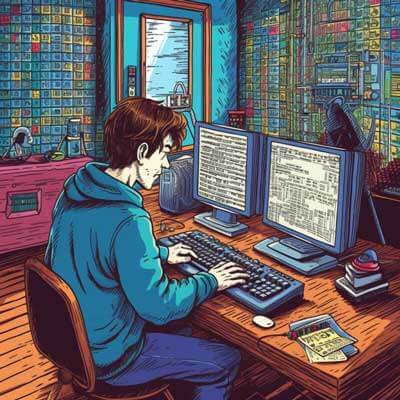
Table of Contents
Introduction to Redis
Redis is an open-source, in-memory data structure store that can be used as a database, cache, and message broker. It is known for its high performance, scalability, and versatility. Redis supports various data structures such as strings, hashes, lists, sets, and sorted sets, making it suitable for a wide range of use cases. In this chapter, we will explore the fundamentals of Redis and its key features.
Related Article: How to Configure a Redis Cluster
Example 1: Basic Redis Operations
To get started with Redis, you first need to install it on your system. Here's an example of how you can use Redis to store and retrieve data:
import redis# Connect to Redisr = redis.Redis(host='localhost', port=6379)# Set a key-value pairr.set('name', 'John')# Get the value for a keyname = r.get('name')print(name.decode())# Increment a counterr.incr('counter')# Get the current value of the countercounter = r.get('counter')print(counter.decode())
In this example, we connect to a Redis server running on the local machine, set a key-value pair ('name' and 'John'), retrieve the value associated with the 'name' key, increment a counter, and retrieve its current value.
Example 2: Redis Pub/Sub Messaging
Redis also supports a publish/subscribe messaging paradigm, allowing multiple clients to communicate asynchronously. Here's an example of how you can use Redis pub/sub:
import redis# Connect to Redisr = redis.Redis(host='localhost', port=6379)# Create a publisherpubsub = r.pubsub()# Subscribe to a channelpubsub.subscribe('channel')# Publish a messager.publish('channel', 'Hello, subscribers!')# Process incoming messagesfor message in pubsub.listen(): print(message['data'].decode())
In this example, we create a publisher that subscribes to a channel named 'channel'. We then publish a message to the channel and process incoming messages using a loop. This simple pub/sub mechanism can be used to implement real-time messaging systems, chat applications, and more.
Introduction to Docker Compose
Docker Compose is a tool that allows you to define and run multi-container Docker applications. It simplifies the process of managing and orchestrating multiple containers by using a declarative YAML file. With Docker Compose, you can define the services, networks, and volumes required by your application and start them with a single command. In this chapter, we will explore the basics of Docker Compose and its key features.
Related Article: How to Use Redis Queue in Redis
Example 1: Docker Compose Configuration
To get started with Docker Compose, you need to create a YAML file called 'docker-compose.yml' in your project directory. Here's an example of a basic Docker Compose configuration:
version: '3'services: redis: image: redis:latest ports: - 6379:6379
In this example, we define a service named 'redis' using the official Redis Docker image. We map the container's port 6379 to the host's port 6379, allowing access to the Redis server from outside the Docker network. With this configuration, we can start a Redis container by running the command docker-compose up
.
Example 2: Docker Compose Networking
Docker Compose provides networking capabilities to allow containers to communicate with each other. Here's an example of how you can define a network in Docker Compose:
version: '3'services: redis: image: redis:latest networks: - mynetworknetworks: mynetwork: driver: bridge
In this example, we define a service named 'redis' and a network named 'mynetwork'. The 'mynetwork' network is a bridge network, which allows containers to communicate using their names as hostnames. By default, Docker Compose creates a default network for all services, but you can also define custom networks to isolate containers or group them based on functionality.
Setting up Redis with Docker Compose
To set up Redis with Docker Compose, you need to define a Redis service in your Docker Compose configuration file. You can use the official Redis Docker image and customize the configuration as needed. Here's an example of how you can set up Redis with Docker Compose:
version: '3'services: redis: image: redis:latest ports: - 6379:6379
In this example, we define a Redis service using the latest Redis Docker image. We map the container's port 6379 to the host's port 6379, allowing access to the Redis server from outside the Docker network. With this configuration, you can start a Redis container by running the command docker-compose up
.
Running Redis Containers with Docker Compose
Once you have set up Redis with Docker Compose, you can start and manage Redis containers using Docker Compose commands. Here are some commonly used commands for running Redis containers with Docker Compose:
- docker-compose up
: Starts the Redis container(s) defined in the Docker Compose configuration file.
- docker-compose up -d
: Starts the Redis container(s) in detached mode.
- docker-compose down
: Stops and removes the Redis container(s) defined in the Docker Compose configuration file.
- docker-compose restart
: Restarts the Redis container(s).
- docker-compose stop
: Stops the Redis container(s) without removing them.
- docker-compose start
: Starts the Redis container(s) that were previously stopped.
These commands allow you to easily start, stop, and manage your Redis containers using Docker Compose.
Related Article: Tutorial on Redis Sentinel: A Deep Look
Managing Redis Containers with Docker Compose
Docker Compose provides several commands for managing Redis containers and their associated resources. Here are some commonly used commands:
- docker-compose ps
: Lists the running containers defined in the Docker Compose configuration file.
- docker-compose logs
: Displays the logs of the Redis container(s).
- docker-compose exec <service_name> <command>
: Executes a command inside the Redis container(s).
- docker-compose build
: Builds or rebuilds the Redis image(s) specified in the Docker Compose configuration file.
- docker-compose pull
: Pulls the latest version of the Redis image(s) specified in the Docker Compose configuration file.
- docker-compose push
: Pushes the Redis image(s) specified in the Docker Compose configuration file to a container registry.
These commands provide essential functionality for managing and troubleshooting Redis containers with Docker Compose.
Scaling Redis with Docker Compose
One of the advantages of using Docker Compose is the ability to scale services easily. Scaling Redis with Docker Compose allows you to increase the number of Redis containers to handle higher loads or distribute the workload across multiple instances. Here's an example of how you can scale Redis with Docker Compose:
version: '3'services: redis: image: redis:latest ports: - 6379:6379 deploy: replicas: 3
In this example, we set the 'replicas' option to 3, indicating that we want to run three Redis containers. When you run the command docker-compose up
, Docker Compose will create three Redis containers, each running in its own isolated environment. This allows you to distribute the workload and improve the performance and availability of your Redis setup.
Use Cases for Redis with Docker Compose
Redis with Docker Compose can be used in a variety of use cases. Some common use cases include:
- Caching: Redis can be used as a high-performance cache for frequently accessed data, improving the response time of your applications.
- Session storage: Redis can be used to store session data, allowing you to scale your application horizontally without relying on sticky sessions.
- Pub/Sub messaging: Redis' publish/subscribe mechanism can be used to implement real-time messaging systems, chat applications, and event-driven architectures.
- Job queues: Redis can be used as a backend for task queues, allowing you to process background jobs asynchronously and distribute the workload across multiple workers.
These are just a few examples of how Redis with Docker Compose can be used to enhance your applications.
Best Practices for Redis with Docker Compose
When using Redis with Docker Compose, it's important to follow some best practices to ensure the reliability, performance, and security of your Redis containers. Here are some best practices to consider:
- Use the official Redis Docker image: The official Redis Docker image is maintained by the Redis community and provides a reliable and secure base for your Redis containers.
- Persist data outside the container: Redis is an in-memory data store, so it's important to persist your data outside the container to avoid data loss. You can use Docker volumes or bind mounts to store Redis data on the host machine.
- Use Redis configuration options: Redis provides various configuration options to optimize performance and security. You can customize the Redis configuration by providing a Redis configuration file or passing configuration options as environment variables in the Docker Compose configuration file.
- Monitor Redis metrics: Monitoring Redis metrics such as memory usage, CPU usage, and throughput can help you identify performance bottlenecks and optimize your Redis setup. You can use tools like Prometheus and Grafana to visualize and analyze Redis metrics.
- Secure Redis: Redis does not have built-in authentication or encryption, so it's important to secure your Redis containers. You can use tools like Redis ACL (Access Control List) or external solutions like stunnel to secure your Redis setup.
Related Article: Tutorial on Integrating Redis with Spring Boot
Real World Examples of Redis with Docker Compose
Redis with Docker Compose is widely used in production environments to power various applications and services. Here are some real-world examples of how Redis with Docker Compose is used:
- E-commerce applications: Redis is used to cache product catalog data, session data, and user preferences, improving the performance and scalability of e-commerce platforms.
- Real-time analytics: Redis is used to store and analyze real-time data such as user events, website traffic, and social media feeds, allowing businesses to make data-driven decisions.
- Chat applications: Redis' pub/sub mechanism is used to implement real-time chat features in messaging applications, enabling instant messaging and group chat functionality.
- Task queues: Redis is used as a backend for task queues, allowing background jobs to be processed asynchronously and distributed across multiple workers.
- Gaming platforms: Redis is used to store game state, leaderboard data, and real-time game events, providing a fast and scalable infrastructure for multiplayer games.
These examples showcase the versatility and power of Redis with Docker Compose in real-world scenarios.
Performance Considerations for Redis with Docker Compose
When running Redis with Docker Compose, there are several performance considerations to keep in mind to ensure optimal performance. Here are some key factors to consider:
- Memory allocation: Redis is an in-memory data store, so it's essential to allocate sufficient memory to accommodate your data set. You can configure the maximum memory limit for Redis by setting the maxmemory
option in the Redis configuration.
- Persistent storage: If you need to persist your Redis data, consider using Redis' built-in persistence options such as RDB snapshots or AOF (Append-Only File). These options allow you to save your data to disk and recover it in case of a restart or failure.
- Network latency: When running Redis containers on multiple hosts or in a distributed environment, network latency can impact performance. Consider optimizing network communication between containers by using Docker overlay networks or other networking solutions.
- Redis Cluster: If you need high availability and scalability, consider using Redis Cluster, which allows you to distribute your data across multiple Redis instances. Docker Compose can be used to deploy and manage Redis Cluster configurations.
- Benchmarking and profiling: Regularly benchmark and profile your Redis setup to identify performance bottlenecks and optimize your configuration. Tools like RedisBench and Redis-Stat can help you measure the performance of your Redis containers.
Advanced Techniques for Redis with Docker Compose
Redis with Docker Compose offers various advanced techniques to enhance the functionality and scalability of your Redis setup. Here are some advanced techniques to consider:
- Redis Sentinel: Redis Sentinel is a high-availability solution for Redis that provides automatic failover and monitoring. You can deploy a Redis Sentinel setup using Docker Compose to ensure the availability and reliability of your Redis containers.
- Redis Streams: Redis Streams is a data structure for log-like messaging, allowing you to store and process streams of messages. You can leverage Redis Streams in your Docker Compose setup to build real-time data processing pipelines.
- Redis Lua scripting: Redis supports Lua scripting, allowing you to execute complex operations on the server side. You can use Lua scripting in combination with Docker Compose to implement custom logic and business rules in your Redis containers.
- Redis Modules: Redis Modules are add-ons that extend the functionality of Redis. They allow you to add new data types, commands, and functionalities to Redis. You can use Redis Modules in your Docker Compose setup to enhance the capabilities of your Redis containers.
These advanced techniques provide useful capabilities for building complex and scalable Redis setups with Docker Compose.
Code Snippet Ideas for Redis with Docker Compose
Here are some code snippet ideas to help you get started with Redis and Docker Compose:
1. Using Redis as a cache with Django:
from django.core.cache import cache# Set a value in the Redis cachecache.set('key', 'value')# Get a value from the Redis cachevalue = cache.get('key')print(value)
2. Using Redis pub/sub in a Node.js application:
const redis = require('redis');// Create a Redis clientconst client = redis.createClient();// Subscribe to a channelclient.subscribe('channel');// Publish a messageclient.publish('channel', 'Hello, subscribers!');// Process incoming messagesclient.on('message', (channel, message) => { console.log(message);});
These code snippets demonstrate how Redis can be integrated into different application frameworks and used for caching and real-time messaging.
Related Article: How to use Redis with Laravel and PHP
Error Handling in Redis with Docker Compose
When working with Redis containers in a Docker Compose setup, it's important to handle errors effectively to ensure the reliability and stability of your Redis setup. Here are some key considerations for error handling:
- Connection errors: Handle connection errors when connecting to Redis from your applications. Retry mechanisms and connection pools can help mitigate connection-related issues.
- Redis command errors: Redis commands can fail due to various reasons such as incorrect syntax, exceeded memory limits, or expired keys. Implement proper error handling and logging in your applications to handle command failures gracefully.
- Container failures: Monitor the health and availability of your Redis containers using Docker Compose health checks or external monitoring tools. Implement automated recovery mechanisms to handle container failures and ensure high availability.