonstrating error handling in Redis Sharding using the Redis client library for Python:
import redis try: # Perform Redis Sharding operation result = redis_client.get(key) except redis.exceptions.RedisError as e: # Handle Redis Sharding error print(f"Error: {str(e)}")
In this code snippet, we catch the RedisError
exception and handle it appropriately, such as logging the error or retrying the operation.
Real World Examples of Redis Sharding
Redis Sharding is widely used in various real-world scenarios to handle high traffic, improve performance, and provide fault tolerance. Here are a few examples:
1. Social Media Applications: Social media platforms often use Redis Sharding to distribute user data across multiple shards, enabling efficient retrieval of user profiles, posts, and interactions.
2. E-commerce Websites: Online marketplaces use Redis Sharding to handle large product catalogs and user shopping carts. Sharding allows for faster search and retrieval of product information, as well as efficient management of user session data.
Related Article: Tutorial on Integrating Redis with Spring Boot
Code Snippet: Implementing Redis Sharding
Here is an example code snippet demonstrating how to implement Redis Sharding in Python using the Redis client library:
import redis shards = [ redis.Redis(host='shard1.example.com', port=6379), redis.Redis(host='shard2.example.com', port=6379), redis.Redis(host='shard3.example.com', port=6379), ] def get_shard(key): shard_index = hash(key) % len(shards) return shards[shard_index] # Example usage shard = get_shard('my_key') shard.set('my_key', 'my_value')
In this code snippet, we define a list of Redis instances representing the shards. The get_shard
function calculates the shard index based on the hash of the key and returns the corresponding Redis instance. We can then use the obtained shard to perform operations on the Redis cluster.
Code Snippet: Load Balancing in Redis Sharding
Load balancing is an important aspect of Redis Sharding to evenly distribute the workload across the shards. Here is an example code snippet demonstrating load balancing using a round-robin strategy in Python:
import redis shards = [ redis.Redis(host='shard1.example.com', port=6379), redis.Redis(host='shard2.example.com', port=6379), redis.Redis(host='shard3.example.com', port=6379), ] def get_next_shard(): # Implement round-robin load balancing global current_shard_index current_shard_index = (current_shard_index + 1) % len(shards) return shards[current_shard_index] # Example usage shard = get_next_shard() shard.set('my_key', 'my_value')
In this code snippet, the get_next_shard
function maintains a global variable current_shard_index
to keep track of the current shard. It then increments the index and returns the corresponding Redis instance, ensuring a round-robin distribution of requests.
Code Snippet: Data Replication in Redis Sharding
Data replication is crucial for ensuring fault tolerance and high availability in Redis Sharding. Here is an example code snippet demonstrating data replication using the Redis Sentinel feature:
import redis sentinel = redis.RedisSentinel('mymaster', sentinel=[('sentinel1.example.com', 26379), ('sentinel2.example.com', 26379), ('sentinel3.example.com', 26379)]) def get_master_redis(): return sentinel.master_for('mymaster') def get_slave_redis(): return sentinel.slave_for('mymaster') # Example usage master = get_master_redis() slave = get_slave_redis() master.set('my_key', 'my_value') value = slave.get('my_key')
In this code snippet, we use the Redis Sentinel feature to connect to the master and slave Redis instances. The get_master_redis
function returns a Redis instance connected to the master, while the get_slave_redis
function returns a Redis instance connected to a slave. This allows for read and write operations to be distributed between the master and slave nodes, providing fault tolerance and load balancing.
Related Article: How to Use Redis with Django Applications
Advanced Technique: Consistent Hashing in Redis Sharding
Consistent Hashing is an advanced technique used in Redis Sharding to distribute data evenly across shards while minimizing the amount of data movement when the number of shards changes. It achieves this by mapping keys to a ring-shaped hash space and assigning shards to different points on the ring.
Here is an example code snippet demonstrating consistent hashing in Redis Sharding:
import hashlib class ConsistentHashing: def __init__(self, shards): self.shards = shards self.ring = {} for shard in shards: for i in range(self.get_virtual_nodes_count(shard)): virtual_node = self.get_virtual_node(shard, i) hash_value = self.get_hash_value(virtual_node) self.ring[hash_value] = shard def get_virtual_nodes_count(self, shard): return 100 # Number of virtual nodes per shard def get_virtual_node(self, shard, index): return f"{shard}:{index}" def get_hash_value(self, node): return int(hashlib.md5(node.encode()).hexdigest(), 16) def get_shard(self, key): hash_value = self.get_hash_value(key) sorted_keys = sorted(self.ring.keys()) for ring_key in sorted_keys: if hash_value <= ring_key: return self.ring[ring_key] return self.ring[sorted_keys[0]] # Example usage shards = ['shard1', 'shard2', 'shard3'] hashing = ConsistentHashing(shards) shard = hashing.get_shard('my_key') shard.set('my_key', 'my_value')
In this code snippet, the ConsistentHashing
class implements consistent hashing by creating virtual nodes for each shard and mapping them to points on the hash ring. The get_shard
method calculates the hash value for the given key and finds the corresponding shard based on the closest point on the ring.
Advanced Technique: Data Partitioning in Redis Sharding
Data partitioning is an advanced technique used in Redis Sharding to divide the data into smaller partitions, allowing for more efficient data retrieval and distribution. Different partitioning strategies can be employed based on the specific requirements of the application.
One common partitioning strategy is range partitioning, where data is divided based on a specific range of keys. Another strategy is hash partitioning, where the hash value of the key is used to determine the partition. Each partition can then be assigned to a different shard.
Here is an example code snippet demonstrating data partitioning in Redis Sharding using range partitioning:
import redis shards = [ redis.Redis(host='shard1.example.com', port=6379), redis.Redis(host='shard2.example.com', port=6379), ] def get_shard(key): if key < 1000: return shards[0] else: return shards[1] # Example usage shard = get_shard('my_key') shard.set('my_key', 'my_value')
In this code snippet, the get_shard
function partitions the data based on the range of keys. Keys below 1000 are assigned to the first shard, while keys greater than or equal to 1000 are assigned to the second shard.
Advanced Technique: Failover Mechanisms in Redis Sharding
Failover mechanisms are crucial in Redis Sharding to ensure high availability and fault tolerance. When a shard becomes unavailable due to network issues or hardware failures, failover mechanisms automatically promote a replica as the new master to ensure uninterrupted service.
Redis Sentinel is a built-in failover mechanism in Redis that monitors the health of the master and slave nodes. When a master node fails, Sentinel selects a new master from the available replicas and reconfigures the cluster accordingly.
Related Article: Tutorial on Installing and Using redis-cli with Redis
Advanced Technique: Scaling Redis Sharding
Scaling Redis Sharding involves adding or removing shards to accommodate the growing or shrinking data volume. When scaling up, new shards can be added to the cluster, and incoming data can be distributed across the expanded set of shards. When scaling down, data can be migrated from the removed shards to the remaining shards.
To scale Redis Sharding, it is important to carefully plan the addition or removal of shards to minimize data movement and ensure balanced data distribution. This can be achieved by using techniques like consistent hashing and range partitioning.
Use Case: Redis Sharding for High Traffic Applications
Redis Sharding is particularly beneficial for high traffic applications that require fast data retrieval and write operations. By distributing the data across multiple shards, Redis Sharding allows for parallel processing and improved performance.
For example, in a social media application, Redis Sharding can be used to distribute user profiles, posts, and interactions across multiple shards. This enables efficient retrieval of user-related data and ensures that the system can handle a large number of concurrent requests.
Use Case: Redis Sharding for Real-time Data Processing
Redis Sharding is also well-suited for real-time data processing scenarios where low-latency access to data is crucial. By distributing the data across multiple shards, Redis Sharding allows for parallel processing of data streams, enabling real-time analytics and insights.
For instance, in a real-time analytics platform, Redis Sharding can be used to distribute incoming data streams across multiple shards. Each shard can then process the data independently and store the results for further analysis, providing real-time insights to users.
Related Article: Leveraging Redis for Caching Frequently Used Queries
Use Case: Redis Sharding for Caching in Distributed Systems
Caching is a common use case for Redis, and Redis Sharding can be employed to scale the caching infrastructure in distributed systems. By distributing the cache across multiple shards, Redis Sharding allows for increased cache capacity and improved cache hit rates.
In a distributed system with multiple application instances, each instance can have its own Redis shard for caching frequently accessed data. This reduces the load on individual Redis instances and improves overall system performance.
These are just a few examples of how Redis Sharding can be applied to various use cases, demonstrating its versatility and effectiveness in different scenarios.