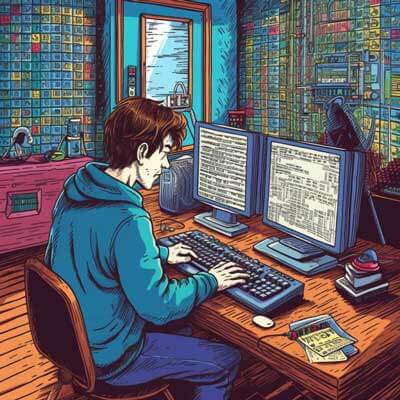
- Introduction to Redis Queue
- Basic Examples of Using Redis Queue
- Advanced Examples of Using Redis Queue
- Use Cases for Redis Queue
- Best Practices for Redis Queue
- Real World Examples of Redis Queue
- Performance Considerations for Redis Queue
- Performance Consideration: Scaling Redis Queue
- Performance Consideration: Monitoring Redis Queue
- Advanced Techniques for Redis Queue
- Advanced Technique: Priority Queues in Redis
- Advanced Technique: Delayed Queues in Redis
- Code Snippet: Adding Items to Redis Queue
- Code Snippet: Consuming Items from Redis Queue
- Code Snippet: Pausing and Resuming Redis Queue
- Error Handling in Redis Queue
Introduction to Redis Queue
Redis Queue is a useful tool that allows developers to manage job queues and distribute work across multiple workers. It is built on top of Redis, an in-memory data structure store that is commonly used for caching and real-time applications. Redis Queue provides a simple and efficient way to handle background tasks, such as processing time-consuming operations, sending emails, or generating reports. By using Redis Queue, developers can offload heavy workloads from their main application, improving performance and scalability.
Related Article: Tutorial on Installing and Using redis-cli with Redis
Basic Examples of Using Redis Queue
To start using Redis Queue, you’ll need to install the Redis and Redis Queue libraries in your project. Once installed, you can create a Redis Queue instance and start adding jobs to the queue. Here’s a basic example:
from redis import Redis from rq import Queue # Create a Redis connection redis_conn = Redis() # Create a Queue queue = Queue(connection=redis_conn) # Define a job function def process_task(task): # Process the task print("Processing task:", task) # Add a job to the queue job = queue.enqueue(process_task, "Task 1") # Wait for the job to finish job_result = job.result # Print the job result print("Job result:", job_result)
In this example, we first import the necessary libraries, create a Redis connection, and then create a Queue object using the Redis connection. We define a function process_task()
that represents the task to be executed by the worker. We enqueue a job by calling queue.enqueue()
and pass the process_task()
function and its arguments. Finally, we wait for the job to finish and print the result.
Advanced Examples of Using Redis Queue
Redis Queue provides several advanced features that can be leveraged to handle more complex job processing scenarios. Let’s explore two of these features: job dependencies and job timeouts.
Job Dependencies:
Job dependencies allow you to define dependencies between jobs, ensuring that a job is only executed once its dependent jobs have completed. This can be useful in situations where the output of one job is required as input for another job. Here’s an example:
from redis import Redis from rq import Queue # Create a Redis connection redis_conn = Redis() # Create a Queue queue = Queue(connection=redis_conn) # Define job functions def process_task1(): # Process Task 1 print("Processing Task 1") def process_task2(): # Process Task 2 print("Processing Task 2") # Add jobs to the queue with dependencies job1 = queue.enqueue(process_task1) job2 = queue.enqueue(process_task2, depends_on=job1) # Wait for the jobs to finish job1_result = job1.result job2_result = job2.result # Print the job results print("Job 1 result:", job1_result) print("Job 2 result:", job2_result)
In this example, we define two job functions process_task1()
and process_task2()
. We enqueue process_task1()
without any dependencies and then enqueue process_task2()
with a dependency on job1
. This ensures that process_task2()
is only executed after process_task1()
has completed.
Job Timeouts:
Job timeouts allow you to specify a maximum time for a job to complete. If a job exceeds the specified timeout, it is marked as failed. This can be useful to prevent jobs from running indefinitely and to handle cases where a job gets stuck or takes longer than expected. Here’s an example:
from redis import Redis from rq import Queue # Create a Redis connection redis_conn = Redis() # Create a Queue with a timeout of 10 seconds queue = Queue(connection=redis_conn, default_timeout=10) # Define a long-running job function def process_task(): # Simulate a long-running task import time time.sleep(15) print("Task completed") # Add a job to the queue job = queue.enqueue(process_task) # Wait for the job to finish or timeout job_result = job.result # Print the job result print("Job result:", job_result)
In this example, we create a Queue with a default timeout of 10 seconds. We define a job function process_task()
that simulates a long-running task using the time.sleep()
function. Since the sleep duration exceeds the timeout, the job will be marked as failed. You can handle failed jobs by implementing error handling logic.
These advanced examples showcase some of the useful features provided by Redis Queue, allowing you to handle complex job processing scenarios efficiently.
Use Cases for Redis Queue
Redis Queue is a versatile tool that can be applied to various use cases. Some common use cases for Redis Queue include:
1. Background Job Processing: Redis Queue excels at processing background jobs, such as image resizing, file processing, or sending notifications. By offloading these tasks to Redis Queue, you can free up your main application to handle user requests more efficiently.
2. Task Scheduling: Redis Queue can be used to schedule tasks to be executed at specific times or intervals. This can be useful for sending periodic reports, performing maintenance tasks, or triggering time-based events.
3. Distributed Workloads: Redis Queue supports distributed workloads, allowing you to distribute jobs across multiple workers or even multiple machines. This enables horizontal scaling and improved performance for high-demand applications.
4. Job Dependencies: Redis Queue’s job dependency feature makes it suitable for scenarios where jobs need to be executed in a specific order or have dependencies on other jobs. This can be useful for complex workflows or data processing pipelines.
5. Job Prioritization: Redis Queue supports job prioritization, allowing you to assign different priorities to jobs. This is useful when certain jobs need to be processed before others or when you want to allocate more resources to high-priority jobs.
These are just a few examples of the many use cases where Redis Queue can be applied. Its flexibility and scalability make it a valuable tool in various application scenarios.
Related Article: How to Configure a Redis Cluster
Best Practices for Redis Queue
To make the most out of Redis Queue and ensure smooth and efficient job processing, consider the following best practices:
1. Use Separate Queues: Group your jobs into separate queues based on their priority or type. This allows you to allocate resources accordingly and prevent high-priority jobs from being delayed by low-priority ones.
2. Handle Failed Jobs: Implement error handling logic to handle failed jobs. This can involve retrying failed jobs, logging errors, or notifying the appropriate stakeholders. By properly handling failures, you can maintain the reliability and stability of your job processing system.
3. Monitor Queue Performance: Monitor the performance of your Redis Queue system to identify bottlenecks, track job execution times, and ensure optimal resource utilization. Tools like Redis Monitor or RedisInsight can help in monitoring the performance of Redis and Redis Queue.
4. Optimize Job Processing: Optimize your job processing code to minimize execution time and resource usage. This can include optimizing database queries, leveraging caching, or optimizing algorithms. Efficient job processing leads to improved overall system performance.
5. Scale Redis and Workers: If your job processing demands increase, consider scaling your Redis instance and workers horizontally. This involves adding more Redis nodes or increasing the number of worker instances to handle higher job volumes. Load balancing techniques can be employed to distribute the workload evenly.
Real World Examples of Redis Queue
Redis Queue is widely adopted in various industries and has proven to be a valuable tool for managing job queues. Here are some real-world examples of how Redis Queue is used:
1. E-commerce Order Processing: In e-commerce applications, Redis Queue is often used to handle order processing tasks, such as inventory updates, order fulfillment, and email notifications. By offloading these tasks to Redis Queue, the main application can focus on serving user requests and providing a responsive user experience.
2. Background Image Processing: Image-heavy applications, such as photo sharing platforms or media processing services, utilize Redis Queue to process image uploads, generate thumbnails, or apply image filters in the background. Redis Queue allows these operations to be performed asynchronously, ensuring a smooth user experience.
3. Task Scheduling in Web Applications: Web applications often require scheduled tasks to be executed at specific times or intervals, such as sending reminders, generating reports, or performing database backups. Redis Queue’s task scheduling capabilities make it an ideal choice for handling these recurring tasks reliably and efficiently.
4. Data Processing Pipelines: Redis Queue’s job dependency feature makes it well-suited for data processing pipelines, where multiple jobs need to be executed in a specific order or have dependencies on each other. This is commonly seen in data analytics platforms or data integration workflows.
These examples highlight the versatility and effectiveness of Redis Queue in real-world scenarios, where it helps improve application performance, scalability, and reliability.
Performance Considerations for Redis Queue
When using Redis Queue, it is important to consider performance aspects to ensure efficient job processing. Here are some performance considerations to keep in mind:
1. Job Payload Size: Keep the size of job payloads as small as possible to reduce memory usage and network overhead. If a job requires large data or files, consider using external storage or passing references instead of embedding the data directly in the job payload.
2. Redis Memory Usage: Monitor the memory usage of your Redis instance, as Redis Queue relies on Redis for storing job data. If the memory usage becomes a bottleneck, consider optimizing your job processing code or scaling your Redis instance.
3. Worker Concurrency: Adjust the number of worker processes or threads based on the available system resources and the workload characteristics. Increasing the concurrency can improve job processing speed, but be cautious not to overload the system.
4. Connection Pooling: Use connection pooling to manage Redis connections efficiently. Creating a new connection for each job can result in increased overhead. Connection pooling libraries, such as redis-py
for Python, can help manage connections effectively.
5. Batch Processing: If your jobs involve similar or repetitive tasks, consider batching them together to reduce the overhead of individual job enqueueing and processing. This can significantly improve performance, especially for jobs with high latency.
Related Article: Redis vs MongoDB: A Detailed Comparison
Performance Consideration: Scaling Redis Queue
Scaling Redis Queue involves horizontally adding more Redis instances or worker processes to handle increased job volumes. Here are some considerations for scaling Redis Queue:
1. Redis Cluster: Redis Cluster allows you to scale Redis horizontally by distributing the data across multiple Redis instances. It provides automatic sharding and failover capabilities, ensuring high availability and improved performance. Consider using Redis Cluster when you need to handle large job volumes.
2. Worker Scaling: To handle increased job volumes, you can scale the number of worker processes or threads. This can be achieved by running multiple worker instances, each connected to the same Redis Queue. Load balancing techniques, such as round-robin or hashing, can be used to distribute the workload evenly across the workers.
3. Load Balancing: When scaling Redis Queue, consider implementing load balancing techniques to distribute the job processing workload across multiple instances or machines. Load balancers can ensure that jobs are evenly distributed, preventing overload on specific Redis instances or worker processes.
4. Redis Sentinel: Redis Sentinel provides high availability and failover capabilities for Redis instances. By using Redis Sentinel, you can ensure that Redis instances are always available, even in the event of failures. This helps maintain the reliability and stability of your Redis Queue system.
5. Monitoring and Optimization: When scaling Redis Queue, closely monitor the performance and resource utilization of your Redis instances and worker processes. Optimize your code and configuration based on the observed metrics to ensure optimal scalability and performance.
Performance Consideration: Monitoring Redis Queue
Monitoring the performance of your Redis Queue system is crucial to identify bottlenecks, track job execution times, and optimize resource allocation. Here are some aspects to consider when monitoring Redis Queue:
1. Redis Metrics: Monitor key Redis metrics such as memory usage, CPU utilization, and network traffic. Redis provides commands and tools to retrieve these metrics. External monitoring tools like Prometheus or Datadog can also be used to collect and visualize Redis metrics.
2. Job Execution Time: Track the execution time of your jobs to identify slow-performing tasks. This can help pinpoint performance bottlenecks and optimize job processing code. You can measure the execution time within your job functions or by using Redis Queue’s built-in monitoring capabilities.
3. Queue Length and Backlogs: Monitor the length of your queues to ensure they don’t become a bottleneck. Long queues can indicate that your system is unable to process jobs at the required pace. Consider adjusting the number of workers or scaling your Redis instance to handle increased job volumes.
4. Failed Jobs: Keep track of failed jobs and analyze the error logs to identify recurring issues or patterns. By proactively addressing failed jobs, you can improve the overall reliability and stability of your Redis Queue system.
5. Worker Performance: Monitor the performance of your worker processes or threads to ensure they are efficiently utilizing system resources. High CPU or memory usage may indicate suboptimal code or resource leaks. Use monitoring tools to capture worker-level metrics.
Advanced Techniques for Redis Queue
Redis Queue offers several advanced techniques that can enhance the functionality and flexibility of your job processing system. Let’s explore two advanced techniques: priority queues and delayed queues.
Related Article: Tutorial on Redis Queue Implementation
Advanced Technique: Priority Queues in Redis
Redis Queue supports priority queues, allowing you to assign different priorities to jobs. This enables you to control the order in which jobs are processed, ensuring that high-priority jobs are executed before lower-priority ones. Here’s an example of using priority queues in Redis Queue:
from redis import Redis from rq import Queue # Create a Redis connection redis_conn = Redis() # Create a priority Queue queue = Queue(connection=redis_conn, default_timeout=10, job_class='rq.job.PriorityJob') # Define job functions def process_high_priority_task(task): # Process high-priority task print("Processing high-priority task:", task) def process_low_priority_task(task): # Process low-priority task print("Processing low-priority task:", task) # Add jobs to the queue with different priorities high_priority_job = queue.enqueue(process_high_priority_task, "High Priority Task", priority=1) low_priority_job = queue.enqueue(process_low_priority_task, "Low Priority Task", priority=2) # Wait for the jobs to finish high_priority_result = high_priority_job.result low_priority_result = low_priority_job.result # Print the job results print("High priority job result:", high_priority_result) print("Low priority job result:", low_priority_result)
In this example, we create a priority Queue by specifying the job_class
parameter as 'rq.job.PriorityJob'
. We define two job functions process_high_priority_task()
and process_low_priority_task()
. We enqueue a high-priority job and a low-priority job by setting the priority
parameter. Redis Queue ensures that high-priority jobs are processed before low-priority jobs.
Advanced Technique: Delayed Queues in Redis
Redis Queue supports delayed queues, allowing you to schedule jobs to be executed at a specific time in the future. This is useful when you need to defer the execution of certain tasks or schedule jobs in advance. Here’s an example of using delayed queues in Redis Queue:
from redis import Redis from rq import Queue # Create a Redis connection redis_conn = Redis() # Create a delayed Queue queue = Queue(connection=redis_conn, job_class='rq.job.DQJob') # Define a delayed job function def process_delayed_task(task): # Process delayed task print("Processing delayed task:", task) # Enqueue a delayed job delayed_job = queue.enqueue_at(datetime(2022, 1, 1, 0, 0), process_delayed_task, "Delayed Task") # Wait for the delayed job to be scheduled time.sleep(5) # Wait for the delayed job to finish delayed_result = delayed_job.result # Print the delayed job result print("Delayed job result:", delayed_result)
In this example, we create a delayed Queue by specifying the job_class
parameter as 'rq.job.DQJob'
. We define a delayed job function process_delayed_task()
and enqueue a job using queue.enqueue_at()
with a specific datetime object representing the desired execution time. Redis Queue ensures that the job is executed at the specified time.
These advanced techniques provide additional flexibility and control over job processing with Redis Queue, allowing you to handle complex scenarios and meet specific requirements.
Code Snippet: Adding Items to Redis Queue
Adding items to a Redis Queue involves enqueuing jobs with the desired task functions and arguments. Here’s a code snippet demonstrating how to add items to a Redis Queue using Python:
from redis import Redis from rq import Queue # Create a Redis connection redis_conn = Redis() # Create a Queue queue = Queue(connection=redis_conn) # Define a job function def process_task(task): # Process the task print("Processing task:", task) # Add items to the queue queue.enqueue(process_task, "Task 1") queue.enqueue(process_task, "Task 2") queue.enqueue(process_task, "Task 3")
In this code snippet, we create a Redis connection and a Queue using the Redis
and Queue
classes from the redis
and rq
libraries respectively. We define a job function process_task()
that represents the task to be executed by the worker. We then enqueue three jobs using queue.enqueue()
and pass the process_task()
function and the corresponding task arguments.
This code snippet demonstrates the basic process of adding items to a Redis Queue. You can customize the tasks and arguments based on your specific application requirements.
Related Article: Tutorial: Installing Redis on Ubuntu
Code Snippet: Consuming Items from Redis Queue
Consuming items from a Redis Queue involves running worker processes that continuously pull and process jobs from the queue. Here’s a code snippet demonstrating how to consume items from a Redis Queue using Python:
from redis import Redis from rq import Queue, Worker # Create a Redis connection redis_conn = Redis() # Create a Queue queue = Queue(connection=redis_conn) # Define a job function def process_task(task): # Process the task print("Processing task:", task) # Create a worker and start consuming jobs from the queue worker = Worker([queue]) worker.work()
In this code snippet, we create a Redis connection and a Queue using the Redis
and Queue
classes. We define a job function process_task()
that represents the task to be executed by the worker. We then create a worker using the Worker
class and pass the queue to consume jobs from. Finally, we start the worker using worker.work()
.
This code snippet demonstrates the basic process of consuming items from a Redis Queue. The worker continuously checks for new jobs in the queue and processes them using the specified task function.
Code Snippet: Pausing and Resuming Redis Queue
Pausing and resuming a Redis Queue allows you to control the processing of jobs temporarily. Here’s a code snippet demonstrating how to pause and resume a Redis Queue using Python:
from redis import Redis from rq import Queue # Create a Redis connection redis_conn = Redis() # Create a Queue queue = Queue(connection=redis_conn) # Pause the queue queue.pause() # Enqueue jobs while the queue is paused queue.enqueue(process_task, "Paused Task 1") queue.enqueue(process_task, "Paused Task 2") queue.enqueue(process_task, "Paused Task 3") # Resume the queue queue.resume() # Enqueue jobs after resuming the queue queue.enqueue(process_task, "Resumed Task 1") queue.enqueue(process_task, "Resumed Task 2") queue.enqueue(process_task, "Resumed Task 3")
In this code snippet, we create a Redis connection and a Queue using the Redis
and Queue
classes. We pause the queue using queue.pause()
and enqueue some jobs while the queue is paused. We then resume the queue using queue.resume()
and enqueue additional jobs.
This code snippet demonstrates how to pause and resume a Redis Queue to control the processing of jobs. Pausing the queue temporarily halts the processing of new jobs, while resuming the queue allows the processing to continue.
Error Handling in Redis Queue
Error handling is an essential aspect of Redis Queue to ensure the reliability and stability of your job processing system. Here are some error handling techniques to consider:
1. Retry Failed Jobs: Implement retry logic for failed jobs to handle transient failures or temporary issues. You can configure Redis Queue to automatically retry failed jobs a certain number of times or until they succeed. This can be achieved by setting the max_retries
parameter when enqueueing jobs.
2. Error Logging: Log errors and exceptions encountered during job processing. This allows you to investigate and debug issues, analyze recurring failures, and identify areas for improvement. Use a logging library or framework to capture and store error logs.
3. Failed Job Callbacks: Redis Queue provides the ability to register callbacks that are executed when a job fails. You can use this feature to implement custom error handling logic, such as sending notifications or performing cleanup tasks. By leveraging these callbacks, you can respond to failed jobs in a tailored manner.
4. Dead Letter Queues: Consider using a dead letter queue to handle jobs that repeatedly fail or cannot be processed successfully. Dead letter queues act as a separate queue where failed jobs are moved, allowing you to analyze and troubleshoot them without affecting the main job processing flow.
5. Graceful Shutdown: Handle graceful shutdowns to ensure that jobs in progress are not abruptly terminated. Graceful shutdown involves allowing currently running jobs to complete before shutting down the worker processes or Redis instances. This prevents data corruption or job loss during system shutdowns or upgrades.