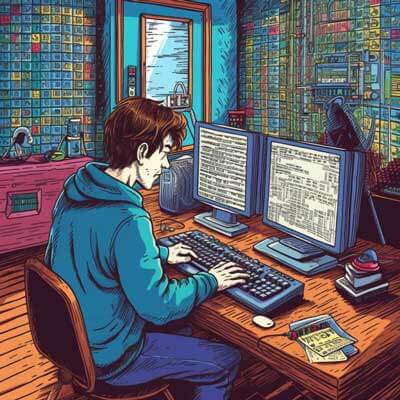
- Introduction to Redis Streams
- Installation and Setup
- Basic Operations
- Stream Data Structure
- Stream Grouping
- Consumer Groups
- Stream Commands
- Use Cases: Real-Time Analytics
- Use Cases: Messaging and Chat Applications
- Use Cases: Event Sourcing
- Best Practices: Naming Conventions
- Best Practices: Error Handling
- Best Practices: Performance Optimization
- Real World Example: Building a Real-Time Dashboard
- Real World Example: Implementing a Message Queue
- Real World Example: Event-Driven Architecture
- Performance Considerations: Memory Management
- Performance Considerations: Scaling
- Performance Considerations: Latency
- Advanced Technique: Stream Aggregation
- Advanced Technique: Stream Joins
- Advanced Technique: Stream Processing
- Code Snippet: Publishing to a Stream
- Code Snippet: Consuming from a Stream
- Code Snippet: Stream Group Creation
- Error Handling: Handling Stream Errors
- Error Handling: Error Logging and Monitoring
Introduction to Redis Streams
Redis Streams is a feature introduced in Redis version 5.0 that provides a robust and scalable data structure for handling continuous streams of data. It is designed to handle real-time data processing and can be used in various use cases such as real-time analytics, messaging and chat applications, and event sourcing.
Unlike traditional databases, Redis Streams allows you to append new data to a stream and consume it in a sequential manner. Each data entry in a stream is identified by a unique ID, which can be used for ordering and retrieval purposes. Streams can also be grouped and consumed by multiple consumers, enabling efficient message distribution and load balancing.
Related Article: Tutorial on Installing and Using redis-cli with Redis
Installation and Setup
To start using Redis Streams, you need to have Redis version 5.0 or above installed on your system. If you don’t have Redis installed, you can follow the official Redis installation guide for your specific operating system.
Once Redis is installed, you can start the Redis server by running the following command:
redis-server
Redis Streams does not require any additional setup or configuration. It is built into Redis itself and can be accessed using the Redis command-line interface (CLI) or any Redis client library.
Basic Operations
Redis Streams provides a set of commands for performing basic operations on streams. Here are a few examples:
1. Creating a Stream:
XADD mystream * field1 value1 field2 value2
This command creates a new stream called “mystream” and appends a new entry to it with the specified fields and values. The special character “*” is used to generate a unique ID for the entry.
2. Reading from a Stream:
XREAD COUNT 10 STREAMS mystream 0
This command reads the last 10 entries from the “mystream” stream starting from the beginning (ID 0). The result is returned as an array of arrays, where each sub-array represents a stream and its entries.
3. Deleting a Stream:
XDEL mystream entry1 entry2
This command deletes the specified entries from the “mystream” stream. You can provide one or more entry IDs to be deleted.
These are just a few examples of the basic operations available in Redis Streams. The complete list of commands and their usage can be found in the Redis documentation.
Stream Data Structure
In Redis Streams, data is organized in a stream data structure, which is essentially an append-only log. Each entry in the stream is a key-value pair, where the key represents the unique ID of the entry and the value contains the actual data.
The ID of an entry consists of two parts: the time of the entry and a sequence number. The time part ensures that the entries are ordered chronologically, while the sequence number ensures uniqueness within a given millisecond.
Here is an example of a stream data structure:
mystream - 1530000000001-0 field1: value1 field2: value2 - 1530000000002-0 field1: value3 field2: value4 field3: value5
In this example, “mystream” is the name of the stream. The entries are identified by their IDs, such as “1530000000001-0” and “1530000000002-0”. Each entry can have multiple fields with corresponding values.
Related Article: How to Configure a Redis Cluster
Stream Grouping
Stream grouping is a useful feature of Redis Streams that allows multiple consumers to consume a stream in a coordinated manner. Consumers can form consumer groups and each group can have multiple consumers.
When a stream is consumed by a consumer group, the entries in the stream are distributed among the consumers in a round-robin fashion. This ensures that each consumer in the group gets an equal share of the workload.
To create a consumer group and join it, you can use the following command:
XGROUP CREATE mystream mygroup 0 XREADGROUP GROUP mygroup consumer1 COUNT 10 STREAMS mystream >
In this example, “mystream” is the name of the stream and “mygroup” is the name of the consumer group. The “0” after the consumer group name specifies the initial ID to start consuming from. The “XREADGROUP” command is used to read entries from the stream within the consumer group.
Consumer Groups
Consumer groups in Redis Streams provide fault-tolerant message consumption. If a consumer fails or disconnects, the other consumers in the group will take over the processing of the unacknowledged entries.
Each consumer in a group maintains its own internal state, including the last ID of the consumed entry. This allows consumers to resume consuming from where they left off in case of failures.
To acknowledge the processing of an entry, you can use the following command:
XACK mystream mygroup 1530000000001-0
This command acknowledges the processing of the entry with ID “1530000000001-0” in the consumer group “mygroup”. Once an entry is acknowledged, it is marked as processed and will not be delivered to other consumers in the group.
Stream Commands
Redis Streams provides a rich set of commands for working with streams. Here are a few commonly used commands:
1. XADD: Appends a new entry to a stream.
2. XLEN: Returns the number of entries in a stream.
3. XREAD: Reads entries from one or more streams.
4. XRANGE: Returns a range of entries from a stream based on the IDs.
5. XTRIM: Trims a stream by removing old entries.
6. XGROUP: Creates a new consumer group or manages existing groups.
7. XACK: Acknowledges the processing of an entry in a consumer group.
These commands, along with many others, provide a comprehensive set of functionalities for working with Redis Streams.
Related Article: Redis vs MongoDB: A Detailed Comparison
Use Cases: Real-Time Analytics
Redis Streams is well-suited for real-time analytics applications where data needs to be processed as it arrives. Here’s an example of how Redis Streams can be used for real-time analytics:
import redis r = redis.Redis() # Append new data to the stream r.xadd('analytics', {'metric': 'clicks', 'value': 10}) r.xadd('analytics', {'metric': 'clicks', 'value': 15}) r.xadd('analytics', {'metric': 'clicks', 'value': 5}) # Aggregate data by metric result = r.xread({'analytics': '$'}, count=3) analytics = result[0][1] clicks = sum(int(entry['value']) for entry in analytics if entry['metric'] == 'clicks') print(f'Total clicks: {clicks}')
In this example, we create an “analytics” stream and append new entries to it representing different metrics. We then read the entries from the stream and aggregate the data by a specific metric, in this case, “clicks”. The total number of clicks is then calculated and printed.
Use Cases: Messaging and Chat Applications
Redis Streams can be used as a useful data structure for building messaging and chat applications. It provides the ability to store and consume messages in an ordered manner, making it ideal for real-time communication. Here’s an example of how Redis Streams can be used for messaging:
import redis r = redis.Redis() # Publish a message to a stream r.xadd('messages', {'sender': 'user1', 'message': 'Hello, World!'}) # Consume messages from the stream result = r.xread({'messages': '0'}, count=1) message = result[0][1][0] print(f'{message["sender"]}: {message["message"]}')
In this example, we publish a new message to the “messages” stream and consume the messages from the stream. The latest message is then printed to the console.
Use Cases: Event Sourcing
Event sourcing is a design pattern where the state of an application is derived from a series of events. Redis Streams can be used as a reliable and scalable storage solution for event sourcing. Here’s an example of how Redis Streams can be used for event sourcing:
import redis r = redis.Redis() # Append new events to the stream r.xadd('events', {'event_type': 'user_created', 'user_id': '123', 'name': 'John Doe'}) r.xadd('events', {'event_type': 'user_updated', 'user_id': '123', 'name': 'Jane Doe'}) # Retrieve events for a specific user result = r.xrange('events', '-', '+') events = [entry[1] for entry in result if entry[1]['user_id'] == '123'] print(events)
In this example, we create an “events” stream and append new events to it representing different actions performed on a user. We then retrieve the events for a specific user and store them in a list for further processing.
Related Article: Tutorial on Redis Queue Implementation
Best Practices: Naming Conventions
When working with Redis Streams, it is important to follow certain naming conventions to ensure consistency and readability. Here are some best practices for naming streams, consumer groups, and entries:
1. Use meaningful and descriptive names for streams and consumer groups. This helps in identifying the purpose of the stream or group.
2. Avoid using special characters, spaces, or uppercase letters in the names. Stick to lowercase alphanumeric characters and underscores.
3. Use a consistent naming convention across your application to make it easier to understand and maintain.
Best Practices: Error Handling
Error handling is an important aspect of any application, and Redis Streams is no exception. Here are some best practices for error handling in Redis Streams:
1. Check the return values of Redis commands to detect and handle errors. Redis commands typically return a status code or an error message.
2. Use try-catch blocks or exception handling mechanisms provided by your programming language to catch and handle exceptions thrown by Redis client libraries.
3. Implement appropriate logging and monitoring mechanisms to track errors and failures in your Redis Streams application. This can help in identifying and resolving issues quickly.
Best Practices: Performance Optimization
To ensure optimal performance when working with Redis Streams, consider the following best practices:
1. Use pipelining or batch commands to reduce the number of round-trips between the client and the Redis server. This can significantly improve performance, especially when dealing with a large number of commands.
2. Leverage Redis clustering or replication to distribute the workload and improve scalability. This allows you to scale horizontally by adding more Redis instances.
3. Monitor the memory usage of your Redis server and use eviction policies or other memory management techniques to prevent memory exhaustion. This is particularly important when dealing with large streams or high-velocity data.
Related Article: Tutorial: Installing Redis on Ubuntu
Real World Example: Building a Real-Time Dashboard
A real-time dashboard is a common use case for Redis Streams. It allows you to display live data and metrics in a visually appealing manner. Here’s an example of how Redis Streams can be used to build a real-time dashboard:
import redis r = redis.Redis() # Subscribe to the stream and update the dashboard pubsub = r.pubsub() pubsub.subscribe('metrics') for message in pubsub.listen(): if message['type'] == 'message': data = message['data'] # Update the dashboard with the new data print(f'Received data: {data}')
In this example, we create a subscription to the “metrics” stream and listen for new messages. Whenever a new message is received, we update the dashboard with the new data. This allows the dashboard to display real-time information as it arrives.
Real World Example: Implementing a Message Queue
Redis Streams can be used as a high-performance message queue for handling asynchronous communication between different components of an application. Here’s an example of how Redis Streams can be used to implement a message queue:
import redis r = redis.Redis() # Enqueue a message r.xadd('queue', {'message': 'Hello, World!'}) # Dequeue a message result = r.xread({'queue': '0'}, count=1) message = result[0][1][0] print(f'Dequeued message: {message["message"]}')
In this example, we enqueue a new message to the “queue” stream using the XADD command. We then dequeue the message from the stream using the XREAD command. This allows different components of the application to communicate asynchronously through the message queue.
Real World Example: Event-Driven Architecture
Event-driven architecture is a popular architectural style where components communicate with each other by emitting and consuming events. Redis Streams can be used as a reliable and scalable event store for implementing an event-driven architecture. Here’s an example:
import redis r = redis.Redis() # Emit an event r.xadd('events', {'event_type': 'order_created', 'order_id': '123'}) # Consume events result = r.xread({'events': '0'}, count=1) event = result[0][1][0] print(f'Event type: {event["event_type"]}, Order ID: {event["order_id"]}')
In this example, we emit an event to the “events” stream using the XADD command. We then consume the events from the stream using the XREAD command. This allows different components of the application to react to events and perform the necessary actions.
Related Article: Tutorial: Comparing Kafka vs Redis
Performance Considerations: Memory Management
When working with Redis Streams, it is important to consider memory management to ensure optimal performance. Here are some considerations:
1. Use the XTRIM command to trim streams and remove old entries that are no longer needed. This can help in reducing memory usage, especially when dealing with large streams.
2. Monitor the memory usage of your Redis server using the INFO command or other monitoring tools. If the memory usage is high, consider optimizing your data structures or scaling your Redis infrastructure.
3. Configure appropriate eviction policies in Redis to automatically remove old or less frequently accessed entries from memory. This can help in maintaining an optimal memory footprint.
Performance Considerations: Scaling
To scale Redis Streams and handle increased workload, consider the following performance considerations:
1. Use Redis clustering or replication to distribute the workload across multiple Redis instances. This allows you to scale horizontally and handle a larger number of streams and consumers.
2. Implement sharding or partitioning strategies to distribute the streams and consumers across different Redis instances. This can help in achieving better load balancing and performance.
3. Use connection pooling or connection multiplexing techniques to efficiently manage connections to Redis. This can reduce the overhead of establishing new connections for each stream or consumer.
Performance Considerations: Latency
Redis Streams is designed for low-latency data processing and can handle high-velocity data streams. However, there are a few considerations to minimize latency:
1. Minimize network round-trips by using pipelining or batch commands. This can reduce the overhead of multiple network requests and improve overall latency.
2. Optimize your data processing algorithms to minimize computational overhead. Use efficient data structures and algorithms to process the data in real-time.
3. Ensure that your Redis infrastructure is properly configured and optimized for low-latency operations. This includes network settings, Redis server configuration, and hardware resources.
Related Article: Exploring Alternatives to Redis
Advanced Technique: Stream Aggregation
Stream aggregation is a useful technique that allows you to aggregate data from multiple streams into a single stream. This can be useful for generating summarized or aggregated views of the data. Here’s an example of how stream aggregation can be achieved:
XREADGROUP GROUP mygroup consumer1 COUNT 10 STREAMS stream1 stream2
In this example, we read entries from two streams, “stream1” and “stream2”, and aggregate them into a single stream. The result is returned as an array of arrays, where each sub-array represents a stream and its entries.
Advanced Technique: Stream Joins
Stream joins enable you to combine data from different streams based on a common attribute. This can be useful for performing complex data processing tasks. Here’s an example of how stream joins can be achieved:
XREADGROUP GROUP mygroup consumer1 COUNT 10 STREAMS stream1 stream2
In this example, we read entries from two streams, “stream1” and “stream2”, and join them based on a common attribute. The result is returned as an array of arrays, where each sub-array represents a stream and its entries.
Advanced Technique: Stream Processing
Stream processing is a useful technique that allows you to process streams in real-time and derive valuable insights. Redis Streams provides the foundation for building stream processing applications. Here’s an example of how stream processing can be achieved:
import redis r = redis.Redis() # Process stream entries in real-time result = r.xread({'stream1': '0', 'stream2': '0'}, block=0) for stream, entries in result: for entry in entries: # Process the entry print(f'Processing entry: {entry}')
In this example, we read entries from multiple streams and process them in real-time. The result is returned as an array of arrays, where each sub-array represents a stream and its entries. The entries are then processed individually.
Related Article: Tutorial on Implementing Redis Sharding
Code Snippet: Publishing to a Stream
To publish data to a stream, you can use the XADD command. Here’s an example of publishing a new entry to a stream:
import redis r = redis.Redis() r.xadd('mystream', {'field1': 'value1', 'field2': 'value2'})
In this example, we use the XADD command to publish a new entry to the “mystream” stream. The entry contains two fields, “field1” and “field2”, with their corresponding values.
Code Snippet: Consuming from a Stream
To consume data from a stream, you can use the XREAD command. Here’s an example of consuming entries from a stream:
import redis r = redis.Redis() result = r.xread({'mystream': '0'}, count=10) for stream, entries in result: for entry in entries: # Process the entry print(f'Processing entry: {entry}')
In this example, we use the XREAD command to consume entries from the “mystream” stream starting from the ID “0”. The result is returned as an array of arrays, where each sub-array represents a stream and its entries. The entries are then processed individually.
Code Snippet: Stream Group Creation
To create a consumer group for a stream, you can use the XGROUP CREATE command. Here’s an example of creating a consumer group for a stream:
XGROUP CREATE mystream mygroup 0
In this example, we use the XGROUP CREATE command to create a consumer group called “mygroup” for the “mystream” stream. The “0” specifies the initial ID to start consuming from.
Related Article: Tutorial: Redis vs RabbitMQ Comparison
Error Handling: Handling Stream Errors
When working with Redis Streams, it is important to handle errors that can occur during stream operations. Here’s an example of how to handle stream errors:
import redis r = redis.Redis() try: result = r.xread({'mystream': '0'}, count=10) except redis.exceptions.RedisError as e: # Handle the stream error print(f'Stream error: {e}')
In this example, we use a try-catch block to catch any exceptions thrown by the XREAD command. If a RedisError occurs, it is caught and the error is handled accordingly.
Error Handling: Error Logging and Monitoring
To effectively handle errors in Redis Streams, it is important to implement error logging and monitoring mechanisms. Here’s an example of how to log and monitor stream errors:
import redis import logging r = redis.Redis() logger = logging.getLogger(__name__) try: result = r.xread({'mystream': '0'}, count=10) except redis.exceptions.RedisError as e: # Log the stream error logger.error(f'Stream error: {e}')
In this example, we use the logging module to log the stream error. The error message is logged using the appropriate logging level, such as ERROR or WARNING. This allows you to track and monitor stream errors effectively.