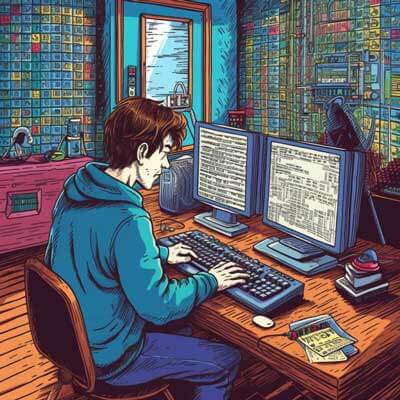
- Inserting Data into a Temporary Table in PostgreSQL
- Selecting Data from a Temporary Table in PostgreSQL
- Modifying Data in a Temporary Table in PostgreSQL
- Dropping a Temporary Table in PostgreSQL
- Using Constraints in a Temporary Table in PostgreSQL
- Indexing a Temporary Table in PostgreSQL
- Managing Transactions with Temporary Tables in PostgreSQL
- Best Practices for Using Temporary Tables in PostgreSQL
- Additional Resources
In PostgreSQL, a temporary table is a table that exists only for the duration of a session or a transaction. It is useful for storing intermediate results or temporary data that you don’t need to persist. The SELECT INTO TEMP statement allows you to create a temporary table and populate it with data from an existing table or a query result.
Creating a temporary table in PostgreSQL is straightforward. You can use the SELECT INTO TEMP statement to create a temporary table and populate it with data from an existing table or a query result. Here’s an example:
SELECT column1, column2 INTO TEMPORARY table_name FROM existing_table;
In the above example, we specify the columns we want to select from an existing table and use the INTO TEMPORARY clause to create a temporary table with the specified name. The data from the existing table is then inserted into the temporary table.
It’s important to note that the temporary table is only visible within the current session or transaction. Once the session or transaction ends, the temporary table is automatically dropped.
Inserting Data into a Temporary Table in PostgreSQL
To insert data into a temporary table in PostgreSQL, you can use the INSERT INTO statement. Here’s an example:
INSERT INTO temporary_table (column1, column2) VALUES (value1, value2);
In the above example, we specify the columns we want to insert data into and use the VALUES clause to provide the corresponding values.
You can also insert data into a temporary table by selecting data from an existing table or a query result. Here’s an example:
INSERT INTO temporary_table (column1, column2) SELECT column1, column2 FROM existing_table;
In the above example, we use the SELECT statement to select the data we want to insert into the temporary table.
Related Article: PostgreSQL HyperLogLog (HLL) & Cardinality Estimation
Selecting Data from a Temporary Table in PostgreSQL
To select data from a temporary table in PostgreSQL, you can use the SELECT statement. Here’s an example:
SELECT column1, column2 FROM temporary_table;
In the above example, we specify the columns we want to select from the temporary table.
You can also use the WHERE clause to filter the data returned. Here’s an example:
SELECT column1, column2 FROM temporary_table WHERE column1 = 'value';
In the above example, we use the WHERE clause to filter the data based on a condition.
Modifying Data in a Temporary Table in PostgreSQL
To modify data in a temporary table in PostgreSQL, you can use the UPDATE statement. Here’s an example:
UPDATE temporary_table SET column1 = 'new value' WHERE column2 = 'value';
In the above example, we use the SET clause to specify the new value for a column and the WHERE clause to filter the rows to be updated.
You can also delete data from a temporary table using the DELETE statement. Here’s an example:
DELETE FROM temporary_table WHERE column1 = 'value';
In the above example, we use the WHERE clause to filter the rows to be deleted.
Dropping a Temporary Table in PostgreSQL
To drop a temporary table in PostgreSQL, you can use the DROP TABLE statement. Here’s an example:
DROP TABLE temporary_table;
In the above example, we specify the name of the temporary table to be dropped.
It’s important to note that a temporary table is automatically dropped at the end of the session or transaction, so you don’t need to explicitly drop it in most cases.
Related Article: How to Check if a Table Exists in PostgreSQL
Using Constraints in a Temporary Table in PostgreSQL
You can use constraints in a temporary table in PostgreSQL to enforce data integrity and ensure that the data in the table meets certain criteria. Here are some examples of constraints you can use:
– Primary Key Constraint: Ensures that a column or a combination of columns in the temporary table uniquely identifies each row. Here’s an example:
CREATE TEMPORARY TABLE temporary_table ( id SERIAL PRIMARY KEY, name VARCHAR(50) );
In the above example, we use the PRIMARY KEY constraint to ensure that the “id” column uniquely identifies each row.
– Foreign Key Constraint: Establishes a relationship between the temporary table and another table based on a column or a combination of columns. Here’s an example:
CREATE TEMPORARY TABLE temporary_table ( id SERIAL PRIMARY KEY, category_id INTEGER, name VARCHAR(50), FOREIGN KEY (category_id) REFERENCES categories (id) );
In the above example, we use the FOREIGN KEY constraint to establish a relationship between the “category_id” column in the temporary table and the “id” column in the “categories” table.
– Check Constraint: Ensures that the values in a column meet certain criteria. Here’s an example:
CREATE TEMPORARY TABLE temporary_table ( id SERIAL PRIMARY KEY, age INTEGER CHECK (age >= 18) );
In the above example, we use the CHECK constraint to ensure that the “age” column in the temporary table has a value greater than or equal to 18.
Indexing a Temporary Table in PostgreSQL
You can create indexes on a temporary table in PostgreSQL to improve the performance of queries that involve the table. Here’s an example of creating an index on a temporary table:
CREATE INDEX index_name ON temporary_table (column1, column2);
In the above example, we specify the name of the index and the columns to be indexed in the temporary table.
You can also create unique indexes and partial indexes on a temporary table for more specific indexing requirements.
Managing Transactions with Temporary Tables in PostgreSQL
Temporary tables in PostgreSQL are automatically dropped at the end of a session or transaction. However, if you want to manage transactions explicitly, you can use the BEGIN, COMMIT, and ROLLBACK statements. Here’s an example:
BEGIN; CREATE TEMPORARY TABLE temporary_table ( id SERIAL PRIMARY KEY, name VARCHAR(50) ); INSERT INTO temporary_table (name) VALUES ('John'); COMMIT;
In the above example, we use the BEGIN statement to start a transaction, create a temporary table, insert data into the temporary table, and finally, use the COMMIT statement to commit the transaction.
If you want to discard the changes made in a transaction, you can use the ROLLBACK statement.
Related Article: Applying Aggregate Functions in PostgreSQL WHERE Clause
Best Practices for Using Temporary Tables in PostgreSQL
– Use temporary tables only when necessary: Temporary tables consume resources and can affect performance, so it’s important to use them only when necessary. Consider other options like subqueries or common table expressions if possible.
– Keep temporary tables small: To minimize the impact on performance, try to keep the size of temporary tables small. If a temporary table becomes too large, consider partitioning or optimizing the query to reduce its size.
– Use appropriate indexes: If you frequently query a temporary table, consider creating indexes on the columns used in the queries to improve performance.
– Drop temporary tables explicitly: Although temporary tables are automatically dropped at the end of a session or transaction, it’s good practice to drop them explicitly when they are no longer needed to free up resources.
– Be aware of transaction isolation levels: Temporary tables are only visible within the current session or transaction. If you need to access a temporary table from a different session or transaction, make sure to set the appropriate transaction isolation level.