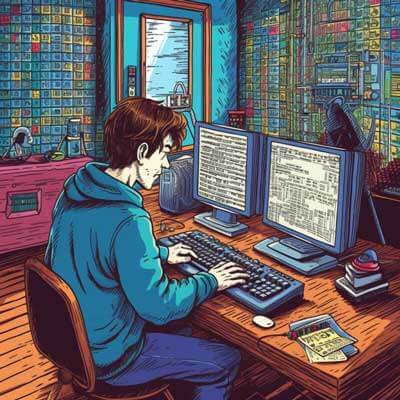
Table of Contents
In SQL, alias field joining refers to the ability to join tables using aliases for the fields. This allows us to give a temporary name to a field or expression in a query and use that alias to refer to the field in other parts of the query, such as in the JOIN clause.
Let's consider an example to illustrate alias field joining in SQL:
Suppose we have two tables, "customers" and "orders", with the following structures:
Table: customers
| customer_id | customer_name | |-------------|---------------| | 1 | John Smith | | 2 | Jane Doe | | 3 | Alice Johnson |
Table: orders
| order_id | customer_id | product | |----------|-------------|-------------| | 1 | 1 | T-shirt | | 2 | 1 | Jeans | | 3 | 2 | Dress | | 4 | 3 | Shoes |
To join these tables using aliases, we can write the following SQL query:
SELECT c.customer_name, o.product FROM customers c JOIN orders o ON c.customer_id = o.customer_id;
In this query, we have used "c" as an alias for the "customers" table and "o" as an alias for the "orders" table. We then use these aliases to refer to the fields in the SELECT and JOIN clauses.
The result of this query will be:
| customer_name | product | |---------------|-------------| | John Smith | T-shirt | | John Smith | Jeans | | Jane Doe | Dress | | Alice Johnson | Shoes |
Using Aliases in SQL
Related Article: How to Determine the Length of Strings in PostgreSQL
Aliases can be used in various parts of an SQL query, including the SELECT clause, FROM clause, JOIN clause, and WHERE clause.
Using Aliases in the SELECT Clause
In the SELECT clause, aliases can be used to give temporary names to fields or expressions. This can make the output of the query more readable and meaningful.
Consider the following example:
SELECT customer_name AS Name, COUNT(*) AS OrderCount FROM customers GROUP BY customer_name;
In this query, we have used the alias "Name" for the field "customer_name" and the alias "OrderCount" for the result of the COUNT(*) function. The result of this query will be:
| Name | OrderCount | |---------------|------------| | John Smith | 2 | | Jane Doe | 1 | | Alice Johnson | 1 |
Using Aliases in the FROM Clause
Aliases can also be used in the FROM clause to give temporary names to tables or subqueries. This can be useful when dealing with complex queries that involve multiple tables or subqueries.
Consider the following example:
SELECT o.product FROM orders AS o JOIN (SELECT customer_id FROM customers WHERE customer_name = 'John Smith') AS c ON o.customer_id = c.customer_id;
In this query, we have used the alias "o" for the "orders" table and the alias "c" for the subquery that selects the customer_id for the customer with the name 'John Smith'. We then join the "orders" table with the subquery using aliases.
Using Aliases in the JOIN Clause
Aliases can also be used in the JOIN clause to join tables using temporary names. This can make the JOIN clause more readable and concise.
Consider the following example:
SELECT c.customer_name, o.product FROM customers AS c JOIN orders AS o ON c.customer_id = o.customer_id;
In this query, we have used the aliases "c" and "o" for the "customers" and "orders" tables, respectively. We then join the tables using these aliases in the ON clause.
Using Aliases in the WHERE Clause
Aliases can also be used in the WHERE clause to refer to fields or expressions defined in the SELECT clause. This can make the WHERE clause more readable and concise.
Consider the following example:
SELECT customer_name, order_date FROM orders WHERE YEAR(order_date) = 2021;
In this query, we have used the alias "order_date" to refer to the result of the YEAR() function in the WHERE clause. This allows us to filter the orders based on the year of the order date.