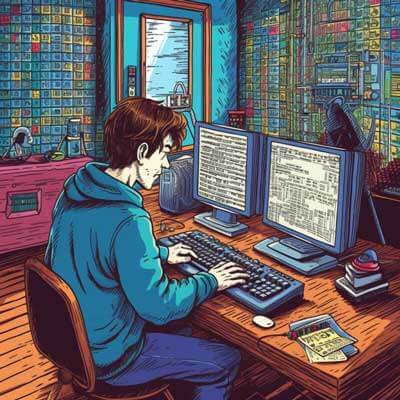
Table of Contents
Introduction to SQL Like
In databases, the SQL Like operator is used to search for patterns in text data. It allows you to perform pattern matching using wildcard characters. The Like operator is commonly used with the SELECT statement to filter data based on specific patterns.
Related Article: How to Insert Multiple Rows in a MySQL Database
Example 1: Basic Pattern Matching
SELECT * FROM users WHERE name LIKE 'J%'
This query will return all the users whose names start with the letter 'J'. The '%' wildcard character represents any number of characters, so the query will match names like 'John', 'Jessica', and 'Jennifer'.
Example 2: Pattern Matching with Multiple Wildcards
SELECT * FROM products WHERE description LIKE '%widget%'
This query will return all the products whose description contains the word 'widget'. The '%' wildcard character can be used at the beginning, middle, or end of the pattern to match any number of characters.
Introduction to SQL Not Like
The SQL Not Like operator is the negation of the Like operator. It is used to exclude rows that match a specified pattern. The Not Like operator is often used with the WHERE clause to filter out unwanted data.
Related Article: Impact of Joins on Missing Data in SQL Databases
Example 1: Excluding Rows with a Specific Pattern
SELECT * FROM customers WHERE email NOT LIKE '%gmail.com'
This query will return all the customers whose email addresses do not end with 'gmail.com'. The '%' wildcard character is used to match any number of characters, so the query will exclude email addresses like 'john@gmail.com' and 'jane@gmail.com'.
Example 2: Excluding Rows with Multiple Patterns
SELECT * FROM products WHERE description NOT LIKE '%widget%' AND description NOT LIKE '%gadget%'
This query will return all the products whose description does not contain the words 'widget' or 'gadget'. The '%' wildcard character can be used multiple times to exclude rows that match different patterns.
Use Cases for SQL Like
Filtering Data based on Patterns
SELECT * FROM employees WHERE name LIKE 'S%'
This query can be used to retrieve all employees whose names start with the letter 'S'. It is useful when you want to filter data based on specific patterns, such as finding all employees with a certain last name initial.
Related Article: Tutorial on SQL IN and NOT IN Operators in Databases
Searching for Partial Matches
SELECT * FROM products WHERE name LIKE '%book%'
This query can be used to search for products whose names contain the word 'book'. It is helpful when you want to find products that are related to a particular category or topic.
Use Cases for SQL Not Like
Excluding Specific Patterns
SELECT * FROM customers WHERE email NOT LIKE '%hotmail.com'
This query can be used to exclude customers whose email addresses end with 'hotmail.com'. It is useful when you want to exclude certain patterns from your result set.
Filtering Out Unwanted Data
SELECT * FROM orders WHERE status NOT LIKE '%cancelled%'
This query can be used to filter out orders with a status of 'cancelled'. It is helpful when you want to focus on active or completed orders and ignore any cancelled ones.
Related Article: How to Insert Multiple Rows in a MySQL Database
Best Practices for SQL Like
Use Indexes for Performance
CREATE INDEX idx_name ON customers (name)
Creating an index on the column used in the Like operator can significantly improve query performance. In this example, an index is created on the 'name' column of the 'customers' table, which can speed up queries that involve pattern matching on names.
Be Mindful of Leading Wildcards
Using a leading wildcard, such as '%text', can cause performance issues as it requires a full table scan. It is recommended to avoid leading wildcards whenever possible, or consider alternative approaches like full-text search.
Best Practices for SQL Not Like
Related Article: Tutorial: Full Outer Join versus Join in SQL
Optimize Patterns with Leading Wildcards
When using the Not Like operator, it is important to optimize patterns that start with a leading wildcard. These patterns can be slow and resource-intensive. Consider using alternative approaches or optimizing the query logic to avoid leading wildcards.
Combine with Other Operators for Precise Filtering
SELECT * FROM products WHERE description NOT LIKE '%widget%' AND price > 10
Combining the Not Like operator with other operators, such as greater than or less than, can provide precise filtering capabilities. In this example, the query returns products whose description does not contain the word 'widget' and have a price greater than 10.
Real World Examples of SQL Like
Searching for Usernames
SELECT * FROM users WHERE username LIKE '%admin%'
This query can be used to search for users whose usernames contain the word 'admin'. It is useful when you want to find administrator accounts or users with specific roles.
Related Article: How to Perform a Full Outer Join in MySQL
Filtering by Zip Code Prefix
SELECT * FROM addresses WHERE zip_code LIKE '123%'
This query can be used to filter addresses based on a specific zip code prefix. It retrieves all addresses where the zip code starts with '123', such as '12345' and '12367'.
Real World Examples of SQL Not Like
Excluding Invalid Email Addresses
SELECT * FROM customers WHERE email NOT LIKE '%@%'
This query can be used to exclude customers with invalid email addresses. It retrieves all customers whose email does not contain the '@' symbol, helping to filter out potential data entry errors.
Filtering Out Profanity
SELECT * FROM comments WHERE content NOT LIKE '%profanity%'
This query can be used to exclude comments that contain profanity. It is helpful when you want to maintain a clean and respectful environment by filtering out inappropriate content.
Related Article: Using Stored Procedures in MySQL
Performance Considerations for SQL Like
Avoid Leading Wildcards
As mentioned earlier, using leading wildcards, such as '%text', can negatively impact query performance. It is recommended to use trailing or embedded wildcards whenever possible to improve the efficiency of pattern matching queries.
Optimize Indexing
Creating appropriate indexes on columns involved in pattern matching queries can significantly improve performance. Analyze your query patterns and identify the frequently used columns for indexing to optimize the execution speed.
Performance Considerations for SQL Not Like
Related Article: Integrating Fluent Bit with PostgreSQL Databases
Optimize Query Logic
When using the Not Like operator, it is important to optimize the query logic to minimize the number of rows that need to be processed. Consider combining the Not Like operator with other operators or using alternative approaches to reduce the overall query execution time.
Use Negation Operators Judiciously
Be cautious when using negation operators, such as Not Like, as they can lead to complex and inefficient queries. Evaluate the query performance and consider alternative approaches if necessary to ensure optimal execution speed.
Advanced Techniques for SQL Like
Case-Insensitive Matching
SELECT * FROM users WHERE LOWER(name) LIKE '%john%'
This query performs a case-insensitive search for users whose names contain the word 'john'. The LOWER function is used to convert the name column to lowercase, allowing the pattern matching to be case-insensitive.
Related Article: Tutorial: Installing PostgreSQL on Amazon Linux
Matching Numeric Patterns
SELECT * FROM invoices WHERE invoice_number LIKE 'INV%'
This query retrieves all invoices with invoice numbers that start with 'INV'. It demonstrates how the Like operator can be used to match patterns in numeric data as well.
Advanced Techniques for SQL Not Like
Excluding Multiple Patterns
SELECT * FROM products WHERE description NOT LIKE '%widget%' AND description NOT LIKE '%gadget%'
This query excludes products whose descriptions contain either the word 'widget' or 'gadget'. It showcases how the Not Like operator can be combined with logical operators to exclude multiple patterns.
Using Regular Expressions
SELECT * FROM users WHERE name NOT REGEXP '^[A-Z]'
This query excludes users whose names do not start with an uppercase letter. It uses regular expressions to define the pattern to be matched or excluded.
Related Article: Efficient Methods for Timing Queries in Cassandra
Code Snippet Ideas for SQL Like - 1
SELECT * FROM products WHERE name LIKE 'A%'
This query retrieves all products whose names start with the letter 'A'. It demonstrates the usage of the Like operator to filter data based on a specific pattern.
SELECT * FROM customers WHERE phone_number LIKE '%555%'
This query retrieves all customers whose phone numbers contain the digits '555'. It showcases how the Like operator can be used to search for patterns in numerical data.
Code Snippet Ideas for SQL Like - 2
SELECT * FROM orders WHERE order_date LIKE '2022-05-%'
This query retrieves all orders placed in May 2022. It utilizes the Like operator to match a specific pattern in the order date column.
SELECT * FROM products WHERE description LIKE '%chair%'
This query retrieves all products whose descriptions contain the word 'chair'. It demonstrates how the Like operator can be used to search for patterns in text data.
Code Snippet Ideas for SQL Like - 3
SELECT * FROM employees WHERE address LIKE '%San Francisco%'
This query retrieves all employees whose addresses contain the phrase 'San Francisco'. It showcases how the Like operator can be used to filter data based on specific patterns in textual columns.
SELECT * FROM customers WHERE email LIKE '%@gmail.com'
This query retrieves all customers whose email addresses end with '@gmail.com'. It demonstrates how the Like operator can be utilized for pattern matching in email addresses.
Code Snippet Ideas for SQL Like - 4
SELECT * FROM products WHERE name LIKE 'P%r'
This query retrieves all products whose names start with 'P' and end with 'r'. It demonstrates how the Like operator can be used to match a pattern with specific characters in the middle.
SELECT * FROM orders WHERE order_number LIKE '%-%-%'
This query retrieves all orders whose order numbers contain at least two hyphens. It showcases how the Like operator can be used to find patterns with specific characters in multiple positions.
Related Article: Tutorial: Testing Cassandra Query Speed
Code Snippet Ideas for SQL Like - 5
SELECT * FROM users WHERE username LIKE '%_admin%'
This query retrieves all users whose usernames contain the substring '_admin'. The underscore '_' wildcard character matches a single character, allowing for more specific pattern matching.
SELECT * FROM products WHERE price LIKE '9%'
This query retrieves all products whose prices start with the digit '9'. It shows how the Like operator can be used to match patterns in numeric data.
Code Snippet Ideas for SQL Not Like - 1
SELECT * FROM customers WHERE email NOT LIKE '%yahoo.com'
This query retrieves all customers whose email addresses do not end with 'yahoo.com'. It demonstrates the usage of the Not Like operator to exclude specific patterns.
SELECT * FROM orders WHERE order_date NOT LIKE '2022-05-%'
This query retrieves all orders placed outside of May 2022. It showcases the Not Like operator's ability to exclude rows based on a specific pattern.
Code Snippet Ideas for SQL Not Like - 2
SELECT * FROM products WHERE description NOT LIKE '%sale%'
This query retrieves all products whose descriptions do not contain the word 'sale'. It demonstrates how the Not Like operator can be used to filter out rows based on a specific pattern.
SELECT * FROM customers WHERE phone_number NOT LIKE '%555%'
This query retrieves all customers whose phone numbers do not contain the digits '555'. It showcases the usage of the Not Like operator to exclude rows based on a pattern in numerical data.
Code Snippet Ideas for SQL Not Like - 3
SELECT * FROM employees WHERE address NOT LIKE '%New York%'
This query retrieves all employees whose addresses do not contain the phrase 'New York'. It demonstrates the usage of the Not Like operator to exclude rows based on a specific pattern in textual columns.
SELECT * FROM customers WHERE email NOT LIKE '%@gmail.com'
This query retrieves all customers whose email addresses do not end with '@gmail.com'. It showcases the Not Like operator's capability to exclude rows based on a pattern in email addresses.
Related Article: How to Set Timestamps With & Without Time Zone in PostgreSQL
Code Snippet Ideas for SQL Not Like - 4
SELECT * FROM products WHERE name NOT LIKE 'P%'
This query retrieves all products whose names do not start with the letter 'P'. It demonstrates the Not Like operator's ability to exclude rows based on a specific pattern.
SELECT * FROM orders WHERE order_number NOT LIKE '%-%-%'
This query retrieves all orders whose order numbers do not contain at least two hyphens. It showcases how the Not Like operator can be used to exclude rows that do not match a specific pattern.
Code Snippet Ideas for SQL Not Like - 5
SELECT * FROM users WHERE username NOT LIKE '%_admin%'
This query retrieves all users whose usernames do not contain the substring '_admin'. It demonstrates how the Not Like operator can exclude rows based on a specific pattern.
SELECT * FROM products WHERE price NOT LIKE '9%'
This query retrieves all products whose prices do not start with the digit '9'. It showcases the usage of the Not Like operator to exclude rows based on a pattern in numeric data.
Error Handling for SQL Like
When using the Like operator, it is important to be aware of potential errors that can occur:
Invalid Pattern Syntax
If the pattern used in the Like operator has invalid syntax, such as an unmatched wildcard, it can result in a syntax error. Ensure that the pattern is correctly formatted and does not contain any syntax errors.
Related Article: Working With PostgreSQL: Extracting Day of Week
Performance Issues with Leading Wildcards
Using a leading wildcard, such as '%text', can cause performance issues, as mentioned earlier. Be cautious when using leading wildcards and consider alternative approaches if performance becomes a concern.
Error Handling for SQL Not Like
When using the Not Like operator, you should be aware of potential errors:
Invalid Pattern Syntax
If the pattern used in the Not Like operator has invalid syntax, it can result in a syntax error. Ensure that the pattern is correctly formatted and does not contain any syntax errors.
Excluding Unintended Rows
When using the Not Like operator, be careful to exclude the desired rows. Double-check the pattern and ensure that it filters out the rows you intend to exclude while not excluding any other relevant data.