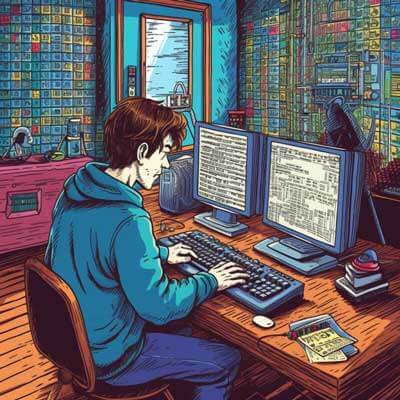
Table of Contents
Introduction
Redis is a popular choice for in-memory data storage due to its speed, simplicity, and versatility. However, there are situations where alternative solutions may be more suitable for specific use cases. In this technical guide, we will explore various alternatives to Redis and examine their strengths and weaknesses. By understanding these alternatives, software engineers can make informed decisions when choosing the right in-memory data storage solution for their applications.
Related Article: How to Use Redis with Django Applications
Use Cases of In-memory Data Storage
In-memory data storage is particularly useful in scenarios where fast access to frequently accessed data is critical. Here are two common use cases where in-memory data storage shines:
Data Caching
Caching is a technique that involves storing frequently accessed data in a cache to improve application performance. In-memory data storage solutions like Redis and Memcached are often used for this purpose. Let's take a look at an example of caching user data using Redis:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) def get_user_details(user_id): # Check if the user details exist in the cache user_details = r.get(f'user:{user_id}') if user_details is None: # Fetch the user details from the database user_details = fetch_user_details_from_database(user_id) # Store the user details in the cache r.set(f'user:{user_id}', user_details) return user_details
Real-time Analytics
In applications where real-time data analysis is required, in-memory data storage can be used to store and process large volumes of data with low latency. For example, consider a real-time analytics dashboard that displays the number of active users on a website. Here's how you can use Redis to track and update the active user count:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) def track_user_activity(user_id): # Increment the active user count r.incr('active_users') # Add the user to the set of active users r.sadd('users:active', user_id) def get_active_user_count(): # Retrieve the active user count return int(r.get('active_users')) def get_active_users(): # Retrieve the set of active users return r.smembers('users:active')
Related Article: How to Use Redis Streams
Best Practices for Data Caching
When implementing data caching with Redis or alternative in-memory data storage solutions, it's important to follow best practices to ensure optimal performance. Here are some key considerations:
Cache Invalidation
Caching introduces the challenge of cache invalidation, where cached data needs to be updated or invalidated when the underlying data changes. One common approach is to use a time-to-live (TTL) value for cache entries. Let's see an example of caching user data with a TTL of 5 minutes:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) def get_user_details(user_id): # Check if the user details exist in the cache user_details = r.get(f'user:{user_id}') if user_details is None: # Fetch the user details from the database user_details = fetch_user_details_from_database(user_id) # Store the user details in the cache with a TTL of 5 minutes r.setex(f'user:{user_id}', user_details, 300) return user_details
Cache Eviction Policies
When the cache reaches its maximum capacity, eviction policies determine which entries to remove from the cache to make room for new entries. Redis offers various eviction policies, such as LRU (Least Recently Used) and LFU (Least Frequently Used). Here's an example of setting the LRU eviction policy in Redis:
$ redis-cli config set maxmemory-policy allkeys-lru
Real World Examples of Distributed Caching
Distributed caching involves using multiple cache nodes to store data across a network, enabling high availability and scalability. Here are two real-world examples of distributed caching:
Related Article: Tutorial on Installing and Using redis-cli with Redis
Amazon ElastiCache
Amazon ElastiCache is a fully managed, in-memory data store service provided by AWS. It supports popular caching engines like Redis and Memcached and offers automatic scaling, backup, and failover capabilities. Here's an example of using Amazon ElastiCache with Redis in a Python application:
import redis # Connect to Amazon ElastiCache r = redis.Redis(host='my-elasticache-endpoint', port=6379, db=0) # Perform caching operations with Redis
Redis Cluster
Redis Cluster is a distributed implementation of Redis that allows data to be sharded across multiple Redis nodes. It provides automatic partitioning, replication, and failover, making it suitable for high-availability caching scenarios. Here's an example of connecting to a Redis Cluster using the Redis-py library:
from rediscluster import RedisCluster # Define Redis Cluster nodes startup_nodes = [ {"host": "node1", "port": "6379"}, {"host": "node2", "port": "6379"}, {"host": "node3", "port": "6379"}, ] # Connect to Redis Cluster r = RedisCluster(startup_nodes=startup_nodes) # Perform caching operations with Redis Cluster
Performance Considerations for High Traffic Applications
When dealing with high traffic applications, performance is crucial. Here are some considerations to optimize performance when using in-memory data storage solutions like Redis:
Data Partitioning
Partitioning data across multiple Redis instances can help distribute the load and improve performance. Redis supports various partitioning techniques, such as consistent hashing and range partitioning. Let's see an example of consistent hashing for data partitioning:
from rediscluster import RedisCluster # Define Redis Cluster nodes startup_nodes = [ {"host": "node1", "port": "6379"}, {"host": "node2", "port": "6379"}, {"host": "node3", "port": "6379"}, ] # Connect to Redis Cluster r = RedisCluster(startup_nodes=startup_nodes) # Perform data partitioning using consistent hashing for i in range(100): key = f'data:{i}' node = r.get_node(key) node.set(key, f'value:{i}')
Related Article: Tutorial on Configuring a Redis Cluster
Pipeline and Batch Operations
Reducing network round trips by batching multiple operations or using pipelines can greatly improve performance. Here's an example of executing multiple Redis commands in a pipeline:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) # Use a pipeline for batch operations pipeline = r.pipeline() # Queue multiple commands in the pipeline pipeline.set('key1', 'value1') pipeline.set('key2', 'value2') pipeline.set('key3', 'value3') # Execute the commands in a single round trip pipeline.execute()
Advanced Techniques for Data Manipulation
In addition to basic data storage and retrieval operations, in-memory data storage solutions like Redis offer advanced techniques for data manipulation. Here are two examples:
Publish/Subscribe Pattern
Redis supports the publish/subscribe pattern, where clients can subscribe to channels and receive messages published to those channels. This pattern is useful for building real-time messaging systems or implementing event-driven architectures. Let's see an example of publishing and subscribing to a channel in Redis:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) # Publish a message to a channel r.publish('my_channel', 'Hello, subscribers!') # Subscribe to a channel and process incoming messages pubsub = r.pubsub() pubsub.subscribe('my_channel') for message in pubsub.listen(): if message['type'] == 'message': print(f"Received message: {message['data']}")
Sorted Sets
Redis provides sorted sets, which are similar to sets but with an associated score for each element. Sorted sets are useful for scenarios where elements need to be sorted based on a specific criteria. Here's an example of using sorted sets in Redis:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) # Add elements to a sorted set r.zadd('leaderboard', {'Alice': 100, 'Bob': 200, 'Charlie': 150}) # Retrieve the top scorers from the leaderboard top_scorers = r.zrevrange('leaderboard', 0, 2, withscores=True) for player, score in top_scorers: print(f"{player}: {score}")
Related Article: How to Use Redis Queue in Redis
Code Snippet Ideas for Redis Integration
When integrating Redis or alternative in-memory data storage solutions into your applications, code snippets can help you get started quickly. Here are two code snippet ideas for Redis integration:
Rate Limiting
Redis can be used for implementing rate limiting, where you restrict the number of requests a user or IP address can make within a specified time period. Here's an example of using Redis to implement a simple rate limiter:
import redis import time # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) def is_request_allowed(user_id, max_requests, time_period): current_time = int(time.time()) # Get the number of requests made within the time period request_count = r.get(f'requests:{user_id}') if request_count is None: # First request, initialize the request count r.setex(f'requests:{user_id}', 1, time_period) return True elif int(request_count) < max_requests: # Increment the request count and update the expiration time r.incr(f'requests:{user_id}') r.expire(f'requests:{user_id}', current_time + time_period) return True else: # Request limit exceeded return False
Distributed Locking
Redis can be used for implementing distributed locking to ensure mutually exclusive access to a shared resource across multiple processes or machines. Here's an example of using Redis to acquire and release a distributed lock:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) def acquire_lock(lock_name, expiration_time): # Attempt to acquire the lock lock_acquired = r.set(lock_name, 'LOCKED', nx=True, ex=expiration_time) return lock_acquired is not None def release_lock(lock_name): # Release the lock by deleting the key r.delete(lock_name)
Error Handling and Fault Tolerance
When working with in-memory data storage solutions like Redis, it's important to handle errors and ensure fault tolerance. Here are some considerations:
Related Article: Tutorial on Redis Sentinel: A Deep Look
Connection Errors
Connections to Redis can fail due to network issues or other factors. It's important to handle connection errors gracefully and implement appropriate retry mechanisms. Here's an example of retrying a Redis operation in case of a connection error:
import redis import time # Retry settings max_retries = 3 retry_delay = 1 def perform_redis_operation(): retries = 0 while retries < max_retries: try: # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) # Perform the Redis operation # ... break except redis.ConnectionError: # Connection error, wait and retry time.sleep(retry_delay) retries += 1 else: # Max retries exceeded, handle the error print("Unable to perform Redis operation.")
Data Integrity
In-memory data storage solutions like Redis store data in memory, which is volatile. It's crucial to ensure data integrity by persisting important data to disk or implementing backup mechanisms. Redis provides options like RDB snapshots and AOF (Append-Only File) for data persistence. Here's an example of enabling AOF persistence in Redis:
$ redis-cli config set appendonly yes
This technical guide has explored various aspects of alternatives to Redis, including their use cases, best practices, real-world examples, performance considerations, advanced techniques, code snippet ideas, and error handling. By understanding and experimenting with these alternatives, software engineers can make informed decisions and choose the most suitable in-memory data storage solution for their specific requirements.