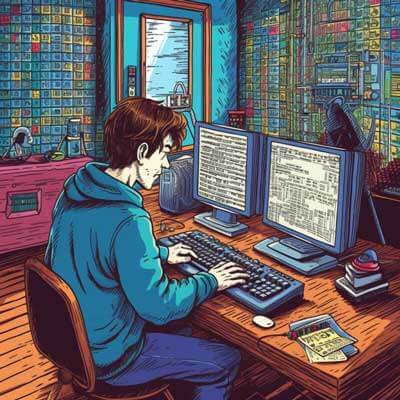
Table of Contents
Comparing Redis and MongoDB
Both Redis and MongoDB are popular choices for managing data, but they have different strengths and use cases. Here are some key points to consider when comparing Redis and MongoDB:
- Data Model: Redis is a key-value store, while MongoDB is a document store. Redis is ideal for simple key-value data, caching, and message brokering, while MongoDB is better suited for storing complex, structured data.
- Performance: Redis excels in performance due to its in-memory nature, making it a great choice for applications that require low-latency data access. MongoDB, on the other hand, provides good performance for read-heavy workloads and can scale horizontally.
- Querying: Redis provides basic querying capabilities based on keys, while MongoDB offers a flexible querying language that allows for complex queries and indexing.
- Durability: Redis can be configured to persist data to disk, but it is primarily an in-memory store. MongoDB provides durable storage by default, making it a better choice for applications where data durability is critical.
- Use Cases: Redis is commonly used for caching, real-time analytics, session management, and pub/sub messaging. MongoDB is often used for content management systems, customer databases, and applications with evolving data models.
Related Article: How to Check & Change the DB Directory in PostgreSQL
Use Cases for Redis
Redis is a versatile data store that can be used in various use cases. Here are a few examples:
Example 1: Caching
Redis is often used as a cache due to its fast read and write performance. By caching frequently accessed data in Redis, you can significantly improve the response time of your application. Here's an example of using Redis as a cache in a web application:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) # Try to fetch data from Redis cache data = r.get('mykey') if data is None: # Fetch data from the database data = fetch_data_from_database() # Store data in Redis cache r.set('mykey', data) r.expire('mykey', 3600) # Set expiry time to 1 hour # Use the data process_data(data)
In this example, we use the Redis Python client to connect to Redis, check if the requested data exists in the cache, and fetch it from the database if not. The data is then stored in Redis for future use.
Example 2: Leaderboards and Ranking
Redis provides sorted sets, which allow you to store a collection of elements with associated scores. This feature is useful for implementing leaderboards and ranking systems. Here's an example of using Redis sorted sets to track player scores in a game:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) # Add player scores to the leaderboard r.zadd('leaderboard', {'player1': 100, 'player2': 150, 'player3': 80}) # Get the top players top_players = r.zrevrange('leaderboard', 0, 2, withscores=True) # Print the leaderboard for player, score in top_players: print(f"{player}: {score}")
In this example, we use the Redis Python client to add player scores to the leaderboard using the zadd
command and retrieve the top players using the zrevrange
command.
Related Article: Positioning WHERE Clause After JOINs in SQL Databases
Use Cases for MongoDB
MongoDB's flexible document model makes it suitable for a wide range of use cases. Here are a couple of examples:
Example 1: Content Management System
MongoDB's document model allows for easy storage and retrieval of structured data, making it a good choice for content management systems (CMS). You can store articles, blog posts, and other content as documents in MongoDB. Here's an example of storing and retrieving an article in MongoDB using the Python driver:
from pymongo import MongoClient # Connect to MongoDB client = MongoClient('mongodb://localhost:27017/') db = client.mydatabase # Insert an article article = { 'title': 'Introduction to MongoDB', 'content': 'MongoDB is a NoSQL database...', 'author': 'John Doe' } db.articles.insert_one(article) # Retrieve an article retrieved_article = db.articles.find_one({ 'title': 'Introduction to MongoDB' }) print(retrieved_article['content'])
In this example, we use the PyMongo driver to connect to MongoDB, insert an article into the articles
collection, and retrieve the article by title.
Example 2: Internet of Things (IoT) Data
MongoDB's flexible schema and scalability make it well-suited for storing and analyzing Internet of Things (IoT) data. You can store sensor readings, device metadata, and other IoT data in MongoDB. Here's an example of storing and querying IoT data in MongoDB:
from pymongo import MongoClient # Connect to MongoDB client = MongoClient('mongodb://localhost:27017/') db = client.mydatabase # Insert a sensor reading reading = { 'sensor_id': 'sensor123', 'temperature': 25.3, 'humidity': 60.2, 'timestamp': datetime.datetime.now() } db.sensor_data.insert_one(reading) # Query sensor data query = { 'sensor_id': 'sensor123', 'timestamp': { '$gte': datetime.datetime(2022, 1, 1) } } results = db.sensor_data.find(query) for result in results: print(result)
In this example, we use the PyMongo driver to connect to MongoDB, insert a sensor reading into the sensor_data
collection, and query the sensor data based on sensor ID and timestamp.
Introduction to Redis
Redis is an open-source, in-memory data structure store that can be used as a database, cache, or message broker. It is known for its high performance and versatility, making it a popular choice for various use cases. Redis stores data in key-value pairs and supports a wide range of data types, including strings, hashes, lists, sets, and sorted sets.
Related Article: Analyzing SQL Join and Its Effect on Records
Example 1: Basic Redis Operations
To get started with Redis, you first need to install it on your system. Once installed, you can interact with Redis using the Redis command-line interface (CLI). Let's take a look at some basic Redis operations:
# Set a key-value pair SET mykey "Hello Redis" # Get the value of a key GET mykey
In this example, we set a key-value pair using the SET
command and retrieve the value using the GET
command.
Example 2: Redis Lists
Redis also provides data structures such as lists, which allow you to store and manipulate ordered collections of strings. Here's an example of working with lists in Redis:
# Push elements to the beginning of a list LPUSH mylist "element1" LPUSH mylist "element2" LPUSH mylist "element3" # Get the elements of a list LRANGE mylist 0 -1
In this example, we use the LPUSH
command to push elements to the beginning of a list and the LRANGE
command to retrieve all elements of the list.
Introduction to MongoDB
MongoDB is a popular NoSQL database that provides high scalability, flexibility, and performance. It stores data in flexible, JSON-like documents, allowing for easy storage and retrieval of complex data structures. MongoDB's document model is particularly well-suited for applications with evolving data requirements.
Example 1: Basic MongoDB Operations
To get started with MongoDB, you need to install the MongoDB server and the MongoDB command-line interface (CLI). Once set up, you can interact with MongoDB using the CLI or through a programming language driver. Here's an example of some basic MongoDB operations using the CLI:
# Insert a document into a collection db.myCollection.insertOne({ name: "John", age: 30 }) # Find documents in a collection db.myCollection.find({ name: "John" })
In this example, we insert a document into a collection using the insertOne
method and retrieve documents using the find
method.
Related Article: How to Create a PostgreSQL Read Only User
Example 2: MongoDB Aggregation Framework
MongoDB provides a powerful aggregation framework that allows you to perform complex data processing operations on your data. Here's an example of using the aggregation framework to calculate the average age of all documents in a collection:
db.myCollection.aggregate([ { $group: { _id: null, avgAge: { $avg: "$age" } } } ])
In this example, we use the $group
and $avg
operators to calculate the average age of all documents in the collection.
Best Practices for Redis
When working with Redis, it's important to follow best practices to ensure optimal performance and reliability. Here are a few best practices for Redis:
Managing Memory
- Set an appropriate maxmemory configuration to limit the amount of memory Redis can use.
- Use Redis' eviction policies (such as LRU or LFU) to automatically remove less frequently used data when memory is full.
- Monitor Redis' memory usage using the INFO command or tools like Redis Monitor.
Handling Persistence
- Configure Redis to persist data to disk using either RDB snapshots or AOF logs for durability.
- Regularly backup your Redis data to prevent data loss in case of hardware failures or other issues.
- Test your disaster recovery procedures by restoring Redis from backups.
Related Article: Tutorial on Redis Queue Implementation
Optimizing Performance
- Use Redis' native data structures and commands whenever possible to leverage its in-memory performance.
- Minimize network round trips by using Redis pipelines or Lua scripts to batch multiple commands into a single request.
- Consider using Redis Cluster or Redis Sentinel for high availability and automatic failover.
Example 1: Using Redis Pipelines
Redis pipelines allow you to send multiple commands to Redis in a batch, reducing network round trips and improving performance. Here's an example of using pipelines in Python:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) # Create a pipeline pipeline = r.pipeline() # Queue multiple commands in the pipeline pipeline.set('key1', 'value1') pipeline.set('key2', 'value2') pipeline.set('key3', 'value3') # Execute the pipeline pipeline.execute()
In this example, we use the Redis Python client to create a pipeline and queue multiple SET
commands. The execute
method is then called to send all commands to Redis in a single network round trip.
Example 2: Using Redis Pub/Sub
Redis also supports publish/subscribe messaging, allowing you to build real-time messaging systems. Here's an example of using Redis pub/sub in Python:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) # Subscribe to a channel pubsub = r.pubsub() pubsub.subscribe('mychannel') # Listen for messages for message in pubsub.listen(): print(message['data'])
In this example, we use the Redis Python client to subscribe to a channel and listen for messages. Any messages published to the channel will be printed to the console.
Best Practices for MongoDB
To ensure optimal performance and reliability when using MongoDB, it's important to follow best practices. Here are a few best practices for MongoDB:
Related Article: Merging Two Result Values in SQL
Indexing
- Identify and create appropriate indexes for queries that are frequently performed.
- Use compound indexes to cover multiple query fields efficiently.
- Regularly monitor the performance of your indexes and consider index optimization if necessary.
Schema Design
- Design your MongoDB schema based on your application's read and write patterns.
- Denormalize data where appropriate to minimize the need for expensive joins or lookups.
- Use MongoDB's document validation feature to enforce data consistency and integrity.
Scaling
- Monitor the performance of your MongoDB deployment and plan for horizontal scaling if necessary.
- Consider using sharding to distribute your data across multiple servers for improved performance and scalability.
- Regularly review and optimize your MongoDB configuration for your specific workload.
Example 1: Creating Indexes
Indexes play a crucial role in optimizing query performance in MongoDB. Here's an example of creating an index in MongoDB using the Python driver:
from pymongo import MongoClient # Connect to MongoDB client = MongoClient('mongodb://localhost:27017/') db = client.mydatabase # Create an index db.myCollection.create_index('myfield')
In this example, we use the PyMongo driver to connect to MongoDB and create an index on the myfield
field in the myCollection
collection.
Related Article: Redis Intro & Redis Alternatives
Example 2: Denormalizing Data
Denormalizing data in MongoDB can improve query performance by reducing the need for expensive joins or lookups. Here's an example of denormalizing data in MongoDB:
from pymongo import MongoClient # Connect to MongoDB client = MongoClient('mongodb://localhost:27017/') db = client.mydatabase # Store related data in a single document db.users.insert_one({ 'name': 'John Doe', 'email': 'john@example.com', 'address': { 'street': '123 Main St', 'city': 'New York', 'state': 'NY', 'zipcode': '10001' } })
In this example, we store the user's address as a nested document within the users
collection, eliminating the need for a separate addresses collection and allowing for more efficient queries.
Real World Examples using Redis
Redis has been widely adopted by various organizations for a range of real-world use cases. Here are a couple of examples:
Example 1: Session Management
Redis is commonly used for session management in web applications. By storing session data in Redis, you can easily share session state across multiple servers and scale your application horizontally. Here's an example of using Redis for session management in a Python web application using the Flask framework:
from flask import Flask, session from flask_session import Session import redis # Create the Flask application app = Flask(__name__) # Configure Flask-Session to use Redis app.config['SESSION_TYPE'] = 'redis' app.config['SESSION_REDIS'] = redis.Redis(host='localhost', port=6379, db=0) # Initialize the Flask-Session extension Session(app) # Use session in your routes @app.route('/') def index(): session['username'] = 'John' return "Session data stored in Redis"
In this example, we configure Flask-Session to use Redis as the session store and store the user's username in the session.
Example 2: Real-time Analytics
Redis' fast read and write performance makes it well-suited for real-time analytics applications. You can use Redis' data structures and commands to aggregate and analyze data in real-time. Here's an example of using Redis for real-time analytics in a Python application:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) # Record user activity def record_user_activity(user_id, activity_type): r.zincrby('user_activity', 1, f'user:{user_id}:{activity_type}') # Get top activities top_activities = r.zrevrange('user_activity', 0, 4, withscores=True) # Print top activities for activity, score in top_activities: print(f"{activity}: {score}")
In this example, we use Redis' sorted sets to record user activity and retrieve the top activities based on their scores.
Related Article: How to Use the WHERE Condition in SQL Joins
Real World Examples using MongoDB
MongoDB is widely used in various industries and applications. Here are a few real-world examples of using MongoDB:
Example 1: Content Management System
MongoDB's flexible document model and scalability make it a popular choice for building content management systems (CMS). MongoDB allows you to store and manage structured content efficiently. Here's an example of using MongoDB in a CMS:
// Insert a new article db.articles.insertOne({ title: 'Getting Started with MongoDB', content: 'MongoDB is a NoSQL database...', author: 'John Doe', createdAt: new Date() }) // Retrieve articles by author db.articles.find({ author: 'John Doe' })
In this example, we insert a new article into the articles
collection and retrieve articles by the author's name.
Example 2: E-commerce Application
MongoDB's flexibility and scalability make it suitable for building e-commerce applications. MongoDB allows you to store product catalogs, customer information, and order data in a flexible and efficient manner. Here's an example of using MongoDB in an e-commerce application:
// Insert a new product db.products.insertOne({ name: 'Smartphone', price: 999.99, description: 'A high-end smartphone', stock: 100 }) // Query products by price range db.products.find({ price: { $gte: 500, $lte: 1000 } })
In this example, we insert a new product into the products
collection and query products within a specific price range.
Performance Considerations for Redis
To ensure optimal performance when using Redis, there are certain considerations to keep in mind. Here are a few performance considerations for Redis:
Related Article: How to Use Redis with Django Applications
Memory Management
- Redis stores data in memory, so it's important to monitor and manage your memory usage.
- Use Redis' eviction policies to automatically remove less frequently used data when memory is full.
- Consider using Redis' data compression techniques, such as the ZIPLIST
encoding for lists or INTSET
encoding for sets with small values.
Scaling
- Redis can be scaled horizontally by using Redis Cluster or Redis Sentinel for high availability and automatic failover.
- Consider sharding your data across multiple Redis instances to distribute the load and improve performance.
Network Round Trips
- Minimize network round trips by using Redis pipelines or Lua scripts to batch multiple commands into a single request.
- Use Redis' MSET and MGET commands to set and retrieve multiple keys in a single operation.
Example 1: Using Redis Pipelines
Redis pipelines allow you to send multiple commands to Redis in a batch, reducing network round trips and improving performance. Here's an example of using pipelines in Python:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) # Create a pipeline pipeline = r.pipeline() # Queue multiple commands in the pipeline pipeline.set('key1', 'value1') pipeline.set('key2', 'value2') pipeline.set('key3', 'value3') # Execute the pipeline pipeline.execute()
In this example, we use the Redis Python client to create a pipeline and queue multiple SET
commands. The execute
method is then called to send all commands to Redis in a single network round trip.
Related Article: How to Join Three Tables in SQL
Example 2: Using Redis Lua Scripting
Redis provides Lua scripting support, allowing you to execute complex operations on the server side. This can reduce the number of network round trips and improve performance. Here's an example of using Lua scripting in Redis:
local value = redis.call('GET', 'mykey') if value == nil then redis.call('SET', 'mykey', 'default_value') end return redis.call('GET', 'mykey')
In this example, we use Lua scripting to check if a key exists and set a default value if it doesn't. The script is executed on the server side, reducing the number of round trips between the client and server.
Performance Considerations for MongoDB
To ensure optimal performance when using MongoDB, there are certain considerations to keep in mind. Here are a few performance considerations for MongoDB:
Indexing
- Identify and create appropriate indexes for queries that are frequently performed.
- Use compound indexes to cover multiple query fields efficiently.
- Regularly monitor the performance of your indexes and consider index optimization if necessary.
Sharding
- MongoDB can be scaled horizontally by sharding your data across multiple servers.
- Carefully choose your shard key to evenly distribute data and queries across shards.
- Monitor the performance and load distribution of your shards to ensure balanced scaling.
Related Article: How to Use Redis Streams
Query Optimization
- Understand the query patterns of your application and optimize your queries accordingly.
- Use MongoDB's explain feature to analyze query execution plans and identify potential performance bottlenecks.
- Consider denormalizing your data to avoid expensive joins or lookups.
Example 1: Creating Indexes
Indexes play a crucial role in optimizing query performance in MongoDB. Here's an example of creating an index in MongoDB using the Python driver:
from pymongo import MongoClient # Connect to MongoDB client = MongoClient('mongodb://localhost:27017/') db = client.mydatabase # Create an index db.myCollection.create_index('myfield')
In this example, we use the PyMongo driver to connect to MongoDB and create an index on the myfield
field in the myCollection
collection.
Example 2: Using Query Optimizer
MongoDB's query optimizer automatically selects the most efficient query execution plan based on the available indexes and statistics. However, in some cases, you may need to provide hints to the optimizer to optimize query performance. Here's an example of using query hints in MongoDB:
db.myCollection.find({ myfield: 'value' }).hint({ myfield: 1 })
In this example, we use the hint
method to provide a hint to the query optimizer to use the index on the myfield
field. This can help optimize the query execution plan and improve performance.
Advanced Techniques in Redis
Redis provides several advanced techniques and features that can be used to enhance your applications. Here are a couple of examples:
Related Article: How to Fix MySQL Error Code 1175 in Safe Update Mode
Pub/Sub Messaging
Redis supports publish/subscribe messaging, allowing you to build real-time messaging systems. Publishers can send messages to specific channels, and subscribers can listen to those channels to receive messages. Here's an example of using Redis pub/sub:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) # Subscribe to a channel pubsub = r.pubsub() pubsub.subscribe('mychannel') # Listen for messages for message in pubsub.listen(): print(message['data'])
In this example, we use the Redis Python client to subscribe to a channel and listen for messages. Any messages published to the channel will be printed to the console.
Lua Scripting
Redis provides Lua scripting support, allowing you to execute complex operations on the server side. Lua scripts are executed atomically and can help reduce the number of network round trips between the client and server. Here's an example of using Lua scripting in Redis:
local value = redis.call('GET', 'mykey') if value == nil then redis.call('SET', 'mykey', 'default_value') end return redis.call('GET', 'mykey')
In this example, we use Lua scripting to check if a key exists and set a default value if it doesn't. The script is executed on the server side, reducing the number of round trips between the client and server.
Advanced Techniques in MongoDB
MongoDB provides several advanced techniques and features that can be used to enhance your applications. Here are a couple of examples:
Aggregation Framework
MongoDB's aggregation framework allows you to perform complex data processing operations on your data. It provides a set of operators and stages that can be used to perform grouping, filtering, sorting, and more. Here's an example of using the aggregation framework in MongoDB:
db.myCollection.aggregate([ { $group: { _id: "$category", total: { $sum: "$quantity" } } }, { $sort: { total: -1 } }, { $limit: 5 } ])
In this example, we use the aggregation framework to group documents by category, calculate the total quantity for each category, sort the results in descending order, and limit the results to the top 5 categories.
Related Article: Implementing a Cross Join SQL in Databases
Change Streams
MongoDB's change streams allow you to capture real-time data changes in a collection. You can use change streams to monitor inserts, updates, and deletes in real-time and take actions based on those changes. Here's an example of using change streams in MongoDB:
const pipeline = [ { $match: { operationType: { $in: ["insert", "update", "delete"] } } } ] const changeStream = db.myCollection.watch(pipeline) changeStream.on('change', (change) => { console.log(change) })
In this example, we create a change stream that captures insert, update, and delete operations in the myCollection
collection. Whenever a change occurs, the change
event is triggered, and the change details are printed to the console.
Code Snippet Ideas for Redis
Redis provides a rich set of commands and data structures that can be used to build various types of applications. Here are a few code snippet ideas for working with Redis:
Example 1: Rate Limiting
Redis can be used for rate limiting to control the number of requests or operations allowed within a certain time period. Here's an example of implementing a simple rate limiter in Redis using the INCR
command:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) # Check if rate limit is exceeded def is_rate_limit_exceeded(user_id, limit, period): key = f"rate_limit:{user_id}" count = r.incr(key) if count == 1: # Set expiration time for the key r.expire(key, period) return count > limit # Usage example user_id = 123 limit = 10 period = 60 # 1 minute if is_rate_limit_exceeded(user_id, limit, period): print("Rate limit exceeded") else: print("Request allowed")
In this example, we use the INCR
command to increment a counter for each request from a specific user. If the counter exceeds the specified limit within the given period, the rate limit is considered exceeded.
Example 2: Distributed Locks
Redis can be used to implement distributed locks, which allow multiple processes or threads to coordinate and ensure exclusive access to a shared resource. Here's an example of implementing a distributed lock in Redis using the SETNX
command:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) # Acquire a lock def acquire_lock(lock_name, timeout): lock_key = f"lock:{lock_name}" acquired = r.setnx(lock_key, 1) if acquired: # Set expiration time for the lock key r.expire(lock_key, timeout) return acquired # Usage example lock_name = 'mylock' timeout = 10 # 10 seconds if acquire_lock(lock_name, timeout): try: # Perform exclusive operations print("Lock acquired") finally: # Release the lock r.delete(lock_name) else: print("Failed to acquire lock")
In this example, we use the SETNX
command to set a lock key in Redis. If the lock key is successfully set (indicating that the lock is acquired), the exclusive operations are performed. Finally, the lock is released by deleting the lock key.
Related Article: Tutorial: Setting Up Redis Using Docker Compose
Code Snippet Ideas for MongoDB
MongoDB provides a rich set of features and query capabilities that can be used to build various types of applications. Here are a few code snippet ideas for working with MongoDB:
Example 1: Aggregation Pipeline
MongoDB's aggregation pipeline allows you to perform complex data processing operations on your data. Here's an example of using the aggregation pipeline in MongoDB with the PyMongo driver:
from pymongo import MongoClient # Connect to MongoDB client = MongoClient('mongodb://localhost:27017/') db = client.mydatabase # Perform aggregation pipeline = [ { '$match': { 'category': 'Books' } }, { '$group': { '_id': '$author', 'total': { '$sum': '$quantity' } } }, { '$sort': { 'total': -1 } }, { '$limit': 5 } ] results = db.myCollection.aggregate(pipeline) # Print results for result in results: print(result)
In this example, we use the PyMongo driver to connect to MongoDB and perform an aggregation on the myCollection
collection. The aggregation pipeline includes stages such as $match
, $group
, $sort
, and $limit
to filter, group, sort, and limit the results.
Example 2: Geospatial Queries
MongoDB supports geospatial queries, allowing you to perform queries based on location data. Here's an example of using geospatial queries in MongoDB with the PyMongo driver:
from pymongo import MongoClient from pymongo import GEO2D # Connect to MongoDB client = MongoClient('mongodb://localhost:27017/') db = client.mydatabase # Enable geospatial indexing db.myCollection.create_index([("location", GEO2D)]) # Perform geospatial query query = { 'location': { '$near': { '$geometry': { 'type': 'Point', 'coordinates': [longitude, latitude] }, '$maxDistance': 1000 # 1 kilometer } } } results = db.myCollection.find(query) # Print results for result in results: print(result)
In this example, we use the PyMongo driver to connect to MongoDB and perform a geospatial query on the myCollection
collection. The query uses the $near
operator to find documents near a specific location within a specified distance.
Error Handling in Redis
When working with Redis, it's important to handle errors properly to ensure the reliability of your applications. Here are a few error handling techniques for Redis:
- Use Redis transactions to ensure atomicity and consistency of multiple operations.
- Monitor Redis' error logs to identify and troubleshoot any issues.
- Handle Redis network errors and connection failures gracefully by implementing retry logic or failover mechanisms.
Related Article: Tutorial: Installing PostgreSQL on Amazon Linux
Example 1: Redis Transactions
Redis transactions allow you to group multiple commands into a single atomic operation. Here's an example of using transactions in Redis using the Python client:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) # Perform a transaction with r.pipeline() as pipe: while True: try: # Start the transaction pipe.multi() # Queue multiple commands in the transaction pipe.set('key1', 'value1') pipe.set('key2', 'value2') # Execute the transaction pipe.execute() break except redis.WatchError: # Retry the transaction if there was a concurrent modification continue
In this example, we use the Redis Python client to perform a transaction. The multi
method starts the transaction, and the execute
method executes all commands in the transaction atomically. If a concurrent modification occurs during the transaction, the WatchError
exception is raised, and the transaction is retried.
Example 2: Handling Redis Network Errors
When working with Redis, it's important to handle network errors and connection failures gracefully. Here's an example of handling network errors in Redis using the Python client:
import redis import time # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) # Retry connection in case of network error def connect_with_retry(): while True: try: # Attempt to connect to Redis r.ping() return except redis.ConnectionError: # Sleep for a while before retrying time.sleep(1) # Usage example connect_with_retry() r.set('key', 'value')
In this example, the connect_with_retry
function attempts to connect to Redis and retries in case of a ConnectionError
. The function uses a sleep period to introduce a delay between connection attempts.