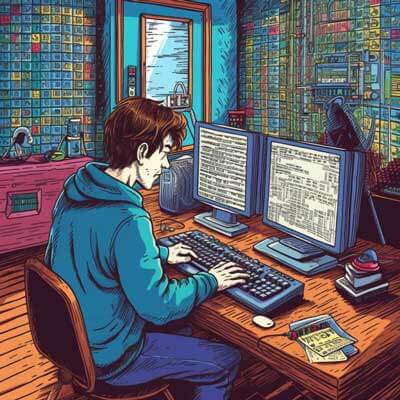
- Introduction
- Redis Overview
- Example 1: Basic Redis Operations
- Example 2: Redis Pub/Sub Messaging
- RabbitMQ Overview
- Example 1: Basic RabbitMQ Operations
- Example 2: RabbitMQ Publish/Subscribe Messaging
- Comparison of Redis and RabbitMQ
- Use Cases for Redis
- Example 1: Redis Caching
- Example 2: Redis Pub/Sub Messaging
- Use Cases for RabbitMQ
- Example 1: RabbitMQ Message Queues
- Example 2: RabbitMQ Publish/Subscribe with Routing
- Best Practices for Redis
- Example 1: Connection Pooling in Python
- Example 2: Pipelining Commands in Node.js
- Best Practices for RabbitMQ
- Example 1: Manual Acknowledgements in Java
- Example 2: Connection and Channel Reuse in Python
Introduction
Redis and RabbitMQ are two popular messaging systems used in modern software development. While both offer solutions for handling data and communication between different components of an application, they have distinct characteristics and use cases. This tutorial aims to provide a comprehensive comparison between Redis and RabbitMQ, covering their features, use cases, best practices, performance considerations, advanced techniques, code snippets, and error handling.
Related Article: Leveraging Redis for Caching Frequently Used Queries
Redis Overview
Redis is an open-source, in-memory data structure store that can be used as a database, cache, and message broker. It supports various data types, including strings, hashes, lists, sets, and sorted sets, and provides efficient operations for manipulating and querying data. Redis is known for its high performance and low latency, making it suitable for use cases that require fast data access and real-time processing.
Example 1: Basic Redis Operations
To get started with Redis, you need to install it on your system and start the Redis server. Once the server is running, you can interact with Redis using various client libraries available for different programming languages. Here’s an example in Python:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) # Set a key-value pair r.set('name', 'John') # Get the value of a key name = r.get('name') print(name.decode()) # Output: John # Increment a value r.incr('counter') counter = r.get('counter') print(counter.decode()) # Output: 1
In this example, we connect to a Redis server running on the localhost and set a key-value pair (‘name’: ‘John’). We then retrieve the value of the ‘name’ key and increment a counter.
Example 2: Redis Pub/Sub Messaging
Redis also supports publish/subscribe messaging, allowing different components of an application to communicate with each other. Here’s an example of using Redis pub/sub in Node.js:
const redis = require('redis'); // Connect to Redis const subscriber = redis.createClient(); const publisher = redis.createClient(); // Subscribe to a channel subscriber.subscribe('notifications'); // Handle incoming messages subscriber.on('message', (channel, message) => { console.log(`Received message on channel ${channel}: ${message}`); }); // Publish a message publisher.publish('notifications', 'New notification: Hello, world!');
In this example, we create a Redis subscriber and publisher, and subscribe to the ‘notifications’ channel. When a message is published to the channel, the subscriber receives it and logs the message to the console.
Related Article: Analyzing Redis Query Rate per Second with Sidekiq
RabbitMQ Overview
RabbitMQ is a robust and flexible message broker that implements the Advanced Message Queuing Protocol (AMQP). It provides a reliable and scalable solution for asynchronous communication between different components of an application. RabbitMQ supports various messaging patterns, including point-to-point, publish/subscribe, request/reply, and routing, making it suitable for a wide range of use cases.
Example 1: Basic RabbitMQ Operations
To use RabbitMQ, you need to install it on your system and start the RabbitMQ server. Once the server is running, you can interact with RabbitMQ using client libraries available for different programming languages. Here’s an example in Java:
import com.rabbitmq.client.ConnectionFactory; import com.rabbitmq.client.Connection; import com.rabbitmq.client.Channel; public class RabbitMQExample { private final static String QUEUE_NAME = "hello"; public static void main(String[] argv) throws Exception { // Create a connection factory ConnectionFactory factory = new ConnectionFactory(); factory.setHost("localhost"); // Create a connection and a channel Connection connection = factory.newConnection(); Channel channel = connection.createChannel(); // Declare a queue channel.queueDeclare(QUEUE_NAME, false, false, false, null); // Publish a message String message = "Hello, world!"; channel.basicPublish("", QUEUE_NAME, null, message.getBytes()); System.out.println("Sent message: " + message); // Close the channel and the connection channel.close(); connection.close(); } }
In this example, we create a RabbitMQ connection factory, connection, and channel. We then declare a queue named ‘hello’ and publish a message to the queue.
Example 2: RabbitMQ Publish/Subscribe Messaging
RabbitMQ’s publish/subscribe messaging pattern allows multiple consumers to receive messages from a single publisher. Here’s an example of using RabbitMQ publish/subscribe in Python:
import pika # Create a connection and a channel connection = pika.BlockingConnection(pika.ConnectionParameters('localhost')) channel = connection.channel() # Declare an exchange channel.exchange_declare(exchange='logs', exchange_type='fanout') # Publish a message message = 'Hello, world!' channel.basic_publish(exchange='logs', routing_key='', body=message) print(f'Sent message: {message}') # Close the channel and the connection channel.close() connection.close()
In this example, we create a RabbitMQ connection and channel and declare a fanout exchange named ‘logs’. We then publish a message to the exchange, which will be delivered to all queues bound to the exchange.
Related Article: How to use Redis with Laravel and PHP
Comparison of Redis and RabbitMQ
Both Redis and RabbitMQ offer solutions for handling data and communication between different components of an application, but they have distinct characteristics and use cases. Here’s a comparison of Redis and RabbitMQ based on various factors:
– Data Model: Redis stores data in various data types, providing efficient operations for manipulating and querying data. RabbitMQ focuses on message-based communication and does not store data persistently.
– Messaging Patterns: Redis supports publish/subscribe messaging, allowing different components to communicate asynchronously. RabbitMQ supports a wide range of messaging patterns, including point-to-point, publish/subscribe, request/reply, and routing.
– Scalability: Redis is designed to be highly scalable and can handle a large number of concurrent operations. RabbitMQ supports horizontal scaling through clustering.
– Durability: Redis can persist data to disk, but its primary focus is in-memory data storage. RabbitMQ provides durable message queues, ensuring messages are not lost even if the broker restarts.
– Performance: Redis is known for its high performance and low latency due to its in-memory nature. RabbitMQ provides reliable and consistent performance but may have higher latency compared to Redis.
– Ease of Use: Redis has a simple and straightforward API, making it easy to get started. RabbitMQ has a more complex API but offers advanced features and flexibility.
– Use Cases: Redis is suitable for use cases that require fast data access, caching, real-time analytics, and pub/sub messaging. RabbitMQ is suitable for use cases that involve reliable message delivery, task scheduling, and complex routing scenarios.
Use Cases for Redis
Redis is widely used in various use cases, including:
– Caching: Redis can be used as a cache to store frequently accessed data and reduce the load on backend systems.
– Real-time Analytics: Redis supports efficient data manipulation and querying, making it suitable for real-time analytics and leaderboard systems.
– Pub/Sub Messaging: Redis’ publish/subscribe messaging allows different components to communicate asynchronously, making it useful for real-time notifications and chat applications.
– Session Storage: Redis can store session data, allowing for efficient and scalable session management in web applications.
– Task Queues: Redis’ list data type and atomic operations make it suitable for implementing task queues and job scheduling systems.
Example 1: Redis Caching
In this example, we demonstrate how to use Redis as a cache in a Python Flask web application. We’ll use the Flask-Caching extension, which provides an easy-to-use interface for caching data with Redis.
from flask import Flask from flask_caching import Cache app = Flask(__name__) cache = Cache(app, config={'CACHE_TYPE': 'redis'}) @app.route('/users/<int:user_id>') @cache.cached(timeout=60) # Cache the response for 60 seconds def get_user(user_id): # Retrieve user data from the database user = db.get_user(user_id) return jsonify(user) if __name__ == '__main__': app.run()
In this example, we define a Flask route that retrieves user data from a database based on the user ID. We use the @cache.cached
decorator to cache the response for 60 seconds. Subsequent requests with the same user ID will be served from the cache, improving the response time and reducing the load on the database.
Related Article: Tutorial on Redis Docker Compose
Example 2: Redis Pub/Sub Messaging
Redis’ publish/subscribe messaging can be used to implement real-time notifications in a chat application. Here’s an example using Node.js and the ioredis
library:
const Redis = require('ioredis'); const redis = new Redis(); // Subscriber redis.subscribe('notifications', (err, count) => { if (err) { console.error('Failed to subscribe:', err); return; } console.log(`Subscribed to ${count} channel(s)`); }); // Message handler redis.on('message', (channel, message) => { console.log(`Received message on channel ${channel}: ${message}`); }); // Publisher redis.publish('notifications', 'New notification: Hello, world!');
In this example, we create a Redis subscriber and subscribe to the ‘notifications’ channel. When a message is published to the channel, the subscriber receives it and logs the message to the console. We also publish a message to the ‘notifications’ channel, which will be received by the subscriber.
Use Cases for RabbitMQ
RabbitMQ is commonly used in the following use cases:
– Message Queues: RabbitMQ provides reliable and scalable message queuing, allowing decoupling of components and enabling asynchronous communication.
– Task Scheduling: RabbitMQ’s delayed message delivery and scheduling capabilities make it suitable for task scheduling and background job processing.
– Event-Driven Architecture: RabbitMQ’s publish/subscribe and routing features make it suitable for implementing event-driven architectures and event-driven microservices.
– Request/Reply Patterns: RabbitMQ supports request/reply patterns, allowing components to send requests and receive responses asynchronously.
– Complex Routing: RabbitMQ provides routing mechanisms such as topic-based routing and direct exchange, making it suitable for complex routing scenarios.
Example 1: RabbitMQ Message Queues
In this example, we demonstrate how to use RabbitMQ to implement a simple message queue in Python using the pika
library:
import pika # Create a connection and a channel connection = pika.BlockingConnection(pika.ConnectionParameters('localhost')) channel = connection.channel() # Declare a queue channel.queue_declare(queue='task_queue', durable=True) # Publish messages for i in range(10): message = f'Task {i+1}' channel.basic_publish( exchange='', routing_key='task_queue', body=message, properties=pika.BasicProperties(delivery_mode=2) # Make messages persistent ) print(f'Sent message: {message}') # Close the channel and the connection channel.close() connection.close()
In this example, we create a RabbitMQ connection and channel, and declare a durable queue named ‘task_queue’. We then publish 10 messages to the queue, making them persistent by setting the delivery_mode
property to 2. The messages will be stored in RabbitMQ even if the broker restarts.
Related Article: Tutorial on Redis Sentinel: A Deep Look
Example 2: RabbitMQ Publish/Subscribe with Routing
RabbitMQ’s routing feature allows messages to be selectively delivered to queues based on routing keys. Here’s an example using Python and the pika
library:
import pika # Create a connection and a channel connection = pika.BlockingConnection(pika.ConnectionParameters('localhost')) channel = connection.channel() # Declare an exchange and queues channel.exchange_declare(exchange='logs', exchange_type='direct') channel.queue_declare(queue='queue1', durable=True) channel.queue_declare(queue='queue2', durable=True) # Bind queues to the exchange with routing keys channel.queue_bind(exchange='logs', queue='queue1', routing_key='info') channel.queue_bind(exchange='logs', queue='queue2', routing_key='warning') # Publish messages with different routing keys channel.basic_publish(exchange='logs', routing_key='info', body='Info message') channel.basic_publish(exchange='logs', routing_key='warning', body='Warning message') # Close the channel and the connection channel.close() connection.close()
In this example, we create a RabbitMQ connection and channel, and declare an exchange named ‘logs’ with a direct exchange type. We also declare two queues (‘queue1’ and ‘queue2’) and bind them to the exchange with different routing keys (‘info’ and ‘warning’). We then publish messages with different routing keys, which will be delivered to the corresponding queues.
Best Practices for Redis
When working with Redis, it is important to follow best practices to ensure optimal performance and reliability. Here are some best practices for using Redis:
– Data Modeling: Understand your data requirements and choose the appropriate Redis data types and operations for efficient storage and retrieval.
– Key Naming: Use meaningful and consistent naming conventions for Redis keys to avoid conflicts and improve maintainability.
– Memory Management: Consider the memory limitations of your Redis server and monitor memory usage. Use Redis’ eviction policies and data expiration to manage memory efficiently.
– Connection Pooling: Use connection pooling to minimize the overhead of establishing and tearing down connections to the Redis server.
– Pipeline Commands: When sending multiple commands to Redis, use pipeline commands to reduce round-trip latency and improve performance.
– Error Handling: Handle exceptions and errors gracefully when interacting with Redis, and implement appropriate retry and fallback mechanisms.
Example 1: Connection Pooling in Python
In Python, you can use the redis-py
library’s connection pooling feature to manage Redis connections efficiently. Here’s an example:
import redis from redis.connection import ConnectionPool # Create a connection pool pool = ConnectionPool(host='localhost', port=6379, db=0, max_connections=10) # Use the connection pool to create Redis connections r1 = redis.Redis(connection_pool=pool) r2 = redis.Redis(connection_pool=pool) # Perform Redis operations using the connections r1.set('key1', 'value1') r2.get('key1')
In this example, we create a connection pool with a maximum of 10 connections. We then create two Redis connections using the connection pool and perform Redis operations using the connections. The connection pool manages the connections and reuses them efficiently, reducing the overhead of creating new connections for each operation.
Related Article: Tutorial: Integrating Redis with Spring Boot
Example 2: Pipelining Commands in Node.js
In Node.js, you can use the ioredis
library’s pipeline feature to send multiple commands to Redis in a single round-trip. Here’s an example:
const Redis = require('ioredis'); const redis = new Redis(); // Create a Redis pipeline const pipeline = redis.pipeline(); // Add multiple commands to the pipeline pipeline.set('key1', 'value1'); pipeline.get('key1'); pipeline.del('key1'); // Execute the pipeline and get the results pipeline.exec((err, results) => { if (err) { console.error('Pipeline execution failed:', err); return; } console.log('Pipeline results:', results); });
In this example, we create a Redis pipeline and add multiple commands to it (set, get, and del). We then execute the pipeline using the exec
method and handle the results in the callback. The commands in the pipeline are sent to Redis in a single round-trip, reducing the latency and improving performance.
Best Practices for RabbitMQ
When working with RabbitMQ, it is important to follow best practices to ensure reliable and efficient message handling. Here are some best practices for using RabbitMQ:
– Message Design: Design messages in a language-agnostic and extensible format, such as JSON or Protobuf, to ensure compatibility and future-proofing.
– Exchange/Queue Design: Carefully design exchanges and queues based on your application’s requirements. Use appropriate exchange types (direct, topic, fanout) and bindings to route messages effectively.
– Prefetch Count: Set a reasonable prefetch count to control the number of unacknowledged messages consumed by each consumer, balancing throughput and resource consumption.
– Acknowledge Messages: Acknowledge messages after processing them to ensure they are not redelivered unnecessarily. Use manual acknowledgements for more control and at-least-once message processing semantics.
– Connection Management: Establish a connection and channel to RabbitMQ and reuse them across multiple operations to minimize the overhead of establishing connections.
– Error Handling: Handle and log errors gracefully when interacting with RabbitMQ, and implement appropriate retry and error handling mechanisms.
Example 1: Manual Acknowledgements in Java
In Java, you can use the RabbitMQ Java client library to manually acknowledge messages after processing them. Here’s an example:
import com.rabbitmq.client.*; public class RabbitMQExample { private final static String QUEUE_NAME = "hello"; public static void main(String[] argv) throws Exception { // Create a connection factory ConnectionFactory factory = new ConnectionFactory(); factory.setHost("localhost"); // Create a connection and a channel Connection connection = factory.newConnection(); Channel channel = connection.createChannel(); // Declare a queue channel.queueDeclare(QUEUE_NAME, false, false, false, null); // Create a consumer Consumer consumer = new DefaultConsumer(channel) { @Override public void handleDelivery(String consumerTag, Envelope envelope, AMQP.BasicProperties properties, byte[] body) throws IOException { String message = new String(body, "UTF-8"); System.out.println("Received message: " + message); // Process the message // Acknowledge the message channel.basicAck(envelope.getDeliveryTag(), false); } }; // Start consuming messages channel.basicConsume(QUEUE_NAME, false, consumer); } }
In this example, we create a RabbitMQ connection and channel, declare a queue, and create a consumer using the DefaultConsumer
class. When a message is delivered, the handleDelivery
method is called, and we process the message. After processing, we call channel.basicAck
to acknowledge the message and remove it from the queue.
Related Article: Tutorial on Redis Sharding Implementation
Example 2: Connection and Channel Reuse in Python
In Python, you can reuse the RabbitMQ connection and channel across multiple operations to minimize the overhead of establishing connections. Here’s an example:
import pika # Create a connection and a channel connection = pika.BlockingConnection(pika.ConnectionParameters('localhost')) channel = connection.channel() # Declare a queue channel.queue_declare(queue='hello') # Publish a message channel.basic_publish(exchange='', routing_key='hello', body='Hello, world!') # Consume messages def callback(ch, method, properties, body): print("Received message:", body.decode()) channel.basic_consume(queue='hello', on_message_callback=callback, auto_ack=True) channel.start_consuming() # Close the channel and the connection channel.close() connection.close()
In this example, we create a RabbitMQ connection and channel, declare a queue, publish a message, and consume messages. The connection and channel are reused for both publishing and consuming operations, reducing the overhead of establishing connections for each operation.