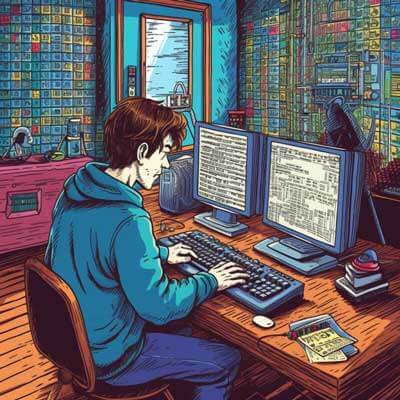
Table of Contents
What is SQL?
Structured Query Language (SQL) is a programming language that is used to manage and manipulate relational databases. It provides a standardized way to interact with databases, allowing users to perform tasks such as querying, inserting, updating, and deleting data. SQL is widely used in the field of data management and is supported by most relational database management systems (RDBMS).
Related Article: Merging Join and Where Clauses in SQL: A Tutorial
What is a SQL query?
In SQL, a query is a request for data or information from a database. It allows users to retrieve specific records or perform calculations on the data. SQL queries are written using the SELECT statement, which specifies the columns to be retrieved and the tables from which to retrieve the data. Queries can also include conditions, sorting instructions, and aggregation functions to further refine the results.
Here is an example of a simple SQL query that retrieves all records from a table named "employees":
SELECT * FROM employees;
This query will return all columns and rows from the "employees" table.
What is an inner join in SQL?
An inner join in SQL is used to combine records from two or more tables based on a related column between them. It returns only the records that have matching values in both tables. Inner joins are commonly used to retrieve data that is spread across multiple tables and needs to be consolidated into a single result set.
Here is an example of an inner join query:
SELECT customers.customer_id, customers.name, orders.order_id, orders.order_date FROM customers INNER JOIN orders ON customers.customer_id = orders.customer_id;
This query joins the "customers" table with the "orders" table based on the "customer_id" column. It retrieves the customer's ID and name from the "customers" table, and the order's ID and date from the "orders" table for the matching records.
What is an outer join in SQL?
An outer join in SQL is used to combine records from two or more tables based on a related column between them, including unmatched records from one or both tables. It returns all records from one table and the matching records from the other table(s), filling in any missing values with NULL.
There are three types of outer joins: left outer join, right outer join, and full outer join.
Related Article: Extracting the Month from a Date in PostgreSQL
What is a left join in SQL?
A left join in SQL returns all records from the left table and the matching records from the right table. If there are no matching records in the right table, NULL values are included for the columns of the right table.
Here is an example of a left join query:
SELECT customers.customer_id, customers.name, orders.order_id, orders.order_date FROM customers LEFT JOIN orders ON customers.customer_id = orders.customer_id;
This query joins the "customers" table with the "orders" table based on the "customer_id" column. It retrieves the customer's ID and name from the "customers" table, and the order's ID and date from the "orders" table for the matching records. If there are no matching records in the "orders" table, NULL values will be included for the order-related columns.
What is a right join in SQL?
A right join in SQL returns all records from the right table and the matching records from the left table. If there are no matching records in the left table, NULL values are included for the columns of the left table.
Here is an example of a right join query:
SELECT customers.customer_id, customers.name, orders.order_id, orders.order_date FROM customers RIGHT JOIN orders ON customers.customer_id = orders.customer_id;
This query joins the "customers" table with the "orders" table based on the "customer_id" column. It retrieves the customer's ID and name from the "customers" table, and the order's ID and date from the "orders" table for the matching records. If there are no matching records in the "customers" table, NULL values will be included for the customer-related columns.
What is a primary key in a database?
A primary key in a database is a column or a combination of columns that uniquely identifies each record in a table. It ensures the integrity and uniqueness of the data within the table. A primary key must have the following characteristics:
- It must contain a unique value for each record.
- It cannot contain NULL values.
- It should be immutable, meaning it should not change over time.
- It should be simple and easy to understand.
Primary keys are typically used for indexing and enforcing referential integrity in a database.
Here is an example of creating a table with a primary key:
CREATE TABLE customers ( customer_id INT PRIMARY KEY, name VARCHAR(50), email VARCHAR(100) );
In this example, the "customer_id" column is defined as the primary key for the "customers" table. It ensures that each customer has a unique ID.
What is a foreign key in a database?
A foreign key in a database is a column or a combination of columns that establishes a relationship between two tables. It references the primary key of another table, creating a link between them. Foreign keys are used to enforce referential integrity, ensuring that the data in the related tables remains consistent.
When a foreign key is defined, it restricts the values that can be inserted into the column to those that already exist in the referenced table's primary key.
Here is an example of creating a table with a foreign key:
CREATE TABLE orders ( order_id INT PRIMARY KEY, customer_id INT, order_date DATE, FOREIGN KEY (customer_id) REFERENCES customers(customer_id) );
In this example, the "orders" table has a foreign key "customer_id" that references the primary key "customer_id" of the "customers" table. It ensures that each order is associated with a valid customer ID.
Related Article: Exploring Left to Right SQL Joins in Databases
Analyzing the effect of SQL join on records
When performing SQL joins, the effect on the resulting records depends on the type of join used. Each type of join has a different effect on the records returned.
- Inner Join: Returns only the matching records from both tables. Records without a match are excluded from the result set.
- Outer Join: Returns all records from one table and the matching records from the other table(s). If there is no match, NULL values are included for the missing records.
- Left Join: Returns all records from the left table and the matching records from the right table. If there is no match, NULL values are included for the missing records from the right table.
- Right Join: Returns all records from the right table and the matching records from the left table. If there is no match, NULL values are included for the missing records from the left table.
The choice of join type depends on the desired outcome and the relationship between the tables. Inner joins are commonly used to retrieve records with matching values, while outer joins are used to include unmatched records as well.
Code snippet: Implementing an inner join in SQL
Here is an example of implementing an inner join in SQL:
SELECT customers.customer_id, customers.name, orders.order_id, orders.order_date FROM customers INNER JOIN orders ON customers.customer_id = orders.customer_id;
This query joins the "customers" table with the "orders" table based on the "customer_id" column. It retrieves the customer's ID and name from the "customers" table, and the order's ID and date from the "orders" table for the matching records.
Code snippet: Implementing an outer join in SQL
Here is an example of implementing an outer join in SQL:
SELECT customers.customer_id, customers.name, orders.order_id, orders.order_date FROM customers LEFT JOIN orders ON customers.customer_id = orders.customer_id;
This query performs a left outer join between the "customers" table and the "orders" table based on the "customer_id" column. It retrieves the customer's ID and name from the "customers" table, and the order's ID and date from the "orders" table for the matching records. If there are no matching records in the "orders" table, NULL values will be included for the order-related columns.
Code snippet: Implementing a left join in SQL
Here is an example of implementing a left join in SQL:
SELECT customers.customer_id, customers.name, orders.order_id, orders.order_date FROM customers LEFT JOIN orders ON customers.customer_id = orders.customer_id;
This query performs a left join between the "customers" table and the "orders" table based on the "customer_id" column. It retrieves the customer's ID and name from the "customers" table, and the order's ID and date from the "orders" table for the matching records. If there are no matching records in the "orders" table, NULL values will be included for the order-related columns.
Related Article: How to Truncate Tables in PostgreSQL
Code snippet: Implementing a right join in SQL
Here is an example of implementing a right join in SQL:
SELECT customers.customer_id, customers.name, orders.order_id, orders.order_date FROM customers RIGHT JOIN orders ON customers.customer_id = orders.customer_id;
This query performs a right join between the "customers" table and the "orders" table based on the "customer_id" column. It retrieves the customer's ID and name from the "customers" table, and the order's ID and date from the "orders" table for the matching records. If there are no matching records in the "customers" table, NULL values will be included for the customer-related columns.
Comparing inner join and outer join in SQL
Inner joins and outer joins in SQL have different effects on the resulting records:
- Inner Join: Returns only the matching records from both tables. Records without a match are excluded from the result set.
- Outer Join: Returns all records from one table and the matching records from the other table(s). If there is no match, NULL values are included for the missing records.
The choice between an inner join and an outer join depends on the desired outcome. If you only want the matching records, an inner join is appropriate. If you want to include unmatched records as well, an outer join is needed.
Understanding primary keys in a database
A primary key in a database is a column or a combination of columns that uniquely identifies each record in a table. It is used to ensure the integrity and uniqueness of the data within the table. A primary key has the following characteristics:
- It must contain a unique value for each record.
- It cannot contain NULL values.
- It should be immutable, meaning it should not change over time.
- It should be simple and easy to understand.
Primary keys are typically used for indexing and enforcing referential integrity in a database. They allow for efficient querying and joining of tables based on the primary key.
Understanding foreign keys in a database
A foreign key in a database is a column or a combination of columns that establishes a relationship between two tables. It references the primary key of another table, creating a link between them. Foreign keys are used to enforce referential integrity, ensuring that the data in the related tables remains consistent.
When a foreign key is defined, it restricts the values that can be inserted into the column to those that already exist in the referenced table's primary key.
Foreign keys play a crucial role in maintaining the integrity of the data by enforcing relationships between tables. They allow for cascading updates and deletions, ensuring that changes in one table are reflected in related tables.
Related Article: Tutorial: Role of PostgreSQL Rollup in Databases
Additional Resources
- What is the purpose of SQL in databases?