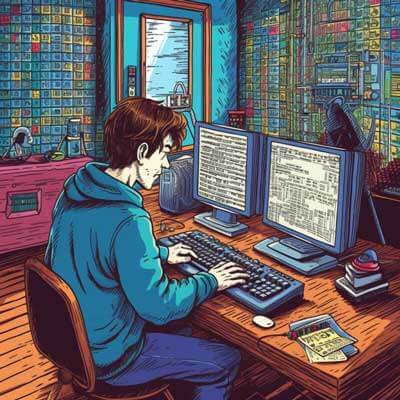
Table of Contents
Introduction
Redis Cluster is a distributed implementation of Redis that allows you to partition your data across multiple nodes for increased scalability and fault tolerance. In this tutorial, we will explore the various aspects of configuring a Redis Cluster, including its basics, designing and setting up a cluster on Linux, best practices, use cases, real-world examples, performance considerations, advanced techniques, error handling, and code snippet ideas.
Related Article: How to Use Redis Streams
Redis Cluster Basics
Redis Cluster operates using a master-slave model, with each master node responsible for a subset of the data and its corresponding slave nodes replicating that data. The cluster uses a distributed hash slot mechanism to distribute keys across the nodes, ensuring a balanced workload.
To interact with a Redis Cluster, you need to use the Redis Cluster API or a client library that provides support for Redis Cluster. Let's take a look at an example of using the Redis Cluster API in Python:
import redis# Connect to the Redis Clustercluster = redis.RedisCluster(host='localhost', port=7000)# Set a key-value paircluster.set('mykey', 'myvalue')# Get the value of a keyvalue = cluster.get('mykey')print(value)
Designing a Redis Cluster on Linux
Designing a Redis Cluster involves determining the number of master and slave nodes based on your requirements and the expected workload. You also need to consider factors like data sharding, fault tolerance, and network topology.
When designing a Redis Cluster on Linux, you need to ensure that each node has a unique IP address and port. Additionally, you should plan for sufficient memory and storage capacity to handle your data and traffic.
Let's take a look at a sample Redis Cluster configuration file:
# redis.confport 7000cluster-enabled yescluster-config-file nodes.confcluster-node-timeout 5000appendonly yes
Setting Up a Redis Cluster on Linux
To set up a Redis Cluster on Linux, you need to follow a series of steps:
1. Install Redis and its dependencies on each node.
2. Configure each Redis instance with a unique port and cluster-related settings.
3. Start the Redis instances on all nodes.
4. Create the Redis Cluster by joining the nodes together.
Here is an example of the command to start a Redis instance as a master node:
redis-server /path/to/redis.conf
And here is an example of the command to start a Redis instance as a slave node:
redis-server /path/to/redis.conf --slaveof <master-ip> <master-port>
Once the Redis instances are running, you can use the redis-cli
tool to create the Redis Cluster:
redis-cli --cluster create <node1-ip>:<node1-port> <node2-ip>:<node2-port> ... --cluster-replicas <num-replicas>
Related Article: Tutorial on Integrating Redis with Spring Boot
Best Practices for Redis Cluster
When working with Redis Cluster, it is important to follow certain best practices to ensure optimal performance and reliability. Here are some recommendations:
1. Monitor the cluster's health using tools like Redis Sentinel or Redis Cluster Manager.
2. Configure proper data replication by assigning an appropriate number of slave nodes for each master.
3. Use consistent hashing to distribute keys evenly across the cluster.
4. Enable persistence to protect against data loss.
5. Regularly backup your Redis Cluster data.
Use Cases for Redis Cluster
Redis Cluster is well-suited for various use cases, including:
1. High-speed caching: Redis Cluster provides fast in-memory caching capabilities, making it ideal for improving the performance of web applications.
2. Real-time analytics: With its ability to handle high throughput and low-latency operations, Redis Cluster is often used for real-time analytics and data processing.
3. Session storage: Storing and managing user sessions in Redis Cluster allows for easy scalability and seamless session management across multiple nodes.
Real World Examples of Redis Cluster
Let's explore a couple of real-world examples where Redis Cluster is used:
1. E-commerce platform: An e-commerce platform can use Redis Cluster for product catalog caching, session management, and real-time inventory updates.
2. Social media platform: A social media platform can leverage Redis Cluster for caching user profiles, managing real-time notifications, and tracking user activity.
Performance Considerations for Redis Cluster
To ensure optimal performance in a Redis Cluster environment, consider the following factors:
1. Network latency: Minimize network latency by deploying Redis Cluster on nodes within the same data center or region.
2. Key distribution: Distribute keys evenly across the cluster to avoid hotspots and ensure a balanced workload.
3. Memory management: Monitor the memory usage of each node to prevent excessive memory consumption and potential performance degradation.
Related Article: Analyzing Redis Query Rate per Second with Sidekiq
Advanced Techniques in Redis Cluster
Redis Cluster supports several advanced techniques that can enhance its functionality:
1. Cross-slot commands: Execute commands that span multiple hash slots using the EVAL
command with Lua scripting.
2. Cluster reconfiguration: Dynamically add or remove nodes from the cluster without disrupting the overall system.
3. Data migration: Move data between nodes in the cluster to rebalance the workload or accommodate changes in the cluster's topology.
Error Handling in Redis Cluster
When working with Redis Cluster, it is important to handle errors effectively to maintain the integrity and availability of your data. Some common error scenarios include network partitions, node failures, and data inconsistencies.
To handle errors in Redis Cluster, you can use techniques such as retrying failed operations, implementing failover mechanisms with Redis Sentinel, and monitoring the cluster's health for early detection of issues.
Code Snippet Ideas for Redis Cluster
Here are a few code snippet ideas to help you get started with Redis Cluster:
1. Creating a connection pool for Redis Cluster using a client library like redis-py-cluster
in Python.
2. Implementing a cache invalidation strategy using Redis Cluster's EXPIRE
command and a background task to refresh the cache.
import redisimport time# Connect to the Redis Clustercluster = redis.RedisCluster(host='localhost', port=7000)# Set a key-value pair with an expiration time of 60 secondscluster.set('mykey', 'myvalue', ex=60)# Get the value of a keyvalue = cluster.get('mykey')print(value)# Sleep for 70 seconds to allow the key to expiretime.sleep(70)# Check if the key still existsexists = cluster.exists('mykey')print(exists) # Output: 0 (Key does not exist)
These code snippets demonstrate basic operations and a cache invalidation strategy using Redis Cluster.