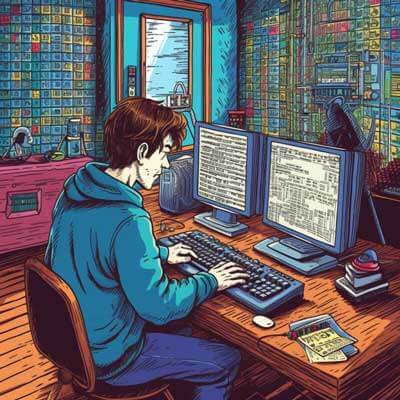
Table of Contents
Introduction to Redis Lua Scripting
Redis Lua Scripting is a powerful feature that allows you to extend the functionality of Redis by executing Lua scripts directly on the server. Lua is a lightweight scripting language that is easy to learn and has a simple syntax, making it an ideal choice for scripting in Redis.
Redis Lua Scripting provides several advantages over traditional approaches such as using Redis commands directly or writing client-side scripts. By executing scripts on the server, you can reduce network overhead and improve performance. Additionally, Lua scripts can be executed atomically, ensuring consistency and eliminating the need for multiple round trips to the server.
In this chapter, we will explore the basics of Redis Lua Scripting and learn how to get started with writing and executing Lua scripts in Redis.
Related Article: How to Use Redis Queue in Redis
Getting Started with Redis Lua Scripting
To begin using Redis Lua Scripting, you need to ensure that you have a Redis server installed and running on your machine. You can download and install Redis from the official website, or use a package manager for your operating system.
Once Redis is installed, you can launch the Redis CLI by running the redis-cli
command in your terminal. The Redis CLI provides an interactive command-line interface to interact with the Redis server.
To execute a Lua script in Redis, you can use the EVAL
command followed by the Lua script itself. The EVAL
command takes the Lua script as its first argument, followed by any additional arguments required by the script.
Here's an example of a simple Lua script that increments a value stored in a Redis key:
local key = KEYS[1] local value = tonumber(redis.call('GET', key)) value = value + 1 redis.call('SET', key, value) return value
In this script, we first retrieve the value stored in the specified Redis key using the GET
command. We then increment the value by 1 and update it in Redis using the SET
command. Finally, we return the updated value.
To execute this Lua script in Redis, you can use the following EVAL
command:
EVAL "local key = KEYS[1]\nlocal value = tonumber(redis.call('GET', key))\nvalue = value + 1\nredis.call('SET', key, value)\nreturn value" 1 mykey
In this command, we pass the Lua script as a string to the EVAL
command, followed by the number of keys required by the script (in this case, 1) and the actual key(s) required by the script (in this case, mykey
).
Executing this command will increment the value stored in the mykey
Redis key by 1 and return the updated value.
Basic Lua Scripting in Redis
Lua scripting in Redis allows you to perform a wide range of operations, from simple data manipulation to complex computations. In this section, we will cover some basic Lua scripting techniques that you can use in Redis.
Variables and Data Types
In Lua, you can declare variables using the local
keyword. Lua has dynamic typing, which means you don't need to specify the data type of a variable explicitly. Lua automatically determines the data type based on the value assigned to the variable.
Here's an example that demonstrates declaring variables and using different data types in Lua:
local name = "John Doe" local age = 30 local isMember = true local grades = {90, 85, 95, 80}
In this example, we declare four variables: name
, age
, isMember
, and grades
. The name
variable is assigned a string value, the age
variable is assigned an integer value, the isMember
variable is assigned a boolean value, and the grades
variable is assigned a table (or an array) of numbers.
Control Structures
Lua provides various control structures that allow you to perform conditional and iterative operations in your scripts. These control structures include if-else
statements, for
loops, while
loops, and more.
Here's an example that demonstrates the usage of if-else
and for
statements in Lua:
local score = 85 if score >= 90 then print("Excellent!") elseif score >= 80 then print("Good!") else print("Needs improvement.") end for i = 1, 5 do print("Count: " .. i) end
In this example, we first check the value of the score
variable using an if-else
statement and print a corresponding message based on the score. Then, we use a for
loop to print the numbers from 1 to 5.
Using Redis Commands in Lua Scripts
One of the key benefits of Redis Lua Scripting is the ability to use Redis commands directly within your Lua scripts. This allows you to leverage the full power of Redis while executing custom logic in your scripts.
To execute a Redis command within a Lua script, you can use the redis.call
function followed by the command and its arguments. The redis.call
function returns the result of the Redis command.
Here's an example that demonstrates using Redis commands in a Lua script:
local key = KEYS[1] local value = ARGV[1] redis.call('SET', key, value) local result = redis.call('GET', key) return result
In this script, we first retrieve the key and value passed as arguments to the script. We then use the SET
command to set the value of the specified key in Redis. Finally, we use the GET
command to retrieve the value of the key and return it as the result of the script.
To execute this Lua script in Redis, you can use the EVAL
command as shown earlier, passing the script and the required key(s) and value(s) as arguments.
Related Article: Tutorial: Setting Up Redis Using Docker Compose
Working with Redis Data Structures
Redis provides a variety of data structures such as strings, lists, sets, hashes, and sorted sets. Lua scripting in Redis allows you to manipulate these data structures efficiently within your scripts.
Strings
Strings are the simplest data type in Redis and can hold any binary data, such as a serialized object, a JSON string, or plain text. Lua provides built-in functions for string manipulation, making it easy to work with Redis strings in Lua scripts.
Here's an example that demonstrates working with Redis strings in a Lua script:
local key = KEYS[1] local value = ARGV[1] redis.call('SET', key, value) local result = redis.call('GET', key) return result
In this script, we first retrieve the key and value passed as arguments to the script. We then use the SET
command to set the value of the specified key in Redis. Finally, we use the GET
command to retrieve the value of the key and return it as the result of the script.
To execute this Lua script in Redis, you can use the EVAL
command as shown earlier, passing the script and the required key(s) and value(s) as arguments.
Lists
Lists in Redis are ordered collections of strings. Lua scripting in Redis allows you to perform various operations on lists, such as adding elements, removing elements, and retrieving elements based on their position.
Here's an example that demonstrates working with Redis lists in a Lua script:
local key = KEYS[1] local values = ARGV for _, value in ipairs(values) do redis.call('LPUSH', key, value) end local result = redis.call('LRANGE', key, 0, -1) return result
In this script, we first retrieve the key and a list of values passed as arguments to the script. We then use a for
loop to iterate over the values and use the LPUSH
command to push each value to the front of the specified list in Redis. Finally, we use the LRANGE
command to retrieve all elements of the list and return them as the result of the script.
To execute this Lua script in Redis, you can use the EVAL
command as shown earlier, passing the script and the required key(s) and value(s) as arguments.
Interacting with Redis Keyspaces in Lua Scripts
Redis uses a key-value data model, where each key is associated with a value. Lua scripting in Redis allows you to interact with Redis keyspaces, perform operations on keys, and manipulate the associated values within your scripts.
Key Operations
Lua scripting in Redis provides several key-related operations, such as checking the existence of a key, deleting a key, and iterating over keys. These operations are useful when you need to perform specific actions based on the presence or absence of keys in Redis.
Here's an example that demonstrates key operations in a Lua script:
local keys = redis.call('KEYS', 'user:*') for _, key in ipairs(keys) do redis.call('DEL', key) end local count = #keys return count
In this script, we use the KEYS
command to retrieve all keys matching a specific pattern (user:*
). We then use a for
loop to iterate over the keys and use the DEL
command to delete each key from Redis. Finally, we return the count of deleted keys.
To execute this Lua script in Redis, you can use the EVAL
command as shown earlier, passing the script and any required arguments.
Value Manipulation
Lua scripting in Redis allows you to manipulate the values associated with keys, such as updating values, incrementing or decrementing numerical values, and performing operations on complex data structures.
Here's an example that demonstrates value manipulation in a Lua script:
local key = KEYS[1] local field = ARGV[1] local increment = tonumber(ARGV[2]) redis.call('HINCRBY', key, field, increment) local result = redis.call('HGET', key, field) return result
In this script, we first retrieve the key, field, and increment passed as arguments to the script. We then use the HINCRBY
command to increment the value of the specified field in the hash associated with the key. Finally, we use the HGET
command to retrieve the updated value of the field and return it as the result of the script.
To execute this Lua script in Redis, you can use the EVAL
command as shown earlier, passing the script and the required key(s), field, and increment as arguments.
Manipulating Redis Streams
Redis Streams is a powerful data structure that allows you to store and consume streams of messages. Lua scripting in Redis allows you to manipulate Redis Streams efficiently within your scripts.
Stream Operations
Lua scripting in Redis provides several operations for working with Redis Streams, such as adding messages to a stream, reading messages from a stream, and acknowledging the processing of messages.
Here's an example that demonstrates stream operations in a Lua script:
local stream = KEYS[1] local message = ARGV[1] redis.call('XADD', stream, '*', 'message', message) local result = redis.call('XREAD', 'STREAMS', stream, '0') return result
In this script, we first retrieve the stream and message passed as arguments to the script. We then use the XADD
command to add the message to the specified stream with a generated ID. Finally, we use the XREAD
command to read all messages from the stream starting from ID 0 and return the result.
To execute this Lua script in Redis, you can use the EVAL
command as shown earlier, passing the script and the required stream and message as arguments.
Lua Scripting for Pub/Sub Messaging in Redis
Redis provides a Pub/Sub messaging system that allows you to publish and subscribe to channels. Lua scripting in Redis enables you to perform custom logic while handling Pub/Sub messages.
Subscribing to Channels
Lua scripting in Redis allows you to subscribe to channels and receive messages in real-time. This enables you to process messages and perform custom actions based on the content of the messages.
Here's an example that demonstrates subscribing to channels in a Lua script:
local channels = ARGV redis.call('SUBSCRIBE', unpack(channels))
In this script, we use the SUBSCRIBE
command to subscribe to multiple channels passed as arguments to the script. The unpack
function is used to unpack the channels array and pass them as individual arguments to the SUBSCRIBE
command.
To execute this Lua script in Redis, you can use the EVAL
command as shown earlier, passing the script and the required channels as arguments.
Publishing Messages
Lua scripting in Redis also allows you to publish messages to channels. This enables you to send messages and trigger custom logic based on the published messages.
Here's an example that demonstrates publishing messages in a Lua script:
local channel = KEYS[1] local message = ARGV[1] redis.call('PUBLISH', channel, message)
In this script, we first retrieve the channel and message passed as arguments to the script. We then use the PUBLISH
command to publish the message to the specified channel.
To execute this Lua script in Redis, you can use the EVAL
command as shown earlier, passing the script and the required channel and message as arguments.
Related Article: Tutorial: Kafka vs Redis
Lua Scripting for Redis Cluster Operations
Redis Cluster is a distributed implementation of Redis that provides high availability and automatic partitioning of data across multiple nodes. Lua scripting in Redis allows you to perform cluster-related operations and execute custom logic in a distributed environment.
Cluster Operations
Lua scripting in Redis provides several operations for working with Redis Cluster, such as retrieving information about cluster nodes, performing cluster-wide operations, and handling cluster events.
Here's an example that demonstrates cluster operations in a Lua script:
local nodes = redis.call('CLUSTER', 'NODES') local result = {} for node in string.gmatch(nodes, "[^\n]+") do table.insert(result, node) end return result
In this script, we use the CLUSTER NODES
command to retrieve information about all cluster nodes. We then iterate over the nodes using a for
loop and insert each node into a table. Finally, we return the table containing the cluster nodes.
To execute this Lua script in Redis, you can use the EVAL
command as shown earlier, passing the script as an argument.
Optimizing Performance in Redis Lua Scripts
Optimizing the performance of Redis Lua scripts is crucial to ensure efficient execution and minimize the impact on Redis server resources. In this chapter, we will explore various techniques and best practices to optimize the performance of Redis Lua scripts.
Reducing Round Trips
Reducing the number of round trips between the client and the Redis server is essential for improving the performance of Lua scripts. Each round trip introduces network latency and can significantly impact the overall execution time of the script.
To reduce round trips, you can batch multiple Redis commands within a single Lua script. By executing multiple commands in a single round trip, you can minimize the network overhead and improve performance.
Here's an example that demonstrates reducing round trips in a Lua script:
local key1 = KEYS[1] local key2 = KEYS[2] local value1 = ARGV[1] local value2 = ARGV[2] redis.call('SET', key1, value1) redis.call('SET', key2, value2)
In this script, we execute two SET
commands to set the values of two different keys. By combining these two commands within a single Lua script, we can reduce the round trips to the Redis server.
To execute this Lua script in Redis, you can use the EVAL
command as shown earlier, passing the script and the required key(s) and value(s) as arguments.
Minimizing Data Transfers
Minimizing the amount of data transferred between the client and the Redis server can significantly improve the performance of Lua scripts. Large data transfers introduce additional network latency and can negatively impact the script's execution time.
To minimize data transfers, you should only retrieve and transfer the necessary data between the client and the Redis server. Avoid transferring large data structures or unnecessary fields within a Lua script.
Here's an example that demonstrates minimizing data transfers in a Lua script:
local key = KEYS[1] local value = redis.call('GET', key) local field1 = redis.call('HGET', key, 'field1') local field2 = redis.call('HGET', key, 'field2') return {value, field1, field2}
In this script, we retrieve the value of a key and two specific fields from a hash associated with the key. By only retrieving the required data, we minimize the data transfer between the client and the Redis server.
To execute this Lua script in Redis, you can use the EVAL
command as shown earlier, passing the script and the required key as an argument.
Error Handling
Proper error handling is essential in Redis Lua scripting to ensure the reliability and stability of your scripts. In this chapter, we will explore error handling techniques and best practices that you can use in Redis Lua scripts.
Error Propagation
In Redis Lua scripting, errors can occur during script execution due to various reasons, such as invalid arguments, Redis command failures, or runtime errors in the Lua script itself. When an error occurs, it is important to propagate the error to the calling code and handle it appropriately.
To propagate an error in a Lua script, you can use the error
function provided by Lua. The error
function throws an error with the specified message and stops the execution of the script.
Here's an example that demonstrates error propagation in a Lua script:
local key = KEYS[1] local value = redis.call('GET', key) if not value then error('Key not found') end return value
In this script, we use the GET
command to retrieve the value of a key. If the key is not found (i.e., the value is nil
), we throw an error with the message 'Key not found'. This error will be propagated to the calling code, allowing you to handle it appropriately.
To execute this Lua script in Redis, you can use the EVAL
command as shown earlier, passing the script and the required key as an argument.
Error Handling Patterns
In addition to propagating errors, you can use various error handling patterns to gracefully handle errors within your Lua scripts. These patterns include using pcall
to catch and handle errors, using assert
to validate conditions, and using xpcall
to handle errors with custom error handlers.
Here's an example that demonstrates error handling patterns in a Lua script:
local key = KEYS[1] local success, value = pcall(redis.call, 'GET', key) if not success then -- Handle the error return nil end assert(value ~= nil, 'Key not found') return value
In this script, we use the pcall
function to wrap the GET
command and catch any errors that occur during its execution. If an error occurs, we handle it appropriately and return nil
. We also use the assert
function to validate that the value is not nil
and throw an error if it is. This pattern helps ensure the script's correctness and handle errors gracefully.
To execute this Lua script in Redis, you can use the EVAL
command as shown earlier, passing the script and the required key as an argument.
Use Cases for Redis Lua Scripting
Redis Lua Scripting can be used in a wide range of use cases to enhance the functionality and performance of Redis. In this chapter, we will explore some common use cases where Lua scripting can be beneficial.
Atomic Operations
Redis Lua Scripting allows you to perform atomic operations, ensuring that multiple Redis commands are executed as a single atomic operation. This is particularly useful when you need to update multiple keys or perform complex operations that require consistency.
Here's an example use case for atomic operations in Redis Lua scripting:
Suppose you have a Redis-based counter that needs to be incremented by 1 and a corresponding log that needs to be updated with the new value. Using Lua scripting, you can perform these two operations atomically, ensuring that both the counter and the log are updated consistently.
local counter = KEYS[1] local log = KEYS[2] local value = redis.call('INCR', counter) redis.call('LPUSH', log, value) return value
In this script, we first increment the value of the counter using the INCR
command. Then, we push the new value to the log using the LPUSH
command. By executing these two commands within a single Lua script, we ensure that both operations are performed atomically.
To execute this Lua script in Redis, you can use the EVAL
command as shown earlier, passing the script and the required keys as arguments.
Data Processing and Transformation
Redis Lua Scripting provides a flexible and efficient way to process and transform data stored in Redis. You can use Lua scripting to perform complex computations, manipulate data structures, and transform data into a different format.
Here's an example use case for data processing and transformation in Redis Lua scripting:
Suppose you have a set of user profiles stored in Redis hashes, and you need to calculate the average age of all users. Using Lua scripting, you can iterate over the user profiles, retrieve the age field, and calculate the average age.
local profiles = redis.call('KEYS', 'user:*') local totalAge = 0 local count = 0 for _, profile in ipairs(profiles) do local age = tonumber(redis.call('HGET', profile, 'age')) if age then totalAge = totalAge + age count = count + 1 end end local averageAge = totalAge / count return averageAge
In this script, we first retrieve all user profiles using the KEYS
command. We then iterate over the profiles using a for
loop and retrieve the age field from each profile using the HGET
command. If the age field exists, we add the age to the totalAge
variable and increment the count
variable. Finally, we calculate the average age and return it as the result of the script.
To execute this Lua script in Redis, you can use the EVAL
command as shown earlier, passing the script as an argument.
Related Article: Tutorial: Installing Redis on Ubuntu
Best Practices for Redis Lua Scripting
Redis Lua Scripting provides a powerful and flexible environment for executing custom logic on the Redis server. To ensure the effectiveness and reliability of your Lua scripts, it is important to follow best practices and adhere to recommended guidelines.
Keep Scripts Simple and Readable
When writing Lua scripts for Redis, it is important to keep the scripts simple and readable. Avoid writing complex scripts with convoluted logic or excessive branching. Instead, focus on writing clear and concise scripts that are easy to understand and maintain.
Use Redis Commands Judiciously
While Redis Lua Scripting allows you to use Redis commands directly within your scripts, it is important to use them judiciously. Avoid using unnecessary or expensive Redis commands, and instead, try to leverage Lua's built-in functions and libraries whenever possible.
Validate Inputs and Handle Errors
Validating inputs and handling errors properly is crucial in Redis Lua Scripting. Always validate the inputs passed to the script and handle any potential errors gracefully. Use error propagation, error handling patterns, and appropriate error messages to ensure that your scripts handle errors correctly.
Optimize Performance and Efficiency
Optimizing the performance and efficiency of your Lua scripts is essential to minimize the impact on the Redis server and ensure efficient execution. Follow performance optimization techniques such as reducing round trips, minimizing data transfers, and leveraging Redis data structures effectively.
Real World Examples of Redis Lua Scripting
Redis Lua Scripting is widely used in real-world scenarios to enhance the functionality and performance of Redis. In this chapter, we will explore some real-world examples of Redis Lua Scripting and how it is used in practice.
Rate Limiting
Rate limiting is a common use case for Redis Lua Scripting. Lua scripts can be used to implement various rate limiting algorithms, such as the token bucket algorithm or the leaky bucket algorithm, to control the rate at which certain operations are allowed.
Here's an example of a Lua script for rate limiting in Redis:
local key = KEYS[1] local limit = tonumber(ARGV[1]) local interval = tonumber(ARGV[2]) local timestamp = tonumber(redis.call('TIME')[1]) local count = tonumber(redis.call('GET', key) or '0') local ttl = redis.call('PTTL', key) if count >= limit then return 0 end redis.call('INCR', key) redis.call('PEXPIRE', key, ttl or interval) return 1
In this script, we first retrieve the rate limit parameters passed as arguments to the script: key
, limit
, and interval
. We then retrieve the current count and time-to-live (TTL) of the key. If the count exceeds the limit, we return 0 to indicate that the operation is not allowed. Otherwise, we increment the count, set the TTL if it doesn't exist, and return 1 to indicate that the operation is allowed.
To execute this Lua script in Redis, you can use the EVAL
command as shown earlier, passing the script and the required key, limit, and interval as arguments.
Distributed Locking
Distributed locking is another common use case for Redis Lua Scripting. Lua scripts can be used to implement distributed locking mechanisms to ensure that only one process or thread can access a shared resource at a time.
Here's an example of a Lua script for distributed locking in Redis:
local key = KEYS[1] local timeout = tonumber(ARGV[1]) local identifier = ARGV[2] if redis.call('SET', key, identifier, 'NX', 'PX', timeout) then return 1 else return 0 end
In this script, we first retrieve the lock parameters passed as arguments to the script: key
, timeout
, and identifier
. We then use the SET
command with the NX
(set if not exists) and PX
(expire time in milliseconds) options to set the lock with the specified timeout. If the lock is acquired successfully, we return 1. Otherwise, we return 0.
To execute this Lua script in Redis, you can use the EVAL
command as shown earlier, passing the script and the required key, timeout, and identifier as arguments.
Advanced Techniques in Redis Lua Scripting
Redis Lua Scripting provides advanced techniques that allow you to perform complex operations and leverage the full power of Redis within your scripts. In this chapter, we will explore some advanced techniques that you can use in Redis Lua scripting.
Script Caching
Redis Lua Scripting provides a built-in script caching mechanism to improve performance and reduce network overhead. Instead of sending the script code with each EVAL
command, you can use the SCRIPT LOAD
command to load the script on the server and retrieve a SHA-1 digest that represents the script.
Here's an example that demonstrates script caching in Redis Lua scripting:
local script = [[ local key = KEYS[1] local value = ARGV[1] redis.call('SET', key, value) local result = redis.call('GET', key) return result ]] local sha1 = redis.call('SCRIPT', 'LOAD', script)
In this script, we define the Lua script as a string and use the SCRIPT LOAD
command to load the script on the server and retrieve the SHA-1 digest. The SCRIPT LOAD
command returns the digest, which can be used in subsequent EVALSHA
commands to execute the script.
To execute this Lua script in Redis, you can use the EVALSHA
command instead of EVAL
, passing the SHA-1 digest and any required arguments.
Using Redis Modules
Redis Lua Scripting can be combined with Redis Modules to extend the functionality of Redis even further. Redis Modules allow you to add new commands, data types, and functionalities to Redis, and Lua scripts can interact with these modules seamlessly.
To use a Redis Module in a Lua script, you need to ensure that the module is loaded on the Redis server. Once the module is loaded, you can use the module's commands within your Lua scripts as you would use any other Redis command.
Here's an example that demonstrates using a Redis Module in a Lua script:
local key = KEYS[1] local value = ARGV[1] redis.call('MODULE_COMMAND', key, value)
In this script, we use the MODULE_COMMAND
provided by a Redis Module, passing the key and value as arguments. This command can be any command provided by the Redis Module you are using.
To execute this Lua script in Redis, you can use the EVAL
command as shown earlier, passing the script and the required key and value as arguments.
Code Snippet Ideas for Redis Lua Scripting
Redis Lua Scripting allows you to write custom logic and perform operations directly on the Redis server. Here are some code snippet ideas to get you started with Redis Lua Scripting:
1. Atomic increment and decrement of a counter:
local key = KEYS[1] local increment = tonumber(ARGV[1]) return redis.call('INCRBY', key, increment)
2. Retrieving the values of multiple keys:
local keys = KEYS return redis.call('MGET', unpack(keys))
3. Sorting a list of values:
local key = KEYS[1] local values = redis.call('LRANGE', key, 0, -1) table.sort(values) return values
4. Performing set operations on multiple sets:
local keys = KEYS local operation = ARGV[1] local result = redis.call(operation, unpack(keys)) return result
5. Calculating the median of a list of values:
local key = KEYS[1] local values = redis.call('SORT', key, 'BY', 'nosort', 'GET', '#') local n = #values if n % 2 == 0 then return (values[n/2] + values[n/2+1]) / 2 else return values[(n+1)/2] end
These code snippets demonstrate only a fraction of what Redis Lua Scripting can do. Feel free to experiment and explore the full potential of Redis Lua Scripting to meet your unique needs.