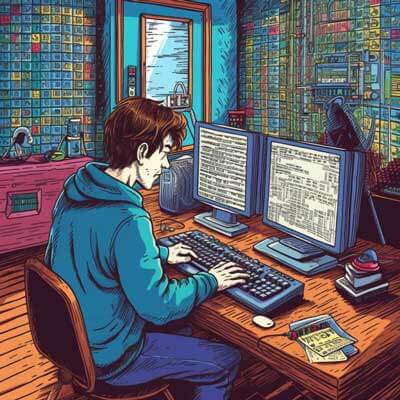
- Introduction
- Kafka Overview
- Code Snippet: Kafka Consumer
- Code Snippet: Kafka Producer
- Redis Overview
- Code Snippet: Redis Pub/Sub
- Comparative Study Methodology
- Use Cases: Kafka
- Use Cases: Redis
- Best Practices: Kafka
- Best Practices: Redis
- Real World Examples: Kafka
- Real World Examples: Redis
- Performance Considerations: Kafka
- Performance Considerations: Redis
- Advanced Techniques: Kafka
- Advanced Techniques: Redis
- Code Snippet: Kafka Consumer
- Code Snippet: Kafka Producer
- Code Snippet: Redis Pub/Sub
- Error Handling: Kafka
- Error Handling: Redis
Introduction
Kafka and Redis are two popular technologies widely used in the field of data processing and messaging systems. While Kafka is a distributed streaming platform, Redis is an in-memory data structure store. Both technologies offer unique features and capabilities that make them suitable for various use cases. In this tutorial, we will explore the differences and similarities between Kafka and Redis, and provide a comparative study to help you understand when to use each technology based on your specific requirements. We will also cover best practices, real-world examples, performance considerations, and advanced techniques for both Kafka and Redis.
Related Article: Tutorial on AWS Elasticache Redis Implementation
Kafka Overview
Kafka is a distributed streaming platform that is designed to handle large volumes of data in a fault-tolerant and scalable manner. It provides a publish-subscribe model, where producers publish messages to Kafka topics, and consumers subscribe to these topics to consume the messages. Kafka is known for its high throughput, low latency, and durability, making it an ideal choice for real-time data streaming applications.
Code Snippet: Kafka Consumer
import org.apache.kafka.clients.consumer.Consumer; import org.apache.kafka.clients.consumer.ConsumerConfig; import org.apache.kafka.clients.consumer.ConsumerRecords; import org.apache.kafka.clients.consumer.KafkaConsumer; import org.apache.kafka.common.serialization.StringDeserializer; import java.time.Duration; import java.util.Collections; import java.util.Properties; public class KafkaConsumerExample { private static final String TOPIC_NAME = "my-topic"; private static final String BOOTSTRAP_SERVERS = "localhost:9092"; private static final String GROUP_ID = "my-consumer-group"; public static void main(String[] args) { Properties props = new Properties(); props.put(ConsumerConfig.BOOTSTRAP_SERVERS_CONFIG, BOOTSTRAP_SERVERS); props.put(ConsumerConfig.GROUP_ID_CONFIG, GROUP_ID); props.put(ConsumerConfig.KEY_DESERIALIZER_CLASS_CONFIG, StringDeserializer.class.getName()); props.put(ConsumerConfig.VALUE_DESERIALIZER_CLASS_CONFIG, StringDeserializer.class.getName()); Consumer<String, String> consumer = new KafkaConsumer<>(props); consumer.subscribe(Collections.singletonList(TOPIC_NAME)); while (true) { ConsumerRecords<String, String> records = consumer.poll(Duration.ofMillis(100)); // Process the received records for (ConsumerRecord<String, String> record : records) { System.out.printf("Received message: key = %s, value = %s%n", record.key(), record.value()); } } } }
Code Snippet: Kafka Producer
import org.apache.kafka.clients.producer.Producer; import org.apache.kafka.clients.producer.ProducerConfig; import org.apache.kafka.clients.producer.ProducerRecord; import org.apache.kafka.common.serialization.StringSerializer; import java.util.Properties; public class KafkaProducerExample { private static final String TOPIC_NAME = "my-topic"; private static final String BOOTSTRAP_SERVERS = "localhost:9092"; public static void main(String[] args) { Properties props = new Properties(); props.put(ProducerConfig.BOOTSTRAP_SERVERS_CONFIG, BOOTSTRAP_SERVERS); props.put(ProducerConfig.KEY_SERIALIZER_CLASS_CONFIG, StringSerializer.class.getName()); props.put(ProducerConfig.VALUE_SERIALIZER_CLASS_CONFIG, StringSerializer.class.getName()); Producer<String, String> producer = new KafkaProducer<>(props); try { for (int i = 0; i < 10; i++) { String key = "key" + i; String value = "value" + i; ProducerRecord<String, String> record = new ProducerRecord<>(TOPIC_NAME, key, value); producer.send(record); System.out.printf("Sent message: key = %s, value = %s%n", key, value); } } finally { producer.close(); } } }
Related Article: Leveraging Redis for Caching Frequently Used Queries
Redis Overview
Redis is an open-source in-memory data structure store that can be used as a database, cache, and message broker. It supports various data structures such as strings, lists, sets, sorted sets, and hashes, along with operations to manipulate and query these data structures. Redis is known for its high performance, flexibility, and simplicity, making it a popular choice for use cases that require fast data access and real-time data processing.
Code Snippet: Redis Pub/Sub
import redis def message_handler(message): print(f"Received message: {message['data'].decode('utf-8')}") if __name__ == '__main__': r = redis.Redis(host='localhost', port=6379, db=0) p = r.pubsub() p.subscribe('my-channel') for message in p.listen(): if message['type'] == 'message': message_handler(message)
Comparative Study Methodology
To conduct a comparative study between Kafka and Redis, we will evaluate various aspects such as their features, performance, use cases, best practices, and advanced techniques. We will also provide real-world examples and code snippets to illustrate the concepts discussed. This comparative study will help you understand the strengths and weaknesses of Kafka and Redis and make an informed decision when choosing the right technology for your specific requirements.
Related Article: Analyzing Redis Query Rate per Second with Sidekiq
Use Cases: Kafka
Kafka is well-suited for the following use cases:
– Log aggregation: Kafka can collect, store, and process log data from various sources in a scalable and fault-tolerant manner.
– Real-time stream processing: Kafka can handle high volumes of data streams in real-time, making it suitable for applications that require real-time analytics and processing.
– Event sourcing: Kafka’s publish-subscribe model allows event-driven architectures, making it ideal for implementing event sourcing patterns.
– Messaging systems: Kafka’s durability and fault-tolerance make it a reliable choice for building messaging systems that require guaranteed message delivery.
Use Cases: Redis
Redis is well-suited for the following use cases:
– Caching: Redis’s in-memory nature provides fast data access, making it an excellent choice for caching frequently accessed data.
– Real-time analytics: Redis supports various data structures and operations that enable real-time analytics on streaming data.
– Pub/Sub messaging: Redis’s publish-subscribe mechanism allows building scalable and distributed messaging systems.
– Session storage: Redis can efficiently store and manage session data, making it suitable for session management in web applications.
Best Practices: Kafka
When using Kafka, consider the following best practices:
– Design topic and partition strategy carefully to ensure optimal data distribution and parallelism.
– Monitor consumer lag to identify potential bottlenecks and optimize consumer performance.
– Enable compression and batching for producers to optimize network and storage utilization.
– Configure replication and retention policies to ensure data durability and availability.
– Use Kafka Connect for seamless integration with external systems.
Related Article: How to use Redis with Laravel and PHP
Best Practices: Redis
When using Redis, consider the following best practices:
– Design data structures based on your application’s access patterns to ensure efficient data retrieval.
– Use Redis persistence mechanisms (RDB and AOF) to ensure data durability.
– Monitor Redis memory usage and configure eviction policies to handle data overflow gracefully.
– Utilize Redis clustering for high availability and scalability.
– Leverage Redis Lua scripting for complex data manipulation and atomic operations.
Real World Examples: Kafka
Kafka is widely used in various industries and for different purposes. Here are a few real-world examples:
– LinkedIn: LinkedIn uses Kafka for real-time data pipeline processing, log aggregation, and activity tracking.
– Uber: Uber leverages Kafka for streaming analytics, real-time data processing, and monitoring of its transportation platform.
– Netflix: Netflix uses Kafka for real-time event processing, data ingestion, and monitoring of its streaming platform.
Real World Examples: Redis
Redis is used by many companies across different industries. Here are a few real-world examples:
– Twitter: Twitter utilizes Redis for caching tweets, user profiles, and timelines to handle high read loads.
– Pinterest: Pinterest uses Redis for caching and real-time analytics to provide a fast and personalized user experience.
– GitHub: GitHub relies on Redis for rate limiting, caching, and real-time notifications to handle its large user base.
Related Article: Tutorial on Redis Docker Compose
Performance Considerations: Kafka
When considering Kafka’s performance, keep the following factors in mind:
– Kafka’s distributed architecture allows horizontal scalability, enabling high throughput and low latency.
– Efficient use of Kafka’s batch processing and compression options can significantly improve performance.
– Carefully configure the number of partitions and replication factor to ensure balanced data distribution and fault tolerance.
– Monitor and optimize consumer lag to prevent backlogs and ensure real-time data processing.
Performance Considerations: Redis
When evaluating Redis’s performance, consider the following aspects:
– Redis’s in-memory nature provides fast data access, resulting in low latency and high throughput.
– Properly configure Redis persistence mechanisms to balance data durability and performance.
– Monitor Redis memory usage and implement strategies like sharding and partitioning for horizontal scalability.
– Utilize Redis pipelining and batch operations to reduce round-trip latency and improve overall performance.
Advanced Techniques: Kafka
Kafka offers several advanced techniques to enhance its capabilities. Here are a few examples:
– Exactly-once processing: Kafka provides idempotent producers and transactional operations to achieve exactly-once processing semantics.
– Kafka Streams: Kafka Streams API allows building real-time stream processing applications directly on top of Kafka.
– Multi-tenancy: Kafka supports multi-tenancy, enabling isolation and resource management for different applications.
– MirrorMaker: Kafka’s MirrorMaker tool facilitates data replication and synchronization between Kafka clusters.
Related Article: Tutorial on Redis Sentinel: A Deep Look
Advanced Techniques: Redis
Redis provides advanced techniques to further extend its functionality. Some notable examples include:
– Lua scripting: Redis supports Lua scripting, allowing complex data manipulation and atomic operations.
– Redis Sentinel: Redis Sentinel provides high availability and automatic failover for Redis instances.
– Redis Cluster: Redis Cluster allows distributed data storage and automatic sharding for improved scalability.
– Redis Modules: Redis Modules enable extending Redis’s functionality with custom data structures and operations.
Code Snippet: Kafka Consumer
from kafka import KafkaConsumer consumer = KafkaConsumer('my-topic', bootstrap_servers='localhost:9092') for message in consumer: print(f"Received message: {message.value.decode('utf-8')}")
Code Snippet: Kafka Producer
from kafka import KafkaProducer producer = KafkaProducer(bootstrap_servers='localhost:9092') for i in range(10): message = f"Message {i}" producer.send('my-topic', message.encode('utf-8')) print(f"Sent message: {message}") producer.close()
Related Article: Tutorial: Integrating Redis with Spring Boot
Code Snippet: Redis Pub/Sub
import redis.clients.jedis.Jedis; import redis.clients.jedis.JedisPubSub; public class RedisPubSubExample { private static final String CHANNEL_NAME = "my-channel"; public static void main(String[] args) { Jedis jedis = new Jedis("localhost"); JedisPubSub jedisPubSub = new JedisPubSub() { @Override public void onMessage(String channel, String message) { System.out.println("Received message: " + message); } }; jedis.subscribe(jedisPubSub, CHANNEL_NAME); } }
Error Handling: Kafka
When working with Kafka, it’s important to consider error handling strategies. Here are some best practices:
– Implement proper error handling in both producers and consumers to handle network issues, timeouts, and other exceptions.
– Use retry mechanisms with exponential backoff to handle transient failures and ensure message delivery.
– Monitor Kafka logs and metrics to identify potential issues and take corrective actions.
– Implement proper error logging and alerting to proactively detect and address errors.
Error Handling: Redis
When using Redis, consider the following error handling practices:
– Implement error handling mechanisms, such as exception handling, in your Redis client code to handle connection failures, timeouts, and other errors.
– Use Redis transactions to ensure atomicity and consistency when executing multiple commands.
– Monitor Redis logs and metrics to identify errors and performance issues.
– Implement proper error logging and alerting to detect and address errors in a timely manner.