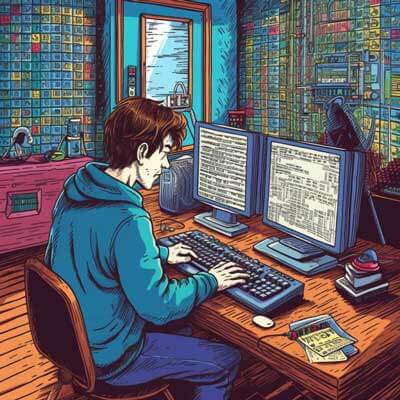
- Introduction to Redis Cluster
- Example 1: Creating a Redis Cluster
- Example 2: Accessing Redis Cluster
- Designing a Redis Cluster on Linux
- Subsection 1: Determining the Number of Nodes
- Subsection 2: Data Sharding
- Setting up a Redis Cluster on Linux
- Subsection 1: Installing Redis
- Subsection 2: Configuring Redis Cluster
- Best Practices for Redis Cluster Configuration
- Subsection 1: Enabling Persistence
- Subsection 2: Monitoring Redis Cluster
- Real World Examples of Redis Cluster Usage
- Subsection 1: Caching with Redis Cluster
- Subsection 2: Real-time Analytics with Redis Cluster
- Performance Considerations for Redis Cluster
- Subsection 1: Key Design Considerations
- Subsection 2: Pipeline and Transactions
- Code Snippet Ideas for Redis Cluster
- Subsection 1: Inserting Data into Redis Cluster
- Subsection 2: Performing Transactions in Redis Cluster
- Error Handling in Redis Cluster
- Subsection 1: Connection Errors
- Subsection 2: Data Integrity Errors
- Advanced Techniques for Redis Cluster Configuration
- Subsection 1: Cluster Node Configuration
- Subsection 2: Adding and Removing Nodes
Introduction to Redis Cluster
Redis Cluster is a distributed implementation of Redis that provides high availability and scalability for your Redis database. It allows you to partition your data across multiple nodes, ensuring that even if some nodes fail, your data remains accessible. Redis Cluster also offers automatic data sharding and master-slave replication, making it a robust solution for handling large datasets.
Related Article: Tutorial on Installing and Using redis-cli with Redis
Example 1: Creating a Redis Cluster
To create a Redis Cluster, you first need to install Redis on multiple nodes in your Linux environment. Once installed, you can use the redis-trib.rb
utility to initialize and configure the cluster.
Here’s an example of how to create a Redis Cluster with 6 nodes:
$ redis-trib.rb create --replicas 1 127.0.0.1:7000 127.0.0.1:7001 \ 127.0.0.1:7002 127.0.0.1:7003 127.0.0.1:7004 127.0.0.1:7005
This command creates a cluster with 6 nodes, where each node has one replica. The 127.0.0.1:7000
to 127.0.0.1:7005
addresses represent the IP and port of each Redis instance.
Example 2: Accessing Redis Cluster
Once your Redis Cluster is up and running, you can connect to it using a Redis client library or the Redis CLI. The Redis client library automatically handles the redirection and distribution of commands across the cluster.
Here’s an example of connecting to a Redis Cluster using the Redis CLI:
$ redis-cli -c -h 127.0.0.1 -p 7000
The -c
flag tells the Redis CLI to enable cluster mode, allowing you to execute commands that require key distribution. The -h
and -p
options specify the IP and port of any node in the cluster.
Designing a Redis Cluster on Linux
Designing a Redis Cluster involves careful consideration of factors such as the number of nodes, data sharding, and fault tolerance. In this chapter, we will explore the key principles and best practices for designing a Redis Cluster on Linux.
Related Article: Redis vs MongoDB: A Detailed Comparison
Subsection 1: Determining the Number of Nodes
To ensure high availability and performance, it is crucial to determine the optimal number of nodes for your Redis Cluster. The number of nodes depends on factors such as the size of your dataset, expected load, and fault tolerance requirements.
One common approach is to use at least three master nodes and one or more replicas for each master. This setup provides fault tolerance, as replicas can take over in case of master node failures.
Subsection 2: Data Sharding
Data sharding is the process of distributing data across multiple nodes in a Redis Cluster. It allows for parallel processing and improves overall performance. Redis Cluster uses a technique called consistent hashing to determine which node stores a specific key.
To shard your data effectively, you can use a hashing algorithm to map keys to specific slots within the Redis Cluster. This ensures that each key is stored on a specific node, making data retrieval efficient.
Setting up a Redis Cluster on Linux
Setting up a Redis Cluster on Linux involves installing Redis on multiple nodes and configuring them to work together. In this chapter, we will guide you through the step-by-step process of setting up a Redis Cluster on a Linux environment.
Related Article: Tutorial on Redis Queue Implementation
Subsection 1: Installing Redis
To get started, you need to install Redis on each node that will be part of the cluster. You can download the latest stable version of Redis from the official website or use your package manager to install it.
On Ubuntu, you can install Redis using the following command:
$ sudo apt-get update $ sudo apt-get install redis-server
Subsection 2: Configuring Redis Cluster
Once Redis is installed on all nodes, you need to configure each instance to enable clustering. Open the Redis configuration file (redis.conf
) using a text editor and make the following changes:
– Set the cluster-enabled
configuration option to yes
.
– Specify a unique cluster-node-name
for each node.
– Uncomment and set the bind
configuration option to the IP address of the current node.
– Uncomment and set the port
configuration option to the desired port number.
After making these changes, save the configuration file and restart Redis on each node.
Best Practices for Redis Cluster Configuration
Configuring a Redis Cluster involves making decisions that impact performance, scalability, and data integrity. In this chapter, we will discuss some best practices to consider when configuring your Redis Cluster.
Related Article: Tutorial: Installing Redis on Ubuntu
Subsection 1: Enabling Persistence
To ensure data durability, it is recommended to enable persistence in your Redis Cluster. Redis supports two persistence options: RDB snapshots and AOF logs.
RDB snapshots create periodic snapshots of your dataset, which can be used to restore data in case of failures. AOF logs, on the other hand, log every write operation, allowing for point-in-time recovery.
To enable RDB snapshots, set the save
configuration option in redis.conf
to a desired frequency. To enable AOF logs, set the appendonly
configuration option to yes
.
Subsection 2: Monitoring Redis Cluster
Monitoring the health and performance of your Redis Cluster is essential for detecting issues and optimizing its configuration. Redis provides a built-in monitoring tool called Redis Sentinel, which monitors Redis instances and performs automatic failover when necessary.
You can configure Redis Sentinel by creating a separate configuration file (sentinel.conf
) and specifying the monitored Redis instances. Start the Sentinel process using the redis-sentinel
command, passing the configuration file as an argument.
Real World Examples of Redis Cluster Usage
Redis Cluster has a wide range of use cases in real-world applications. In this chapter, we will explore some practical examples of how Redis Cluster can be used to solve common problems.
Related Article: Tutorial: Comparing Kafka vs Redis
Subsection 1: Caching with Redis Cluster
One of the most common use cases for Redis Cluster is caching. By leveraging Redis’s in-memory data storage and fast access times, you can significantly improve the performance of your application.
For example, you can use Redis Cluster to cache frequently accessed database queries or expensive computation results. By storing the results in Redis, subsequent requests can be served directly from the cache, reducing the load on your backend systems.
Subsection 2: Real-time Analytics with Redis Cluster
Redis Cluster’s fast read and write capabilities make it well-suited for real-time analytics applications. You can use Redis’s sorted sets and counters to track and analyze user behavior, perform time-series analysis, and generate real-time reports.
For instance, you can store user events in Redis Cluster and use Redis commands to calculate metrics such as page views, unique visitors, or conversion rates. This allows you to gain insights into user behavior and make data-driven decisions.
Performance Considerations for Redis Cluster
Optimizing performance is crucial when working with a Redis Cluster. In this chapter, we will explore some performance considerations and techniques to ensure your Redis Cluster operates efficiently.
Related Article: Exploring Alternatives to Redis
Subsection 1: Key Design Considerations
When designing your data model for Redis Cluster, it’s essential to consider the size and structure of your keys. Keys larger than 512 bytes can negatively impact performance, so it’s recommended to keep keys as short as possible.
Additionally, avoid using patterns like key prefixes or suffixes that could result in data skew. Uneven data distribution across nodes can lead to performance bottlenecks and increased network traffic.
Subsection 2: Pipeline and Transactions
To improve performance, Redis Cluster supports pipeline and transaction operations. Pipelining allows you to send multiple commands to the cluster in a single network round trip, reducing latency.
Transactions, on the other hand, allow you to group multiple commands into a single atomic operation. This ensures that either all commands in the transaction are executed or none of them, maintaining data consistency.
Code Snippet Ideas for Redis Cluster
Using code snippets, we will showcase some practical examples of how to interact with Redis Cluster programmatically. These snippets will cover common operations such as data insertion, retrieval, and manipulation.
Related Article: Tutorial on Implementing Redis Sharding
Subsection 1: Inserting Data into Redis Cluster
To insert data into Redis Cluster, you can use the Redis client library for your programming language of choice. Here’s an example using Python and the redis-py
library:
import redis # Connect to Redis Cluster redis_cluster = redis.RedisCluster( startup_nodes=[ {"host": "127.0.0.1", "port": 7000}, {"host": "127.0.0.1", "port": 7001}, {"host": "127.0.0.1", "port": 7002} ] ) # Set a key-value pair redis_cluster.set("mykey", "myvalue") # Get the value of a key value = redis_cluster.get("mykey") print(value)
Subsection 2: Performing Transactions in Redis Cluster
Redis Cluster supports transactions, allowing you to group multiple commands into a single atomic operation. Here’s an example of performing a transaction using the MULTI
, EXEC
, and DISCARD
commands in Python:
import redis # Connect to Redis Cluster redis_cluster = redis.RedisCluster( startup_nodes=[ {"host": "127.0.0.1", "port": 7000}, {"host": "127.0.0.1", "port": 7001}, {"host": "127.0.0.1", "port": 7002} ] ) # Begin the transaction pipeline = redis_cluster.pipeline() pipeline.multi() # Add commands to the transaction pipeline.set("key1", "value1") pipeline.set("key2", "value2") # Execute the transaction pipeline.execute() # Discard the transaction pipeline.discard()
Error Handling in Redis Cluster
Error handling is an essential aspect of working with Redis Cluster. In this chapter, we will explore common error scenarios and discuss techniques for handling errors effectively.
Related Article: Tutorial: Redis vs RabbitMQ Comparison
Subsection 1: Connection Errors
When working with Redis Cluster, connection errors can occur if a node becomes unavailable or there are network issues. To handle connection errors, it is recommended to implement retry logic with exponential backoff.
For example, in Python, you can use the redis.exceptions.ConnectionError
exception and a retry decorator to automatically retry failed operations:
import redis from redis.exceptions import ConnectionError from tenacity import retry, stop_after_attempt, wait_exponential @retry(stop=stop_after_attempt(3), wait=wait_exponential()) def perform_redis_operation(): try: # Perform Redis operation redis_cluster.set("key", "value") except ConnectionError as e: # Handle connection error print("Connection error:", e) # Call the function perform_redis_operation()
Subsection 2: Data Integrity Errors
Data integrity errors can occur when executing commands in Redis Cluster. For example, if a command is executed on a replica node that has not yet received the latest data from the master, an error can occur.
To handle data integrity errors, you can catch specific exceptions like redis.exceptions.ResponseError
and handle them accordingly. It’s important to analyze the specific error message to determine the appropriate action.
Advanced Techniques for Redis Cluster Configuration
Redis Cluster offers advanced configuration options that allow you to fine-tune its behavior and optimize performance. In this chapter, we will explore some advanced techniques for configuring Redis Cluster.
Related Article: Tutorial on Integrating Redis with Spring Boot
Subsection 1: Cluster Node Configuration
Redis Cluster provides several configuration options that allow you to customize the behavior of individual nodes. For example, you can set the cluster-node-timeout
configuration option to specify the timeout value for cluster nodes.
Additionally, you can configure specific nodes to have different roles, such as master or slave. This allows you to control the distribution and replication of data within the cluster.
Subsection 2: Adding and Removing Nodes
Redis Cluster allows you to add or remove nodes dynamically, allowing for easy scaling and maintenance. To add a new node to the cluster, you can use the redis-trib.rb
utility with the add-node
command.
For example, to add a new node with the IP and port 127.0.0.1:7006
to an existing cluster, you can run the following command:
$ redis-trib.rb add-node 127.0.0.1:7006 127.0.0.1:7000
To remove a node from the cluster, you can use the remove-node
command. Be cautious when removing nodes, as it can impact the data distribution and availability of the cluster.
This concludes our tutorial on configuring Redis Cluster. We have covered the basics of Redis Cluster, designing and setting up a cluster, best practices, real-world examples, performance considerations, code snippets, error handling, and advanced configuration techniques. With this knowledge, you can confidently deploy and manage a Redis Cluster for your applications.