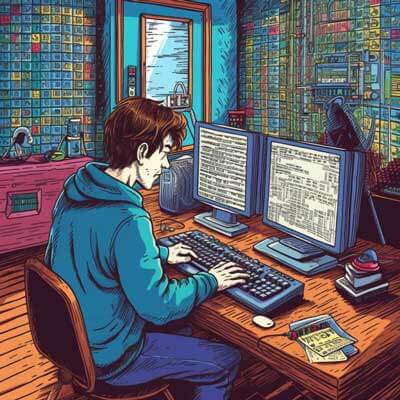
- Introduction to Django Redis
- Setting Up Redis for Django
- Code Snippet: Starting Redis Server
- Configuring Django to Use Redis
- Connecting Django Models to Redis
- Using Redis for Caching in Django
- Using Redis for Session Storage in Django
- Using Redis Pub/Sub in Django
- Using Redis for Rate Limiting in Django
- Using Redis for Task Queues in Django
- Use Case: Real-Time Analytics with Django and Redis
- Use Case: Real-Time Chat Application with Django and Redis
- Best Practices for Using Redis with Django
- Performance Considerations with Redis and Django
- Example: Building a Scalable Django App with Redis
- Example: Implementing Real-Time Notifications with Django and Redis
- Advanced Technique: Using Redis Lua Scripting with Django
- Advanced Technique: Using Redis Streams with Django
- Error Handling in Django Redis
Introduction to Django Redis
Django Redis is a very useful tool that allows developers to integrate Redis, an open-source in-memory data structure store, with Django, a popular Python web framework. By leveraging the features of Redis, Django applications can benefit from high-performance caching, session storage, real-time messaging, rate limiting, task queues, and more. In this chapter, we will explore the various use cases and techniques for using Django Redis to enhance the functionality and performance of Django applications.
Related Article: Tutorial on Rust Redis: Tools and Techniques
Setting Up Redis for Django
Before we can start using Django Redis, we need to set up Redis on our system. Here are the steps to install and configure Redis:
1. Download and install Redis from the official website or using package managers like apt or brew.
2. Start the Redis server by running the appropriate command for your system.
3. Verify that Redis is running by using the Redis CLI and executing the ping
command.
Code Snippet: Starting Redis Server
redis-server
Configuring Django to Use Redis
To use Redis as the backend for caching, session storage, or other functionalities in Django, we need to configure Django settings to connect to the Redis server. Here’s an example of how to configure Django to use Redis:
1. Install the django-redis
package using pip.
2. Add 'django_redis'
to the INSTALLED_APPS
list in your Django settings file.
3. Configure the Redis connection settings by adding the following code to your settings file:
CACHES = { 'default': { 'BACKEND': 'django_redis.cache.RedisCache', 'LOCATION': 'redis://localhost:6379/1', 'OPTIONS': { 'CLIENT_CLASS': 'django_redis.client.DefaultClient', } } } SESSION_ENGINE = 'django.contrib.sessions.backends.cache' SESSION_CACHE_ALIAS = 'default'
In the above example, we configure Redis as the backend for both the cache and session storage. The LOCATION
parameter specifies the Redis server’s URL, and the CLIENT_CLASS
parameter sets the client class to the default Django Redis client.
Related Article: Redis vs MongoDB: A Detailed Comparison
Connecting Django Models to Redis
Django Redis provides a convenient way to connect Django models to Redis, allowing efficient storage and retrieval of data. To connect Django models to Redis, follow these steps:
1. Install the django-redis
package if you haven’t already.
2. Import the necessary classes from django_redis
in your models file.
3. Create a Redis connection using the get_redis_connection
function.
4. Use the Redis connection to perform operations on Redis, such as storing and retrieving data.
Here’s an example of how to connect a Django model to Redis and store/retrieve data:
from django_redis import get_redis_connection from django.db import models class ExampleModel(models.Model): name = models.CharField(max_length=100) redis_connection = get_redis_connection() def save(self, *args, **kwargs): self.redis_connection.set(self.name, self.name) super().save(*args, **kwargs) def retrieve_data(self): return self.redis_connection.get(self.name)
In the above example, we create a Redis connection using the get_redis_connection
function and store the model’s name as a key-value pair in Redis. The retrieve_data
method retrieves the value associated with the key from Redis.
Using Redis for Caching in Django
Caching is a common technique used to improve the performance of web applications by storing frequently accessed data in memory. Django Redis provides a powerful caching backend that integrates seamlessly with Django’s cache framework. To use Redis for caching in Django, follow these steps:
1. Configure Django to use Redis as the cache backend, as discussed in the previous chapter.
2. Use the Django cache API to store and retrieve data from the cache.
Here’s an example of how to use Redis for caching in Django:
from django.core.cache import cache def get_data(): data = cache.get('my_data') if data is None: # Retrieve data from the database or another source data = retrieve_data() cache.set('my_data', data, timeout=3600) # Cache for 1 hour return data
In the above example, we use the cache
object provided by Django to store and retrieve data from the cache. If the data is not found in the cache, we retrieve it from the database or another source and cache it for future use.
Using Redis for Session Storage in Django
Django Redis also offers a session backend that allows storing session data in Redis. Storing session data in Redis can provide better scalability and performance compared to using the default database backend. To use Redis for session storage in Django, follow these steps:
1. Configure Django to use Redis as the session backend, as discussed in the previous chapter.
2. Access and manipulate session data using Django’s session API.
Here’s an example of how to use Redis for session storage in Django:
def set_session_data(request): request.session['username'] = 'john' request.session['is_logged_in'] = True def get_session_data(request): username = request.session.get('username') is_logged_in = request.session.get('is_logged_in') return username, is_logged_in
In the above example, we use the request.session
object provided by Django to set and retrieve session data. The session data is stored in Redis, allowing it to be accessed across multiple requests and servers.
Related Article: How To Reorder Columns In Python Pandas Dataframe
Using Redis Pub/Sub in Django
Redis Pub/Sub (Publish/Subscribe) is a messaging pattern that allows communication between different parts of an application. It enables real-time messaging and event-driven architectures. Django Redis provides support for Pub/Sub, allowing Django applications to leverage this powerful feature. To use Redis Pub/Sub in Django, follow these steps:
1. Create a Redis Pub/Sub connection using the get_redis_connection
function.
2. Use the connection to publish messages to channels or subscribe to channels and receive messages.
Here’s an example of how to use Redis Pub/Sub in Django:
from django_redis import get_redis_connection def publish_message(channel, message): redis_connection = get_redis_connection() redis_connection.publish(channel, message) def subscribe_channel(channel): redis_connection = get_redis_connection() pubsub = redis_connection.pubsub() pubsub.subscribe(channel) for message in pubsub.listen(): # Handle the received message handle_message(message)
In the above example, we use the get_redis_connection
function to create a Redis connection for Pub/Sub. We can then publish messages to a specific channel or subscribe to a channel and receive messages using the pubsub
object.
Using Redis for Rate Limiting in Django
Rate limiting is an essential technique to control the number of requests or operations performed within a specific time frame. Django Redis offers rate limiting functionality that can be used to prevent abuses, protect resources, and ensure fair usage. To use Redis for rate limiting in Django, follow these steps:
1. Configure Django to use Redis as the cache backend, as discussed in a previous chapter.
2. Use Django’s cache API to implement rate limiting logic.
Here’s an example of how to use Redis for rate limiting in Django:
from django.core.cache import cache from django.core.exceptions import ValidationError def perform_operation(user_id): key = f'rate_limit:{user_id}' count = cache.get(key) if count is None: count = 1 else: count += 1 cache.set(key, count, timeout=60) # Limit to 1 request per minute if count > 10: raise ValidationError('Rate limit exceeded') else: # Perform the operation perform_operation_logic()
In the above example, we use Django’s cache API to implement a simple rate limiting mechanism. We store the count of requests made by a user within a specific time frame in Redis. If the count exceeds a predefined limit, we raise a ValidationError
to indicate that the rate limit has been exceeded.
Using Redis for Task Queues in Django
Task queues are essential for executing background or long-running tasks asynchronously. Django Redis provides integration with Redis task queues, allowing developers to offload resource-intensive or time-consuming tasks from the main application flow. To use Redis for task queues in Django, follow these steps:
1. Install the django-rq
package using pip.
2. Create a Redis connection using the get_redis_connection
function.
3. Use the connection to enqueue tasks using the django_rq.enqueue
decorator or function.
Here’s an example of how to use Redis for task queues in Django:
from django_rq import job from django_redis import get_redis_connection @job def process_data(data): # Perform the data processing logic process_data_logic(data) def enqueue_task(data): redis_connection = get_redis_connection() process_data.delay(data)
In the above example, we use the django_rq
package to integrate Redis task queues with Django. We define a task using the @job
decorator and enqueue it using the delay
method. The task will be executed asynchronously, allowing the main application to continue processing other requests.
Related Article: How To Write Pandas Dataframe To CSV File
Use Case: Real-Time Analytics with Django and Redis
Real-time analytics is crucial for applications that require up-to-date insights and metrics. By combining Django and Redis, we can build a real-time analytics system that handles high volumes of data and provides timely information. In this use case, we will explore how to implement real-time analytics with Django and Redis.
Example 1: Tracking Page Views in Real-Time
from django_redis import get_redis_connection from django.http import HttpResponse def track_page_view(request): redis_connection = get_redis_connection() redis_connection.incr('page_views') return HttpResponse('Page view tracked successfully')
In the above example, we use Redis to track page views in real-time. Each time a user visits a page, we increment the page_views
key in Redis. This allows us to keep track of the total number of page views and retrieve real-time analytics.
Example 2: Displaying Real-Time Analytics
from django_redis import get_redis_connection from django.shortcuts import render def display_real_time_analytics(request): redis_connection = get_redis_connection() page_views = redis_connection.get('page_views') return render(request, 'analytics.html', {'page_views': page_views})
In this example, we retrieve the real-time analytics, such as the total number of page views, from Redis and display them in a template using Django’s rendering functionality.
Use Case: Real-Time Chat Application with Django and Redis
Real-time chat applications require efficient and scalable communication between users. By combining Django and Redis, we can build a real-time chat application that can handle a large number of concurrent users and deliver messages in real-time. In this use case, we will explore how to implement a real-time chat application with Django and Redis.
Example 1: Sending and Receiving Messages
from django_redis import get_redis_connection from django.http import HttpResponse def send_message(request, room_id, message): redis_connection = get_redis_connection() redis_connection.publish(f'chat:{room_id}', message) return HttpResponse('Message sent successfully') def receive_message(request, room_id): redis_connection = get_redis_connection() pubsub = redis_connection.pubsub() pubsub.subscribe(f'chat:{room_id}') for message in pubsub.listen(): # Handle the received message handle_message(message)
In the above example, we use Redis Pub/Sub to send and receive messages in real-time. The send_message
function publishes a message to a specific chat room, while the receive_message
function subscribes to the chat room’s channel and listens for incoming messages.
Example 2: Displaying Real-Time Chat
from django_redis import get_redis_connection from django.shortcuts import render def display_chat(request, room_id): redis_connection = get_redis_connection() pubsub = redis_connection.pubsub() pubsub.subscribe(f'chat:{room_id}') messages = [] for message in pubsub.listen(): # Add the received message to the list of messages messages.append(message) return render(request, 'chat.html', {'messages': messages})
In this example, we retrieve the real-time chat messages from Redis and display them in a template using Django’s rendering functionality.
Best Practices for Using Redis with Django
When using Redis with Django, it is important to follow best practices to ensure optimal performance and reliability. Here are some best practices for using Redis with Django:
1. Use connection pooling: Configure Django to use a connection pool for Redis, which improves performance by reusing connections instead of creating new ones for each request.
2. Implement data serialization: Serialize complex data structures before storing them in Redis to ensure compatibility and efficient storage.
3. Set appropriate timeouts: Set appropriate timeouts for cached data, session data, and other Redis-backed functionalities to balance performance and data freshness.
4. Monitor Redis usage: Monitor Redis metrics to identify potential bottlenecks, such as high memory usage or slow queries, and optimize accordingly.
5. Handle Redis failures gracefully: Implement error handling and fallback mechanisms to handle Redis failures and provide fallback behavior when Redis is unavailable.
6. Secure Redis: Configure Redis security settings to protect sensitive data and prevent unauthorized access.
Related Article: How to Access Python Data Structures with Square Brackets
Performance Considerations with Redis and Django
When using Redis with Django, it is important to consider performance considerations to ensure optimal performance and scalability. Here are some performance considerations to keep in mind:
1. Use Redis data structures effectively: Choose the appropriate Redis data structures, such as strings, lists, sets, or sorted sets, depending on the use case to maximize performance and efficiency.
2. Batch operations: Whenever possible, use batch operations to minimize round trips to Redis and improve performance. For example, use the pipeline
feature to execute multiple commands in a single round trip.
3. Use Redis Lua scripting: Leverage Redis Lua scripting to reduce the number of network round trips and execute complex operations atomically on the server side.
4. Monitor Redis performance: Regularly monitor Redis performance using tools like Redis CLI or third-party monitoring solutions to identify bottlenecks, optimize queries, and fine-tune Redis configuration.
5. Optimize cache usage: Carefully choose what data to cache and set appropriate cache expiration times to balance performance and data freshness.
6. Scale Redis: If the workload exceeds the capacity of a single Redis instance, consider using Redis Cluster or Redis Sentinel to distribute the load and improve scalability.
Example: Building a Scalable Django App with Redis
Building a scalable Django application requires careful consideration of performance, reliability, and scalability. By leveraging Redis, we can enhance the scalability of a Django app by offloading resource-intensive tasks, implementing caching, and managing real-time communication. Here’s an example of how to build a scalable Django app using Redis:
1. Implement Redis caching to reduce the load on the database and improve response times.
2. Use Redis task queues to offload background tasks and long-running processes, allowing the main application to handle requests more efficiently.
3. Utilize Redis Pub/Sub to implement real-time communication for features like chat, notifications, or real-time analytics.
4. Configure Redis as the session backend to improve scalability and performance for session management.
5. Monitor Redis performance and usage to identify bottlenecks and optimize Redis configuration.
6. Scale the Redis infrastructure as needed, either by using Redis Sentinel for high availability or Redis Cluster for horizontal scaling.
Example: Implementing Real-Time Notifications with Django and Redis
Real-time notifications are a common requirement in modern web applications. By using Django and Redis, we can implement a real-time notification system that delivers notifications to users in real-time. Here’s an example of how to implement real-time notifications with Django and Redis:
1. Create a Redis Pub/Sub channel for notifications.
2. Implement a Django view or API endpoint that publishes notifications to the Redis Pub/Sub channel.
3. Use JavaScript and websockets to listen for notifications on the client-side and display them to the user in real-time.
4. When a user performs an action that triggers a notification, publish the notification message to the Redis Pub/Sub channel.
5. On the client-side, establish a websocket connection and subscribe to the Redis Pub/Sub channel to receive real-time notifications.
6. Handle incoming notifications on the client-side and display them to the user using HTML and JavaScript.
Related Article: Deploying Flask Web Apps: From WSGI to Kubernetes
Advanced Technique: Using Redis Lua Scripting with Django
Redis Lua scripting allows executing complex operations atomically on the server side, reducing the number of network round trips and improving performance. Django Redis provides support for Redis Lua scripting, enabling developers to leverage this powerful feature. Here’s an example of how to use Redis Lua scripting with Django:
from django_redis import get_redis_connection def perform_atomic_operation(): redis_connection = get_redis_connection() script = """ local count = tonumber(redis.call('get', KEYS[1]) or 0) count = count + tonumber(ARGV[1]) redis.call('set', KEYS[1], count) return count """ result = redis_connection.eval(script, 1, 'counter', 5) return result
In the above example, we define a Lua script that retrieves the value of a key, increments it by a specified amount, and sets the new value. The script is executed atomically on the Redis server using the eval
method provided by Django Redis.
Advanced Technique: Using Redis Streams with Django
Redis Streams is a powerful data structure that provides a log-like data structure with automatic trimming and retention capabilities. Django Redis supports Redis Streams, allowing developers to leverage this feature in Django applications. Here’s an example of how to use Redis Streams with Django:
from django_redis import get_redis_connection def add_event(event_data): redis_connection = get_redis_connection() redis_connection.xadd('events', event_data)
In the above example, we use the xadd
method provided by Django Redis to add an event to a Redis Stream named ‘events’. The event data is passed as a dictionary and automatically assigned a unique ID by Redis.
Error Handling in Django Redis
When working with Django Redis, it is essential to handle errors gracefully to ensure the stability and reliability of the application. Here are some key points to consider when handling errors in Django Redis:
1. Catch and handle Redis connection errors: Wrap Redis operations with appropriate exception handling to handle connection errors and provide fallback behavior if Redis is unavailable.
2. Monitor Redis errors: Monitor Redis error logs and metrics to identify potential issues and take appropriate actions to address them.
3. Implement retry mechanisms: Implement retry logic for critical Redis operations to handle transient errors or network issues.
4. Use Django’s logging framework: Utilize Django’s logging framework to log Redis-related errors and exceptions for debugging and monitoring purposes.
5. Test error scenarios: Test error scenarios, such as Redis being unavailable or experiencing high latencies, to ensure that the application handles them gracefully without crashing or causing unexpected behavior.