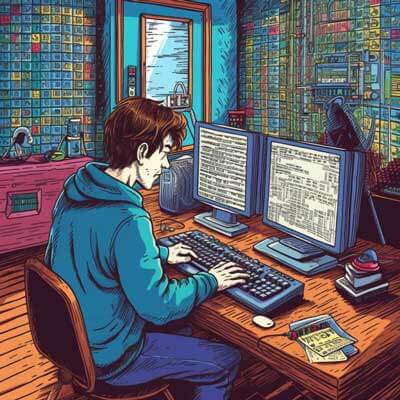
- Introduction to Redis
- Key-Value Data Model
- Publish/Subscribe Messaging
- Getting Started with Spring Boot
- Setting Up a Spring Boot Project
- Connecting to Redis
- Integrating Redis with Spring Boot
- Storing and Retrieving Data
- Working with Lists
- Basic Examples of Using Redis
- Caching with Redis
- Session Management with Redis
- Advanced Examples of Using Redis
- Distributed Locks with Redis
- Pub/Sub Messaging with Redis
- Use Cases for Redis and Spring Boot
- Real-Time Analytics with Redis
- Leaderboard System with Redis
- Best Practices for Using Redis
- Connection Pooling
- Serialization and Deserialization
- Real World Examples of Redis Integration
- Session Clustering with Redis
- Caching with Redis for Microservices
- Performance Considerations for Redis
- Optimizing Redis Operations
- Scaling Redis
- Advanced Techniques for Redis Integration
- Data Partitioning with Redis
- Rate Limiting with Redis
- Code Snippet Ideas for Redis and Spring Boot
- Using Redis for Caching
- Implementing Distributed Locks with Redis
- Error Handling in Redis Integration
- Handling Redis Connection Errors
- Handling Redis Command Errors
Introduction to Redis
Redis is an open-source, in-memory data structure store that can be used as a database, cache, and message broker. It is known for its high performance, flexibility, and rich set of data types. Redis is widely used in modern web applications to improve performance and scalability. In this chapter, we will explore the key features and concepts of Redis.
Related Article: How to Configure a Redis Cluster
Key-Value Data Model
Redis uses a key-value data model, where data is stored as a key and its corresponding value. Keys are unique and can be of various types, such as strings, lists, sets, hashes, and more. Let’s take a look at an example of storing and retrieving data using Redis in a Spring Boot application:
// RedisTemplate is a convenient wrapper around Redis operations @Autowired private RedisTemplate<String, String> redisTemplate; public void storeData(String key, String value) { redisTemplate.opsForValue().set(key, value); } public String retrieveData(String key) { return redisTemplate.opsForValue().get(key); }
Publish/Subscribe Messaging
Redis also provides a publish/subscribe messaging system, where publishers send messages to channels and subscribers receive messages from those channels. This feature is useful for real-time updates and event-driven architectures. Let’s see an example of publishing and subscribing to messages using Redis in a Spring Boot application:
// RedisMessageListenerContainer handles message subscription and processing @Autowired private RedisMessageListenerContainer listenerContainer; // Subscriber implementation public class MessageSubscriber implements MessageListener { @Override public void onMessage(Message message, byte[] pattern) { System.out.println("Received message: " + new String(message.getBody())); } } // Subscribe to a channel listenerContainer.addMessageListener(new MessageSubscriber(), new ChannelTopic("channel-name")); // Publisher implementation public class MessagePublisher { @Autowired private RedisTemplate<String, String> redisTemplate; public void publishMessage(String channel, String message) { redisTemplate.convertAndSend(channel, message); } }
Getting Started with Spring Boot
Spring Boot is a useful framework that simplifies the development of Java applications, including those that integrate with Redis. It provides a convention-over-configuration approach, allowing developers to focus on writing business logic rather than dealing with infrastructure concerns. In this chapter, we will explore the basics of Spring Boot and how to set up a project that integrates with Redis.
Related Article: Tutorial on installing and using redis-cli in Redis
Setting Up a Spring Boot Project
To get started with Spring Boot, you need to set up a new project. You can use Spring Initializr, a web-based tool, or your preferred IDE to generate a basic Spring Boot project structure. Make sure to include the necessary dependencies for Redis integration.
After setting up the project, you can configure the Redis connection in the application.properties or application.yml file:
spring.redis.host=localhost spring.redis.port=6379
Connecting to Redis
To connect to Redis in a Spring Boot application, you can use the Jedis or Lettuce libraries. Jedis is a simple and lightweight Redis client for Java, while Lettuce is a more advanced and thread-safe client.
Here’s an example of configuring and connecting to Redis using Jedis:
@Configuration public class RedisConfig { @Value("${spring.redis.host}") private String redisHost; @Value("${spring.redis.port}") private int redisPort; @Bean public JedisConnectionFactory jedisConnectionFactory() { RedisStandaloneConfiguration config = new RedisStandaloneConfiguration(redisHost, redisPort); return new JedisConnectionFactory(config); } @Bean public RedisTemplate<String, String> redisTemplate() { RedisTemplate<String, String> template = new RedisTemplate<>(); template.setConnectionFactory(jedisConnectionFactory()); return template; } }
Integrating Redis with Spring Boot
Integrating Redis with Spring Boot is straightforward, thanks to the available Redis support provided by Spring Data Redis. Spring Data Redis is an abstraction over the Redis client libraries, offering a simplified and consistent API for interacting with Redis. In this chapter, we will explore how to integrate Redis with Spring Boot using Spring Data Redis.
Related Article: Tutorial on Redis Queue Implementation
Storing and Retrieving Data
To store and retrieve data from Redis, you can use the RedisTemplate provided by Spring Data Redis. The RedisTemplate offers various operations for different data types, such as strings, lists, sets, hashes, and more.
Here’s an example of storing and retrieving data using RedisTemplate in a Spring Boot application:
@Autowired private RedisTemplate<String, String> redisTemplate; public void storeData(String key, String value) { redisTemplate.opsForValue().set(key, value); } public String retrieveData(String key) { return redisTemplate.opsForValue().get(key); }
Working with Lists
Redis lists are ordered collections of strings, allowing for efficient insertion and retrieval of elements at both ends. Spring Data Redis provides operations to work with lists, such as pushing elements, popping elements, and retrieving a range of elements.
Here’s an example of working with lists using RedisTemplate in a Spring Boot application:
@Autowired private RedisTemplate<String, String> redisTemplate; public void pushToList(String key, String value) { redisTemplate.opsForList().rightPush(key, value); } public String popFromList(String key) { return redisTemplate.opsForList().leftPop(key); } public List<String> getRangeFromList(String key, long start, long end) { return redisTemplate.opsForList().range(key, start, end); }
Basic Examples of Using Redis
In this chapter, we will explore basic examples of using Redis in a Spring Boot application. We will cover common use cases such as caching, session management, rate limiting, and feature toggling.
Related Article: Tutorial on AWS Elasticache Redis Implementation
Caching with Redis
Caching is a common technique used to improve the performance of applications by storing frequently accessed data in memory. Redis provides a fast and scalable caching solution, allowing you to cache method results, database queries, or any other data that can be expensive to compute or retrieve.
Here’s an example of caching method results using Redis in a Spring Boot application:
@Cacheable("books") public Book getBookById(String id) { // Retrieve book from the database return bookRepository.findById(id); }
Session Management with Redis
Session management is an essential aspect of web applications. Redis can be used as a distributed session store, allowing you to store session data outside the application server and enabling session sharing across multiple instances.
Here’s an example of using Redis for session management in a Spring Boot application:
@Configuration @EnableRedisHttpSession public class HttpSessionConfig { @Bean public LettuceConnectionFactory connectionFactory() { return new LettuceConnectionFactory(); } }
Advanced Examples of Using Redis
In this chapter, we will delve into advanced examples of using Redis in a Spring Boot application. We will explore features like distributed locks, pub/sub messaging, Lua scripting, and more.
Related Article: Tutorial on Configuring a Redis Cluster
Distributed Locks with Redis
Distributed locks are used to coordinate access to shared resources in a distributed system. Redis provides support for distributed locks using its SETNX (set if not exists) command. By using Redis locks, you can prevent multiple instances of an application from executing the same critical section simultaneously.
Here’s an example of using distributed locks with Redis in a Spring Boot application:
@Autowired private RedisTemplate<String, String> redisTemplate; public boolean acquireLock(String lockKey, String requestId, int expireTime) { return redisTemplate.opsForValue().setIfAbsent(lockKey, requestId, expireTime, TimeUnit.SECONDS); } public void releaseLock(String lockKey, String requestId) { if (requestId.equals(redisTemplate.opsForValue().get(lockKey))) { redisTemplate.delete(lockKey); } }
Pub/Sub Messaging with Redis
Redis pub/sub messaging allows applications to communicate with each other using publish/subscribe channels. Publishers send messages to channels, and subscribers receive messages from those channels. This feature is useful for building real-time applications, event-driven architectures, and message queues.
Here’s an example of using pub/sub messaging with Redis in a Spring Boot application:
@Autowired private RedisMessageListenerContainer listenerContainer; // Subscriber implementation public class MessageSubscriber implements MessageListener { @Override public void onMessage(Message message, byte[] pattern) { System.out.println("Received message: " + new String(message.getBody())); } } // Subscribe to a channel listenerContainer.addMessageListener(new MessageSubscriber(), new ChannelTopic("channel-name")); // Publisher implementation public class MessagePublisher { @Autowired private RedisTemplate<String, String> redisTemplate; public void publishMessage(String channel, String message) { redisTemplate.convertAndSend(channel, message); } }
Use Cases for Redis and Spring Boot
In this chapter, we will explore various use cases where Redis and Spring Boot can be combined to build scalable and performant applications. We will cover scenarios such as real-time analytics, leaderboard systems, job queues, and more.
Related Article: Redis vs MongoDB: A Detailed Comparison
Real-Time Analytics with Redis
Real-time analytics is the process of collecting, processing, and analyzing data in real-time. Redis, with its in-memory storage and high-performance capabilities, is well-suited for real-time analytics scenarios. By leveraging Redis data structures and commands, you can quickly aggregate data, perform calculations, and generate real-time insights.
Here’s an example of using Redis for real-time analytics in a Spring Boot application:
@Autowired private RedisTemplate<String, String> redisTemplate; public void trackEvent(String event) { redisTemplate.opsForHyperLogLog().add("events", event); } public long countDistinctEvents() { return redisTemplate.opsForHyperLogLog().size("events"); }
Leaderboard System with Redis
A leaderboard system is commonly used in gaming applications to track and display the top players based on their scores. Redis, with its sorted sets data structure, makes it easy to implement a leaderboard system. You can store player scores as sorted set members and use Redis commands to update and retrieve leaderboard data.
Here’s an example of implementing a leaderboard system with Redis in a Spring Boot application:
@Autowired private RedisTemplate<String, String> redisTemplate; public void updateScore(String player, double score) { redisTemplate.opsForZSet().add("leaderboard", player, score); } public List<String> getTopPlayers(int count) { return redisTemplate.opsForZSet().reverseRange("leaderboard", 0, count - 1); }
Best Practices for Using Redis
In this chapter, we will discuss best practices for using Redis in a Spring Boot application. Following these best practices will help you optimize performance, ensure data consistency, and avoid common pitfalls.
Related Article: Tutorial: Installing Redis on Ubuntu
Connection Pooling
Establishing a connection to Redis can be an expensive operation. To improve performance and reduce connection overhead, it is recommended to use connection pooling. Spring Boot provides built-in support for connection pooling with libraries like Jedis and Lettuce.
Here’s an example of configuring connection pooling with Lettuce in a Spring Boot application:
spring.redis.lettuce.pool.max-active=20 spring.redis.lettuce.pool.max-idle=10 spring.redis.lettuce.pool.min-idle=5
Serialization and Deserialization
When storing objects in Redis, you need to serialize them into a byte array and deserialize them back into objects. Spring Boot provides automatic serialization and deserialization support through its default RedisTemplate. However, it is important to choose an efficient serialization format like JSON or MessagePack to minimize storage and network overhead.
Here’s an example of configuring JSON serialization with RedisTemplate in a Spring Boot application:
@Configuration public class RedisConfig { @Bean public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory redisConnectionFactory) { RedisTemplate<String, Object> template = new RedisTemplate<>(); template.setConnectionFactory(redisConnectionFactory); template.setDefaultSerializer(new Jackson2JsonRedisSerializer<>(Object.class)); return template; } }
Real World Examples of Redis Integration
In this chapter, we will explore real-world examples of Redis integration in Spring Boot applications. We will discuss how leading organizations leverage Redis to solve common challenges and improve application performance.
Related Article: How to Use Redis Queue in Redis
Session Clustering with Redis
Organizations with high-traffic web applications often face challenges related to session management, especially when dealing with multiple application instances. Redis can be used as a session store to achieve session clustering and sharing across multiple instances, ensuring session data consistency and scalability.
Caching with Redis for Microservices
Microservices architectures require efficient and scalable caching solutions to improve performance and reduce database load. Redis’s in-memory caching capabilities make it an excellent choice for caching microservices. By caching frequently accessed data, microservices can respond faster and handle higher loads.
Performance Considerations for Redis
In this chapter, we will discuss performance considerations when using Redis in a Spring Boot application. Understanding these considerations will help you optimize the performance of your Redis integration and ensure a smooth user experience.
Related Article: Tutorial: Comparing Kafka vs Redis
Optimizing Redis Operations
To maximize Redis performance, it is important to minimize the number of Redis operations and optimize the execution of those operations. Batch operations, pipelining, and Lua scripting are some techniques that can help reduce the round-trip time between the application and Redis.
Scaling Redis
As the load on your application increases, you may need to scale your Redis infrastructure to handle the increased traffic. Redis supports various scaling techniques such as replication, clustering, and sharding. Understanding these techniques and choosing the right scaling strategy is crucial for achieving high performance and availability.
Advanced Techniques for Redis Integration
In this chapter, we will explore advanced techniques for integrating Redis with Spring Boot. We will discuss topics such as data partitioning, rate limiting, distributed caching, and more.
Related Article: Redis Tutorial: How to Use Redis
Data Partitioning with Redis
Data partitioning is a technique used to distribute data across multiple Redis instances, allowing for horizontal scalability and improved performance. Redis supports different partitioning strategies, such as consistent hashing and range partitioning. By partitioning your data, you can handle larger datasets and higher throughput.
Rate Limiting with Redis
Rate limiting is a technique used to control the rate of incoming requests to prevent abuse or overload of resources. Redis can be used to implement rate limiting by leveraging its atomic operations and data structures. You can use Redis’s counters, sorted sets, and Lua scripting to enforce rate limits and protect your application.
Code Snippet Ideas for Redis and Spring Boot
In this chapter, we will provide code snippet ideas for Redis and Spring Boot integration. These code snippets can serve as a starting point for implementing specific features or solving common challenges.
Related Article: Exploring Alternatives to Redis
Using Redis for Caching
@Autowired private RedisTemplate<String, String> redisTemplate; @Cacheable("books") public Book getBookById(String id) { // Retrieve book from the database return bookRepository.findById(id); }
Implementing Distributed Locks with Redis
@Autowired private RedisTemplate<String, String> redisTemplate; public boolean acquireLock(String lockKey, String requestId, int expireTime) { return redisTemplate.opsForValue().setIfAbsent(lockKey, requestId, expireTime, TimeUnit.SECONDS); } public void releaseLock(String lockKey, String requestId) { if (requestId.equals(redisTemplate.opsForValue().get(lockKey))) { redisTemplate.delete(lockKey); } }
Error Handling in Redis Integration
In this chapter, we will discuss error handling techniques when integrating Redis with Spring Boot. It is important to handle errors gracefully to ensure the robustness and reliability of your application.
Related Article: How to Use Redis Streams
Handling Redis Connection Errors
When working with Redis, connection errors can occur due to various reasons such as network issues, Redis server failures, or misconfigurations. It is essential to handle these errors and implement appropriate error handling mechanisms, such as retrying failed operations or falling back to alternative data sources.
Handling Redis Command Errors
Redis commands can fail due to various reasons, such as invalid command arguments, exceeding memory limits, or data corruption. It is important to handle command errors and provide meaningful error messages to users or log detailed error information for troubleshooting purposes.
These chapters cover the basics, advanced techniques, and real-world examples of integrating Redis with Spring Boot. By following best practices and considering performance considerations, you can leverage Redis to build scalable, performant, and reliable applications.