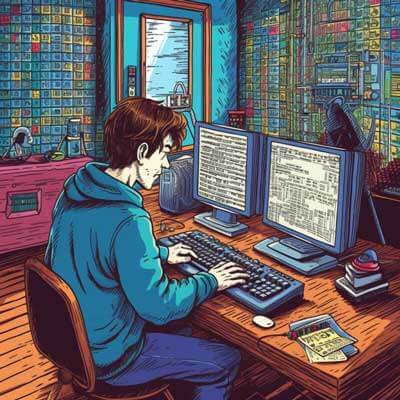
Table of Contents
Join Syntax in SQL
SQL provides a standard syntax for performing joins between tables. The syntax varies slightly depending on the type of join you want to perform.
The general syntax for joining two tables is as follows:
SELECT column1, column2, ... FROM table1 JOIN_TYPE JOIN table2 ON table1.column = table2.column;
Here, JOIN_TYPE can be replaced with the specific join type you want to use, such as INNER JOIN, LEFT JOIN, RIGHT JOIN, or FULL OUTER JOIN.
The ON keyword is used to specify the condition on which the join should be performed. It specifies the column or columns that should be used to match rows between the tables.
It's important to note that the join condition should be carefully chosen to ensure accurate and meaningful results. The columns used for joining should have the same datatype and represent the same or related information.
Related Article: How to Use Alias Field Joining in SQL
Performing Join Operations in SQL
Performing join operations in SQL involves combining data from multiple tables. By using the appropriate join type and join condition, we can retrieve the desired data.
To perform a join operation, follow these steps:
1. Identify the tables that you want to join based on the relationship between them. Determine which table will be the left table and which will be the right table.
2. Understand the relationship between the tables and identify the column or columns that should be used for joining. This could be a primary key and a foreign key, or any other related columns.
3. Choose the appropriate join type based on the desired result. Inner join, left join, right join, full outer join, or cross join can be used depending on the requirement.
4. Write the SQL query using the join syntax. Specify the tables to join, the join type, and the join condition using the ON keyword.
5. Execute the query and review the result. Make sure the join operation has produced the expected output.
6. If necessary, refine the join condition or modify the query to include additional filters or aggregations to further refine the result.
Joining Three Tables in SQL
Joining three tables in SQL follows the same principles as joining two tables. We can extend the join syntax to include a third table and specify the appropriate join conditions.
The general syntax for joining three tables is as follows:
SELECT column1, column2, ... FROM table1 JOIN_TYPE JOIN table2 ON table1.column = table2.column JOIN_TYPE JOIN table3 ON table2.column = table3.column;
Here, we join the first two tables using the specified join type and condition. Then, we join the resultant table with the third table using another join type and condition.
Let's consider an example of three tables: "orders", "customers", and "products". The "orders" table has columns for order_id, customer_id, and product_id. The "customers" table has columns for customer_id, customer_name, and customer_address. The "products" table has columns for product_id, product_name, and product_price. We can join these three tables to get the order details along with the customer and product information.
SELECT orders.order_id, orders.order_date, customers.customer_name, products.product_name FROM orders INNER JOIN customers ON orders.customer_id = customers.customer_id INNER JOIN products ON orders.product_id = products.product_id;
This query will return the order_id, order_date, customer_name, and product_name for all orders.
Joining three tables can become complex as the number of tables increases. It's important to carefully define the join conditions and choose the appropriate join types to ensure accurate and meaningful results.
SQL Join Examples
Let's explore some examples of SQL joins to better understand their usage and functionality.
Example 1: Inner Join
Consider two tables, "employees" and "departments". The "employees" table has columns for employee_id, employee_name, and department_id. The "departments" table has columns for department_id and department_name. We can join these two tables based on the department_id column to get the employee details along with the department information.
SELECT employees.employee_id, employees.employee_name, departments.department_name FROM employees INNER JOIN departments ON employees.department_id = departments.department_id;
This query will return the employee_id, employee_name, and department_name for all employees.
Example 2: Left Join
Continuing with the previous example, let's say we want to retrieve all departments along with their employees. If a department doesn't have any employees, the employee details should be NULL.
SELECT employees.employee_id, employees.employee_name, departments.department_name FROM employees LEFT JOIN departments ON employees.department_id = departments.department_id;
This query will return all employees, along with their employee_id, employee_name, and department_name if available. If a department doesn't have any employees, the department_name will be NULL.
These examples demonstrate the usage of inner join and left join. By combining different join types and conditions, we can retrieve the desired data and establish relationships between tables.
Related Article: How to Check and Change Postgresql's Default Port
Understanding Join Algorithms
When performing joins in SQL, the database engine uses various algorithms to efficiently process the join operation. Understanding these join algorithms can help us optimize our queries and improve performance.
Here are some commonly used join algorithms:
1. Nested Loop Join: This algorithm is suitable for small tables or when there is no index available for the join columns. It loops through each row of the outer table and matches it with the corresponding rows in the inner table.
2. Hash Join: This algorithm is suitable for large tables or when there is a suitable index available for the join columns. It builds a hash table from the smaller table and uses it to probe the larger table for matches.
3. Merge Join: This algorithm is suitable when both tables are sorted on the join columns. It compares the values of the join columns in both tables and merges the matching rows.
4. Sort-Merge Join: This algorithm is similar to the merge join but requires sorting both tables on the join columns before merging.
The database engine automatically chooses the most appropriate join algorithm based on various factors such as table sizes, available indexes, and system resources. However, we can influence the choice of join algorithm by optimizing our queries and providing relevant indexes.
Optimizing SQL Joins
Optimizing SQL joins is essential for improving query performance and reducing the execution time. By following some best practices and techniques, we can optimize our join operations.
Here are some tips for optimizing SQL joins:
1. Use the appropriate join type: Choose the join type that best suits your requirements. Inner join, left join, right join, full outer join, and cross join have different purposes and usage scenarios.
2. Select the right columns: Only select the columns you need in the result set. Avoid selecting unnecessary columns, especially when dealing with large tables.
3. Create appropriate indexes: Indexes on the join columns can significantly improve join performance. Analyze your query execution plans and consider creating indexes on frequently joined columns.
4. Use join hints: In some cases, the database engine may not choose the optimal join algorithm. Use join hints to explicitly specify the join algorithm and enforce your preferred approach.
5. Optimize the join condition: Ensure that the join condition is specific and precise. Avoid using complex expressions or functions in the join condition, as they can impact performance.
6. Limit the result set: If you only need a subset of the joined data, consider adding appropriate filters or conditions to limit the result set. This can reduce the amount of data processed and improve performance.
7. Monitor query performance: Regularly monitor the performance of your join queries. Use query execution plans, profiling tools, and database statistics to identify bottlenecks and areas for optimization.
Joining Tables with Filters
When joining tables in SQL, it's common to include additional filters or conditions to further refine the result set. By combining join conditions with filters, we can retrieve specific subsets of data from multiple tables.
To join tables with filters, follow these steps:
1. Identify the tables you want to join based on their relationships.
2. Determine the columns that should be used for joining. These could be primary keys, foreign keys, or any other related columns.
3. Choose the appropriate join type based on the desired result.
4. Write the SQL query using the join syntax, including the join type and join condition.
5. Add additional filters or conditions using the WHERE clause to further refine the result set.
6. Execute the query and review the result. Make sure the join operation and filters have produced the expected output.
Let's consider an example to demonstrate joining tables with filters. Suppose we have two tables: "orders" and "customers". The "orders" table has columns for order_id, customer_id, and order_date, while the "customers" table has columns for customer_id, customer_name, and customer_address. We want to retrieve all orders placed by customers in a specific city.
SELECT orders.order_id, orders.order_date, customers.customer_name FROM orders INNER JOIN customers ON orders.customer_id = customers.customer_id WHERE customers.customer_city = 'New York';
This query joins the "orders" and "customers" tables based on the customer_id column and applies a filter on the customer_city column to only include customers from New York. The result will include the order_id, order_date, and customer_name for all orders placed by customers in New York.
Joining tables with filters allows us to narrow down our result set and retrieve specific subsets of data based on our requirements.
Joining Tables with Aggregates
When joining tables in SQL, we can also perform aggregation operations on the joined data. Aggregates allow us to summarize and analyze data from multiple tables, providing valuable insights.
To join tables with aggregates, follow these steps:
1. Identify the tables you want to join based on their relationships.
2. Determine the columns that should be used for joining. These could be primary keys, foreign keys, or any other related columns.
3. Choose the appropriate join type based on the desired result.
4. Write the SQL query using the join syntax, including the join type and join condition.
5. Use aggregate functions such as SUM, COUNT, AVG, MAX, or MIN to perform calculations on the joined data.
6. Group the result set by one or more columns using the GROUP BY clause.
7. Execute the query and review the result. Make sure the join operation and aggregates have produced the expected output.
Let's consider an example to demonstrate joining tables with aggregates. Suppose we have two tables: "products" and "order_items". The "products" table has columns for product_id, product_name, and product_price, while the "order_items" table has columns for order_id, product_id, and quantity. We want to retrieve the total quantity of each product sold.
SELECT products.product_name, SUM(order_items.quantity) AS total_quantity FROM products INNER JOIN order_items ON products.product_id = order_items.product_id GROUP BY products.product_name;
This query joins the "products" and "order_items" tables based on the product_id column, calculates the sum of the quantity for each product, and groups the result by the product_name column. The result will include the product_name and the total_quantity for each product.
Joining tables with aggregates allows us to perform calculations and obtain meaningful insights from the combined data.
Related Article: Exploring Natural Join in PostgreSQL Databases
Handling Null Values in Joins
When joining tables in SQL, it's important to consider the presence of NULL values in the join columns. NULL values can affect the join operation and produce unexpected results if not handled properly.
To handle NULL values in joins, follow these guidelines:
1. Understand the data: Identify columns that can contain NULL values and consider their impact on the join operation.
2. Use appropriate join types: Different join types handle NULL values differently. Choose the join type that best suits your requirements.
3. Handle NULL values explicitly: If you want to include or exclude NULL values in the join, specify the condition explicitly in the join condition or using additional filters.
4. Use IS NULL or IS NOT NULL: When comparing columns with NULL values, use the IS NULL or IS NOT NULL operators instead of the equality operator (=).
5. Consider NULL-safe comparisons: Some database systems provide NULL-safe comparison operators that handle NULL values in a consistent manner. Use these operators if available.
Let's consider an example to demonstrate handling NULL values in joins. Suppose we have two tables: "orders" and "customers". The "orders" table has columns for order_id, customer_id, and order_date, while the "customers" table has columns for customer_id, customer_name, and customer_address. Some orders may not have a corresponding customer_id, resulting in NULL values.
SELECT orders.order_id, orders.order_date, customers.customer_name FROM orders LEFT JOIN customers ON orders.customer_id = customers.customer_id WHERE customers.customer_id IS NOT NULL;
This query performs a left join between the "orders" and "customers" tables, ensuring that only orders with a valid customer_id are included in the result. The WHERE clause filters out NULL values from the customer_id column.
Joining Tables with Alias
When joining tables in SQL, it's common to use table aliases to simplify the query and improve readability. Table aliases provide a shorthand notation for referring to tables and their columns.
To join tables with aliases, follow these steps:
1. Identify the tables you want to join based on their relationships.
2. Determine the columns that should be used for joining. These could be primary keys, foreign keys, or any other related columns.
3. Choose the appropriate join type based on the desired result.
4. Assign table aliases to each table using the AS keyword.
5. Write the SQL query using the join syntax, including the join type, join condition, and table aliases.
6. Use the table aliases to refer to the columns in the select list, join condition, and other parts of the query.
7. Execute the query and review the result. Make sure the join operation and aliases have produced the expected output.
Let's consider an example to demonstrate joining tables with aliases. Suppose we have two tables: "employees" and "departments". The "employees" table has columns for employee_id, employee_name, and department_id, while the "departments" table has columns for department_id and department_name. We want to retrieve the employee details along with the department information.
SELECT emp.employee_id, emp.employee_name, dept.department_name FROM employees AS emp INNER JOIN departments AS dept ON emp.department_id = dept.department_id;
This query joins the "employees" and "departments" tables using aliases emp and dept. The result includes the employee_id, employee_name, and department_name for all employees.
Using table aliases in joins can simplify the query and make it easier to read and understand.
Joining Tables with Subqueries
When joining tables in SQL, it's sometimes necessary to use subqueries to retrieve the desired data. Subqueries allow us to perform intermediate calculations or retrieve data from a subset of the tables before joining them.
To join tables with subqueries, follow these steps:
1. Identify the tables you want to join based on their relationships.
2. Determine the columns that should be used for joining. These could be primary keys, foreign keys, or any other related columns.
3. Choose the appropriate join type based on the desired result.
4. Write subqueries to retrieve the required data from one or more tables.
5. Use the subqueries as virtual tables in the join operation, specifying the join condition between the subqueries.
6. Write the SQL query using the join syntax, including the join type, join condition, and subqueries.
7. Execute the query and review the result. Make sure the join operation and subqueries have produced the expected output.
Let's consider an example to demonstrate joining tables with subqueries. Suppose we have three tables: "orders", "customers", and "products". The "orders" table has columns for order_id, customer_id, and order_date, the "customers" table has columns for customer_id, customer_name, and customer_address, and the "products" table has columns for product_id, product_name, and product_price. We want to retrieve the order details along with the customer and product information.
SELECT orders.order_id, orders.order_date, customers.customer_name, products.product_name FROM orders INNER JOIN ( SELECT customer_id, customer_name FROM customers ) AS customers ON orders.customer_id = customers.customer_id INNER JOIN ( SELECT product_id, product_name FROM products ) AS products ON orders.product_id = products.product_id;
In this query, we use subqueries to retrieve the customer_id and customer_name from the "customers" table and the product_id and product_name from the "products" table. We then join these subqueries with the "orders" table using appropriate join conditions.
Joining tables with subqueries allows us to perform intermediate calculations or retrieve data from a subset of the tables before joining them, providing flexibility and control over the join operation.
Joining Tables with Functions
When joining tables in SQL, we can also use functions to manipulate the data or perform calculations on the joined columns. Functions allow us to transform the data during the join operation, providing additional flexibility and control.
To join tables with functions, follow these steps:
1. Identify the tables you want to join based on their relationships.
2. Determine the columns that should be used for joining. These could be primary keys, foreign keys, or any other related columns.
3. Choose the appropriate join type based on the desired result.
4. Write SQL functions or built-in functions to manipulate or calculate the joined columns.
5. Use the functions in the join condition or select list to apply the desired transformations.
6. Write the SQL query using the join syntax, including the join type, join condition, and functions.
7. Execute the query and review the result. Make sure the join operation and functions have produced the expected output.
Let's consider an example to demonstrate joining tables with functions. Suppose we have two tables: "employees" and "departments". The "employees" table has columns for employee_id, employee_name, and department_id, while the "departments" table has columns for department_id and department_name. We want to retrieve the employee details along with the uppercase department names.
SELECT emp.employee_id, emp.employee_name, UPPER(dept.department_name) AS department_name FROM employees AS emp INNER JOIN departments AS dept ON emp.department_id = dept.department_id;
This query joins the "employees" and "departments" tables using aliases emp and dept. The result includes the employee_id, employee_name, and uppercase department_name for all employees.
Using functions in joins allows us to manipulate and transform data during the join operation, providing additional flexibility and control.
Related Article: Is ANSI SQL Standard Compatible with Outer Joins?
Joining Tables with Views
When joining tables in SQL, we can also use views to simplify the join operation and improve query readability. Views are virtual tables based on the result of a query, and they can be used just like physical tables in SQL statements.
To join tables with views, follow these steps:
1. Create views for the tables you want to join. Views can be created using the CREATE VIEW statement.
2. Identify the views you want to join based on their relationships.
3. Determine the columns that should be used for joining. These could be primary keys, foreign keys, or any other related columns.
4. Choose the appropriate join type based on the desired result.
5. Write the SQL query using the join syntax, including the join type, join condition, and views.
6. Execute the query and review the result. Make sure the join operation and views have produced the expected output.
Let's consider an example to demonstrate joining tables with views. Suppose we have two tables: "employees" and "departments". The "employees" table has columns for employee_id, employee_name, and department_id, while the "departments" table has columns for department_id and department_name. We can create views for these tables to simplify the join operation.
CREATE VIEW vw_employees AS SELECT employee_id, employee_name, department_id FROM employees; CREATE VIEW vw_departments AS SELECT department_id, department_name FROM departments; SELECT emp.employee_id, emp.employee_name, dept.department_name FROM vw_employees AS emp INNER JOIN vw_departments AS dept ON emp.department_id = dept.department_id;
In this example, we create views vw_employees and vw_departments for the "employees" and "departments" tables, respectively. We then join these views using the appropriate join conditions.
Joining tables with views can simplify the join operation, improve query readability, and provide a modular approach to SQL development.
Joining Tables with CTEs
When joining tables in SQL, we can also use Common Table Expressions (CTEs) to simplify the join operation and improve query readability. CTEs allow us to define temporary result sets that can be referenced multiple times in a query.
To join tables with CTEs, follow these steps:
1. Define CTEs for the tables you want to join. CTEs can be created using the WITH keyword.
2. Identify the CTEs you want to join based on their relationships.
3. Determine the columns that should be used for joining. These could be primary keys, foreign keys, or any other related columns.
4. Choose the appropriate join type based on the desired result.
5. Write the SQL query using the join syntax, including the join type, join condition, and CTEs.
6. Execute the query and review the result. Make sure the join operation and CTEs have produced the expected output.
Let's consider an example to demonstrate joining tables with CTEs. Suppose we have two tables: "employees" and "departments". The "employees" table has columns for employee_id, employee_name, and department_id, while the "departments" table has columns for department_id and department_name. We can define CTEs for these tables to simplify the join operation.
WITH cte_employees AS ( SELECT employee_id, employee_name, department_id FROM employees ), cte_departments AS ( SELECT department_id, department_name FROM departments ) SELECT emp.employee_id, emp.employee_name, dept.department_name FROM cte_employees AS emp INNER JOIN cte_departments AS dept ON emp.department_id = dept.department_id;
In this example, we define CTEs cte_employees and cte_departments for the "employees" and "departments" tables, respectively. We then join these CTEs using the appropriate join conditions.
Joining tables with CTEs can simplify the join operation, improve query readability, and provide a modular approach to SQL development.
Joining Tables with Temp Tables
When joining tables in SQL, we can also use temporary tables to simplify the join operation and improve query performance. Temporary tables are created and used within the scope of a single session or transaction.
To join tables with temporary tables, follow these steps:
1. Create temporary tables for the tables you want to join. Temporary tables can be created using the CREATE TEMPORARY TABLE statement.
2. Populate the temporary tables with the data from the original tables. This can be done using INSERT INTO statements.
3. Identify the temporary tables you want to join based on their relationships.
4. Determine the columns that should be used for joining. These could be primary keys, foreign keys, or any other related columns.
5. Choose the appropriate join type based on the desired result.
6. Write the SQL query using the join syntax, including the join type, join condition, and temporary tables.
7. Execute the query and review the result. Make sure the join operation and temporary tables have produced the expected output.
8. Drop the temporary tables to free up resources. This can be done using the DROP TABLE statement.
Let's consider an example to demonstrate joining tables with temporary tables. Suppose we have two tables: "employees" and "departments". The "employees" table has columns for employee_id, employee_name, and department_id, while the "departments" table has columns for department_id and department_name. We can create temporary tables for these tables to simplify the join operation.
CREATE TEMPORARY TABLE temp_employees AS SELECT employee_id, employee_name, department_id FROM employees; CREATE TEMPORARY TABLE temp_departments AS SELECT department_id, department_name FROM departments; SELECT emp.employee_id, emp.employee_name, dept.department_name FROM temp_employees AS emp INNER JOIN temp_departments AS dept ON emp.department_id = dept.department_id; DROP TABLE temp_employees; DROP TABLE temp_departments;
In this example, we create temporary tables temp_employees and temp_departments for the "employees" and "departments" tables, respectively. We then join these temporary tables using the appropriate join conditions. Finally, we drop the temporary tables to free up resources.
Joining tables with temporary tables can simplify the join operation, improve query performance, and provide a temporary storage solution for intermediate results.
Joining Tables with Indexes
When joining tables in SQL, it's important to consider the presence of indexes on the join columns. Indexes can significantly improve join performance by providing efficient access to the data.
To join tables with indexes, follow these guidelines:
1. Identify the columns that are used for joining. These could be primary keys, foreign keys, or any other related columns.
2. Analyze the query execution plan to determine if appropriate indexes are being used.
3. Create indexes on the join columns if necessary. Indexes can be created using the CREATE INDEX statement.
4. Monitor the query performance and analyze the query execution plan to ensure that the indexes are being utilized effectively.
5. Consider the overall performance impact of the indexes. Indexes improve join performance but can slow down insert, update, and delete operations.
Let's consider an example to demonstrate joining tables with indexes. Suppose we have two tables: "employees" and "departments". The "employees" table has columns for employee_id, employee_name, and department_id, while the "departments" table has columns for department_id and department_name. We want to join these tables based on the department_id column.
CREATE INDEX idx_employees_department_id ON employees (department_id); CREATE INDEX idx_departments_department_id ON departments (department_id); SELECT emp.employee_id, emp.employee_name, dept.department_name FROM employees AS emp INNER JOIN departments AS dept ON emp.department_id = dept.department_id; DROP INDEX idx_employees_department_id ON employees; DROP INDEX idx_departments_department_id ON departments;
In this example, we create indexes idx_employees_department_id and idx_departments_department_id on the department_id columns of the "employees" and "departments" tables, respectively. These indexes improve the join performance by providing efficient access to the data. Finally, we drop the indexes to free up resources.
Joining tables with indexes can significantly improve join performance and optimize query execution.
Related Article: Tutorial: Nested SQL Joins in Databases
Troubleshooting Join Issues
When joining tables in SQL, it's common to encounter issues that can affect the join operation and produce unexpected results. Troubleshooting these issues requires a systematic approach to identify and resolve the problems.
Here are some common join issues and their solutions:
1. Incorrect join condition: Verify that the join condition is correct and ensures accurate matching of rows between the tables. Check for typos, missing columns, or incorrect column names.
2. Data type mismatch: Ensure that the join columns have the same data type. Data type mismatches can prevent accurate matching of rows and produce unexpected results.
3. Null values in join columns: Handle NULL values appropriately in the join condition. Use IS NULL or IS NOT NULL operators to compare NULL values.
4. Missing or incomplete data: Check if any necessary data is missing or incomplete in the join columns. Missing data can lead to incomplete or inaccurate join results.
5. Inefficient join algorithm: Analyze the query execution plan and identify if the database engine is using the most efficient join algorithm. Consider using join hints or creating appropriate indexes to optimize the join operation.
6. Large result set: Ensure that the join operation doesn't produce an excessively large result set. Limit the result set using filters or conditions to improve query performance.
7. Insufficient system resources: Check if the system has sufficient resources to perform the join operation. Insufficient memory or disk space can lead to performance issues or failures.
8. Locking and blocking: If multiple users or processes are accessing the tables simultaneously, locking and blocking issues may occur. Ensure proper concurrency control mechanisms are in place to avoid conflicts.
Best Practices for SQL Joins
When joining tables in SQL, it's important to follow best practices to ensure efficient and accurate results. By adhering to these best practices, we can optimize our join operations and improve query performance.
Here are some best practices for SQL joins:
1. Understand the data: Thoroughly analyze the data and understand the relationships between the tables. This will help in choosing the appropriate join type and condition.
2. Choose the right join type: Select the join type that best suits your requirements. Inner join, left join, right join, full outer join, or cross join have different purposes and usage scenarios.
3. Use appropriate indexes: Identify the join columns and create indexes on them if necessary. Indexes can significantly improve join performance by providing efficient access to the data.
4. Optimize the join condition: Ensure that the join condition is specific and precise. Avoid using complex expressions or functions in the join condition, as they can impact performance.
5. Limit the result set: If you only need a subset of the joined data, consider adding appropriate filters or conditions to limit the result set. This can reduce the amount of data processed and improve performance.
6. Monitor query performance: Regularly monitor the performance of your join queries. Use query execution plans, profiling tools, and database statistics to identify bottlenecks and areas for optimization.
7. Test with sample data: Before running join queries on large datasets, test them with sample data to ensure accuracy and performance. This will help identify any issues or inefficiencies early on.
8. Document the join conditions: Document the join conditions and their purpose for future reference. This will help in understanding and maintaining the queries.
9. Use table aliases: Assign table aliases to improve query readability and reduce typing. Table aliases provide a shorthand notation for referring to tables and their columns.
10. Validate the join results: Cross-check the join results against the expected output to ensure accuracy. Compare the joined data with the original tables to validate the join operation.
SQL Join Types
When working with relational databases, it is common to have data spread across multiple tables. SQL joins allow us to combine data from two or more tables based on a common column. There are several types of SQL joins, each serving a different purpose. Let's explore each join type in detail:
Inner Join Explained
The inner join is the most commonly used type of join. It returns only the rows that have matching values in both tables based on the specified column. Here's the syntax for an inner join:
SELECT column1, column2, ... FROM table1 INNER JOIN table2 ON table1.column = table2.column;
Let's consider an example to better understand the inner join. Suppose we have two tables: "orders" and "customers". The "orders" table has columns for order_id, customer_id, and order_date, while the "customers" table has columns for customer_id, customer_name, and customer_address. We can join these two tables based on the customer_id column to get the order details along with the customer information.
SELECT orders.order_id, orders.order_date, customers.customer_name FROM orders INNER JOIN customers ON orders.customer_id = customers.customer_id;
This query will return the order_id, order_date, and customer_name for all orders.
Related Article: Creating a Bash Script for a MySQL Database Backup
Left Join Explained
The left join, also known as the left outer join, returns all rows from the left table and the matching rows from the right table. If there are no matches, it returns NULL values for the columns of the right table. Here's the syntax for a left join:
SELECT column1, column2, ... FROM table1 LEFT JOIN table2 ON table1.column = table2.column;
Let's continue with our previous example of the "orders" and "customers" tables. If we use a left join and there are orders without corresponding customer information, those orders will still be included in the result, with NULL values for customer_name.
SELECT orders.order_id, orders.order_date, customers.customer_name FROM orders LEFT JOIN customers ON orders.customer_id = customers.customer_id;
This query will return all orders, along with the order_id, order_date, and customer_name if available. If there is no customer information for an order, the customer_name will be NULL.
Right Join Explained
The right join, also known as the right outer join, is the opposite of the left join. It returns all rows from the right table and the matching rows from the left table. If there are no matches, it returns NULL values for the columns of the left table. Here's the syntax for a right join:
SELECT column1, column2, ... FROM table1 RIGHT JOIN table2 ON table1.column = table2.column;
Using the same example, if we perform a right join between the "orders" and "customers" tables, it will return all customer information, along with the order details if available. If there is no order information for a customer, the order_id and order_date will be NULL.
SELECT orders.order_id, orders.order_date, customers.customer_name FROM orders RIGHT JOIN customers ON orders.customer_id = customers.customer_id;
This query will return all customers, along with the order_id, order_date, and customer_name if available. If there are no orders for a customer, the order_id and order_date will be NULL.
Full Outer Join Explained
The full outer join combines the results of both the left and right joins. It returns all rows from both tables, with NULL values for columns where there is no match. Here's the syntax for a full outer join:
SELECT column1, column2, ... FROM table1 FULL OUTER JOIN table2 ON table1.column = table2.column;
In our example, if we perform a full outer join between the "orders" and "customers" tables, it will return all orders and customers, combining the information from both tables. If there is no order information for a customer or no customer information for an order, the respective columns will have NULL values.
SELECT orders.order_id, orders.order_date, customers.customer_name FROM orders FULL OUTER JOIN customers ON orders.customer_id = customers.customer_id;
This query will return all orders and customers, along with the order_id, order_date, and customer_name if available. If there is no order information for a customer or no customer information for an order, the respective columns will be NULL.
Cross Join Explained
The cross join, also known as the Cartesian join, returns the Cartesian product of the two tables. It combines each row from the first table with every row from the second table. The result is a large result set with all possible combinations. Here's the syntax for a cross join:
SELECT column1, column2, ... FROM table1 CROSS JOIN table2;
Let's consider an example of two tables, "colors" and "sizes". The "colors" table has columns for color_id and color_name, while the "sizes" table has columns for size_id and size_name. If we perform a cross join between these two tables, we will get all possible combinations of colors and sizes.
SELECT colors.color_name, sizes.size_name FROM colors CROSS JOIN sizes;
This query will return all possible combinations of colors and sizes. For example, if there are 5 colors and 3 sizes, the result will have 15 rows.
Related Article: Tutorial on SQL IN and NOT IN Operators in Databases
Additional Resources
- SQL Joins - w3schools.com