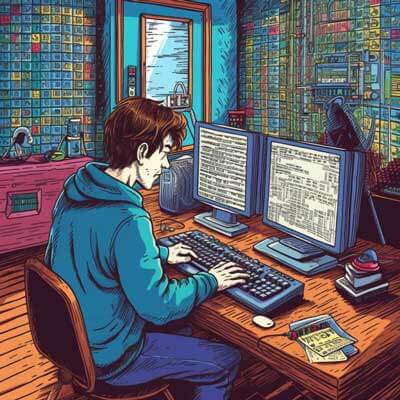
- Measuring Redis Query Rate in Sidekiq
- Average Number of Queries per Second in Sidekiq
- Optimizing Redis Query Performance in Sidekiq
- Factors Affecting Query Speed in Sidekiq
- Increasing Query Throughput in Sidekiq
- Tools for Monitoring Redis Query Rate in Sidekiq
- Understanding a Good Redis Query Rate in Sidekiq
- Impact of Sidekiq Workers on Redis Query Rate
- Limiting Redis Query Rate in Sidekiq
- Troubleshooting Slow Redis Queries in Sidekiq
Measuring Redis Query Rate in Sidekiq
Redis is an in-memory data structure store that can be used as a database, cache, or message broker. It is commonly used in applications that require high-performance data storage and retrieval. Sidekiq is a popular background processing framework for Ruby that allows developers to offload time-consuming tasks to a separate process or worker.
When working with Sidekiq and Redis, it is essential to monitor the query rate to ensure optimal performance and identify any potential bottlenecks. Measuring the Redis query rate in Sidekiq can be done by tracking the number of queries executed per second.
To measure the Redis query rate in Sidekiq, you can use the Sidekiq web UI or custom monitoring tools. The Sidekiq web UI provides a dashboard that displays real-time information about the number of queries executed per second, among other metrics.
Here’s an example of how you can use the Sidekiq web UI to measure the Redis query rate:
1. Start by running Sidekiq in your Ruby application.
2. Access the Sidekiq web UI by navigating to the /sidekiq
path in your application’s URL, for example, http://localhost:3000/sidekiq
.
3. In the Sidekiq web UI, you will find a section called “Redis Info” that provides detailed information about the Redis server, including the number of queries executed per second.
Here’s an example of the Redis Info section in the Sidekiq web UI:
Redis Info ----------- Connected clients: 10 Total queries per second: 500
In this example, the Redis server is handling 500 queries per second. Monitoring this metric can help you identify if the query rate is within acceptable limits and detect any sudden spikes or drops in performance.
Another option for measuring the Redis query rate in Sidekiq is by using custom monitoring tools. These tools can provide more flexibility and allow you to track additional metrics specific to your application’s requirements.
For example, you can use the redis-rb
gem to interact with Redis programmatically and retrieve information about the query rate. Here’s an example code snippet that demonstrates how to measure the query rate using the redis-rb
gem:
require 'redis' redis = Redis.new # Get the number of queries executed per second info = redis.info query_rate = info['instantaneous_ops_per_sec'] puts "Query rate: #{query_rate} queries per second"
In this example, we create a new Redis client using the redis-rb
gem and retrieve the instantaneous_ops_per_sec
metric from the Redis server’s INFO
command. This metric represents the number of commands processed per second by the Redis server.
Related Article: Tutorial: Setting Up Redis Using Docker Compose
Average Number of Queries per Second in Sidekiq
The average number of queries per second in Sidekiq refers to the average rate at which Redis queries are executed by the Sidekiq workers. This metric is crucial for understanding the workload on the Redis server and ensuring optimal performance.
To calculate the average number of queries per second in Sidekiq, you need to collect the query rate data over a specific period and then calculate the average value.
Here’s an example of how you can calculate the average number of queries per second in Sidekiq:
1. Use a monitoring tool or the Sidekiq web UI to collect the number of queries executed per second at regular intervals, for example, every 5 minutes.
2. Record the query rate values over a specific duration, such as 1 hour.
3. Sum up all the query rate values recorded during the duration.
4. Divide the sum by the number of data points recorded to calculate the average.
Here’s an example code snippet that demonstrates how to calculate the average number of queries per second in Sidekiq using Ruby:
query_rates = [500, 600, 700, 800, 900] # Example query rate values in queries per second # Calculate the sum of query rates sum = query_rates.sum # Calculate the average query rate average = sum / query_rates.length puts "Average query rate: #{average} queries per second"
In this example, we have an array query_rates
that contains the query rate values recorded at regular intervals. We calculate the sum of all the query rates and then divide it by the number of data points to obtain the average query rate.
Monitoring the average number of queries per second in Sidekiq can help you identify any trends or patterns in the workload and make informed decisions to optimize the performance of your application.
Optimizing Redis Query Performance in Sidekiq
Optimizing Redis query performance in Sidekiq is crucial for ensuring efficient and responsive background processing. By optimizing the way Sidekiq interacts with Redis, you can reduce latency, improve throughput, and minimize the load on the Redis server.
Here are some strategies for optimizing Redis query performance in Sidekiq:
1. Minimize the number of Redis queries: Each Redis query adds overhead, so reducing the number of queries can significantly improve performance. Instead of making multiple queries for related data, consider using Redis data structures like hashes, lists, and sets to store and retrieve complex objects in a single query.
2. Batch Redis queries: Instead of making individual queries for each operation, batch multiple operations into a single Redis pipeline. A pipeline allows you to send multiple commands to Redis in one go, reducing round-trip time and improving overall performance.
Here’s an example code snippet that demonstrates how to use a Redis pipeline in Sidekiq:
require 'redis' redis = Redis.new redis.pipelined do redis.incr('counter') redis.set('name', 'John Doe') end
In this example, we use the pipelined
method provided by the redis-rb
gem to batch multiple Redis commands (incr
and set
) into a single pipeline. This reduces the number of network round-trips and improves performance.
3. Leverage Redis caching: Redis can be used as a cache to store frequently accessed data and reduce the need for expensive database queries. By caching the results of computationally expensive operations or frequently accessed data, you can improve response times and reduce the load on the Redis server.
Here’s an example code snippet that demonstrates how to use Redis as a cache in Sidekiq:
require 'redis' require 'json' redis = Redis.new def get_user(id) # Check if the user data is cached in Redis user_data = redis.get("user:#{id}") if user_data # User data found in cache, parse it and return JSON.parse(user_data) else # User data not found in cache, fetch from the database user = User.find(id) # Store the user data in Redis cache redis.set("user:#{id}", user.to_json) user end end
In this example, we define a get_user
method that checks if the user data is cached in Redis using the user’s ID as the cache key. If the data is found in the cache, it is parsed and returned. Otherwise, the data is fetched from the database, stored in the Redis cache, and returned.
Factors Affecting Query Speed in Sidekiq
The speed at which Sidekiq executes Redis queries can be influenced by various factors. Understanding these factors can help you identify potential bottlenecks and optimize the query speed in Sidekiq.
Here are some factors that can affect the query speed in Sidekiq:
1. Network latency: The network latency between the Sidekiq worker and the Redis server can impact the query speed. If the network latency is high, it can introduce delays in sending and receiving Redis commands, resulting in slower query execution. To minimize network latency, ensure that the Sidekiq worker and the Redis server are deployed within the same network or data center.
2. Redis server configuration: The configuration settings of the Redis server can affect the query speed. Settings like maxclients
, maxmemory
, and maxmemory-policy
can impact the Redis server’s ability to handle concurrent connections, memory allocation, and eviction policies. Optimizing these configuration settings based on your application’s requirements can improve query speed.
3. Redis command complexity: The complexity of the Redis commands executed by Sidekiq can impact the query speed. Some Redis commands, like SORT
or SCAN
, can be computationally expensive and may take longer to execute compared to simpler commands like GET
or SET
. If you are experiencing slow query speed, review the complexity of the Redis commands used in your Sidekiq workers and consider optimizing them if necessary.
4. Sidekiq worker concurrency: The concurrency level of Sidekiq workers can affect the query speed. If you have a high number of Sidekiq workers processing Redis queries concurrently, it can put a strain on the Redis server and impact query performance. Adjusting the concurrency level based on the available system resources and Redis server capacity can help optimize query speed.
5. Redis server load: The overall load on the Redis server can impact query speed. If the Redis server is under heavy load due to a high number of queries or other factors, it may not be able to respond quickly to Sidekiq queries, resulting in slower query speed. Monitoring the Redis server load and optimizing the workload can help improve query speed.
Related Article: Tutorial: Redis vs RabbitMQ Comparison
Increasing Query Throughput in Sidekiq
Increasing query throughput in Sidekiq involves optimizing the system to handle a higher number of Redis queries per second. By improving the efficiency of query execution and maximizing the resources available, you can achieve higher query throughput and process more tasks in a given timeframe.
Here are some strategies to increase query throughput in Sidekiq:
1. Optimize Sidekiq worker concurrency: Increasing the concurrency level of Sidekiq workers can help process more Redis queries simultaneously. By allowing more workers to operate concurrently, you can distribute the workload and handle a higher number of queries per second. However, it’s important to consider the available system resources and the capacity of the Redis server to avoid overloading the system.
2. Implement connection pooling: Connection pooling allows you to reuse established connections to the Redis server instead of creating a new connection for each query. By reusing connections, you can reduce the overhead of establishing new connections and improve query throughput. There are various connection pooling libraries available for different programming languages, such as ConnectionPool
for Ruby.
Here’s an example code snippet that demonstrates how to use connection pooling with Sidekiq and Redis in Ruby:
require 'connection_pool' require 'redis' # Create a connection pool with a maximum of 10 connections redis_pool = ConnectionPool.new(size: 10) { Redis.new } # Sidekiq worker using connection pool class MyWorker include Sidekiq::Worker def perform redis_pool.with do |redis| # Perform Redis queries using the connection from the pool redis.set('key', 'value') redis.get('key') end end end
In this example, we create a connection pool redis_pool
with a maximum of 10 connections using the ConnectionPool
gem. Inside the Sidekiq worker’s perform
method, we use the with
method provided by the connection pool to acquire a connection from the pool and execute Redis queries.
3. Enable Redis pipelining: Redis pipelining allows you to send multiple commands to the Redis server in one go, reducing the round-trip time for each query and improving query throughput. By batching multiple queries into a single pipeline, you can achieve higher query throughput.
Here’s an example code snippet that demonstrates how to enable Redis pipelining in Sidekiq:
require 'redis' redis = Redis.new redis.pipelined do 100.times do |i| redis.set("key#{i}", "value#{i}") end end
In this example, we use the pipelined
method provided by the redis-rb
gem to enable Redis pipelining. Inside the block, we execute multiple set
commands in a loop, batching them into a single pipeline.
4. Scale the Redis infrastructure: If your application requires a significantly higher query throughput, you may need to scale your Redis infrastructure. This can involve deploying multiple Redis instances in a cluster or using a Redis cluster solution that provides sharding and replication capabilities. Scaling the Redis infrastructure can distribute the query workload and improve overall query throughput.
Tools for Monitoring Redis Query Rate in Sidekiq
Monitoring the Redis query rate in Sidekiq is essential for ensuring optimal performance and identifying any potential issues. Several tools can help you monitor the query rate and gain insights into the workload on the Redis server.
Here are some tools for monitoring the Redis query rate in Sidekiq:
1. Sidekiq Web UI: The Sidekiq web UI provides a built-in dashboard that displays real-time information about the number of queries executed per second, among other metrics. You can access the Sidekiq web UI by navigating to the /sidekiq
path in your application’s URL. The Redis Info section in the Sidekiq web UI provides detailed information about the Redis server, including the query rate.
2. Redis INFO command: Redis provides an INFO
command that returns information about the Redis server, including metrics related to the query rate. You can use the redis-rb
gem or any Redis client library to execute the INFO
command programmatically and retrieve the query rate metric.
Here’s an example code snippet that demonstrates how to use the INFO
command to monitor the query rate in Sidekiq using Ruby:
require 'redis' redis = Redis.new # Get the Redis server information info = redis.info # Retrieve the query rate metric query_rate = info['instantaneous_ops_per_sec'] puts "Query rate: #{query_rate} queries per second"
In this example, we use the redis-rb
gem to create a new Redis client and execute the INFO
command to retrieve the Redis server information. We then extract the instantaneous_ops_per_sec
metric from the server info to obtain the query rate.
3. Custom monitoring tools: You can also build custom monitoring tools that collect and analyze data specific to your application’s requirements. These tools can provide more flexibility and allow you to track additional metrics beyond the query rate. You can use programming languages like Ruby, Python, or Go to create custom monitoring scripts or integrate with existing monitoring frameworks like Prometheus or Grafana.
Understanding a Good Redis Query Rate in Sidekiq
Understanding what constitutes a good Redis query rate in Sidekiq is crucial for evaluating the performance of your application and ensuring optimal resource utilization. The ideal query rate can vary based on the specific requirements and workload of your application.
A good Redis query rate in Sidekiq depends on several factors, including the complexity of the queries, the number of Sidekiq workers, and the capacity of the Redis server. It is important to strike a balance between query throughput and resource consumption to ensure efficient background processing.
Here are some considerations to understand a good Redis query rate in Sidekiq:
1. Benchmarking: Benchmarking your application under different workloads can help you establish a baseline for the query rate. By simulating realistic scenarios and measuring the query rate, you can determine the upper limits of your application’s performance and identify any potential bottlenecks.
2. Application requirements: The query rate should align with the requirements and expected performance of your application. Consider factors such as response time, throughput, and the desired user experience when evaluating the query rate. A good query rate should meet these requirements while effectively utilizing the available resources.
3. Scalability: The query rate should be scalable to handle increasing workloads. As your application grows and the number of users or background processing tasks increases, the query rate should be able to scale accordingly. This may involve optimizing the Redis infrastructure, adjusting Sidekiq worker concurrency, or scaling the application horizontally.
4. Resource utilization: A good query rate should not overload the Redis server or consume excessive system resources. It is important to consider the capacity and limitations of the Redis server, as well as the available system resources, when evaluating the query rate. Monitoring resource usage metrics like CPU, memory, and network bandwidth can help determine if the query rate is within acceptable limits.
5. Stability and reliability: A good query rate should allow the application to operate in a stable and reliable manner. If the query rate is too high, it can lead to increased latency, response time degradation, or even system failures. It is important to ensure that the query rate does not compromise the stability and reliability of the application.
Related Article: Tutorial: Kafka vs Redis
Impact of Sidekiq Workers on Redis Query Rate
Sidekiq workers play a significant role in determining the Redis query rate in Sidekiq. The number of Sidekiq workers and their concurrency level can directly impact the query rate and overall performance of the application.
Here’s how Sidekiq workers can impact the Redis query rate in Sidekiq:
1. Concurrency level: The concurrency level of Sidekiq workers determines how many workers can execute Redis queries simultaneously. Increasing the concurrency level allows more workers to operate concurrently and process a higher number of queries per second. However, it’s important to consider the available system resources and the capacity of the Redis server. If the concurrency level is set too high, it can overload the Redis server and impact query performance.
Here’s an example code snippet that demonstrates how to configure the concurrency level in Sidekiq:
Sidekiq.configure_server do |config| config.options[:concurrency] = 10 # Set the concurrency level to 10 end
In this example, we use the configure_server
block provided by Sidekiq to set the concurrency level to 10. This means that 10 Sidekiq workers can execute Redis queries concurrently.
2. Queue distribution: Sidekiq workers are responsible for fetching and processing jobs from the Sidekiq queues. The distribution of jobs across workers can impact the query rate. If the workload is evenly distributed among the workers, it can ensure efficient utilization of resources and maximize the query rate. On the other hand, if some workers are idle while others are overloaded, it can lead to suboptimal performance.
Here’s an example code snippet that demonstrates how to distribute jobs across Sidekiq workers:
class MyWorker include Sidekiq::Worker sidekiq_options queue: 'default' # Set the queue for this worker end
In this example, we use the sidekiq_options
method provided by Sidekiq to set the queue for the MyWorker
class to default
. This ensures that jobs for this worker are fetched from the “default” queue.
3. Worker availability: The availability of Sidekiq workers can impact the query rate. If workers are frequently unavailable due to downtime, resource limitations, or other factors, it can result in slower query execution and reduced query rate. Ensuring the availability and health of Sidekiq workers is crucial for maintaining optimal performance.
Limiting Redis Query Rate in Sidekiq
Limiting the Redis query rate in Sidekiq can be necessary to prevent overwhelming the Redis server and ensure optimal performance. By imposing a rate limit on the number of Redis queries executed per second, you can control the workload and avoid overloading the system.
Here are some strategies for limiting the Redis query rate in Sidekiq:
1. Throttling Sidekiq workers: Throttling involves limiting the rate at which Sidekiq workers process Redis queries. By introducing a delay between queries or limiting the number of queries processed within a specific timeframe, you can control the query rate. This can be achieved by using libraries or techniques that provide rate-limiting capabilities, such as the sidekiq-throttler
gem for Ruby.
Here’s an example code snippet that demonstrates how to use the sidekiq-throttler
gem to limit the query rate in Sidekiq:
require 'sidekiq' require 'sidekiq/throttled' Sidekiq::Throttled.setup! class MyWorker include Sidekiq::Worker sidekiq_options :throttle => { :threshold => 10, :period => 1 } def perform # Perform Redis queries here end end
In this example, we use the sidekiq-throttler
gem to set up rate limiting for Sidekiq workers. By specifying the :throttle
option in the sidekiq_options
, we can define the maximum number of queries (:threshold
) allowed per second and the time period (:period
) over which the limit is enforced.
2. Implementing a Redis proxy: A Redis proxy can act as a middle layer between Sidekiq and the Redis server, allowing you to control the query rate. The proxy can enforce rate limits, perform query caching, and provide additional security measures. Examples of Redis proxy solutions include Twemproxy and MyRedis.
3. Redis cluster: If your application requires a higher query rate, you can consider using Redis clustering. Redis clustering allows you to distribute the workload across multiple Redis instances, enabling higher query throughput. By leveraging the built-in sharding and replication capabilities of Redis clustering, you can handle a larger number of queries per second.
Troubleshooting Slow Redis Queries in Sidekiq
Slow Redis queries in Sidekiq can impact the overall performance and responsiveness of your application. Troubleshooting and optimizing slow Redis queries is crucial for maintaining efficient background processing and delivering a seamless user experience.
Here are some steps to troubleshoot slow Redis queries in Sidekiq:
1. Monitor query response times: Start by monitoring the response times of the Redis queries executed by Sidekiq. This can help you identify if specific queries are consistently slow. You can use the Sidekiq web UI, custom monitoring tools, or Redis server logs to collect and analyze response time data.
2. Analyze query complexity: Evaluate the complexity of the Redis queries used in your Sidekiq workers. Some Redis commands, such as SORT
, SCAN
, or commands involving complex data structures, can be computationally expensive and result in slower query execution. Review the query logic and consider optimizing or simplifying complex queries if necessary.
3. Check Redis server performance: Slow Redis queries can be an indication of performance issues on the Redis server itself. Monitor the Redis server’s CPU, memory, and network usage to identify any resource bottlenecks. Adjusting the Redis server configuration, upgrading hardware, or scaling the Redis infrastructure can help alleviate performance issues.
4. Optimize Sidekiq worker concurrency: The concurrency level of Sidekiq workers can impact query performance. If the concurrency level is set too high, it can overload the Redis server and result in slower queries. Consider adjusting the concurrency level based on the available system resources and the capacity of the Redis server.
5. Use Redis pipeline or batch queries: Redis pipelining and batching can help improve query performance by reducing round-trip time and minimizing the overhead of establishing new connections. If you have multiple related Redis queries, consider using a pipeline or batching them into a single query to improve performance.
6. Implement Redis caching: Redis caching can help reduce the need for expensive database queries and improve query performance. By caching frequently accessed data or the results of computationally expensive operations, you can reduce the load on the Redis server and improve response times.
7. Review network connectivity: Slow network connectivity between Sidekiq and the Redis server can impact query performance. Ensure that the network connection is stable and free from congestion or latency issues. Consider deploying Sidekiq and Redis within the same data center or network to minimize network latency.
8. Profile and optimize code: Profile the code executed by Sidekiq workers to identify any performance bottlenecks. Use profiling tools to measure the execution time of different sections of code and identify areas that can be optimized. This can involve optimizing algorithm complexity, reducing unnecessary database or Redis queries, or improving overall code efficiency.