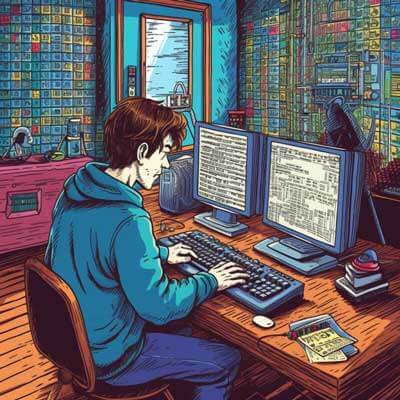
Table of Contents
Checking for a Table Presence with the System Catalog Tables
One of the simplest ways to verify table existence in PostgreSQL is by querying the system catalog tables. PostgreSQL stores metadata about tables, columns, indexes, and other database objects in system catalog tables. By querying these catalog tables, we can determine if a specific table exists in the database.
Here is an example of how to check if a table exists using the system catalog tables:
SELECT EXISTS ( SELECT 1 FROM pg_tables WHERE schemaname = 'public' AND tablename = 'my_table' );
In this example, we use the pg_tables
catalog table to check if a table named 'my_table' exists in the 'public' schema. The query returns a boolean value indicating whether the table exists or not.
Another approach to check table presence is by using the information_schema
views. The information_schema
views provide a standardized way to access metadata about database objects. Here is an example using the information_schema.tables
view:
SELECT EXISTS ( SELECT 1 FROM information_schema.tables WHERE table_schema = 'public' AND table_name = 'my_table' );
This query is similar to the previous example but uses the information_schema.tables
view instead. It also returns a boolean value indicating the existence of the table.
Related Article: Merging Two Result Values in SQL
Determining Table Existence with to_regclasss
In addition to querying the system catalog tables, PostgreSQL provides a built-in function called to_regclass
that can be used to determine the existence of a table. The to_regclass
function takes a table name as an argument and returns the OID (Object ID) of the table if it exists, or NULL
if it does not exist.
Here is an example of how to use the to_regclass
function to determine table existence:
SELECT to_regclass('public.my_table');
In this example, we pass the fully qualified table name ('public.my_table') to the to_regclass
function. If the table exists, the function will return the OID of the table. Otherwise, it will return NULL
.
Using the pg_class Catalog table
Related Article: Executing Queries to Remove Duplicate Rows in PostgreSQL
Another way to find table existence in PostgreSQL is by querying the pg_class
catalog table. The pg_class
table stores information about various objects in the database, including tables. By filtering the table name and schema name, we can determine if a specific table exists.
Here is an example of how to find table existence using the pg_class
table:
SELECT EXISTS ( SELECT 1 FROM pg_class c JOIN pg_namespace n ON n.oid = c.relnamespace WHERE n.nspname = 'public' AND c.relname = 'my_table' AND c.relkind = 'r' );
In this example, we join the pg_class
table with the pg_namespace
table to get the schema information. We then filter the results based on the schema name ('public'), table name ('my_table'), and object type ('r' for a table). The query returns a boolean value indicating the existence of the table.