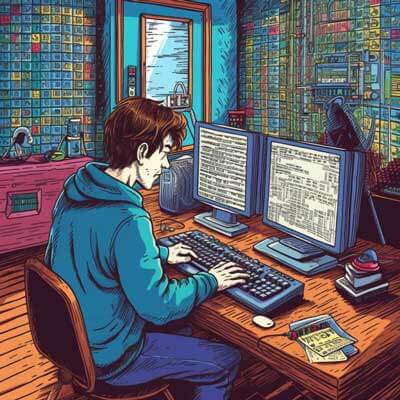
- Understanding Caching
- Exploring Frequently Used Queries
- Key-Value Stores in a Nutshell
- In-Memory Data Structure Stores: A Deep Dive
- An Overview of NoSQL Databases
- Redis and Performance Optimization
- Scalability Considerations with Redis
- Redis and Data Persistence
- How Redis Handles Caching
- Expiration
- Data Eviction Policies
- Pipeline and Batch Operations
- Leveraging Redis for Frequently Used Queries
- Redis as a Key-Value Store
- Redis as an In-Memory Data Structure Store
- Redis as a NoSQL Database
- Redis for Performance Improvement
- Redis for Scalability Enhancement
- Redis and Data Persistence Support
Understanding Caching
Caching is a technique used in software development to improve the performance and efficiency of applications. It involves storing frequently accessed data in a faster and more accessible location, such as memory, to reduce the need to fetch the data from the original data source repeatedly. This can significantly speed up the application’s response time and reduce the load on the underlying infrastructure.
Caching can be applied to various aspects of an application, including frequently used queries, computations, or even entire web pages. In this article, we will focus on caching frequently used queries and explore how Redis can be leveraged for this purpose.
Related Article: How to Configure a Redis Cluster
Exploring Frequently Used Queries
In many applications, a significant portion of the processing time is spent on executing database queries. These queries fetch data from a database based on certain criteria or conditions. However, not all queries are executed equally. Some queries may be executed frequently, while others are rarely used.
Frequently used queries are those that are executed repeatedly within a short span of time, often returning the same or similar results. Examples of frequently used queries include retrieving a user’s profile information, fetching the latest news articles, or retrieving product details for an e-commerce website.
When these frequently used queries are executed, they can put a strain on the database server, leading to increased response times and decreased overall performance of the application. Caching these queries can help alleviate this strain by storing the results in a cache and serving them directly from the cache instead of executing the query again.
Key-Value Stores in a Nutshell
Key-value stores are a type of database that stores data as a collection of key-value pairs. They are designed for fast and efficient data retrieval by using keys to access values directly, without the need for complex querying mechanisms. Key-value stores offer high performance and scalability, making them a popular choice for caching frequently used queries.
Redis is one such key-value store that is widely used for caching purposes. It provides a simple, yet useful, data model where data is stored as key-value pairs. Redis is an in-memory data structure store, meaning that the data is stored in memory for fast access. It also offers persistence options to ensure data durability.
In-Memory Data Structure Stores: A Deep Dive
In-memory data structure stores, such as Redis, are designed to store and manipulate data structures directly in memory. This allows for extremely fast data access and manipulation, making them ideal for caching frequently used queries.
Redis offers a wide range of data structures, including strings, lists, sets, sorted sets, and hashes. These data structures can be used to represent various types of data and enable efficient operations such as fetching, updating, and deleting data. For caching purposes, Redis’s string data type is commonly used to store the results of frequently used queries.
Let’s take a look at an example of caching a frequently used query using Redis:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) # Check if the query result is already cached cached_result = r.get('user_profile:123') if cached_result: # Return the cached result return cached_result else: # Execute the query to fetch the user's profile information result = db.execute_query('SELECT * FROM users WHERE id = 123') # Cache the query result for future use r.set('user_profile:123', result) # Return the query result return result
In the above example, we first check if the query result is already cached in Redis using the key 'user_profile:123'
. If the result is found in the cache, we return it directly. Otherwise, we execute the query to fetch the user’s profile information from the database, cache the result in Redis using the same key, and return the result.
Related Article: Tutorial on installing and using redis-cli in Redis
An Overview of NoSQL Databases
NoSQL databases are a type of database that deviate from the traditional relational database model. They are designed to handle large amounts of unstructured or semi-structured data, providing high scalability and performance. NoSQL databases come in various forms, including key-value stores, document stores, column stores, and graph databases.
Redis falls into the category of key-value stores, which we have already discussed. Key-value stores offer simplicity, scalability, and fast data access, making them suitable for caching frequently used queries.
Redis and Performance Optimization
Redis is known for its excellent performance characteristics, which make it a popular choice for caching frequently used queries. It achieves this performance through several key optimizations.
Firstly, Redis stores data in memory, allowing for extremely fast data access. This eliminates the need to fetch data from disk, which is typically much slower. Additionally, Redis is designed to be single-threaded, avoiding the overhead of thread synchronization and context switching.
Furthermore, Redis employs various data structures and algorithms optimized for specific use cases. For example, Redis’s sorted sets data structure allows for efficient range queries and ranking operations. These optimizations contribute to Redis’s overall performance and make it well-suited for caching frequently used queries.
Scalability Considerations with Redis
Scalability is a crucial aspect of caching frequently used queries, as the cache needs to handle a potentially large volume of data and requests. Redis offers several features and techniques to ensure scalability.
One such technique is data partitioning, where the data is distributed across multiple Redis instances or nodes. Each node is responsible for a subset of the data, allowing for parallel processing and improved performance. Redis supports both manual and automatic partitioning strategies, giving developers flexibility in scaling their cache.
Redis also provides replication capabilities, allowing for data redundancy and high availability. By replicating data across multiple Redis instances, the cache can continue to serve requests even in the event of a node failure. Replication also enables load balancing, as client requests can be distributed across multiple nodes.
Related Article: Tutorial on Redis Queue Implementation
Redis and Data Persistence
While Redis is primarily an in-memory data store, it also offers persistence options to ensure data durability. Persistence allows Redis to save its data to disk, enabling data recovery in case of a system failure or restart.
Redis provides two main mechanisms for data persistence: snapshotting and append-only file (AOF) persistence.
Snapshotting involves periodically writing the entire dataset to disk as a binary file. This process can be configured to occur at regular intervals or when a certain number of changes have been made to the dataset. In the event of a failure, Redis can recover the dataset by loading the latest snapshot.
AOF persistence, on the other hand, logs every write operation to a file. This log can be replayed to reconstruct the dataset in case of a failure. AOF persistence offers better durability than snapshotting, as it logs every change made to the dataset.
How Redis Handles Caching
Redis provides various features and capabilities that make it well-suited for handling caching tasks. Let’s explore some of these features in detail.
Expiration
One key feature of Redis caching is the ability to set an expiration time for cached data. When storing data in Redis, developers can specify a time-to-live (TTL) value, which determines how long the data will remain in the cache. Once the TTL expires, Redis automatically removes the data from the cache.
The expiration feature is particularly useful for caching frequently used queries, as it ensures that the cache remains up-to-date and avoids serving stale data. By setting an appropriate TTL based on the frequency of query updates, developers can strike a balance between cache freshness and performance.
Related Article: Tutorial on AWS Elasticache Redis Implementation
Data Eviction Policies
Redis also provides various data eviction policies to handle situations where the cache becomes full and needs to make room for new data. When the cache reaches its memory limit, Redis can automatically remove less frequently used data to accommodate new entries.
Some of the common data eviction policies supported by Redis include:
– Least Recently Used (LRU): Removes the least recently accessed data first.
– Least Frequently Used (LFU): Removes the least frequently accessed data first.
– Random: Removes data randomly.
These eviction policies ensure that the cache remains efficient and avoids wasting memory on data that is rarely or never accessed.
Pipeline and Batch Operations
Redis supports pipeline and batch operations, which allow developers to execute multiple commands in a single round trip to the Redis server. This can significantly improve performance when dealing with multiple cache operations, such as setting or retrieving multiple keys.
Here’s an example of using pipeline and batch operations in Redis:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) # Create a pipeline pipeline = r.pipeline() # Add multiple cache operations to the pipeline pipeline.set('key1', 'value1') pipeline.set('key2', 'value2') pipeline.get('key1') pipeline.get('key2') # Execute the pipeline results = pipeline.execute() # Print the results for result in results: print(result)
In the above example, we create a pipeline and add multiple cache operations to it, including setting and retrieving key-value pairs. We then execute the pipeline, which sends all the commands to the Redis server in a single round trip. Finally, we print the results of the executed commands.
Leveraging Redis for Frequently Used Queries
Now that we have explored the various features and capabilities of Redis, let’s delve into how we can leverage Redis for caching frequently used queries. Redis can be used in multiple ways, depending on the specific requirements and characteristics of the application.
Related Article: Tutorial on Configuring a Redis Cluster
Redis as a Key-Value Store
One of the simplest and most common ways to use Redis for caching frequently used queries is by treating it as a key-value store. In this approach, the query results are stored as values in Redis, with a unique key representing each query.
When a query needs to be executed, the application first checks if the result is already cached in Redis by using the query as the key. If the result is found in the cache, it is returned directly. Otherwise, the query is executed, and the result is stored in Redis for future use.
Here’s an example of using Redis as a key-value store for caching frequently used queries:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) def execute_query(query): # Check if the query result is already cached cached_result = r.get(query) if cached_result: # Return the cached result return cached_result else: # Execute the query result = db.execute_query(query) # Cache the query result r.set(query, result) # Return the query result return result
In the above example, we define a function execute_query
that takes a query as input. We first check if the query result is already cached in Redis using the query as the key. If the result is found in the cache, we return it directly. Otherwise, we execute the query using a hypothetical db.execute_query
function and cache the result in Redis using the same query as the key.
Redis as an In-Memory Data Structure Store
Another approach to leveraging Redis for caching frequently used queries is by utilizing its in-memory data structure store capabilities. Redis provides various data structures, such as lists, sets, sorted sets, and hashes, which can be used to store and manipulate query results.
For example, a sorted set in Redis can be used to store a list of users along with their scores, where the score represents a certain metric or attribute of the user. This can be useful for caching queries that involve ranking or sorting users based on a specific criterion.
Here’s an example of using Redis as an in-memory data structure store for caching frequently used queries:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) def get_top_users(): # Check if the top users are already cached cached_result = r.zrange('top_users', 0, -1) if cached_result: # Return the cached top users return cached_result else: # Fetch the top users from the database result = db.get_top_users() # Cache the top users in Redis for user in result: r.zadd('top_users', {user.name: user.score}) # Return the top users return result
In the above example, we define a function get_top_users
that fetches the top users from the database. We first check if the top users are already cached in Redis using the sorted set top_users
. If the top users are found in the cache, we return them directly. Otherwise, we fetch the top users from the database using a hypothetical db.get_top_users
function and cache them in Redis using the sorted set top_users
, where the user’s name is the member and the user’s score is the score.
Redis as a NoSQL Database
Redis can also be used as a full-fledged NoSQL database for caching frequently used queries. In this approach, Redis is used as the primary data store for the application, storing both the original data and the cached query results.
Here’s an example of using Redis as a NoSQL database for caching frequently used queries:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) def get_user_profile(user_id): # Check if the user profile is already cached cached_result = r.hgetall(f'user_profile:{user_id}') if cached_result: # Return the cached user profile return cached_result else: # Fetch the user profile from the database result = db.get_user_profile(user_id) # Cache the user profile in Redis r.hmset(f'user_profile:{user_id}', result) # Return the user profile return result
In the above example, we define a function get_user_profile
that retrieves a user’s profile from the database. We first check if the user profile is already cached in Redis using a hash with the key user_profile:{user_id}
. If the user profile is found in the cache, we return it directly. Otherwise, we fetch the user profile from the database using a hypothetical db.get_user_profile
function and cache it in Redis using the same hash key.
Related Article: Redis vs MongoDB: A Detailed Comparison
Redis for Performance Improvement
Using Redis for caching frequently used queries can significantly improve the performance of an application. By storing query results in Redis, subsequent requests for the same query can be served directly from the cache, avoiding the need to hit the database again. This reduces the response time and improves the overall performance of the application.
Redis’s in-memory data storage and optimized data structures enable fast data access and manipulation, further enhancing performance. Additionally, Redis’s support for pipeline and batch operations allows for efficient retrieval and storage of query results, reducing the overhead of network latency.
Let’s consider an example where an e-commerce website needs to fetch product details for a specific product. Without caching, each request for the product details would require a database query, leading to increased response times and decreased performance.
Redis for Scalability Enhancement
Redis’s scalability features make it an excellent choice for caching frequently used queries in high-traffic applications. Redis supports data partitioning, allowing the data to be distributed across multiple Redis instances or nodes. This enables parallel processing and improved performance, especially when handling large volumes of data and requests.
Furthermore, Redis provides replication capabilities, allowing for data redundancy and high availability. By replicating data across multiple Redis instances, the cache can continue to serve requests even in the event of a node failure. Replication also enables load balancing, as client requests can be distributed across multiple nodes, further enhancing scalability.
The combination of data partitioning and replication in Redis ensures that the cache can handle a large volume of data and requests, making it suitable for highly scalable applications.
Redis and Data Persistence Support
Although Redis is primarily an in-memory data store, it provides persistence options to ensure data durability. Redis offers both snapshotting and append-only file (AOF) persistence mechanisms to save data to disk.
Snapshotting involves periodically writing the entire dataset to disk as a binary file. This process can be configured to occur at regular intervals or when a certain number of changes have been made to the dataset. In the event of a failure, Redis can recover the dataset by loading the latest snapshot.
AOF persistence, on the other hand, logs every write operation to a file. This log can be replayed to reconstruct the dataset in case of a failure. AOF persistence offers better durability than snapshotting, as it logs every change made to the dataset.